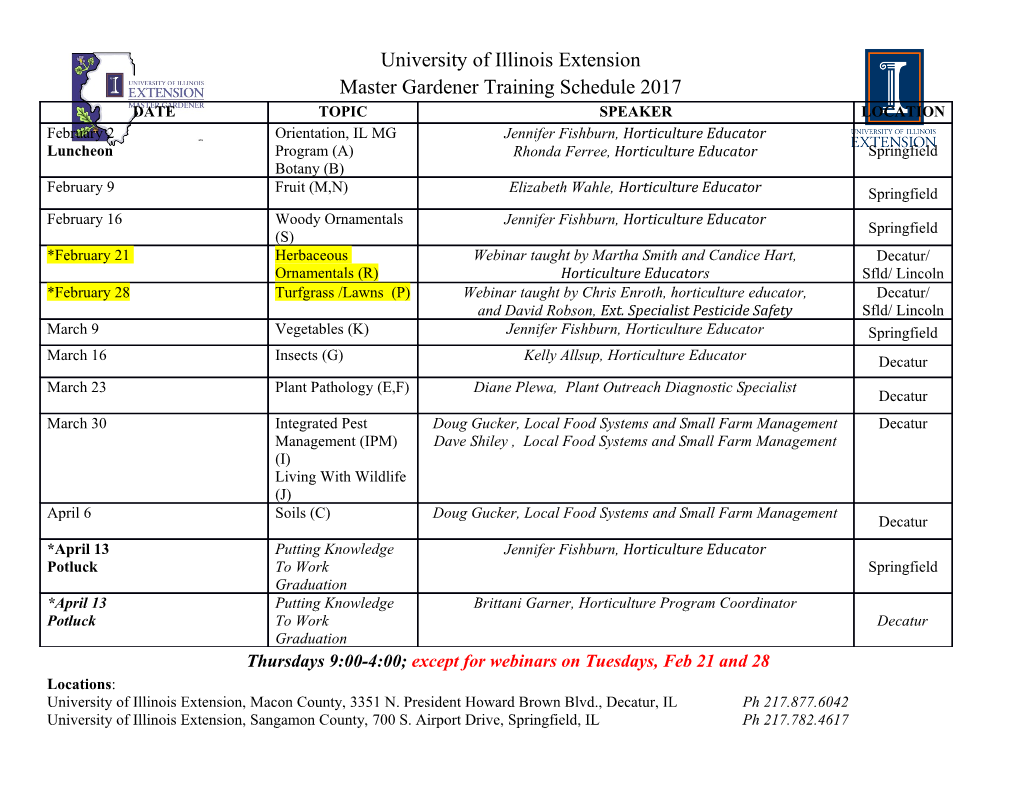
Monad P1 : Side Effects (1A) Young Won Lim 3/26/19 Copyright (c) 2016 - 2019 Young W. Lim. Permission is granted to copy, distribute and/or modify this document under the terms of the GNU Free Documentation License, Version 1.2 or any later version published by the Free Software Foundation; with no Invariant Sections, no Front-Cover Texts, and no Back-Cover Texts. A copy of the license is included in the section entitled "GNU Free Documentation License". Please send corrections (or suggestions) to [email protected]. This document was produced by using LibreOffice. Young Won Lim 3/26/19 Based on Variables and functions https://en.wikibooks.org/wiki/Haskell/Variables_and_functions Purity https://wiki.haskell.org/Functional_programming#Purity Side Effects (1A) Young Won Lim 3 3/26/19 Variables Imperative programming: ● variables as changeable locations in a computer's memory ● imperative programs explicitly commands (instructs) the computer what to do functional programming ● a way to think in higher-level mathematical terms ● defining how variables relate to one another ● the compiler will translate these functions and variables to instructions so that the computer can process. https://en.wikibooks.org/wiki/Haskell/Variables_and_functions Side Effects (1A) Young Won Lim 4 3/26/19 Haskell Language Features (I) Haskell Functional Programming (I) ● Immutability ● Recursive Definition : only in functions ● No Data Dependency https://en.wikibooks.org/wiki/Haskell/Variables_and_functions Side Effects (1A) Young Won Lim 5 3/26/19 Redefinition : not allowed imperative programming: r = 5 after setting r = 5 and then changing it to r = 2. r = 2 Hakell programming: r = 5 an error: "multiple declarations of r". within a given scope, a variable in Haskell r = 2 are defined only once and cannot change, like variables in mathematics. no mutation in Haskell https://en.wikibooks.org/wiki/Haskell/Variables_and_functions Side Effects (1A) Young Won Lim 6 3/26/19 Variables in a file Vars.hs Immutable: they can change only based on a = 100 the data we enter to run the program. r = 5 We cannot define r two ways in the same code, pi = 3.14159 but we could change the value by changing the file e = 2.7818 https://en.wikibooks.org/wiki/Haskell/Variables_and_functions Side Effects (1A) Young Won Lim 7 3/26/19 No Mutation *Main> r = 33 <interactive>:12:3: parse error on input ‘=’ No mutation, Immutable $ ghci GHCi, version 7.10.3: http://www.haskell.org/ghc/ :? for help Prelude> r = 333 <interactive>:2:3: parse error on input ‘=’ Prelude> let r = 33 let r = 33 https://en.wikibooks.org/wiki/Haskell/Variables_and_functions Side Effects (1A) Young Won Lim 8 3/26/19 Loading a variable definition file $ ghci :load Var1.hs GHCi, version 7.10.3: http://www.haskell.org/ghc/ :? for help Prelude> :load Var1.hs :load Var1.hs [1 of 1] Compiling Main ( var.hs, interpreted ) Ok, modules loaded: Main. definition with initialization *Main> r 5 Var1.hs file *Main> :t r r = 5 r :: Integer x = 1 *Main> y = 3.14 … *Main> :load Var2.hs Var2.hs file [1 of 1] Compiling Main ( var2.hs, interpreted ) r = 55 Ok, modules loaded: Main. x = 1 *Main> r y = 3.14 55 … https://en.wikibooks.org/wiki/Haskell/Variables_and_functions Side Effects (1A) Young Won Lim 9 3/26/19 Incrementing by one imperative programming: r = r + 1 incrementing the variable r (updating the value in memory) Hakell programming: No compound assignment like operations r = 3 r = r + 1 if r had been defined with any value beforehand, then r = r + 1 in Haskell would bring an error message. multiple definition not allowed the expression r = r + 1 is a recursive definition add1 x = x + 1 allowed in a function definition r = 3 r = add1 r https://en.wikibooks.org/wiki/Haskell/Variables_and_functions Side Effects (1A) Young Won Lim 10 3/26/19 Arguments and parameters of a function binding an argument and a parameter of a function add1 x = x + 1 add1 x = x + 1 101 x (parameter) r = 100 add1 100 100 (argument) add1 r r = add1 r https://en.wikibooks.org/wiki/Haskell/Variables_and_functions Side Effects (1A) Young Won Lim 11 3/26/19 Recursive Definition Hakell programming: a recursive definition of r a += b (a = a + b) recursive function a -= b (a = a – b) factorial 0 = 1 (defining it in terms of itself) a *= b (a = a * b) factorial n = n * factorial (n – 1) a /= b (a = a / b) non-recursive function No compound assignment like operations are allowed add1 x = x + 1 if a had been defined with any value beforehand, recursive definitions are allowed only in function definition then a = a + b in Haskell would multiply defined https://en.wikibooks.org/wiki/Haskell/Variables_and_functions Side Effects (1A) Young Won Lim 12 3/26/19 Simulating imperative codes The most primitive way of x = v is to use a function x = v taking x as a parameter, and pass the argument v to that function. i = s = 0; // sum 0..100 while (i <= 100) { s = s+i; i++; } return s; sum = f 0 0 -- the initial values where f i s | i <=100 = f (i+1) (s+i) -- increment i, augment s i = (i+1) | otherwise = s -- return s at the end s = (s+i) This code is not pretty functional programing code, but it is simulating imperative code https://stackoverflow.com/questions/43525193/how-can-i-re-assign-a-variable-in-a-function-in-haskell Side Effects (1A) Young Won Lim 13 3/26/19 No Data Dependency y = x * 2 x = 3 x = 3 y = x * 3 Hakell programming: because the values of variables do not change variables can be defined in any order no mandatory : "x being declared before y" https://en.wikibooks.org/wiki/Haskell/Variables_and_functions Side Effects (1A) Young Won Lim 14 3/26/19 Evaluation examples area 5 area r = pi * r^2 => { replace the LHS area r = ... by the RHS ... = pi * r^2 } pi * 5 ^ 2 pi = 3.141592653589793 => { replace pi by its value } 3.141592653589793 * 5 ^ 2 => { apply exponentiation (^) } 5^2 = 25 3.141592653589793 * 25 => { apply multiplication (*) } 3.141592653589793 * 25 = 78.53981633974483 78.53981633974483 https://en.wikibooks.org/wiki/Haskell/Variables_and_functions Side Effects (1A) Young Won Lim 15 3/26/19 Translation to instructions functional programming ● making the compiler translate functions and variables to the step-by-step instructions that the computer can process. LHS = RHS replace each function and variable with its definition LHS repeatedly replace the results until a single value remains. to apply or call a function means RHS to replace the LHS of its definition by its RHS. https://en.wikibooks.org/wiki/Haskell/Variables_and_functions Side Effects (1A) Young Won Lim 16 3/26/19 Scope Scope rules define the visibility rules for names in a programming language. What if you have references to a variable named k in different parts of the program? Do these refer to the same variable or to different ones? https://courses.cs.washington.edu/courses/cse341/03wi/imperative/scoping.html Side Effects (1A) Young Won Lim 17 3/26/19 Haskell Scope Most languages, including Haskell, are statically scoped. ● A block defines a new scope. invisible ● Variables can be declared in that scope, and are not visible from the outside. visible ● However, variables outside the scope (in enclosing scopes) are visible unless they are overridden. ● In Haskell, these scope rules also apply to the names of functions. Static scoping is also sometimes called lexical scoping. https://courses.cs.washington.edu/courses/cse341/03wi/imperative/scoping.html Side Effects (1A) Young Won Lim 18 3/26/19 Side Effects Definition a function or expression is said to have a side effect if it modifies some state outside its scope or has an observable interaction with its calling functions or the outside world besides returning a value. a particular function might ● modify a global variable or static variable ● modify one of its arguments ● raise an exception ● write data to a display or file ● read data from a keyboard or file ● call other side-effecting functions https://en.wikipedia.org/wiki/Side_effect_(computer_science) Side Effects (1A) Young Won Lim 19 3/26/19 Some Monad types to handle side effects State monad actions in State, Error, IO monad manages global variables have side effects Error monad enables exceptions IO monad handles interactions with the file system, and other resources outside the program the program itself has no side effects the action in monads does have side effects the functional nature of the program is maintained (pure, no side effects) https://blog.osteele.com/2007/12/overloading-semicolon/ Side Effects (1A) Young Won Lim 20 3/26/19 History, Order, and Context In the presence of side effects, a program's behaviour may depend on history; the order of evaluation matters. the context and histories imperative programming : frequent utilization of side effects. functional programming : side effects are rarely used. The lack of side effects makes it easier to do formal verifications of a program https://en.wikipedia.org/wiki/Side_effect_(computer_science) Side Effects (1A) Young Won Lim 21 3/26/19 Side Effects Examples in C int i, j; i = j = 3; i = (j = 3); // j = 3 returns 3, which then gets assigned to i // The assignment function returns 10 // which automatically casts to "true" // so the loop conditional always evaluates to true while (b = 10) { } https://en.wikipedia.org/wiki/Side_effect_(computer_science) Side Effects (1A) Young Won Lim 22 3/26/19 Haskell Language Features (II) Haskell Functional Programming (II) ● Pure Function ● Simple IO ● Laziness ● Sequencing https://en.wikibooks.org/wiki/Haskell/Variables_and_functions Side Effects (1A) Young Won Lim 23 3/26/19 Pure Languages Haskell is a pure language no side effects immutability programs are made of functions pure functions st1 = 10 that cannot change any global state or variables, they can only do some computations and return their results.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages73 Page
-
File Size-