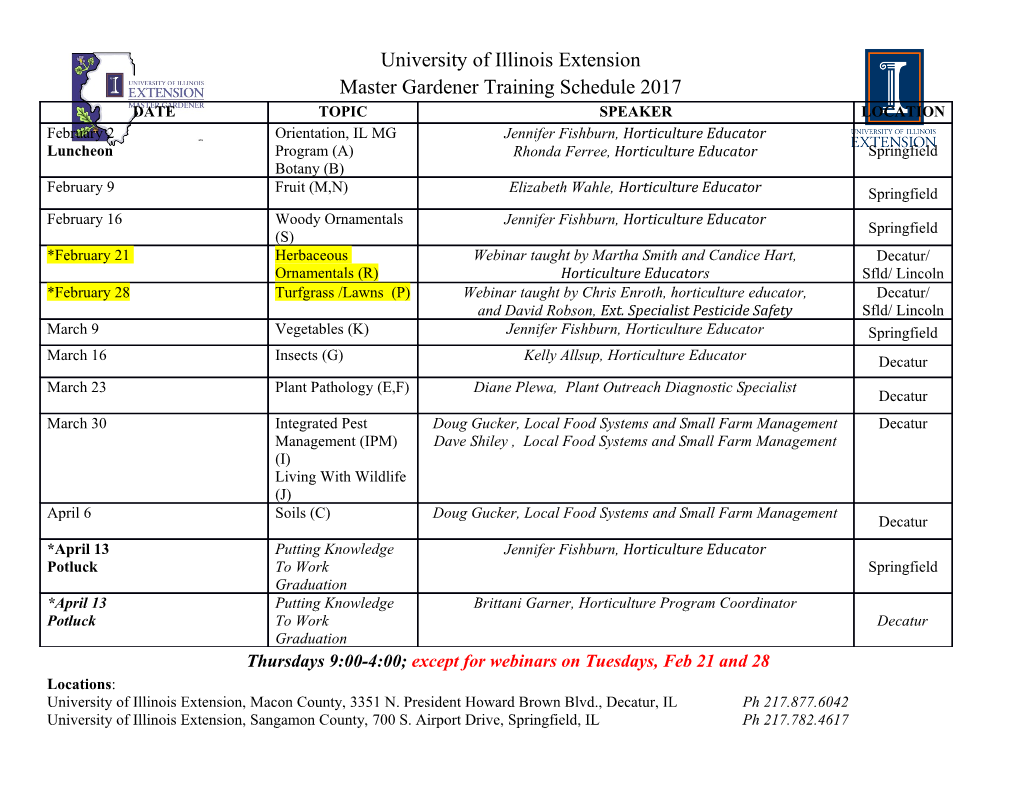
Transact-SQL Page 1 of 53 Chapter 9 Transact-SQL This chapter introduces the many options available for working with the SQL query language, along with the extensions and special features of Microsoft SQL Server/MSDE’s SQL dialect. The SQL standard was developed by the American National Standards Institute (ANSI) and the International Organization for Standardization (ISO). Today there are several standards whose names include the year in which they were released: SQL-89, SQL-92, and so on. The different standards vary in language functions and performance. Database vendors have fully or partially implemented the SQL standards in their products. Unfortunately, they have not yet agreed on a unified standard. Almost all have added their own extensions to the SQL implementations, so there are some significant differences among the SQL variations of individual products. Microsoft Access 2000 and Microsoft Access 2002 use Jet Engine 3.6—the database core for Access mdb databases—to support SQL-89 Level 1 with several extensions, as well as SQL-92 (albeit not completely) if you are using Jet Engine 4 with the ADO database interface. SQL Server/MSDE supports SQL-92, which for the most part conforms to the ANSI/ISO standard. The SQL language functions consist of two parts: The Data Definition Language (DDL) and the Data Manipulation Language (DML). With DDL, you can create, edit, and delete table structures and define indexes. The DML is used to query data or to edit and delete data. In Access, you usually only work with the DML, as tables and indexes are created with the tools available in Access. Access mdb users rarely have to deal with SQL directly because the Access user interface makes it possible to create queries interactively, and it then generates the actual SQL command itself. In Access projects, you can interactively create SQL commands for views only with the View Designer. All other commands in stored procedures must be entered manually, directly in SQL. You can use the Access support functions for .mdb files when creating SQL commands for your Access projects, as well. Simply create an .mdb file in parallel with your Access project, and then use the file to define table links for the tables on SQL Server/MSDE (select File, then Get External Data, then Link Tables). If you then create new queries, you can select View, then SQL in Design mode to view the SQL commands that the query you created is based on. Copy the SQL text to the Windows Clipboard and then paste it into a stored procedure, for example, in the Access project. Note that character strings in Access mdb databases are delineated by double quotation marks, whereas SQL Server/MSDE uses single quotation marks. Access mdb databases let you test SQL queries without first saving the query in the mdb database. By contrast, in Access projects, you must first save a query (with views as well as with stored procedures) before implementing it. You must do this in Access projects because saving the query transmits it to SQL Server/MSDE, which also checks the query to make sure the syntax is correct. If you want to perform ad hoc tests of your queries, you can use the frmSQL form, which is covered in Chapter 14, “Command Objects.” You can also use the SQL Server Query Analyzer (see Chapter 19, “SQL Server Tools”) if you are using SQL Server and not just MSDE. file://C:\Documents%20and%20Settings\richard.welke.ECI\Local%20Settings\Temp\~hh8... 3/21/2004 Transact-SQL Page 2 of 53 Select Queries with SELECT SELECT is the most important and most frequently used SQL command. It initiates select queries, which are queries that obtain data from tables and return a resultset. The following is the complex SELECT command’s general, if highly simplified, format: SELECT select_list [INTO new_table_] FROM table_source [WHERE search_condition] [GROUP BY group_by_expression] [HAVING search_condition] [ORDER BY order_expression [ASC │ DESC] ] The following simple query returns all movies in the table tblFilms. The asterisk serves as the variable for all columns in the table. SELECT * FROM tblFilms Specify the column names to select specific columns, as shown here: SELECT FilmNr, Filmtitle FROM tblFilms To avoid unnecessary server and network load, select only those columns that you actually need. If you use column names that contain spaces or special characters, you must enclose the names in angle brackets, as shown here, or apostrophes: SELECT [Field name with spaces] FROM Table The following is a better example that conforms to the ANSI SQL standard: SELECT "Field name with spaces" FROM Table Identity Column Output You can use the default column name IDENTITYCOL to output the column that you defined as the table’s identity column. For example, this command SELECT IDENTITYCOL, Filmtitle FROM tblFilms returns the FilmNr and the Filmtitle columns. The FilmNr column is defined as the identity column for file://C:\Documents%20and%20Settings\richard.welke.ECI\Local%20Settings\Temp\~hh8... 3/21/2004 Transact-SQL Page 3 of 53 the table tblFilms. Use of Quotation Marks The ANSI SQL standard allows only double quotation marks to identify database object names, whereas character strings are enclosed in single quotation marks. In Access mdb databases, angle brackets are used to enclose database object names, and double quotation marks are used to identify character strings. If you copy queries from Access mdb databases, you must at least substitute single quotation marks for the double quotation marks that enclose character strings. If the character string already contains single quotation marks, you must replace them with two single quotation marks. For example, “Big Momma’s House” would become ‘Big Momma’’s House.’ In SQL Server/MSDE, you can also use angle brackets for object names. However, if you adhere to the ANSI SQL programming standard, you should use double quotation marks, and make sure that the QUOTED_IDENTIFIER database option is ON. If the QUOTED_IDENTIFIER database option is OFF, you have to use angle brackets to delimit identifiers, and you may use double quotes to delimit character strings. Aliases with AS In the Access results display, the column names are used as the column headings. You can edit the column names with the AS command to specify new names, as shown here: SELECT FilmNr AS Filmnumber, Filmtitle AS 'Film Title' FROM tblFilms If the new column names contain spaces or special characters, be sure to enclose the column names in single quotation marks. For aliases, you can also use the abbreviated version, as displayed here SELECT FilmNr Filmnumber, Filmtitle 'Film Title' FROM tblFilms without the AS command. You can also place an alias in front, like this: SELECT Filmnumber = FilmNr, 'Film Title' = Filmtitle FROM tblFilms You can use aliases for table names as well, such as, for example: SELECT f.FilmNr, f.Filmtitle FROM tblFilms AS f Tables from Other Users, Databases, or Servers SQL Server/MSDE allows a user to create his or her own tables, provided that the user has the required permissions. This makes it possible for multiple users to choose the same table name. In those cases, SQL Server/MSDE differentiates between the tables by adding the user name as a prefix. By default, users can see only their own tables and those tables by the user dbo. With the appropriate permissions, a user can also access others’ tables, even those in other databases located on the same or different servers. The general syntax is as follows: file://C:\Documents%20and%20Settings\richard.welke.ECI\Local%20Settings\Temp\~hh8... 3/21/2004 Transact-SQL Page 4 of 53 SELECT * FROM server.database.user.table Figure 9-1 illustrates the definition of a view that displays the name and dbname fields for the syslogins table from the master database. Figure 9-1 Access to the master database SQL Server/MSDE lets you use tables and views from other servers. These other servers may be SQL Server/MSDE systems or systems that run other database products, such as Oracle or Microsoft Access. The servers must be accessible through the object linking and embedding database (OLE DB) provider or open database connectivity (ODBC) driver. Setting up access to another database server requires definition of a linked server or of linked tables through data sources. See Chapter 24, “External Data Sources,” for more information on setting up linked servers. The view shown in Figure 9-2 accesses the server PN-Notebook, where it reads the tblFilms table in the ContosoSQL database. PN-Notebook was set up as a linked server. file://C:\Documents%20and%20Settings\richard.welke.ECI\Local%20Settings\Temp\~hh8... 3/21/2004 Transact-SQL Page 5 of 53 Figure 9-2 Access to a linked server PN-Notebook Special Cases The programming of stored procedures (see Chapter 8, “Stored Procedures”) often involves special cases of the SELECT command. For example, the commands SELECT 'Text' or SELECT 12+34/3 return a resultset based on a text column or a calculated value. If a query results in exactly one value, you can assign this value to a variable, such as SELECT @Variable = Value or SELECT @Variable = Fieldname FROM Table. SQL Server/MSDE allows the syntax SELECT 'Text' or SELECT NumericalExpression. With Access mdb queries, however, you must always specify a FROM table as well, even if 'Text' or NumericalExpression are not relevant to the table. Calculated Columns Columns based on SELECT commands can also be calculated, which means they can be derived from other columns. The following query, for example, returns every film’s length, plus 45 minutes: SELECT Filmtitle, Length + 45 FROM tblFilms If you do not use AS to specify a column name for a calculated field, SQL Server/MSDE uses the name Expr<n>, with n denoting the column number.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages53 Page
-
File Size-