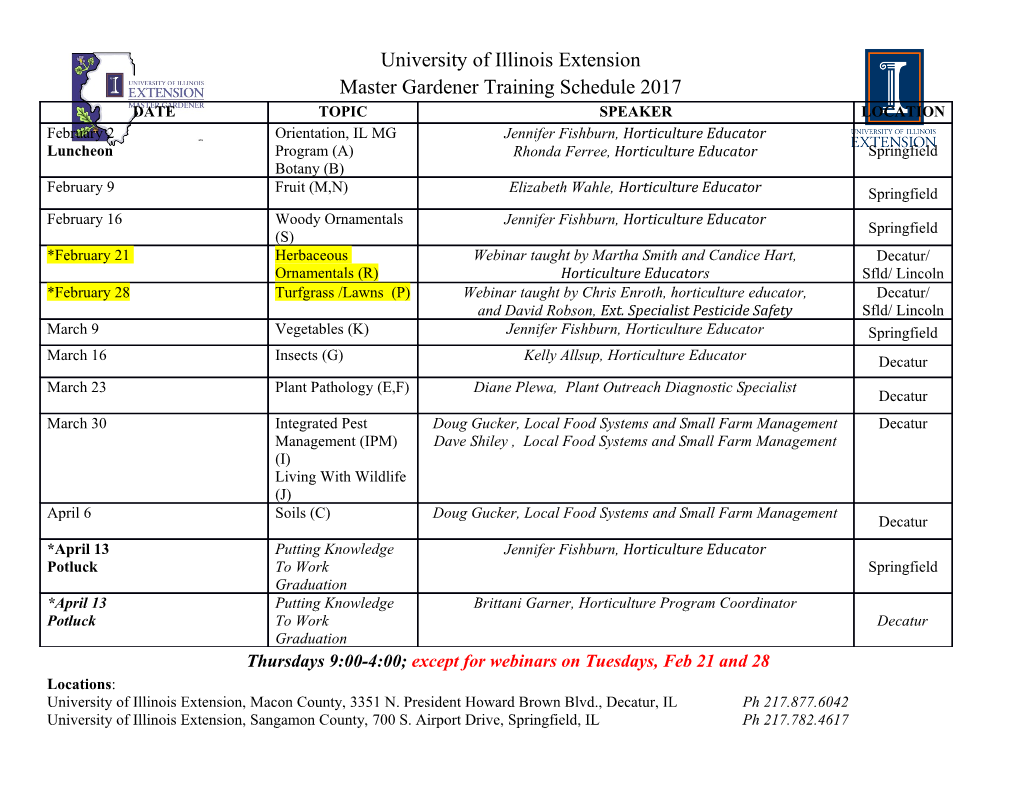
Neuron® C Programmer's Guide Revision 7 C o r p o r a t i o n 078-0002-02G Echelon, LNS, LonBuilder, LonManager, LonMaker, LonTalk, LONWORKS, Neuron, NodeBuilder, 3120, 3150, and the Echelon logo are trademarks of Echelon Corporation registered in the United States and other countries. LONMARK and ShortStack are trademarks of Echelon Corporation. Touch Memory is a trademark of the Dallas Semiconductor Corp. Other brand and product names are trademarks or registered trademarks of their respective holders. Smart Transceivers, Neuron Chips, Serial LonTalk® Adapters, and other OEM Products were not designed for use in equipment or systems which involve danger to human health or safety or a risk of property damage and Echelon assumes no responsibility or liability for use of these products in such applications. Parts manufactured by vendors other than Echelon and referenced in this document have been described for illustrative purposes only, and may not have been tested by Echelon. It is the responsibility of the customer to determine the suitability of these parts for each application. ECHELON MAKES NO REPRESENTATION, WARRANTY, OR CONDITION OF ANY KIND, EXPRESS, IMPLIED, STATUTORY, OR OTHERWISE OR IN ANY COMMUNICATION WITH YOU, INCLUDING, BUT NOT LIMITED TO, ANY IMPLIED WARRANTIES OF MERCHANTABILITY, SATISFACTORY QUALITY, FITNESS FOR ANY PARTICULAR PURPOSE, NONINFRINGEMENT, AND THEIR EQUIVALENTS. No part of this publication may be reproduced, stored in a retrieval system, or transmitted, in any form or by any means, electronic, mechanical, photocopying, recording, or otherwise, without the prior written permission of Echelon Corporation. Document No. 29300 Printed in the United States of America. Copyright ©1990-2003 by Echelon Corporation Echelon Corporation 550 Meridian Avenue San Jose, CA. USA 95126 www.echelon.com Preface This guide describes how to write programs using the Neuron® C Version 2.1 language. Neuron C is a programming language based on ANSI C that is designed for Neuron Chips and Smart Transceivers. It includes network communication, I/O, and event-handling extensions to ANSI C, which make it a powerful tool for the development of LONWORKS® applications. Key concepts in programming with Neuron C are explained through the use of specific code examples and diagrams. A general methodology for designing and implementing a LONWORKS application is also presented. Audience The Neuron C Programmer’s Guide is intended for application programmers who are developing LONWORKS® applications. Readers of this guide are assumed to have some C programming experience. For a complete description of ANSI C consult the following references: • American National Standard X3.159-1989, Programming Language C, D.F. Prosser, American National Standards Institute, 1989. • Standard C: Programmer’s Quick Reference, P. J. Plauger and Jim Brodie, Microsoft Press, 1989. • C: A Reference Manual, Samuel P. Harbison and Guy L. Steele, Jr., 4th edition, Prentice-Hall, Inc., 1994. • The C Programming Language, Brian W. Kernighan and Dennis M. Ritchie, 2nd edition, Prentice-Hall, Inc., 1988. Content The Neuron C Programmer’s Guide • Outlines a recommended general approach to developing a LONWORKS application, and • Explains key concepts of programming in Neuron C through the use of code fragments and examples. Related Manuals The NodeBuilder® User’s Guide lists and describes all tasks related to LONWORKS application development using the NodeBuilder Development Tool. Refer to that guide for detailed information on the user interface and features of the NodeBuilder tool. The LonBuilder® User’s Guide lists and describes all tasks related to LONWORKS application development using the LonBuilder Development Tool. Refer to that guide for detailed information on the user interface to the LonBuilder tool. The Neuron C Reference Guide provides the reference information for writing programs using the Neuron C language. The NodeBuilder Errors Guide lists and describes all warning and error messages related to the NodeBuilder software. The LonMaker® User’s Guide lists and describes all tasks related to LONWORKS network installation, operation, and maintenance using the LonMaker Integration Tool. Refer to that guide for detailed information on the user interface and features of the LonMaker tool. iv Preface The Gizmo 4 User's Guide describes the Gizmo 4 hardware and software. Refer to that guide for detailed information on the hardware and software interface of the Gizmo 4. The FT 3120® and the FT 3150® Smart Transceivers Databook and PL 3120/PL 3150 Power Line Smart Transceiver Databook describe the hardware and architecture for Echelon's Smart Transceivers. These books are also called the Smart Transceiver databooks elsewhere in this manual. Other Neuron Chip information is available from the respective manufacturers of those devices. Typographic Conventions for Syntax Type Used For Example boldface type keywords network literal characters { italic type abstract elements identifier square brackets optional fields [bind-info] vertical bar a choice between input | output two elements For example, the syntax for declaring a network variable is network input | output [netvar modifier] [class] type [bind-info] identifier Punctuation other than square brackets and vertical bars must be used where shown (quotes, parentheses, semicolons, etc.). Code examples appear in the Courier font: #include <mem.h> unsigned array1[40], array2[40]; // See if array1 matches array2 if (memcmp(array1, array2, 40) != 0) { // The contents of the two areas do not match } Neuron C Programmer’s Guide v Contents Preface iii Audience iv Content iv Related Manuals iv Typographic Conventions for Syntax v Contents vi Chapter 1 Overview 1-1 What Is Neuron C? 1-2 Unique Aspects of Neuron C 1-2 Neuron C Integer Constants 1-4 Neuron C Variables 1-5 Network Variables, SNVTs, and UNVTs 1-9 Configuration Properties 1-10 Functional Blocks and Functional Profiles 1-10 Event-Driven vs. Polled Scheduling 1-12 Low-Level Messaging 1-12 I/O Devices 1-12 Differences between Neuron C and ANSI C 1-13 Neuron C Language Implementation Characteristics 1-15 Translation (F.3.2) 1-15 Environment (F.3.2) 1-16 Identifiers (F.3.3) 1-16 Characters (F.3.4) 1-17 Integers (F.3.5) 1-18 Floating Point (F.3.6) 1-19 Arrays and Pointers (F.3.7) 1-20 Registers (F.3.8) 1-20 Structures, Unions, Enumerations, and Bit-Fields (F.3.9) 1-21 Qualifiers (F.3.10) 1-21 Declarators (F.3.11) 1-22 Statements (F.3.12) 1-22 Preprocessing Directives (F.3.13) 1-22 Library Functions (F.3.14) 1-24 Chapter 2 Focusing on a Single Device 2-1 What Happens on a Single Device? 2-2 The Scheduler 2-2 When Clauses 2-2 When Statement 2-4 Types of Events Used in When Clauses 2-4 Predefined Events 2-5 User-defined Events 2-9 Scheduling of When Clauses 2-10 Priority When Clauses 2-11 Function Prototypes 2-12 Timers 2-13 Declaring Timers 2-13 The timer_expires Event 2-14 Input/Output 2-16 I/O Object Types 2-17 vi Preface Declaring I/O Objects 2-21 Overlaying I/O Objects 2-25 Performing I/O: Functions and Events 2-26 Relationship between I/O Measurements, Outputs, and Functions 2-33 I/O Multiplexing 2-34 Device Self-Documentation 2-38 Examples 2-38 Example 1: Thermostat Interface 2-38 Example 2: Simple Light Dimmer Interface 2-42 Example 3: Seven-Segment LED Display Interface 2-44 Input Clock Frequency and Timer Accuracy 2-44 Fixed Timers 2-45 Scaled Timers and I/O Objects 2-45 Calculating Accuracy for Software Timers 2-46 Delay Functions 2-49 EEPROM Write Timer 2-50 Chapter 3 How Devices Communicate Using Network Variables 3-1 Major Topics 3-2 Overview 3-3 Behavior of Writer and Reader Devices 3-4 When Updates Occur 3-5 Declaring Network Variables 3-5 Network Variable Modifiers 3-6 Network Variable Classes 3-7 Network Variable Connection Information 3-9 Network Variable Initializer 3-9 Network Variable Types 3-10 Examples of Network Variable Declarations 3-11 Connecting Network Variables 3-12 Use of the is_bound( ) Function 3-12 Network Variable Events 3-13 The nv_update_occurs Event 3-14 The nv_update_succeeds and nv_update_fails Events 3-14 The nv_update_completes Event 3-15 Sample Program 3-16 Synchronous Network Variables 3-18 Declaring Synchronous Network Variables 3-18 Synchronous vs. Nonsynchronous Network Variables 3-18 Updating Synchronous Network Variables 3-19 Processing Completion Events for Network Variables 3-19 Partial Completion Event Testing 3-20 Comprehensive Completion Event Testing 3-20 Tradeoffs 3-21 Polling Network Variables 3-21 Declaring a Network Variable as Polled 3-24 Explicit Propagation of Network Variables 3-26 Monitoring Network Variables 3-28 Authentication 3-29 Setting Up Devices to Use Authentication 3-30 How Authentication Works 3-31 Changeable-Type Network Variables 3-32 Processing Changes to a SCPTnvType CP 3-35 Changeable-Type Example 3-40 Neuron C Programmer’s Guide vii Chapter 4 Using Configuration Properties to Configure Device Behavior 4-1 Overview 4-2 Declaring Configuration Properties 4-2 Declaring Configuration Properties Within Files 4-3 Declaration of Configuration Network Variables 4-5 Instantiation of Configuration Properties 4-7 Device Property Lists 4-8 Network Variable Property Lists 4-10 Accessing Property Values from a Program 4-11 Advanced Configuration Property Features 4-13 Configuration Properties Applying to Arrays 4-14 Initialization of Configuration Properties at Instantiation 4-16 Sharing of Configuration Properties 4-18 Configuration Property Sharing Rules 4-19 Type-Inheriting Configuration Properties
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages328 Page
-
File Size-