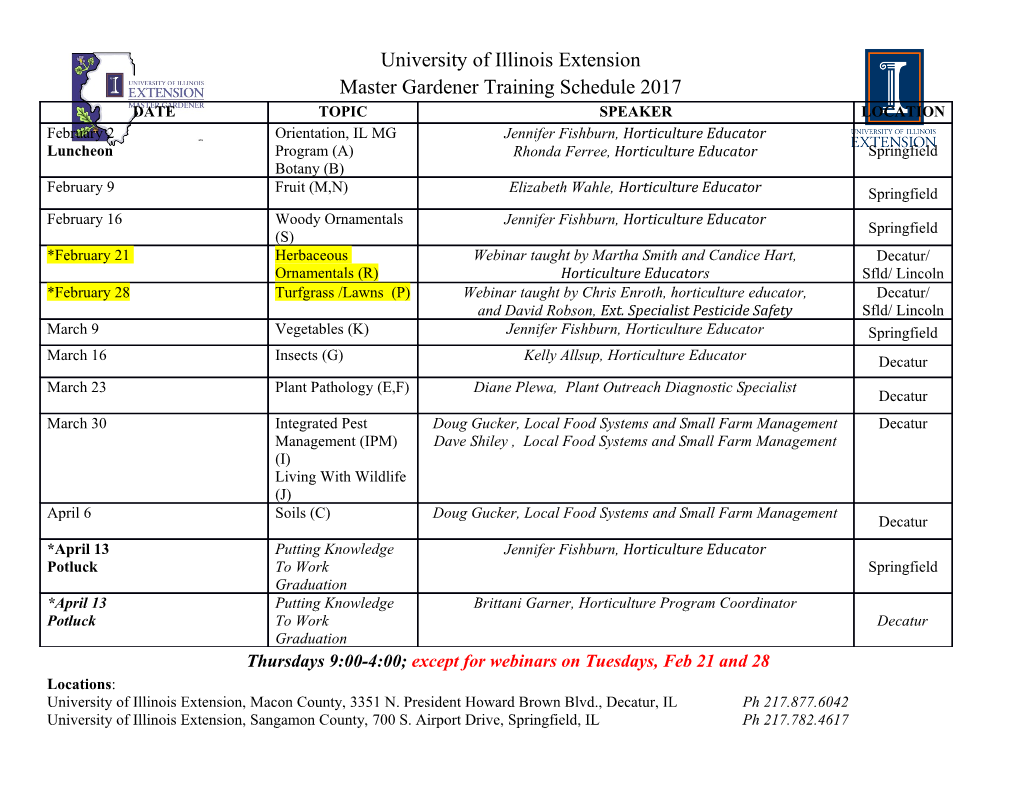
Unix-Linux 6 Files System Calls #include <sys/types.h> #include <sys/stat.h> #include <unistd.h> #include <fcntl.h> int open(const char *pathname, int flags, [mode_t mode]); flags O_RDONLY (read only) O_WRONLY (write only) O_RDWR (read-write) O_CREAT (create) O_EXCL (exclusive) O_TRUNC (truncate) return values -1 open( ) failure n >= 0 file descriptor o 0 stdin o 1 stdout o 2 stderr o n > 2 smallest non-negative file descriptor not already being used by the process making the call mode only used with the O_CREAT flag filedes = open(“/tmp/newfile”, O_WRONLY | O_CREAT, 0644); 1. example – open( ) char *workfile = “junk”; if (( filedes = open(workfile, O_RDWR )) == -1) { printf(“%s failed to open”, workfile); exit(1); } // remainder of program close(filedes); POSIX Specification : Number of Open Files == 20 per process 2. example – creat( ) #include <sys/types.h> #include <sys/stat.h> #include <fcntl.h> int creat(const char *pathname, mode_t mode); always truncates an existing file before returning the file descriptor always opens a file for writing only 3. example – close ( ) #include <unistd.h> int close( int filedes); returns -1 error returns 0 successful 4. example – read ( ) #include <unistd.h> ssize_t read(int filedes, void *buffer, size_t n); int fd; const int SOMEVALUE = 256; ssize_t nread; char buffer[SOMEVALUE]; nread = read(fd, buffer, SOMEVALUE); maximum number of characters to be read nread >= 0 number of characters ACTUALLY read nread == -1 read failure number of characters requested > number of characters remaining in file system transfers the characters remaining in the file & sets nread to the number of characters read testing for end of file within a program testing nread == zero For best performance make sure that BUFSIZE #define BUFSIZE 512 is a multiple of the disk blocking factor long total = 0; If (( filedes = open(“anotherfile”, O_RDONLY)) == -1) {printf(“ … “); exit(1);} while (( nread = read(filesdes, buffer, BUFSIZE)) > 0) total += nread; Efficiency printf(“Total Read: %ld\n”, total); Define the BUFSIZE to be a multiple exit(0); of the disk block size of the system; see /usr/include/stdio.h File Pointer for each file descriptor, there exists an unique read-write file pointer records the next byte to be read/written via that file descriptor sequential read-write operations file pointer position maintained by the operating system random access read-write operations file pointer position is explicitly changed by the lseek( ) system call off_t lseek (int filedes, off_t offset, int start_flag); file descriptor new position of the specifies the starting point from which to read-write, i.e., offset, measure the offset file pointer; it is the number of bytes to be values added to the starting SEEK_SET : beginning of the file position of the file SEEK_CUR : current position of the pointer SEEK_END : end of the file off_t newpos; casting … new position is 16 bytes before the end of the file … newpos = lseek(fd, (off_t)-16, SEEK_END); new position is 20 bytes after newpos = lseek(fd, (off_t)20, SEEK_BEG); the beginning of the file newpos = lseek(fd, (off_t)+5, SEEK_CUR); new position is 5 bytes after the last position new position of the read-write offset pointer attempting to move the offset pointer before the start of the file, i.e., newpos = lseek(fd, (off_t)-1, SEEK_BEG); results in an error it is possible to move to a position beyong the current end of the file, i.e., newpos = lseek(fd, (off_t)100, SEEK_END); the file may have no data in the gap, it will appear to be filled with the ASCII null character filedes = open(filename, O_RDWR); open file for read-write; lseek(filedes, (off_t)0, SEEK_END); set offset pointer to end of the file; write(filedes, outbuf, OBSIZE); write a block of data to the end of the file filedes = open(filename, O_WRONLY | O_APPEND); (write only, append to end of file) write(filedes, appbuf, BUFFSIZE); subsequent writes will continue to write to the current end of the file determine the file size filesize = lseek(filedes, (off_t)0, SEEK_END); removing regular files int unlink(const char *pathname); int remove(const char *pathname); returns 0 success removing directories -1 failure int rmdir(const char *pathname); int remove(const char *pathname); control of open files very irregular function, i.e., a “strange beast”, that performs #include <sys/types.h> a mixed bag of diverse tasks in Unix, thus the ellipsis “ … “ #include <unistd.h> specifying the third parameter in the definition; the third #include <fcntl.h> parameter depends on the value of the second parameter Int fcntl(int filedes, int cmd, … ); cmd values F_GETFL : return the current file status flags F_SETFL : reset the file status flags only certain flags can be reset by this command int filestatus(int filedes) { int arg1; if(( arg1 = fcntl(filedes, F_GETFL)) == -1) { printf (“filestatus failed\n”); return (-1); } printf (“file descriptor %d: “, filedes); switch ( arg1 & O_ACCMODE) O_ACCMODE is a mask defined in <fcntl.h> { the file status bits in arg1 are compared to case O_WRONLY: those in O_ACCMODE by the bit-wise operator printf(“write-only”); AND, i.e., “&” break: case O_RDWR: : return the current file status flags printf(“read-write”); F_SETFL : reset the file status flags break: case O_RDONLY: printf(“read-only”); break: default: printf(“mode does not exist”); } if (arg1 & O_APPEND) printf(“ – append flag set”); printf (“\n”); return (0); } If ( fcntl( filedes, F_SETFL, O_APPEND) == -1) printf(“fcntl edrror \n”); // all future writes will append to the end of the file Standard I/O Library stdin 0 stdout 1 stderr 2 #include <stdio.h> #include <stdlib.h> main( ) { FILE *stream; if ( stream = fopen(“junk”, “r”)) == NULL) { printf(“file junk could not be opened\n”); exit(1); } } FILE Structure Open File Table Buffer stream fd int getc(FILE *istream); // read a character from istream int putc(int c, FILE *ostream); // write a character to ostream while( ( c = getc(istream)) != EOF) putc(c, ostream); EOF == -1 The first call to getc( ) or to putc( ) results in the construction of a buffer of BUFSIZE which is processes by either a read( ) or a write( ) system call; on input, the buffer is filled by the first call to getc( ) and the following BUFSIZE -1 calls obtains data from the buffer; the next call to getc( ) refills the buffer; output works in reverse. Error Numbers errno : error variable #include < stdio.h> #include < fcntl.h> #include < errno.h> main ( ) errno is not reset – only use errno immediately after { a system call has failed int fd; if ( ( fd = open(“acct_rec”, O_RDONLY) ) == -1) fprintf(sterr, “error, acct_rec not opened %d\n”, errno); perror(“error, acct_rec not opened”); } include only one of these statements Executable File Permissions set user-id on execution 000 100 000 000 000 o let a program be created and stored in a file with this bit set o the process which executes the program assumes the effective user-id of the files’ owner (rather than that of the process which is executing the program) set group-id on execution 000 010 000 000 000 o let a program be created and stored in a file with this bit set o the process which executes the program assumes the effective group-id of the files’ owner save-text-image (sticky bit) 000 001 000 000 000 o only defined for directories – discussed later Change Ownership int chown( const char *pathname, uid_t owner_id, gid_t group_id); new owner new group Hard Links ( hard links are only used within a particular filesystem) link count : number of links associated with a particular file int link( const char *original_path, const char *new_path); original file name new path & file name not possible to create a link to a directory cannot be used to link across filesystems o each filesystem has a unique set of inodes o hardlinks use the inode numbers Rename int rename( const char *oldname, const char *newpathname); // rename files and directories Symbolic Links symbolic link is a file that contains the pathname to the linked file symbolic link can be thought of as a pointer to the linked file int symlink(const char *realname, const char *symname); file symname is created and points to the file realname int readlink( const char *sympath, char *buffer, size_t bufsize); opens file sympath, reads bufsize characters into buffer and finally closes the sympath file File Status #include <sys/types.h> #include <sys/stat.h> int stat( const char *pathname, struct stat buf); int fstat( int filedes, struct stat buf); // used with open files only ● ● ● struct stat s; int filedes, retval; filedes = open(“/tmp/junk”, O_RDWR); retval = stat(“/tmp/junk”, &s); // OR retval = fstat(filedes, &s); <sys/stat.h> includes the definition of struct stat the types used in struct stat are defined in <sys/types.h> struct stat includes dev_t st_dev; // logical device ino_t st_ino; // inode number of the file mode_t st_mode; // lower 12 bits records the file permissions nlink_t st_nlink; // number of non-symbolic links uid_t st_uid; gid_t st_gid; dev_t st_rdev; // used if the file entry is used to describe a device off_t st_size; // logical size of the file in bytes time_t st_atime; // time the file was last read time_t st_mtime; // time the file was last modified time_t st_ctime; // last time any data item in struct stat was altered long st_blksize; // specific block size for this particular file long st_blocks; // number of physical file system blocks allocated to this particular file ● ● ● Unix File Structure directories regular files o text files o binary files o script files
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages16 Page
-
File Size-