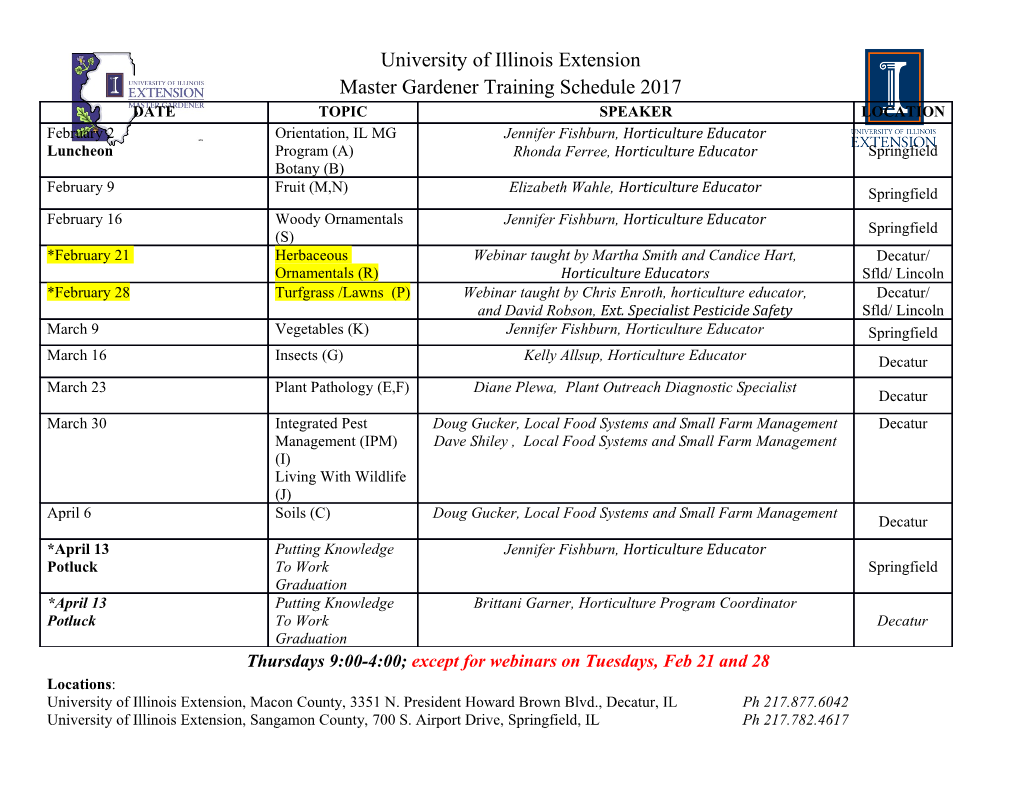
The Visual Development of GCC Plug-ins with GDE A Thesis Presented by Daniel Joseph Dean to The Graduate School in Partial Fulfillment of the Requirements for the Degree of Master of Science in Computer Science Stony Brook University May 2009 Technical Report FSL-09-04 Stony Brook University The Graduate School Daniel Joseph Dean We, the thesis committee for the above candidate for the Master of Science degree, hereby recommend acceptance of this thesis. ————————————————————————- Prof. Erez Zadok, Thesis Advisor Associate Professor, Computer Science ————————————————————————- Prof. Annie Liu, Thesis Committee Chair Professor, Computer Science ————————————————————————- Prof. Robert Kelly Associate Chair, Computer Science This thesis is accepted by the Graduate School Lawrence Martin Dean of the Graduate School ii Abstract of the Thesis The Visual Development of GCC Plug-ins with GDE by Daniel Joseph Dean Master of Science in Computer Science Stony Brook University 2009 Being able to directly affect code compilation with code transformations allows the seamless addition of custom optimizations and specialized functionality to code at compile time. Tradi- tionally, this has only been possible by directly modifying compiler source code: a very difficult task. Using GCC plug-ins, developers can directly affect code compilation, without actually mod- ifying the source code of GCC. Although this makes applying a completed plug-in easy, plug-in development is transformation development nonetheless: an arduous task. The plug-in developer is required to have the same thorough understanding of compiler internals, complex compiler internal representations, and non-trivial source to internal representation mappings as any other transformation developer. Recently, simplified representations, such as CIL, have been developed to help developers overcome some transformation design challenges. Although useful in their own respect, repre- sentations like CIL are often language-specific by design. This requires the developer to make the unfortunate choice between the relative ease of development on a simplified representation or language generality on a more complex representation. We have developed a visual approach to transformation development consisting of a two com- ponents: a plug-in to extract GCC’s intermediate representation and a Java-based tool to visualize it. This thesis will clearly demonstrate how our visual technique significantly reduces many of the problems facing transformation development without sacrificing the inherent benefits of a more generalized intermediate representation. iii To Roxana. You believe in me and make me want to be a better person. Contents 1 Introduction 1 2 Background 4 2.1 Front-End...................................... 4 2.2 Middle-End ..................................... 5 2.3 Back-End ...................................... 6 3 Development Methodology 10 3.1 GCCPlug-ins.................................... 10 3.2 Verbose-DumpPlug-in . 11 3.3 DB-DumpPlug-in.................................. 12 4 Design 14 4.1 OverviewWindow ................................. 15 4.1.1 CFG: .................................... 15 4.1.2 CallGraph ................................. 16 4.2 GIMPLETreeView................................. 17 4.3 SourceWindow................................... 19 4.4 GDBConsole.................................... 20 4.5 Extensible...................................... 20 5 Intermediate Dump Analysis 23 5.1 FilesExamined ................................... 23 5.2 DumpSizes..................................... 23 5.3 PotentialUses................................... 24 6 Use Cases 27 6.1 DissectingGIMPLETrees . 27 6.2 Dissecting Complex Expressions . ...... 28 6.3 APIUsage...................................... 30 6.4 DebuggingBadCode ................................ 30 6.5 CFGInspection ................................... 31 6.6 GDBConsole .................................... 32 v 7 Related Work 34 7.1 GraphicalDevelopment. 34 7.2 Compiler Visualization . 35 7.3 CIntermediateLanguage . 36 8 Conclusions 37 9 Future Work 38 9.1 Zooming....................................... 38 9.2 OnlineFunctionality .... ...... ..... ...... ...... .. 38 9.3 RTL......................................... 38 vi List of Figures 1.1 C to intermediate representation . ....... 2 2.1 The GCC compilation process . ... 7 2.2 AnexampleCFGrenderedbyGDE. 8 2.3 Anexamplecallgraph .............................. 9 3.1 A figure showing the plug-in loading process. ......... 11 3.2 Sample out from the verbose-dump GCC plug-in. ........ 12 4.1 TheGDEuserinterface... ...... ..... ...... ...... ... 14 4.2 TheCFGrenderedbyGDE. ...... ..... ...... ...... ..... 16 4.3 The call graph rendered by GDE . 17 4.4 Alargebasicblock ................................ 18 4.5 AnexamplecyclicGIMPLEaccess . 19 4.6 GCCcallingprocess ............................... 20 4.7 TheGDBConsoleofGDE.. ...... ..... ...... ...... ..... 21 4.8 GDEclassstructure............................... 22 5.1 Database size vs.number of statements . ........ 24 5.2 Database size vs.number of statements without test.c.reference . 25 6.1 Using GDE to get information about a COND EXPR................ 29 6.2 Using GDE to see how a particular statement is gimplified. ............ 29 6.3 Using GDE to help determine which macro to use. ....... 30 6.4 Invalid and valid versions of a duplicated control-flow graph. 32 vii List of Tables 5.1 Showing DB-dump key statistics . ..... 24 viii Acknowledgments Justin Seyster for his comments on an early draft of the thesis. Sean Callanan designed and implemented the GCC plug-in system. This work was partially made possible thanks to a Computer Systems Research NSF award (CNS-0509230) and an NSF CAREER award in the Next Generation Software program (CNS- 0133589). Chapter 1 Introduction Developers have long wanted greater control over compilation in order to automatically add fea- tures like application-specific custom optimizations, integrated type checking, function call log- ging, or parallism to code at compile time [2] [32] [26] [23]. Code transformations give developers this ability by modifying the compiler’s internal representation of compiling code. The traditional development of code transformations,however, requires the direct modification of compiler source files, a difficult and error prone task. As Chapter 3 explains, GNU Compiler Collection (GCC) plug-ins are code transformations which do not require the developer modify the compiler source itself [6]. Although this makes the application and deployment of completed transformations a relatively simple process, plug-in development is an arduous task. The GCC developer community has a great deal of expertise in developing code transforma- tions due to their intimate knowledge of the compiler. Non-GCC developers, however, must first learn the inner workings of GCC before developing a transformation. One of the most daunting tasks in understanding the inner workings of GCC is understanding the various intermediate rep- resentations that GCC creates. As shown in Figure 1.1, a single line of C code produces many GIMPLE trees, with each GIMPLE tree containing internal information. Although each GIMPLE tree node is used by the compiler in one way or another, a typical transformation is only interested in a subset of nodes. Unfortunately, for the developer this often leads to hours of sorting through low-level intermediate code to find a needle in the vast intermediate-representation haystack. This thesis presents a visualization technique for the development of GCC plug-ins. Our tech- nique involves the design and implementation of a visualization tool, the GIMPLE Development Environment (GDE), along with a GCC plug-in to extract and format GCC internal informations. GDE provides developers with four types of visualizations: (1) the control flow graph, (2) the call graph, (3) the GIMPLE trees, and (4) the mapping from source to internal representation. We demonstrate with a series of use cases, how these visual representations significantly reduce the difficulty of interpreting and understanding the intermediate representation that GCC generates while compiling a program. The remainder of this thesis is organized as follows. Chapter 2 gives an overview of GCC as a whole by presenting the fundamentals of GCC. It is here we introduce the various phases of compilation, explain why each phase exists, and finally describe the intermediate representation at each phase. Although each phase is useful in its own right, this thesis focuses primarily on the 1 #include <sys/types.h> uint64_t facts[ 21 ]; uint64_t fact( unsigned char x) { if (!facts[x]) { if (x == 0) facts[x] = 1; else facts[x] = x * fact(x- 1); } return facts[x]; } D.3155 = ( int ) x; D.3156 = (uint64_t) x; D.3157 = x + 255 ; D.3158 = ( int ) D.3157; D.3159 = fact (D.3158); D.3160 = D.3156 * D.3159; facts[D.3155] = D.3160; GIMPLE_MODIFY_STMT 0,2 TYPE_NAME: FUNCTION_DECL 0,0 TYPE_MODE=13 INTEGER_TYPE 0,0 VAR_DECL 12,0 TYPE_DECL 7,0 DECL_ABSTRACT_ORIGIN=NULL_TREE TYPE_POINTER_TO: TREE_INT_CST_LOW=64 TREE_TYPE: TREE_TYPE: DECL_ALIGN=8 POINTER_TYPE 0,0 TREE_INT_CST_HIGH=0 INTEGER_TYPE 12,0 INTEGER_TYPE 0,0 DECL_SIZE: TYPE_NEXT_VARIANT: DECL_MODE=13 TYPE_SIZE: DECL_NAME: INTEGER_CST 0,0 INTEGER_TYPE 0,0 DECL_INITIAL=NULL_TREE INTEGER_CST 3,0 IDENTIFIER_NODE 0,0 DECL_MODE=10 TYPE_MAIN_VARIANT: DECL_SOURCE_FILE=test.c TREE_TYPE: DECL_CONTEXT=NULL_TREE DECL_ARG_TYPE: INTEGER_TYPE 0,0 DECL_SOURCE_LINE=15 INTEGER_TYPE 0,0 DECL_ABSTRACT_ORIGIN=NULL_TREE INTEGER_TYPE
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages50 Page
-
File Size-