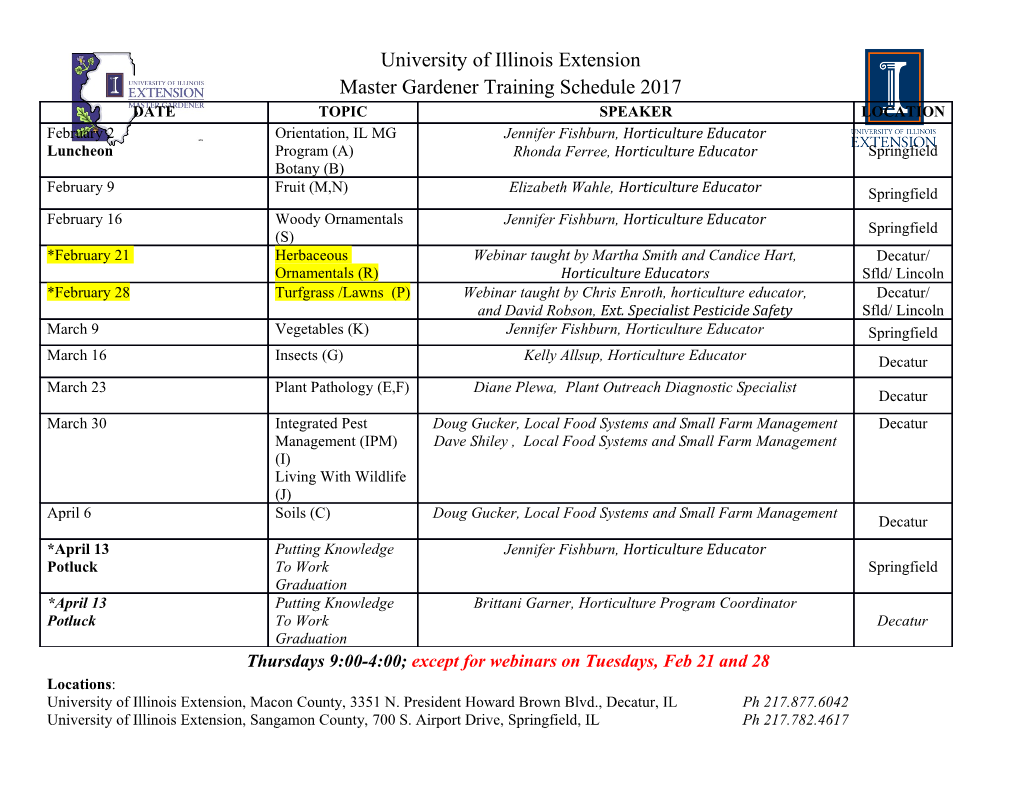
Elements of Functional Computing Dan R. Ghica 2015 Contents 1 Introduction 4 1.1 Basic concepts . .4 1.2 Programming the machine, a historical outlook . .4 1.3 Abstraction . .5 1.4 Functional programming: a historical outlook . .6 2 \Formal" programming: rewrite systems 8 2.0.1 \Theorems" . .9 2.1 Further reading . 10 3 Starting with OCaml/F# 11 3.1 Predefined types . 11 3.2 Toplevel vs. local definition . 13 3.3 Type errors . 13 3.4 Defined types: variants . 14 4 Functions 15 4.1 Pattern-matching and if . 16 5 Multiple arguments. Polymorphism. Tuples. 20 5.1 Multiple arguments . 20 5.2 Polymorphism . 20 5.3 Notation . 21 5.4 Tuples . 22 5.5 More on pattern-matching . 23 5.6 Some comments on type . 24 6 Isomorphism of types 25 6.1 Quick recap of OCaml syntax . 26 6.2 Further reading . 26 7 Lists 27 7.1 Arrays versus lists . 27 7.2 Getting data from a list. 28 8 Recursion. 30 8.0.1 Sums . 30 8.0.2 Count . 31 8.1 Creating lists . 32 8.2 Further reading . 34 8.3 More on patterns . 34 1 9 Case study: graph search 37 9.1 Graph search . 37 9.2 Running and debugging . 41 10 Structural induction 47 10.1 Examples for structural induction . 49 10.1.1 Example . 49 10.1.2 Example . 49 10.1.3 Example . 50 11 Search and sort 51 11.1 Searching and filtering . 51 11.2 Quick-sort . 52 11.3 Selection sort . 53 11.4 Performance of quick-sort . 55 11.5 Mergesort . 56 11.6 Questions . 60 12 Correctness of sorting 61 13 Number representations 65 13.1 Natural numbers . 65 13.2 Arbitrary numeral systems . 68 13.3 Canonical representation . 69 13.4 Questions . 70 14 Data types 72 14.1 Syntax of Ocaml types . 72 14.2 Handling errors through data types . 73 14.3 Integers . 74 14.4 Rationals . 75 14.5 Real numbers . 75 14.6 Floating point . 76 14.7 An application: logistic map . 78 15 Introduction to complexity 81 15.1 Profiling . 84 15.2 Tail recursion . 85 16 Arrays and imperative programming 87 16.1 Searching and sorting . 90 17 Binary trees as a data types 94 17.1 Tree traversals . 96 17.1.1 Complexity of traversal . 98 17.2 Dictionaries as binary search trees . 100 17.2.1 Search . 101 17.3 Search tree manipulation . 101 17.3.1 Insertion . 101 17.3.2 Deletion . 103 17.3.3 Balancing . 105 17.4 Expression evaluation . 108 17.4.1 Logic . 108 17.4.2 Arithmetic . 109 17.5 Arithmetic-logic expressions . 109 2 18 Queues 111 18.1 Naive implementation . 112 18.2 Banker's queues . 113 18.2.1 Amortised complexity . 115 18.3 Interaction nets . 115 18.4 Imperative queues . 117 3 1 Introduction 1.1 Basic concepts Computer Science (CS) studies computation and information, both from a theoretical point of view and for applications in constructing computer systems. It is perhaps not very helpful to try and define these two basic, and deeply connected, notions of \computation" and \information", but it is perhaps helpful to talk about some properties they enjoy. Information is what is said to be exchanged in the course of communication. Information is said to be used to represent data, knowledge or ideas. A wonderful example of information is the DNA: it is a pattern of molecules that defines the formation and development of an organism without any need for a conscious or insightful mind. This lack of insight is essential for the understanding of computation, which is the deliberate, automated, mechanical processing or transformation of information. So the questions that CS answers are commonly about what can be computed and how it can be computed. The answers to these questions can be both qualitative, indicating that something can/cannot be computed, and quantitative, indicating how much of a certain resource (time, space, energy) must be consumed in order to perform a computation. The engineering and design side of CS is concerned with how to improve quantitative measures of computation. Computation is carried out by a mindless, uninsightful program executed by a machine. The machines can be either physical (case in which they are called computers) or virtual (a machine which is in some logical sense running on top of another machine) or abstract (a theoretical con- traption which embodies certain ideals of what can be computed). Programs are always written in a language which needs to be well-defined in at least two ways: Syntax. Just like English, or any other natural language, it is important to distinguish between well-formed phrases and gibberish. Note that to make this distinction it is not important to understand the meaning, just the rules of what a well-formed phrase looks like. Consider the opening stanza of the poem Jabberwocky by Lewis Caroll: 'Twas brillig, and the slithy toves Did gyre and gimble in the wabe; All mimsy were the borogoves, And the mome raths outgrabe. It has the syntactic form of English despite using nonsensical made-up words. Programming languages, unlike natural languages, have much stricter and clearly defined syntax. A good programming language has no ambiguity as to whether a phrase is legal or not, and if it is legal as to what its syntactic structure is. In a program a single-character typo can usually render a program illegal. Semantics. If syntax is concerned with what a meaningful program should look like, in semantics the concern is what the program actually means. Just like the syntax of a programming language must have a complete, unambiguous specification so its semantics must define com- pletely and unambiguously what each program means. Defining, or even just understanding, the syntax and semantics of languages is an advanced topic with which we will not deal in this module. Instead we will focus on the activity of writing programs, i.e. programming. 1.2 Programming the machine, a historical outlook Programming is actually a very old activity. The oldest recognisable device used to perform calculations, the abacus, is known since 2400 BCE, although all its calculations are manual. The first mechanical calculators were used to perform astrological calculations, for example the astrolabe, which was invented around 150 BCE. More modern efforts are Pascal's 1642 calculating machine and Leibniz's Stepped Reckoner from 1672. The latter was made into a commercial product, the Arithmometer by C.X. Thomas in 1820. Perhaps the most famous was Babbage's analytical engine, a design continuously improved from 4 1833 until 1871, the year of Babbage's death. Notably, its programs were stored on punchcards and it had control features similar to modern computers. Ada Lovelace is officially the world's first computer programmer, having written a program for the analytical engine to compute a sequence of numbers known as Bernoulli numbers. The first electronic computers appeared before and during World War II. Replacing mechanical components with electronic circuits allowed higher speeds and smaller sizes. Variously claiming to be the first computer are Zuse's Z3 (Germany, 1941), the Atanasoff-Berry computer (USA, 1937) or the Colossus (UK, 1943). ENIAC (US, 1946) was the first computer to resemble in internal structure today's computers. It executed a few thousands instructions per second, it weighed 27 tons and it consumed 150 kW of power. For comparison, a smartphone contains a computer which.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages119 Page
-
File Size-