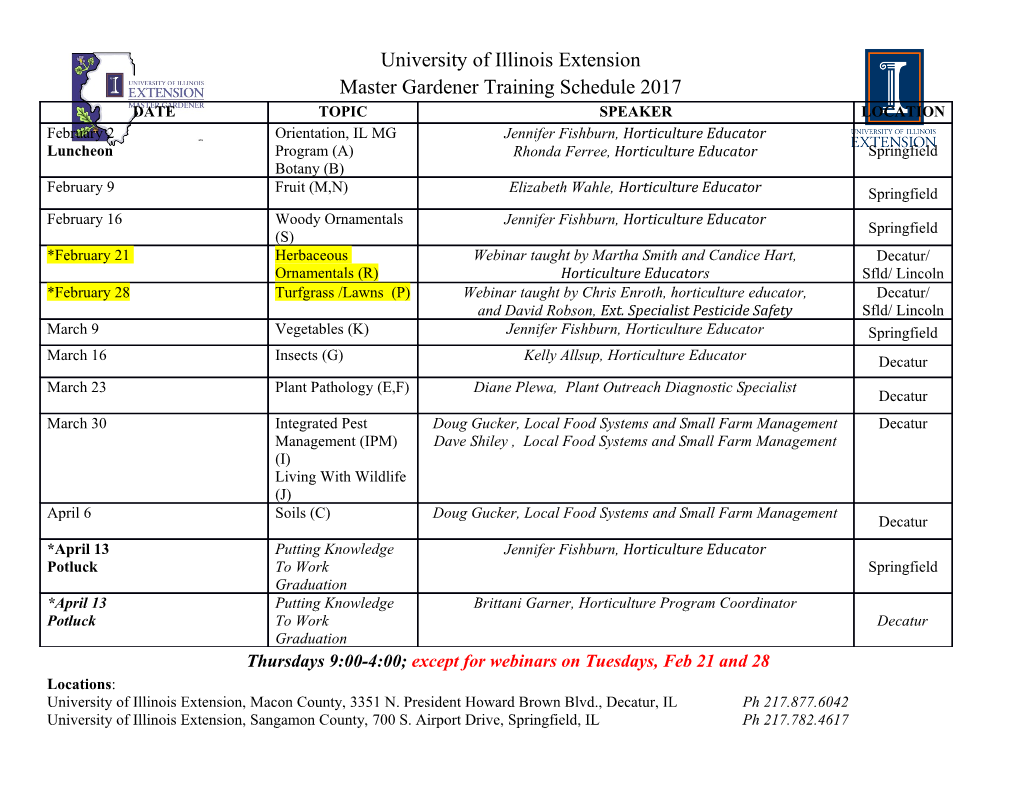
Overview of JavaScript Application Development Juho Vepsäläinen Mathematical Information Technology University of Jyväskylä Tietotekniikan teemaseminaari (TIEA255) April 5, 2011 1 Abstract This article will provide an overview of JavaScript application de- velopment. CanvasDraw, an actual web based drawing application, will be used to highlight various technologies available. These tech- nologies include HTML5 Canvas and two JavaScript libraries: RightJS and RequireJS. In addition the feasibility of JavaScript based applica- tion development is discussed in some detail. 1 Introduction Software development has gone through radical changes during the last few decades. As minituarization and competition has driven the progress of hard- ware it has become commoditized, particularly in the Western world. Before computing resources were scarce. Now they have become abun- dant. These days there is often more computing power available than we are able to properly utilize. This development has made the role of a software developer crucial, even more so than before. These days it is possible even for small, agile teams to succeed. They just need to work the right way on the right market. Web development provides one feasible target for these kind of teams. Thanks to the prevalence of web it's now possible to reach millions of po- tential users with a relative ease. You could say web browser has subsumed the role of operating system and blurred the distinction between various platforms. Almost ubiquitously supported JavaScript programming language and still developing HTML5 standard combined together form the dynamic duo of web development. Throw in CSS3 and you have got a winner. In some cases amalgamation of this technology provides a signicant alternative to established Flash and Java based solutions. JavaScript a Joke Language? Before, in the 90s, developers may have sneered at JavaScript and treated it as a language for script kiddies. Perhaps the language deserved some of that. Surprisingly, the core of the language is quite powerful. Perceived prob- lems often stem from various cross-browser incompabilities and poor browser APIs. Particularly DOM is a good example of this. JavaScript Libraries Various JavaScript libraries have arisen to work around these problems. jQuery in particular has become a shining example 2 of one. It manages to hide nasty cross-browser issues and make it possible for a casual coder to focus on getting things done. There is a huge collection of libraries like this out there just waiting for you. There is rarely any good reason to stick with vanilla JavaScript. Goals The main purpose of this article is to provide some insight to JavaScript based app development. I will cover very basics of JavaScript, the program- ming language. After that I will have a look at it as compared to a full web development stack ranging from client to server. Once I'm done with JavaScript, I will move onto HTML5 and discuss the concept a bit further. It is way too broad to cover in full detail in this context. I do aim to provide some starting points for a reader interested in the subject. Finally I will discuss a JavaScript and HTML5 Canvas based application, CanvasDraw. I will revisit the concepts discussed in a brief conclusion. 2 JavaScript Programming Language Java is to JavaScript as ham is to hamster. Often people not familiar with JavaScript think it's the same thing as Java. The truth could not be further apart. The name JavaScript has been derived through a poorly thought out decision by marketers at Netscape [6]. It was probably thought to give the language some kind of leverage since Java was the hip thing in the golden 90s. Oh well, even despite this poor decision the language has managed to thrive, or at least become popular. It is easily one of the most deployed languages out there. Almost every web browser provides some kind of support for JavaScript. This makes it a truly ubiquitous platform to develop for. 2.1 History Brendan Eich started developing JavaScript in 1995 for Netscape's then pop- ular Navigator web browser. The idea was to provide some kind of scripting interface to make it easier for programmers to develop various functionality to otherwise static webpages. [6] 3 Microsoft responded by implementing a similar language, JScript. Even though the languages nowadays look pretty much the same, there are some minor dierences between them. They are not big enough for me to worry about, though, so I won't bug you about them. Since then the specication of the language has been formalized. This specication is also known as ECMAScript [1]. Various implementations are based on it. Perhaps the most known ones besides JavaScript is Adobe's ActionScript [2] and its versions. 2.2 Outlook At the time Eich was particularly impressed by Self, Scheme and similar languages. From Java 1.0 he took Math and Time libraries. As a result he integrated various features from these languages to his mighty little hack of a week [6]. Overall the language is quite simple. It looks a bit like C and Java. Par- ticularly bracketed syntax is probably familiar to friends of these languages. Similarity-wise that's about it, though. 2.3 Typing JavaScript uses weak, dynamic typing [1]. Since the language accepts almost anything except for the most blatant errors, this makes it quite exible. It also means that it's quite easy to make inadvertent errors. These will get caught later on as some poor user does some nasty thing the developer didn't anticipate properly. Especially comparison operator (==) may be tricky for a beginning pro- grammer. It coerces the comparable items to the same type by default and executes the comparison based on that. In some cases this may yield unex- pected result. This is the reason why some favor using non-coercing operator (===) instead. 2.4 Basic Types The language provides basic types including Object, Array, String, Number, null and undened [1]. Object is the core type of the language. It is simply a hash (key-value pairs). In most, if not all JavaScript implementations, it is also ordered. This means the keys of an Object appear in given order when iterated. Note that 4 this is not guaranteed by the ECMAScript specication [1] so it may not be quite safe to rely on this behavior. Here's a small example of what Object looks like: var duck = { name: 'donald', age : 42 }; // let's print out some values console.log(duck.name, duck[ 'age']); // set some property duck.height = 123; Note that duck.name and duck['age'] achieve pretty much the same thing. The latter syntax is used particularly when key happens to clash with some JavaScript keyword. It is also handy while iterating values of an Object. Array in JavaScript is indexed from zero just like in most popular lan- guages perhaps with Lua as an exception. They may also be treated as queues or stacks using the API. An Array can look like this: var lotteryNums = [12, 25, 5, 2, 6, 3, 21]; // extra number lotteryNums.push(22); // not gonna need it. got good numbers already lotteryNums.pop(); String simply signies an array of characters. It is possible to iterate it just like a regular Array. A very simple String: var name = 'Joe'; Number type is a bit special. Even though the language contains functions such as parseInt, it stores all numbers using some kind of oating point presentation. This is a good enough solution as long as you are not doing 5 any high precision mathematics. There are libraries such as BigNumber1 that work around this issue, though. A couple of Numbers: var a = 2 ; var b = 5 . 2 ; null and undened null and undened are more or less equivalent se- mantically. Of these particularly undened is used. It comes around in many places. JavaScript Garden [8] lists its usages as follows: • Accessing the (unmodied) global variable undened. • Implicit returns of functions due to missing return statements. • return statements which do not explicitly return anything. • Lookups of non-existent properties. • Function parameters which do not had any explicit value passed. • Anything that has been set to the value of undened. Often null may be replaced simply by using undened. Some parts of JavaScript's internals rely on the usage of null, though [8]. The following example shows null and undened in action: var c ; var d = undefined; var e = n u l l ; console.log(c); // undefined console.log(d); // undefined console.log(e); // n u l l 2.5 Basic Structures JavaScript includes a variety of handy language structures. I will cover basic conditionals, exceptions, loops and functions next. 1http://jsfromhell.com/classes/bignumber 6 Conditionals form the core of most modern languages. JavaScript pro- vides very standard conditionals. The basic one looks like this: var a = true ; var b = 21; var r e s u l t ; i f ( a ) { result = 'got a'; } else i f (b == 13) { result = 'no a, b matched'; } else { result = 'no match; } console.log(result); In addition it is possible to use Cish ternary operator like this: var a = ' 13 ' ; var b = a == 14? 'got a': 'noo'; There are also the usual and and or: var a, b=1, 0; // evaluates as false, selects b var c = a && b ; // evaluates as true, selects a var d = a | | b ; The language contains also a switch statement: var favoriteMovie = 'Rambo'; var r e s u l t ; switch (favoriteMovie) { case 'Rambo ' : result = 'Awesome! '; break ; case 'Water World ' : 7 result = 'That sucks. '; break ; default : result = 'Ok... '; } console.log(result); Exceptions work as well: var triggerError = function() { throw new Error( 'Uh oh, did something nasty! '); }; try { // do something nasty to trigger exception triggerError (); } catch ( e ) { // catch i t console.log(e); } Looping Arrays is quite simple in JavaScript.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages35 Page
-
File Size-