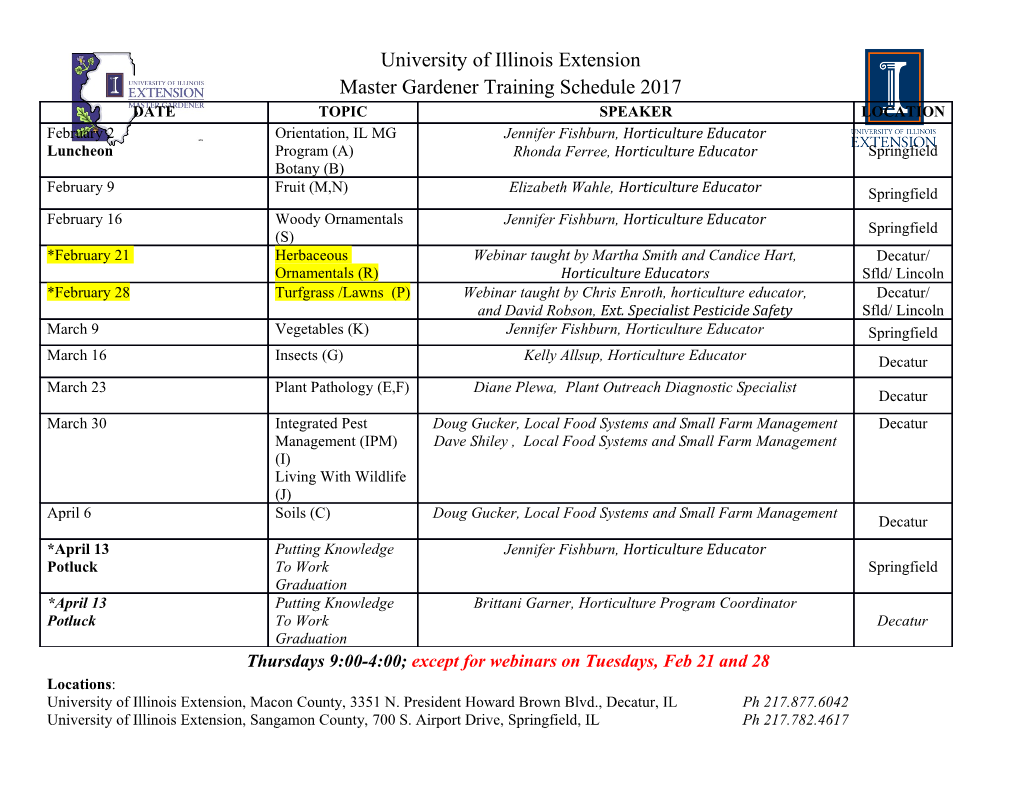
F, 10/04/19 Fall’19 CSCE 629 Lecture 14 Analysis of • Dijkstra’s algorithm: shortest path (non-negative weight) Algorithms Fang Song Texas A&M U Recall: shortest path problem § Input. graph !, node " and # • Every edge has a length )* (can be negative) § Output. $%"#(", #) • Length of a path ) + = ∑*∈/ )* • Distance $%"# 0, 1 = 2%3 )(+) /:5↝7 § Special cases • All edge of equal length: BFS 8(2 + 3) • DAG: DP in topological order 8(2 + 3) Non-negative length: Dijkstra 8((2 + 3)log3) § General: Bellman-Ford 8(23) 1 Dynamic Programing ReRecap 1. Formulate the problem recursively • Overlapping subproblems • May be easy to first compute optimal value & then construct an optimal solution 2. Build solutions to your recurrence • Bottom-up: determine dependencies & find a right order (topo. order in DAG) • To p -down: smart recursion (i.e. without repetition) by momoization Examples • Explicit DAG: shortest path in DAG; longest increasing subsequences (a.k.a. longest path in DAG) • Binary choice: weighted interval scheduling • Multi-way choice: matrix-chain mult.; longest common subsequence; • Adding a variable: shortest path with negative length (Bellman-Ford) 2 Edsger W. Dijkstra § Pioneer in graph algorithms, distributed computing, concurrent computing, programming … § 1984 - 1999 UT Austin, TX § 2002 passed away in Nuenen, Netherlands “What's the shortest way to travel from Rotterdam to Groningen? It is the algorithm for the shortest path, which I designed in about 20 minutes. One morning I was shopping in Amsterdam with my young fiancée, and tired, we sat down on the café terrace to drink a cup of coffee and I was just thinking about whether I could do this, and I then designed the algorithm for the shortest path. ” https://cacm.acm.org/magazines/2010/8/96632-an-interview-with-edsger-w-dijkstra/fulltext 3 Reducing to BFS B D %1 %2 BFS(*) B D A A C E C E B 2 D B D 2 A 1 2 A 3 1 C 4 E C E 4 An alarm-clock algorithm Idea. Convert ; to ;’ by inserting dummy nodes. Run BFS on ;’ B B 100 …… … A 50 A 200 …… C C %&'()*+(-, /) // set alarm clock for 1 at time 0 Repeat until no more alarms; Suppose next alarm goes off at T for node 2 3415 1, 2 ← 7 For each neighbor 8 of 2 If no alarm for 8, set one for time 7 + :(2, 8) Else If current alarm larger, reset it for time 7 + :(2, 8) 5 Dijkstra’s algorithm: priority queue for alarms PriorityQueue Q: set of ! elements w. associated key values (alarm) • Change-key(x). change key value of an element • Delete-min. Return the element with smallest key, and remove it. • Can be done in " log ! time (by a heap) &'()*+,-(/, *) // initialize 2(3) = 0, others 2 6 = ∞ Dijkstra Make Q from V using 2(⋅) as key value "((G + !)log!) While Q not empty "(!log!) Further improvement 6 ← Delete−min @ possible by Fibonacci // pick node with shortest distance to s heap [More to come] For all edges 6, A ∈ C If 2 A > 2 6 + F(6, A) "(Glog!) 2 A ← 2 6 + F(6, A) and Change-key(v) N.B. BFS uses ordinary Queue. Dijkstra = BFS+Priority Queue 6 Demo ! ! B 2 D B 2 D Source A 0 Source A 0 4 4 4 4 B ∞ B 4 A 3 1 1 A 3 1 1 3 C ∞ 3 C 2 2 D ∞ 2 D ∞ 5 C 5 E E ∞ C E E ∞ While Q not empty ( ← Delete−min 1 // pick node with shortest distance to s For all edges (, 3 ∈ 5 If ! 3 > ! ( + 8((, 3) ! 3 ← ! ( + 8((, 3) and Change−key(v) 7 Demo ! ! B 2 D B 2 D Source A 0 Source A 0 4 4 4 4 B 3 B 3 A 3 1 1 A 3 1 1 3 C 3 3 C 3 2 D 6 2 D 5 C 5 E E 7 C 5 E E 6 While Q not empty ) ← Delete−min 2 // pick node with shortest distance to s For all edges ), 4 ∈ 6 If ! 4 > ! ) + 9(), 4) ! 4 ← ! ) + 9(), 4) and Change-key(v) 8 Demo ! ! B 2 D B 2 D Source A 0 Source A 0 4 4 4 4 B 3 B 3 A 3 1 1 A 3 1 1 3 C 3 3 C 3 2 D 5 2 D 5 C 5 E E 6 C 5 E E 6 While Q not empty ( ← Delete−min 1 // pick node with shortest distance to s For all edges (, 3 ∈ 5 If ! 3 > ! ( + 8((, 3) ! 3 ← ! ( + 8((, 3) and Change-key(v) 9 How it fails on negative lengths? # 3 2 ( ! 1 −6 " Jumping to a short one too early! 10 Reflection on Dijkstra: greedy stays ahead § Known region R: in which the shortest distance to ! is known § Growing R: adding # that has the shortest distance to ! § How to Identify #? The one that minimizes % " + '(", #) for " ∈ , Shortest path to some " in known region, followed by a single edge (", #) ! -./0(1, 2) // initialize %(!) = 0, % " = ∞ , R=∅ " While R ≠ 8 Pick # ∉ , w. smallest %(") // by Priority Q Known Add # to , Region # For all edges #, : ∈ ; If % # > % " + '(", #) % # ← % " + '(", #) 11 Contrast with Bellman-Ford § Dijkstra (Greedy) 7 & = min 7 : + 5(:, &) 8∈9 • Positive weight: no need to wait; more edges in a path do not help § Bellman-Ford (Dynamic programming) OPT $, & = min OPT $ − 1, & , min {OPT $ − 1, 3 + 5-→/} -→/∈1 vGlobal vs. Local • Dijkstra’s requires global information: known region & which to add • Bellman-Ford uses only local knowledge of neighbors, suits distributed setting 12 Network routing: distance-vector protocol § Communication network • Nodes: routers • Edges: direct communication links naturally nonnegative, but • Cost of edge: delay on link. Bellman-Ford used anyway! § Distance-vector protocol [“routing by rumor”] • Each router maintains a vector of shortest-path lengths to every other node (distances) and the first hop on each path (directions). • Algorithm: each router performs ! separate computations, one for each potential destination node. § Path-vector protocol: coping with dynamic costs 13.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages14 Page
-
File Size-