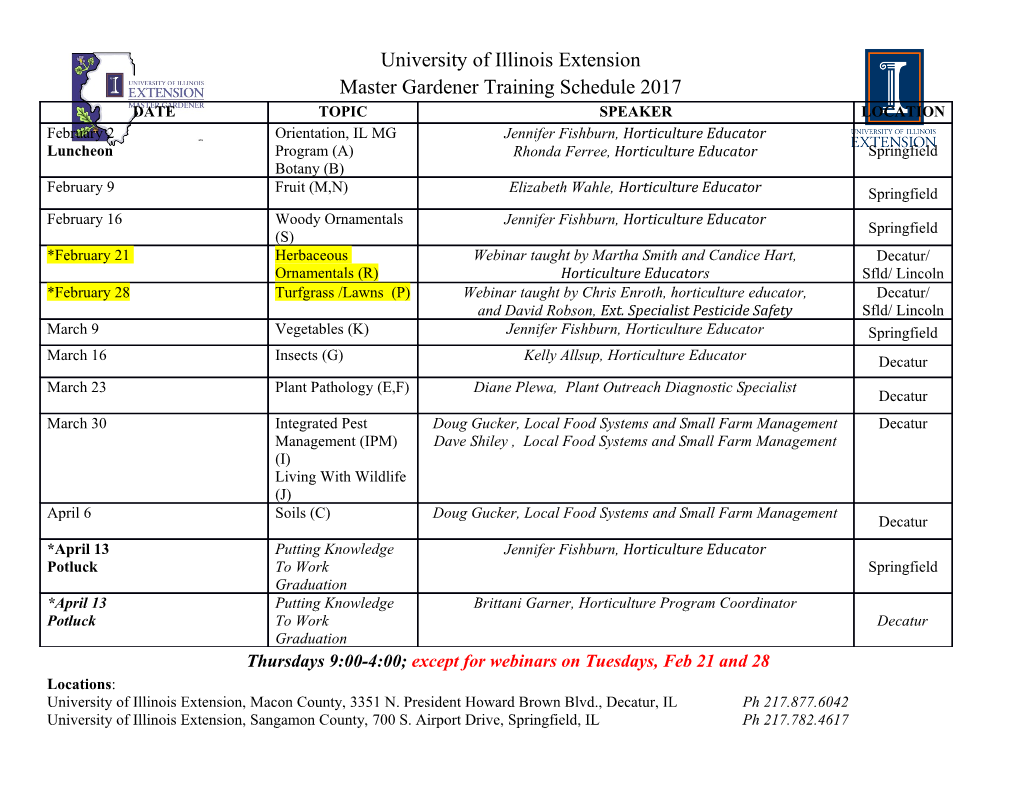
Dive Into Python Python from novice to pro Mark Pilgrim Dive Into Python: Python from novice to pro by Mark Pilgrim Published 20 May 2004 Copyright © 2000, 2001, 2002, 2003, 2004 Mark Pilgrim [mailto:[email protected]] This book lives at http://diveintopython.org/. If you©re reading it somewhere else, you may not have the latest version. Permission is granted to copy, distribute, and/or modify this document under the terms of the GNU Free Documentation License, Version 1.1 or any later version published by the Free Software Foundation; with no Invariant Sections, no Front-Cover Texts, and no Back-Cover Texts. A copy of the license is included in Appendix G, GNU Free Documentation License. The example programs in this book are free software; you can redistribute and/or modify them under the terms of the Python license as published by the Python Software Foundation. A copy of the license is included in Appendix H, Python license. Table of Contents 1. Chapter 1 ........................................................................................................................ 1 Which Python is right for you? ....................................................................................... 1 Python on Windows ..................................................................................................... 1 Python on Mac OS X ................................................................................................... 3 Python on Mac OS 9 .................................................................................................... 4 Python on RedHat Linux ............................................................................................... 5 Python on Debian GNU/Linux ....................................................................................... 6 Python Installation from Source ..................................................................................... 7 The Interactive Shell .................................................................................................... 8 Summary ................................................................................................................... 9 2. Chapter 2 ...................................................................................................................... 10 Diving in .................................................................................................................. 10 Declaring Functions ................................................................................................... 11 How Python©s Datatypes Compare to Other Programming Languages .......................... 11 Documenting Functions .............................................................................................. 12 Everything Is an Object ............................................................................................... 13 The Import Search Path ....................................................................................... 13 What©s an Object? .............................................................................................. 14 Indenting Code .......................................................................................................... 15 Testing Modules ........................................................................................................ 16 3. Chapter 3 ...................................................................................................................... 18 Introducing Dictionaries .............................................................................................. 18 Defining Dictionaries .......................................................................................... 18 Modifying Dictionaries ....................................................................................... 19 Deleting Items From Dictionaries .......................................................................... 20 Introducing Lists ........................................................................................................ 21 Defining Lists ................................................................................................... 21 Adding Elements to Lists ..................................................................................... 23 Searching Lists .................................................................................................. 25 Deleting List Elements ........................................................................................ 26 Using List Operators ........................................................................................... 27 Introducing Tuples ..................................................................................................... 27 Declaring variables ..................................................................................................... 29 Referencing Variables ......................................................................................... 30 Assigning Multiple Values at Once ........................................................................ 30 Formatting Strings ..................................................................................................... 31 Mapping Lists ........................................................................................................... 33 Joining Lists and Splitting Strings ................................................................................. 35 Historical Note on String Methods ........................................................................ 36 Summary .................................................................................................................. 36 4. Chapter 4 ...................................................................................................................... 38 Diving In .................................................................................................................. 38 Using Optional and Named Arguments ........................................................................... 39 Using type, str, dir, and Other Built-In Functions .............................................................. 40 The type Function .............................................................................................. 40 The str Function ................................................................................................ 41 Built-In Functions .............................................................................................. 43 Getting Object References With getattr ........................................................................... 44 getattr with Modules ........................................................................................... 45 getattr As a Dispatcher ........................................................................................ 46 Filtering Lists ............................................................................................................ 47 iii Dive Into Python The Peculiar Nature of and and or ................................................................................. 49 Using the and-or Trick ........................................................................................ 50 Using lambda Functions .............................................................................................. 51 Real-World lambda Functions .............................................................................. 51 Putting It All Together ................................................................................................. 53 Summary .................................................................................................................. 55 5. Chapter 5 ...................................................................................................................... 57 Diving In .................................................................................................................. 57 Importing Modules Using from module import ................................................................ 60 Defining Classes ........................................................................................................ 61 Initializing and Coding Classes ............................................................................. 63 Knowing When to Use self and __init__ ................................................................. 64 Instantiating Classes ................................................................................................... 65 Garbage Collection ............................................................................................ 65 Exploring UserDict: A Wrapper Class ............................................................................ 66 Special Class Methods ................................................................................................ 69 Getting and Setting Items .................................................................................... 70 Advanced Special Class Methods .................................................................................. 72 Introducing Class Attributes ......................................................................................... 74 Private Functions ....................................................................................................... 76 Summary .................................................................................................................
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages359 Page
-
File Size-