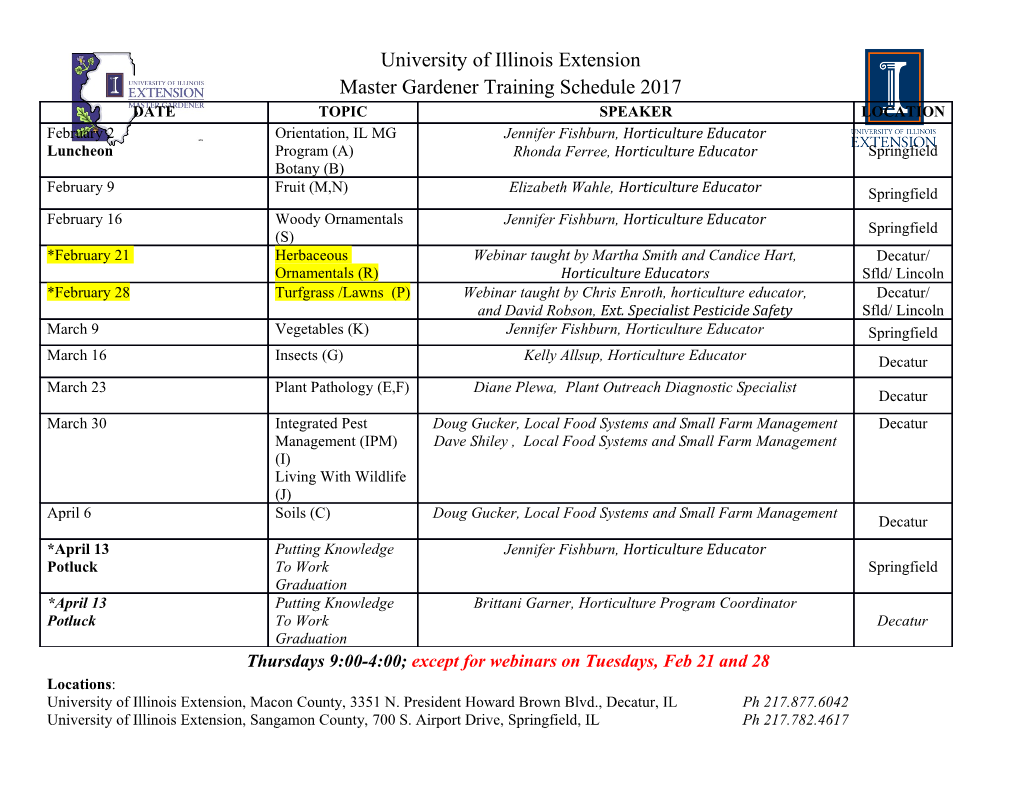
Flask Documentation 0.9dev 2015 06 09 Contents I User’s Guide1 1 Foreword3 1.1 What does “micro” mean?...........................3 1.2 Configuration and Conventions.......................3 1.3 Growing with Flask..............................4 2 Foreword for Experienced Programmers5 2.1 Thread-Locals in Flask.............................5 2.2 Develop for the Web with Caution......................5 2.3 The Status of Python 3.............................6 3 Installation7 3.1 virtualenv....................................7 3.2 System-Wide Installation...........................8 3.3 Living on the Edge...............................9 3.4 pip and distribute on Windows........................9 4 Quickstart 11 4.1 A Minimal Application............................ 11 4.2 Debug Mode.................................. 12 4.3 Routing..................................... 13 4.4 Static Files.................................... 17 4.5 Rendering Templates.............................. 17 4.6 Accessing Request Data............................ 19 4.7 Redirects and Errors.............................. 22 4.8 About Responses................................ 23 4.9 Sessions..................................... 24 4.10 Message Flashing................................ 25 4.11 Logging..................................... 25 4.12 Hooking in WSGI Middlewares....................... 26 4.13 Deploying to a Web Server.......................... 26 i 5 Tutorial 27 5.1 Introducing Flaskr............................... 27 5.2 Step 0: Creating The Folders......................... 28 5.3 Step 1: Database Schema........................... 29 5.4 Step 2: Application Setup Code........................ 29 5.5 Step 3: Creating The Database........................ 31 5.6 Step 4: Request Database Connections.................... 32 5.7 Step 5: The View Functions.......................... 33 5.8 Step 6: The Templates............................. 34 5.9 Step 7: Adding Style.............................. 36 5.10 Bonus: Testing the Application........................ 37 6 Templates 39 6.1 Jinja Setup.................................... 39 6.2 Standard Context................................ 39 6.3 Standard Filters................................. 40 6.4 Controlling Autoescaping........................... 41 6.5 Registering Filters............................... 41 6.6 Context Processors............................... 42 7 Testing Flask Applications 43 7.1 The Application................................ 43 7.2 The Testing Skeleton.............................. 43 7.3 The First Test.................................. 44 7.4 Logging In and Out.............................. 45 7.5 Test Adding Messages............................. 46 7.6 Other Testing Tricks.............................. 46 7.7 Keeping the Context Around......................... 47 7.8 Accessing and Modifying Sessions...................... 48 8 Logging Application Errors 49 8.1 Error Mails................................... 49 8.2 Logging to a File................................ 50 8.3 Controlling the Log Format.......................... 51 8.4 Other Libraries................................. 52 9 Debugging Application Errors 55 9.1 When in Doubt, Run Manually........................ 55 9.2 Working with Debuggers........................... 55 10 Configuration Handling 57 10.1 Configuration Basics.............................. 57 10.2 Builtin Configuration Values......................... 58 10.3 Configuring from Files............................. 60 10.4 Configuration Best Practices......................... 61 10.5 Development / Production.......................... 61 10.6 Instance Folders................................ 62 11 Signals 65 ii 11.1 Subscribing to Signals............................. 65 11.2 Creating Signals................................ 67 11.3 Sending Signals................................. 67 11.4 Signals and Flask’s Request Context..................... 68 11.5 Decorator Based Signal Subscriptions.................... 68 11.6 Core Signals................................... 68 12 Pluggable Views 71 12.1 Basic Principle................................. 71 12.2 Method Hints.................................. 72 12.3 Method Based Dispatching.......................... 73 12.4 Decorating Views................................ 73 12.5 Method Views for APIs............................ 74 13 The Application Context 77 13.1 Purpose of the Application Context..................... 77 13.2 Creating an Application Context....................... 78 13.3 Locality of the Context............................. 78 14 The Request Context 79 14.1 Diving into Context Locals.......................... 79 14.2 How the Context Works............................ 80 14.3 Callbacks and Errors.............................. 80 14.4 Teardown Callbacks.............................. 81 14.5 Notes On Proxies................................ 82 14.6 Context Preservation on Error........................ 82 15 Modular Applications with Blueprints 85 15.1 Why Blueprints?................................ 85 15.2 The Concept of Blueprints........................... 86 15.3 My First Blueprint............................... 86 15.4 Registering Blueprints............................. 86 15.5 Blueprint Resources.............................. 87 15.6 Building URLs................................. 88 16 Flask Extensions 91 16.1 Finding Extensions............................... 91 16.2 Using Extensions................................ 91 16.3 Flask Before 0.8................................. 91 17 Working with the Shell 93 17.1 Creating a Request Context.......................... 93 17.2 Firing Before/After Request......................... 94 17.3 Further Improving the Shell Experience................... 94 18 Patterns for Flask 95 18.1 Larger Applications.............................. 95 18.2 Application Factories............................. 97 18.3 Application Dispatching............................ 99 iii 18.4 Using URL Processors............................. 102 18.5 Deploying with Distribute.......................... 104 18.6 Deploying with Fabric............................. 107 18.7 Using SQLite 3 with Flask........................... 111 18.8 SQLAlchemy in Flask............................. 113 18.9 Uploading Files................................. 117 18.10 Caching..................................... 120 18.11 View Decorators................................ 121 18.12 Form Validation with WTForms....................... 124 18.13 Template Inheritance.............................. 127 18.14 Message Flashing................................ 128 18.15 AJAX with jQuery............................... 131 18.16 Custom Error Pages.............................. 134 18.17 Lazily Loading Views............................. 135 18.18 MongoKit in Flask............................... 137 18.19 Adding a favicon................................ 139 18.20 Streaming Contents.............................. 140 18.21 Deferred Request Callbacks.......................... 142 19 Deployment Options 145 19.1 mod_wsgi (Apache).............................. 145 19.2 Standalone WSGI Containers......................... 148 19.3 uWSGI...................................... 150 19.4 FastCGI..................................... 151 19.5 CGI........................................ 155 20 Becoming Big 157 20.1 Read the Source................................. 157 20.2 Hook. Extend.................................. 157 20.3 Subclass...................................... 157 20.4 Wrap with middleware............................. 158 20.5 Fork........................................ 158 20.6 Scale like a pro.................................. 158 20.7 Discuss with the community.......................... 159 II API Reference 161 21 API 163 21.1 Application Object............................... 163 21.2 Blueprint Objects................................ 182 21.3 Incoming Request Data............................ 186 21.4 Response Objects................................ 188 21.5 Sessions..................................... 189 21.6 Session Interface................................ 190 21.7 Test Client.................................... 192 21.8 Application Globals.............................. 193 21.9 Useful Functions and Classes......................... 193 21.10 Message Flashing................................ 200 iv 21.11 Returning JSON................................ 201 21.12 Template Rendering.............................. 202 21.13 Configuration.................................. 203 21.14 Extensions.................................... 204 21.15 Stream Helpers................................. 205 21.16 Useful Internals................................. 205 21.17 Signals...................................... 208 21.18 Class-Based Views............................... 209 21.19 URL Route Registrations........................... 210 21.20 View Function Options............................ 212 III Additional Notes 215 22 Design Decisions in Flask 217 22.1 The Explicit Application Object........................ 217 22.2 The Routing System.............................. 218 22.3 One Template Engine............................. 218 22.4 Micro with Dependencies........................... 219 22.5 Thread Locals.................................. 220 22.6 What Flask is, What Flask is Not....................... 220 23 HTML/XHTML FAQ 221 23.1 History of XHTML............................... 221 23.2 History of HTML5............................... 222 23.3 HTML versus XHTML............................
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages281 Page
-
File Size-