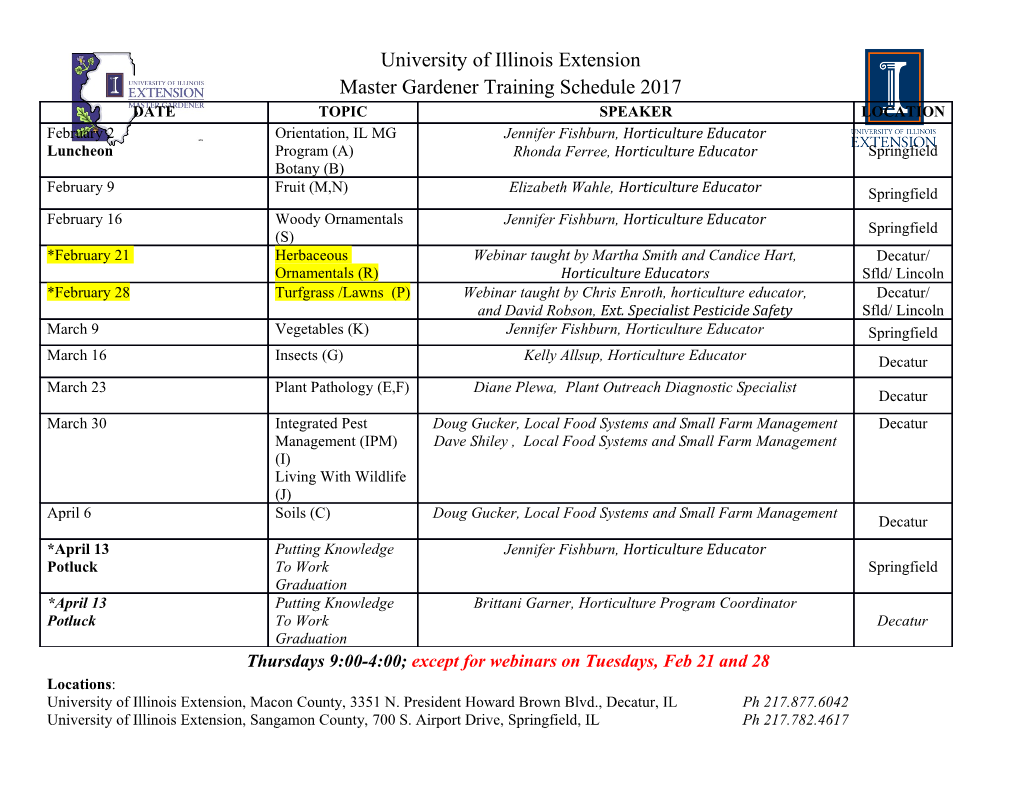
Appendix A Symbolic Computation with Mathematica (for Scalar and Vector Fields) Abstract To enhance concept development, Mathematica combines Lisp (McCarthy et al., LISP I Programmers manual. Tech. rep., Artificial Intelligence Group, M.I.T. Computation Center and Research Laboratory, Cambridge, 1960) and Prolog (Dougherty, Natural language computing: an English generative grammar in prolog. Lawrence Erlbaum, Hilsdale, 1994) structures. Thus codes presented in this textbook for 2D continua can be employed in 3D with minor modifications. All useful Mathematica concepts and syntaxes are explained here. All necessary Mathematica routines are included in the book. The reader does not have to type ASCII source codes. Copy-paste from PDF to Mathematica notebook is encouraged. Readers can easily acquaint themselves with using notations such as: @@, /@, /. They behave like other familiar symbols like: +, * Implicit looping via the Map function makes the code quite readable. The pure function construct with #, & furnishes on-the-fly definitions that are handy and easy to modify. The reader should consult available on-line help files to learn the complete capabilities of symbolic formulations in concept development. For example, Nest, NestList, Fold, FoldList, FixedPoint to name a few accelerate experimentations with algorithms and computer code development. For example: http://www.mathprogramming-intro.org/book/node535.html presents the Gram– Schmidt orthogonalization procedure that is enormously helpful in a multitude of designs of computing procedures. For engineers and general readers the texts (Ruskeepää H, Mathematica naviga- tor: mathematics, statistics and graphics. Elsevier/Academic, Amsterdam/Boston, 2009; Trott, The mathematica guidebook for programming. Springer, New York, 2004) provide an excellent introduction. For physicists (Grozin, Introduction to mathematicaR for physicists. Springer, New York, 2013) is recommended. © Springer Science+Business Media, LLC 2018 205 G. Dasgupta, Finite Element Concepts, DOI 10.1007/978-1-4939-7423-8 206 A Symbolics A.1 Everything Is a Variable If the UNIX system is conceived to be a ‘giant’ word processor, then the finite element method, by the same token, behaves like a general purpose interpolation scheme. The analyst should furnish the order of interpolations from physical considerations to navigate through a ‘third party’ code. Students and practitioners can avoid the back-box approach by judiciously implementing algebraic constructs of concept development by harnessing the power of symbolic computation. Therein, all mathematical structures and operations (even the rules of addition and multipli- cation) are variable and hence, can be modified. This signifies the marked difference between (regular) mathematics and computer mathematics. A.1.1 Changing Addition and Multiplication Rules Will it not be effective and desirable to calculate the sensitivities of a generic solution with respect to parent design parameters? The problem is therefore to obtain the solution, say s.a/; associated with a parent parameter a; when a ! a C b; b W small. Here is how ds=da can be effectively obtained alongside s.a/ by re-defining symbolically addition, multiplication, and exponential operations—as indicated in Listing A.1. In[1]:= Global\[RawBackquote]b In[2]:= Unprotect[Expand]; Expand[x_[a_. + y_. b]] := ( x[a]+ybx’[a])/;( x =!= Plus && x =!= Times ) Protect[Expand]; In[5]:=Unprotect[Power, Times]; (a_+y_.b)^n_:=a^n+na^(n-1)yb 0.b:=0 b^n_/;n>1:=0 a_./(c_ + d_ b) := (a/c) (1 - (d/c) b) Times[(x_ + y_ b), (xx_ + yy_ b)] := x xx + (xx *y+yy* x) b Times[c_ , (x_ + y_ b)] := x c + y c b Protect[Power, Times]; In[13]:=$Post = Expand Listing A.1 Sensitivity analysis; by changing definitions of Times and Power First time readers can skip the Mathematica syntax in Listing A.1. An eigenvalue computation example can be cited when the code in Listing A.1 is pre-loaded. Let us start with a random symmetric matrix: A.1 Variables 207 Ä 77092 274 117; 99 calculated eigenvalues are: and Œ 383 383 x D 99; 85 I (A.1) using the Mathematica function Eigenvalues Now Œx is perturbed to Œy: Ä 407 b 77092 295 b 274 117 99 C b eigenvalues: C and C Œy D I 702 383 702 383 99 C b 85 after the first order perturbation with a ‘small’ b (A.2) The result can be organized, for the eigenvalue solution function s,as: 8 9 8 9 77092 Áˇ 407 ˆ > ˇ ˆ > Á < 383 = @s Œx ˇ <702= s Œx ˇ D ˆ > and @ ˇ D ˆ > (A.3) :ˆ 274 ;> b ˇ :ˆ295;> bD0 383 702 when b is the perturbation on the diagonal terms. The aforementioned idea can be generalized for more than one perturbation variable. The important point to be recognized in this example is that even the standard rules of arithmetic operations can be modified as needed. These capabilities in Mathematica have contributed to the concept of Computer Mathematics where ‘restrictions’ in conventional mathematics can be conveniently bypassed. A useful example with vectors is now presented. 8 9 <a= in Mathematica: v={a,b,c}I with the List operator: v f g D :b; I v=List[a,b,c] where List is the function name c the arguments are encased in square brackets [ and ] With a scalar t; using the in-fix notation in classical mathematics: 8 9 <t a= v v t C f g is undefinedI but t f g is :t b; I however, (A.4a) t c 8 9 <a C t= in Mathematica : v+t yields List[a+t, b+t, c+t]: :b C t; (A.4b) c C t The argument is that by treating and C as symbols Mathematica extends the same idea without jeopardizing any other mathematical concept. For example, For amatrixŒm, Mathematica defines t C Œm accordingly. 208 A Symbolics A.1.2 Rewriting Listing A.1 as a Mathematica Package Let us note that a user can define ‘structured functions’ in Mathematica utilizing the BeginPackage[] constructs, as in Listing A.2. BeginPackage["FirstOrderWithEpsilon\[RawBackquote]", "Global\[RawBackquote]"] (*copyright 1994, Gautam Dasgupta *) FirstOrderWithEpsilon::usage = "FirstOrderWithEpsilon[x] sets up rules for first order arithmetic." FirstOrderPerturbation::usage = "FirstOrderPerturbation[\[Lambda]] sets up rules for first order perturbation with \[Lambda]." Begin["\[RawBackquote]Private\[RawBackquote]"] FirstOrderWithEpsilon[\[Epsilon]_] := Module[{headers}, headers = {Plus, Times, Equal}; Unprotect[Expand]; Expand[x_[a_. + b_. \[Epsilon]]] := (x[a]+b\[Epsilon] x’[a]) /; ( Not[ MemberQ[headers, x]] ); Protect[Expand]; Unprotect[Power, Times]; (a_ + b_. \[Epsilon])^n_ := a^n+na^(n-1)b\[Epsilon]; 0. \[Epsilon] := 0; \[Epsilon]^n_ /; n > 1 := 0; a_./(c_ + d_ \[Epsilon]) := (a/c) (1 - (d/c) \[Epsilon]) ; Times[(c_ + d_ \[Epsilon]), (a_ + b_ \[Epsilon])] := a c + (a d + b c) \[Epsilon] ; Times[c_ , (a_ + b_ \[Epsilon])] := a c + b c \[Epsilon] ; Protect[Power, Times]; $Post = Expand; ] FirstOrderPerturbation[\[Lambda]_] := FirstOrderWithEpsilon[\[Lambda]] End[] EndPackage[] Listing A.2 Do not use this code. First time readers—focus only on the structures with [RawBackquote]: Begin and End, BeginPackage and EndPackage.Use Listing A.3 for testing To execute Mathematica we should also use [RawDoubleQuote] as in Listing A.3. A.1 Variables 209 A.1.3 Rewriting Listing A.1 for Use We can copy-paste Listing A.3 on a Mathematica notebook. BeginPackage[ \[RawDoubleQuote]FirstOrderWithEpsilon\[RawBackquote] \[RawDoubleQuote],\[RawDoubleQuote]Global\[RawBackquote] \[RawDoubleQuote] ] (*copyright 1994, Gautam Dasgupta *) FirstOrderWithEpsilon::usage = "FirstOrderWithEpsilon[x] sets up rules for first order arithmetic." FirstOrderPerturbation::usage = "FirstOrderPerturbation[\[Lambda]] sets up rules for first order perturbation with \[Lambda]." Begin[ \[RawDoubleQuote]\[RawBackquote]Private\[RawBackquote] \[RawDoubleQuote] ] FirstOrderWithEpsilon[\[Epsilon]_] := Module[{headers}, headers = {Plus, Times, Equal}; Unprotect[Expand]; Expand[x_[a_. + b_. \[Epsilon]]] := (x[a]+b\[Epsilon] x’[a]) /; ( Not[ MemberQ[headers, x]] ); Protect[Expand]; Unprotect[Power, Times]; (a_ + b_. \[Epsilon])^n_ := a^n+na^(n-1)b\[Epsilon]; 0. \[Epsilon] := 0; \[Epsilon]^n_ /; n > 1 := 0; a_./(c_ + d_ \[Epsilon]) := (a/c) (1 - (d/c) \[Epsilon]) ; Times[(c_ + d_ \[Epsilon]), (a_ + b_ \[Epsilon])] := a c + (a d + b c) \[Epsilon] ; Times[c_ , (a_ + b_ \[Epsilon])] := a c + b c \[Epsilon] ; Protect[Power, Times]; $Post = Expand; ] FirstOrderPerturbation[\[Lambda]_] := FirstOrderWithEpsilon[\[Lambda]] End[] EndPackage[] Listing A.3 Use this code with [RawBackquote] and [RawDoubleQuote] As in the case of a built-in function: ?FirstOrderWithEpsilon reproduces the usage message provided for FirstOrderWithEpsilon: 210 A Symbolics A.2 A Mathematica Overview All necessary Mathematica routines are included in the book. The reader does not have to type. Take a PDF and run an OCR1 and obtain the source code in ASCII2 characters. In the early chapters, Mathematica Greek characters are not used (some PDF readers improperly interpret special characters). The user can input a Greek character; for example, by typing \[Alpha] the character ˛ is generated. There is a short-cut to typing Greek characters; the escape key esc should be pressed before and after a to get ˛ on the screen. Similarly esc b esc will write ˇ and the palettes pull-down menu in Mathematica facilitates the input of special mathematical characters and symbols. A.2.1 Input and Output The notebook frontend is the development environment in Mathematica with a complete user-interface. It generates a file with extension .nb as a record of the session activity. In addition, the compact version is saved with the .m extension. The frontend communicates with the Mathematica engine, which is called the kernel, via a two-way connection provided by the program MathLink.Thefrontend allows user-friendly syntax but the kernel stores information input data in an unambiguous rigorous form akin to the LISP programming language, as shown in Listing A.4. In[1]:= a = x/Sqrt[5] (*define a to be x divided by square root of 5 *) Out[1]= x/Sqrt[5] In[2]:= FullForm[a] Out[2]//FullForm= Times[Power[5,Rational[-1,2]],x] Listing A.4 FullForm operation 1Optical Character Recognition program.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages127 Page
-
File Size-