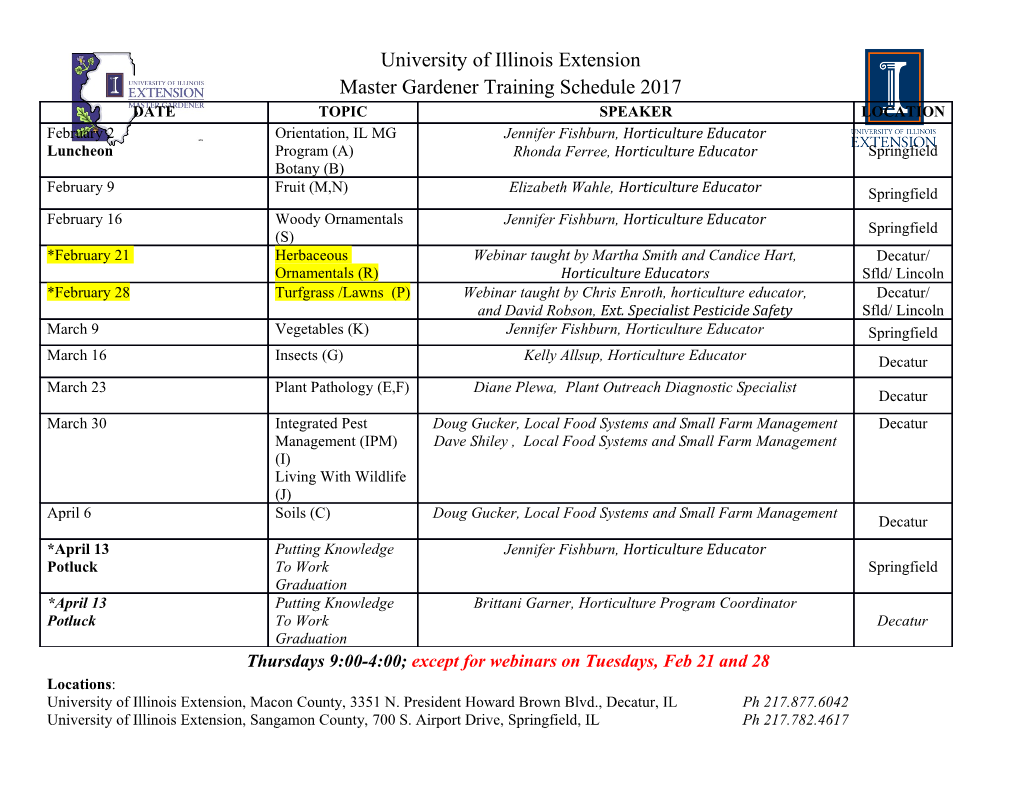
UCLA Extension Course OO Programming with C++ Design Principles and Guidelines Overview UCLA Extension Course Design Principles Software Design Principles and Guidelines – Important design concepts – Useful design principles Douglas C. Schmidt Development Methodologies Department of Electrical Engineering and Computer Science Vanderbilt University – Traditional approaches [email protected] – Extreme programming Design Guidelines http://www.cs.wustl.edu/ schmidt/ – Motivation – Common Design Mistakes – Design Rules Copyright c 1997-2003 Vanderbilt University 1 UCLA Extension Course OO Programming with C++ UCLA Extension Course OO Programming with C++ Motivation: Goals of the Design Phase Motivation: Goals of the Design Phase (cont’d) Decompose System into Modules Specify Module Interfaces – i.e., identify the software architecture – Modules are abstractions that should: – Interfaces should be well-defined be independent, facilitate independent module testing have well-specified interfaces, and improve group communication have high cohesion and low coupling. Describe Module Functionality Determine Relations Between Modules – Informally – Identify module dependencies e.g., comments or documentation – Determine the form of intermodule communication, e.g., – Formally global variables e.g., via module interface specification languages parameterized function calls shared memory RPC or message passing Copyright c 1997-2003 Vanderbilt University 2 Copyright c 1997-2003 Vanderbilt University 3 UCLA Extension Course OO Programming with C++ UCLA Extension Course OO Programming with C++ Primary Design Phases Key Design Concepts and Principles Preliminary Design Important design concepts and design principles include: – External design describes the real-world model – Decomposition – Architectural design decomposes the requirement specification – Abstraction into software subsystems – Information Hiding – Modularity Detailed Design – Hierarchy – Formally specify each subsystem – Separating Policy and Mechanism – Further decomposed subsystems, if necessary Main purpose of these concepts and principles is to manage software Note: in design phases the orientation moves system complexity and improve software quality factors. – from customer to developer – from what to how Copyright c 1997-2003 Vanderbilt University 4 Copyright c 1997-2003 Vanderbilt University 5 UCLA Extension Course OO Programming with C++ UCLA Extension Course OO Programming with C++ Decomposition Decomposition (cont’d) Decomposition is a concept common to all life-cycle and design Some guiding decomposition principles techniques. – Because design decisions transcend execution time, modules Basic concept is very simple: might not correspond to execution steps . – Decompose so as to limit the effect of any one design decision on 1. Select a piece of the problem (initially, the whole problem) the rest of the system 2. Determine its components using the mechanism of choice, e.g., – Remember, anything that permeates the system will be expensive functional vs data structured vs object-oriented to change 3. Show how the components interact – Modules should be specified by all information needed to use the 4. Repeat steps 1 through 3 until some termination criteria is met module and nothing more (e.g., customer is satisfied, run out of money, etc.;-)) Copyright c 1997-2003 Vanderbilt University 6 Copyright c 1997-2003 Vanderbilt University 7 UCLA Extension Course OO Programming with C++ UCLA Extension Course OO Programming with C++ Abstraction Abstraction (cont’d) Abstraction provides a way to manage complexity by emphasizing Three basic abstraction mechanisms essential characteristics and suppressing implementation details. – Procedural abstraction Allows postponement of certain design decisions that occur at e.g., closed subroutines various levels of analysis, e.g., – Data abstraction e.g.,ADTs – Representational/Algorithmic considerations – Control abstraction – Architectural/Structural considerations iterators, loops, multitasking, etc. – External/Functional considerations Copyright c 1997-2003 Vanderbilt University 8 Copyright c 1997-2003 Vanderbilt University 9 UCLA Extension Course OO Programming with C++ UCLA Extension Course OO Programming with C++ Information Hiding Information Hiding (cont’d) Motivation: details of design decisions that are subject to change Information to be hidden includes: should be hidden behind abstract interfaces, i.e., modules. – Data representations – Information hiding is one means to enhance abstraction. i.e., using abstract data types – Algorithms e.g., sorting or searching techniques Modules should communicate only through well-defined interfaces. – Input and Output Formats Each module is specified by as little information as possible. Machine dependencies, e.g., byte-ordering, character codes – Policy/mechanism distinctions If internal details change, client modules should be minimally affected i.e., when vs how (may require recompilation and relinking, however . .) e.g., OS scheduling, garbage collection, process migration – Lower-level module interfaces e.g., Ordering of low-level operations, i.e., process sequence Copyright c 1997-2003 Vanderbilt University 10 Copyright c 1997-2003 Vanderbilt University 11 UCLA Extension Course OO Programming with C++ UCLA Extension Course OO Programming with C++ Modularity Modularity (cont’d) A Modular System is a system structured into highly independent Modularity facilitates certain software quality factors, e.g.: abstractions called modules. – Extensibility - well-defined, abstract interfaces Modularity is important for both design and implementation phases. – Reusability - low-coupling, high-cohesion – Compatibility - design “bridging” interfaces Module prescriptions: – Portability - hide machine dependencies – Modules should possess well-specified abstract interfaces. Modularity is an important characteristic of good designs because it: – Modules should have high cohesion and low coupling. – allows for separation of concerns – enables developers to reduce overall system complexity via decentralized software architectures – enhances scalability by supporting independent and concurrent development by multiple personnel Copyright c 1997-2003 Vanderbilt University 12 Copyright c 1997-2003 Vanderbilt University 13 UCLA Extension Course OO Programming with C++ UCLA Extension Course OO Programming with C++ Modularity (cont’d) Modularity (cont’d) A module is A module interface consists of several sections: – A software entity encapsulating the representation of an – Imports abstraction, e.g.,anADT Services requested from other modules – A vehicle for hiding at least one design decision – Exports – A “work” assignment for a programmer or group of programmers Services provided to other modules – a unit of code that – Access Control has one or more names not all clients are equal! (e.g., C++’s distinction between has identifiable boundaries protected/private/public) can be (re-)used by other modules – Heuristics for determining interface specification encapsulates data define one specification that allows multiple implementations hides unnecessary details anticipate change can be separately compiled (if supported) e.g., use structures and classes for parameters Copyright c 1997-2003 Vanderbilt University 14 Copyright c 1997-2003 Vanderbilt University 15 UCLA Extension Course OO Programming with C++ UCLA Extension Course OO Programming with C++ Modularity Dimensions Principles for Ensuring Modular Designs Modularity has several dimensions and encompasses specification, design, and implementation levels: Language Support for Modular Units – Criteria for evaluating design methods with respect to modularity – Modules must correspond to syntactic units in the language used. Modular Decomposability Few Interfaces Modular Composability Modular Understandability – Every module should communicate with as few others as possible. Modular Continuity Small Interfaces (Weak Coupling) Modular Protection – Principles for ensuring modular designs: – If any two modules communicate at all, they should exchange as Language Support for Modular Units little information as possible. Few Interfaces Small Interfaces (Weak Coupling) Explicit Interfaces Information Hiding Copyright c 1997-2003 Vanderbilt University 16 Copyright c 1997-2003 Vanderbilt University 17 UCLA Extension Course OO Programming with C++ UCLA Extension Course OO Programming with C++ Principles for Ensuring Modular Designs (cont’d) The Open/Closed Principle Explicit Interfaces A satisfactory module decomposition technique should yield modules that are both open and closed: – Whenever two modules A and B communicate, this must be obvious from the text of A or B or both. – Open Module: is one still available for extension. This is necessary because the requirements and specifications are rarely completely Information Hiding understood from the system’s inception. – All information about a module should be private to the module – Closed Module: is available for use by other modules, usually unless it is specifically declared public. given a well-defined, stable description and packaged in a library. This is necessary because otherwise code sharing becomes unmanageable because reopening a module may trigger changes in many clients. Copyright c 1997-2003 Vanderbilt University 18 Copyright c 1997-2003 Vanderbilt University 19 UCLA Extension Course OO Programming with C++ UCLA Extension Course OO
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages12 Page
-
File Size-