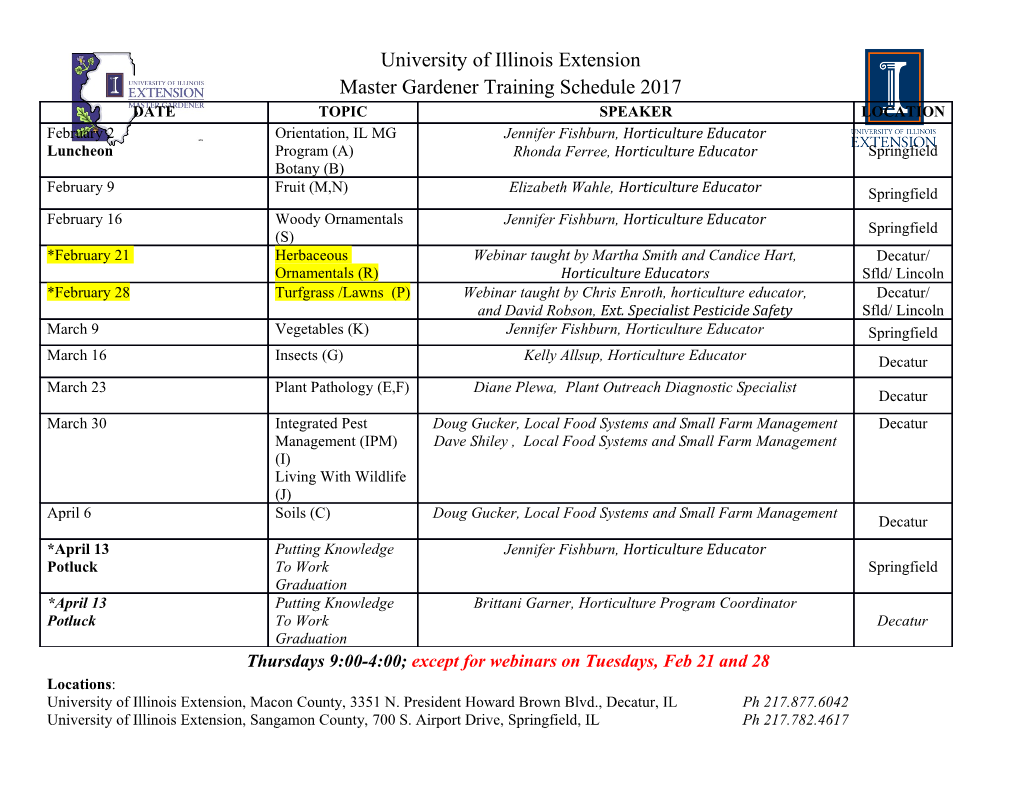
1 By Joe Mayo Foreword by Daniel Jebaraj 2 Copyright © 2015 by Syncfusion, Inc. 2501 Aerial Center Parkway Suite 200 Morrisville, NC 27560 USA All rights reserved. I mportant licensing information. Please read. This book is available for free download from www.syncfusion.com on completion of a registration form. If you obtained this book from any other source, please register and download a free copy from www.syncfusion.com. This book is licensed for reading only if obtained from www.syncfusion.com. This book is licensed strictly for personal or educational use. Redistribution in any form is prohibited. The authors and copyright holders provide absolutely no warranty for any information provided. The authors and copyright holders shall not be liable for any claim, damages, or any other liability arising from, out of, or in connection with the information in this book. Please do not use this book if the listed terms are unacceptable. Use shall constitute acceptance of the terms listed. SYNCFUSION, SUCCINCTLY, DELIVER INNOVATION WITH EASE, ESSENTIAL, and .NET ESSENTIALS are the registered trademarks of Syncfusion, Inc. Technical Reviewer: Stephen Haunts Copy Editor: Ben Ball Acquisitions Coordinator: Hillary Bowling, marketing coordinator, Syncfusion, Inc. Proofreader: Graham High, content producer, Syncfusion, Inc. 3 Table of Contents The Story behind the Succinctly Series of Books ................................................................................... 7 About the Author ......................................................................................................................................... 9 Chapter 1 Introducing C# and .NET ....................................................................................................... 10 What can I do with C#? .......................................................................................................................... 10 What is .NET? ........................................................................................................................................ 10 Writing, Running, and Deploying a C# Program .................................................................................... 11 Starting a New Program ................................................................................................................... 11 Namespaces and Code Organization .................................................................................................... 12 Running the Program ........................................................................................................................ 14 Deploying the Program ..................................................................................................................... 15 Summary ................................................................................................................................................ 16 Chapter 2 Coding Expressions and Statements ................................................................................... 17 Writing Simple Statements..................................................................................................................... 17 Overview of C# Types and Operators ................................................................................................... 18 Operator Precedence and Associativity ................................................................................................. 22 Formatting Strings .................................................................................................................................. 22 Branching Statements ............................................................................................................................ 23 Arrays and Collections ........................................................................................................................... 25 Looping Statements ............................................................................................................................... 26 Wrapping Up .......................................................................................................................................... 28 Summary ................................................................................................................................................ 30 Chapter 3 Methods and Properties ........................................................................................................ 31 Starting at Main ...................................................................................................................................... 31 Modularizing with Methods .................................................................................................................... 31 4 Simplifying Code with Methods .............................................................................................................. 34 Adding Properties .................................................................................................................................. 34 Exception Handling ................................................................................................................................ 37 Summary ................................................................................................................................................ 41 Chapter 4 Writing Object-Oriented Code ............................................................................................... 42 Implementing Inheritance....................................................................................................................... 42 Access Modifiers and Encapsulation ..................................................................................................... 44 Designing Types: Class vs. Struct ......................................................................................................... 44 Creating Enums ..................................................................................................................................... 48 Enabling Polymorphism ......................................................................................................................... 49 Writing Abstract Classes ........................................................................................................................ 53 Exposing Interfaces ............................................................................................................................... 54 Object Lifetime ....................................................................................................................................... 56 Summary ................................................................................................................................................ 61 Chapter 5 Handling Delegates, Events, and Lambdas ......................................................................... 62 Referencing Methods with Delegates .................................................................................................... 62 Firing Events .......................................................................................................................................... 63 Working with Lambdas ........................................................................................................................... 65 More FCL Delegate Types ..................................................................................................................... 68 Expression-Bodied Members ................................................................................................................. 69 Summary ................................................................................................................................................ 70 Chapter 6 Working with Collections and Generics .............................................................................. 71 Using Collections ................................................................................................................................... 71 Writing Generic Code ............................................................................................................................. 74 Summary ................................................................................................................................................ 79 Chapter 7 Querying Objects with LINQ .................................................................................................. 80 Getting Started ....................................................................................................................................... 80 5 Querying Collections .............................................................................................................................. 81 Filtering Data .......................................................................................................................................... 83 Ordering Collections .............................................................................................................................. 84 Joining Objects ...................................................................................................................................... 84 Using Standard Operators ..................................................................................................................... 85 Summary
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages100 Page
-
File Size-