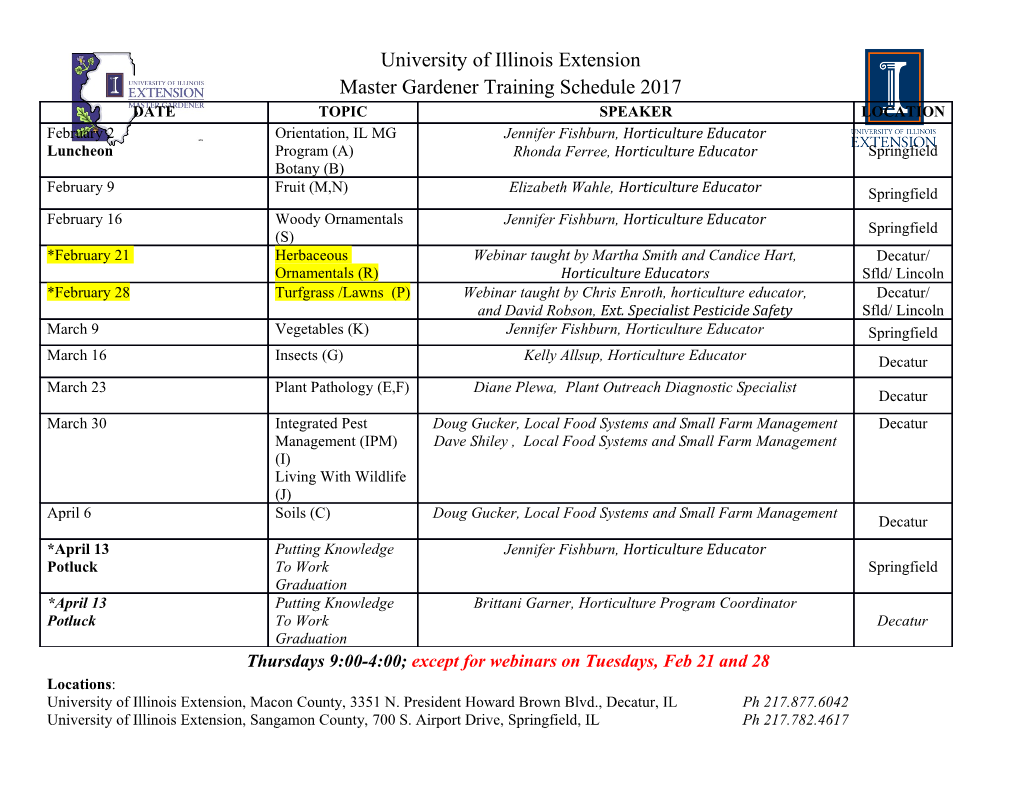
Introduction to Computational Complexity Introduction In the lecture on Turing reducibility, we witnessed how an instance of problem A can be solved by successively solving instances of another problem B. This represents a \positive" use of reducibility, in that an algorithm for one problem leads to an algorithm for another. In this lecture we look at a \negative" use of reducibility, in the sense that a reduction from a hard problem A (i.e. a problem for which no known efficient algorithm exists) to a problem B lends evidence to the belief that B is also hard. Despite obtaining a \negative" result, this approach seems quite useful, since many problems encountered in practice are inherently (but often not provably) difficult, and reducibility helps lend supporting evidence. The logic of negative reducibility proceeds as follows. Suppose it is believed, if not proved, that there is no efficient (e.g. polynomial-time) algorithm for solving problem A. Now suppose A can be efficiently reduced to problem B. Then if there is an efficient algorithm for solving B, then it implies an efficient algorithm for solving A. Therefore, it must be believed, if not proved, that no such algorithm for solving B exists. Working with Decision Problems Based on the kind of reducibility that will be employed in this lecture, it will seem more convenient to assume that each problem is a decision problem; i.e. a problem whose solution involves returning a true or false value. For example, SAT, CNF-SAT, k-SAT, 3SAT, 2SAT, and Perfect Matching are all examples of decision problems. Moreover, even a problem whose solution involves determining a structure or numerical value can be defined as a decision problem. For example, the problem of finding a minimum spanning tree for a weighted connected graph G can be defined as the problem of deciding if G has a spanning tree of cost not exceeding k, for some integer k. Thus, by introducing an additional parameter k, the minimum-spanning tree optimization problem is transformed into a decision problem. 1 Example 1. Recall that the Minimum Spanning Tree (MST) problem takes as input a connected weighted graph G = (V; E; w) and returns a spanning tree for G whose edge weights sum to a minimum value. Re-define this as a decision problem. 2 It should be emphasized that there is little if anything lost by treating a problem as a decision problem. For example, if the original problem involves finding a structure that optimizes some quantity, and its corresponding decision problem can be efficiently solved, then almost invariably the original problem can also be efficiently solved. Example 2. Suppose the MST decision problem can also be solved in polynomial time. Show that its corresponding optimization problem can be solved in polynomial time, and that an mst can be constructed in polynomial time. 3 When working with decision problem L, we often replace the phrase \L can be solved" with that of \L can be decided". Similarly, solvable decision problems are often referred to as being decidable. Many-to-one Reducibility Given a decision problem L and a problem instance x of L, we call x a positive instance of L, if an algorithm for solving L returns true on input x. Similarly, x a negative instance of L, if an algorithm for solving L returns false on input x. For example, x1 _ x2 is a positive instance of SAT, while x1 ^ x1 is a negative instance of SAT. Let L1 and L2 be two decision problems. We say that L1 is many-to-one, polynomial-time p reducible to L2, written as L1 ≤m L2, iff there exists a function f : L1 ! L2 that satisfies the following properties. 1. f(x) is polynomial-time computable in the size jxj of an L1-problem instance x. 2. f(x) is a positive instance of L2 iff x is a positive instance of L1. Theorem 1. The Clique problem is the problem of deciding if a simple graph G = (V; E) has a clique of size k, for some integer k ≥ 0; i.e. a subset C ⊆ V of k vertices that are pairwise adjacent. Show that 3SAT is polynomial-time many-to-one reducible to Clique. Proof of Theorem 1. Let C be a collection of m clauses, where clause ci, 1 ≤ i ≤ m, has the form ci = li1 _ li2 _ li3. We proceed to construct a simple graph f(C) = G = (V; E) that has an m-clique if and only if C is satisfiable. G is defined as follows. V consists of 3m vertices, one for each literal lij, 1 ≤ i ≤ m and 1 ≤ j ≤ 3. Then (lij; lrs) 2 E iff i 6= r and lij is not the negation of lrs (i.e. they are logically consistent). Then G can be constructed from C in time polynomial in the size of C, since the order of G is m 3m, and the size of G is not more than 9 = O(m2). 2 Now assume, that C is satisfiable. Given a satisfying assignment for C, let liji , 1 ≤ i ≤ m, de- note a literal from clause i that is satisfied by this assignment. Then, since each pair of these literals is consistent, (liji ; lrjr ) 2 E for all i 6= r. In other words, l1j1 ; l2j2 ; : : : ; lmjm is an m-clique for G. Conversely, assume G has an m-clique. Then the clique must have the form R = fl1j1 ; l2j2 ; : : : ; lmjm g, since no two literals from the same clause are adjacent. Moreover, by the definition of G, R is a consistent set of literals and the i th literal of R is a member of ci. Hence the assignment aR induced by R satisifes every clause of C, and thus C is satisfiable. 4 Example 3. Show the reduction given in the previous example using C = f(x1; x1; x2); (x1; x2; x2); (x1; x2; x2)g: 5 The Complexity Class P A complexity class represents a set of decision problems, each of which can be solved within a certain limit placed on time and space (i.e. memory). For example, the complexity class P denotes all decision problems that can be solved in a polynomial amount of time. More specifically, decision problem L is a member of P iff there exists a predicate function P (x) for which 1 if x is a positive instance of L P (x) = 0 otherwise and P (x) can be decided in an amount of time bounded by p(jxj), for some polynomial p. Example 4. From previous lectures we may conclude that the decision-problem versions of the the following problems belong to class P: Minimum Spanning Tree, Single Source Distances, Unit Task Scheduling, Huffman Coding, Fuel Reloading, Fractional Knapsack, Find Statistic, Find Median, Minimum Distance Pair, Addition, Multiplication, Matrix Multiplication, Minimum Subsequence Sum, Edit Distance, Optimal Binary Search Tree, Matrix Chain Multiplication, DAG Longest Path, Optimal Change, All-Pairs Distances, 2SAT, Maximum Matching, Maximum Flow. The Complexity Class NP Decision problem L is a member of complexity class NP iff there exists a polynomial-time decidable predicate P (x; y), such that x is a positive instance of L iff the predicate 9yP (x; y) evaluates to true, where the domain elements of y that are tested in P satisfy jyj ≤ p(jxj), for some polynomial p. Although, for any given value of y, P (x; y) can be be decided in polynomial time, we see that the addition of quantifier 9y adds a potential order of complexity to the deciding of x, since it may be necessary to compute P (x; y) an exponential number of times in order to find an appropriate witness y0, for which P (x; y0) evaluates to true. This is true since there can potentially exist an exponential number of domain values of y whose size does not exceed p(jxj), for some polynomial p, and hence it may be necessary to evaluate P (x; y) an exponential number of times before identifying a witness. 6 Example 5. We show that SAT 2 NP. Given Boolean formula instance F (x1; : : : ; xn) of SAT, let y be a variable whose domain consists of all possible assignments α over V = fx1; : : : ; xng. Then F is satisfiable iff 9y eval(F; y) evaluates to true. Moreover, from the previous lecture, we know that eval(F; y) can be decided in time proportional to jF j. Moreover, each assignment α 2 dom(y) satisfies jαj = n ≤ jF j. Therefore, SAT 2 NP. 7 Example 6. An instance of Clique is i) a simple graph G = (V; E), and ii) a nonnegative integer k ≤ jV j = n. The problem is to decide if G has a clique of size at least k. Show that Clique 2 NP. 8 Theorem 2. P ⊆ NP. Proof of Theorem 2. Let L 2 P be given. Then there is a polynomial-time computable predicate function P (x) that decides L. Let y be a variable for which dom(y) = f1g consists of a single element. Define predicate function P 0(x; y) as P 0(x; y) = P (x). Then P 0 is polynomial-time computable, and x is a positive instance of L iff P (x) evaluates to true, iff P 0(x; 1) evlauates to true, iff 9yP (x; y) evlauates to true. Therefore, by definition, L 2 NP. Do there exist decision problems that are in NP but not in P? This would imply that the use of the 9 operation is necessary for solving some problems with a polynomial-time predicate function.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages34 Page
-
File Size-