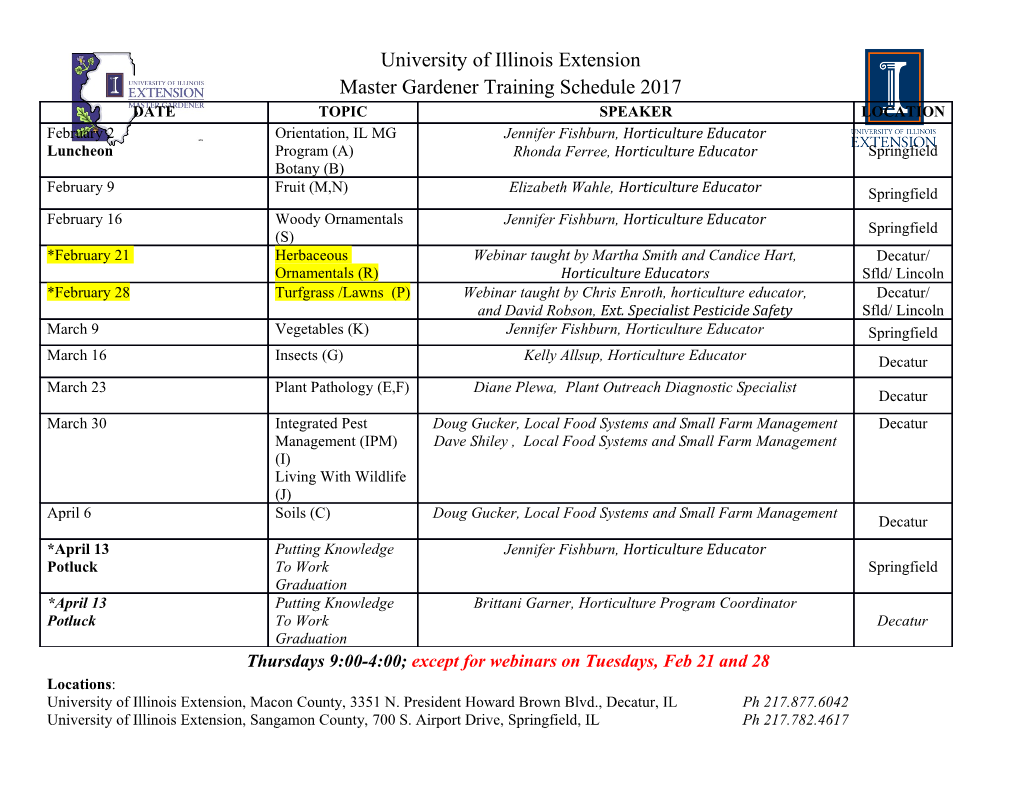
C++: Operator Overloading in C++ Operator overloading is an important concept in C++. It is a type of polymorphism in which an operator is overloaded to give user defined meaning to it. Overloaded operator is used to perform operation on user-defined data type. For example '+' operator can be overloaded to perform addition on various data types, like for Integer, String(concatenation) etc. Almost any operator can be overloaded in C++. However there are few operator which can not be overloaded. Operator that are not overloaded are follows • scope operator - :: • sizeof • member selector - . • member pointer selector - * • ternary operator - ?: Operator Overloading Syntax Implementing Operator Overloading in C++ Operator overloading can be done by implementing a function which can be : 1. Member Function 2. Non-Member Function 3. Friend Function Operator overloading function can be a member function if the Left operand is an Object of that class, but if the Left operand is different, then Operator overloading function must be a non- member function. Operator overloading function can be made friend function if it needs access to the private and protected members of class. Restrictions on Operator Overloading in C++ Following are some restrictions to be kept in mind while implementing operator overloading. 1. Precedence and Associativity of an operator cannot be changed. 2. Arity (numbers of Operands) cannot be changed. Unary operator remains unary, binary remains binary etc. 3. No new operators can be created, only existing operators can be overloaded. 4. Cannot redefine the meaning of a procedure. You cannot change how integers are added. Overloading Arithmetic Operator in C++ Arithmetic operator are most commonly used operator in C++. Almost all arithmetic operator can be overloaded to perform arithmetic operation on user-defined data type. In the below example we have overridden the + operator, to add to Time(hh:mm:ss) objects. Example: overloading + Operator to add two Time class object #include <iostream.h> #include <conio.h> class Time { int h,m,s; public: Time() { h=0, m=0; s=0; } void setTime(); void show() { cout<< h<< ":"<< m<< ":"<< s; } //overloading '+' operator Time operator+(time); }; Time Time::operator+(Time t1) //operator function { Time t; int a,b; a = s+t1.s; t.s = a%60; b = (a/60)+m+t1.m; t.m = b%60; t.h = (b/60)+h+t1.h; t.h = t.h%12; return t; } void time::setTime() { cout << "\n Enter the hour(0-11) "; cin >> h; cout << "\n Enter the minute(0-59) "; cin >> m; cout << "\n Enter the second(0-59) "; cin >> s; } void main() { Time t1,t2,t3; cout << "\n Enter the first time "; t1.setTime(); cout << "\n Enter the second time "; t2.setTime(); t3 = t1 + t2; //adding of two time object using '+' operator cout << "\n First time "; t1.show(); cout << "\n Second time "; t2.show(); cout << "\n Sum of times "; t3.show(); getch(); } While normal addition of two numbers return the sumation result. In the case above we have overloaded the + operator, to perform addition of two Time class objects. We add the seconds, minutes and hour values separately to return the new value of time. In the setTime() funtion we are separately asking the user to enter the values for hour, minute and second, and then we are setting those values to the Time class object. For inputs, t1 as 01:20:30(1 hour, 20 minute, 30 seconds) and t2 as 02:15:25(2 hour, 15 minute, 25 seconds), the output for the above program will be: 1:20:30 2:15:25 3:35:55 First two are values of t1 and t2 and the third is the result of their addition. Example: overloading << Operator to print Class Object We will now overload the << operator in the Time class, #include<iostream.h> #include<conio.h> class Time { int hr, min, sec; public: // default constructor Time() { hr=0, min=0; sec=0; } // overloaded constructor Time(int h, int m, int s) { hr=h, min=m; sec=s; } // overloading '<<' operator friend ostream& operator << (ostream &out, Time &tm); }; // define the overloaded function ostream& operator << (ostream &out, Time &tm) { out << "Time is: " << tm.hr << " hour : " << tm.min << " min : " << tm.sec << " sec"; return out; } void main() { Time tm(3,15,45); cout << tm; } Time is: 3 hour : 15 min : 45 sec This is simplied in languages like Core Java, where all you need to do in a class is override the toString() method of the String class, and you can define how to print the object of that class. Overloading Relational Operator in C++ You can also overload relational operators like == , != , >= , <= etc. to compare two object of any class. Let's take a quick example by overloading the == operator in the Time class to directly compare two objects of Time class. class Time { int hr, min, sec; public: // default constructor Time() { hr=0, min=0; sec=0; } // overloaded constructor Time(int h, int m, int s) { hr=h, min=m; sec=s; } //overloading '==' operator friend bool operator==(Time &t1, Time &t2); }; /* Defining the overloading operator function Here we are simply comparing the hour, minute and second values of two different Time objects to compare their values */ bool operator== (Time &t1, Time &t2) { return ( t1.hr == t2.hr && t1.min == t2.min && t1.sec == t2.sec ); } void main() { Time t1(3,15,45); Time t2(4,15,45); if(t1 == t2) { cout << "Both the time values are equal"; } else { cout << "Both the time values are not equal"; } } Both the time values are not equal Operator Precedence and Associativity: Precedence Rules: The precedence rules specify which operator is evaluated first when two operators with different precedence are adjacent in an expression. For example: x=a+++b This expression can be seen as postfix increment on a and addition with b or prefix increment on b and addtion to a. Such issues are resolved by using precedence rules. Associativity Rules: The associativity rules specify which operator is evaluated first when two operators with the same precedence are adjacent in an expression. For example: a∗b/c Operator Precedence: The following table describes the precedence order of the operators mentioned above. Here, the operators with the highest precedence appear at the top and those with the lowest at the bottom. In any given expression, the operators with higher precedence will be evaluated first. LR= Left to Right RL=Right to Left Category Associativity Operator Postfix LR ++ -- Unary RL + - ! ~ ++ -- Multiplicative LR * / % Additive LR + - Shift LR << >> Relational LR < <= > >= Equality LR == != Bitwise AND LR & Bitwise XOR LR ^ Bitwise OR LR | Logical AND LR && Logical OR LR || Conditional RL ?: Assignment RL = += -= *= /= %= >>= <<= &= ^= |= C++ Functions C++ functions are a group of statements in a single logical unit to perform some specific task. Along with the main function, a program can have multiple functions that are designed for different operation. The results of functions can be used throughout the program without concern about the process and the mechanism of the function. In POP (Procedural Oriented Programming) language like C, programs are divided into different functions but in OOP (Object Oriented Programming) approach program is divided into objects where functions are the components of the object. Generally, C++ function has three parts: • Function Prototype • Function Definition • Function Call C++ Function Prototype While writing a program, we can’t use a function without specifying its type or without telling the compiler about it. So before calling a function, we must either declare or define a function. Thus, declaring a function before calling a function is called function declaration or prototype which tells the compiler that at some point of the program we will use the function of the name specified in the prototype. Syntax return_type function_name(parameter_list); Note: function prototype must end with a semicolon. Here, return_type is the type of value that the function will return. It can be int, float or any user-defined data type. function_name means any name that we give to the function. However, it must not resemble any standard keyword of C++. Finally, parameter_list contains a total number of arguments that need to be passed to the function. C++ Function Call Function call means calling the function with a statement. When the program encounters the function call statement the specific function is invoked. Syntax function_name( argument_list ); Here, function_name is the name of the called function and argument_list is the comma-separated list of expressions that constitute the arguments. The syntax is similar to that of prototype except that return_type is not used. C++ functions definition Function definition is a part where we define the operation of a function. It consists of the declarator followed by the function body. Syntax return_type function_name( parameter_list ) { function body; } Call by Value and Call by Reference in C++ On the basis of arguments there are two types of function are available in C++ language, they are; • With argument • Without argument If a function takes any arguments, it must declare variables that accept the values as a arguments. These variables are called the formal parameters of the function. There are two ways to pass value or data to function in C++ language which is given below; • call by value • call by reference Call by value In call by value, original value can not be changed or modified. In call by value, when you passed value to the function it is locally stored by the function parameter in stack memory location. If you change the value of function parameter, it is changed for the current function only but it not change the value of variable inside the caller function such as main(). Call by value #include<iostream.h> #include<conio.h> void swap(int a, int b) { int temp; temp=a; a=b; b=temp; } void main() { int a=100, b=200; clrscr(); swap(a, b); // passing value to function cout<<"Value of a"<<a; cout<<"Value of b"<<b; getch(); } Output Value of a: 200 Value of b: 100 Call by reference In call by reference, original value is changed or modified because we pass reference (address).
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages25 Page
-
File Size-