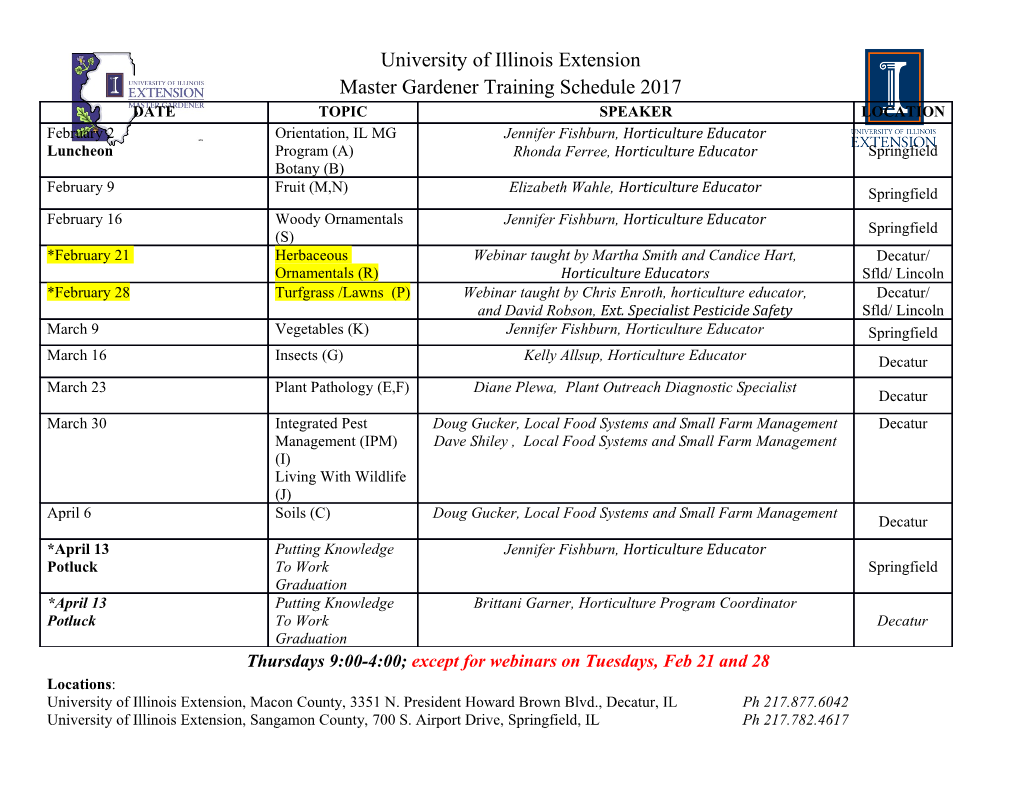
11/3/16 Breadth-First Search using a FIFO Queue Example BFS Traversal BFS-Init (G): BFS (G, s): 1. for all nodes v in V 1. s.d = 0 G H 2. if v is white 2. s.c = gray 3. BFS(G, v) 3. for each node v != s 4. v.d = ∞ A E 5. v.c = white An initialization 6. Q.enqueue (s) C F algorithm like that 7. while Q ≠ ∅ 8. u = Q.dequeue() D B shown above would 9. for each v adjacent to u ensure that all nodes 10. if v.c == white are visited, even in 11. v.c = gray J I disjoint sets of nodes. 12. v.d = u.d + 1 13. v.π = u 14. Q.enqueue(v) Order of visiting: a1 c2 d3 e4 f5 b6 g7 h8 i9 j10 15. u.c = black Distance of vertex : 0 1 1 1 2 3 ∞ ∞ ∞ ∞ Breadth-first Search Forest Bipartite Graphs G A A graph is bipartite if all its vertices can be partitioned into two disjoint subsets X and Y so H that every edge connects a vertex in X with a E C D vertex in Y, i.e., if its vertices can be colored in I B 2 colors so that every edge has its end points colored in different colors. J F A C E Tree edges are solid lines and dashed lines are cross D F B edges. Bipartite Graphs Bipartite Graphs Explain how BFS could be used to detect Is this graph bipartite? No. The edge a bipartite graph. (B,C) would have to connect nodes of the same color A C E Mark the source, A, with color1, mark the nodes at level 1 with A B D F B color2, and so on. Every node on an C D A F E even numbered level will be color1 and every odd color2 D C B 1 11/3/16 Depth-First Traversal Depth-First Search DFS algorithm maintains the following information for each Depth-First Traversal is another algorithm for traversing a vertex u: graph. - u.c (white, gray, or black) : indicates status Called depth-first because it searches "deeper" in the graph white = not discovered yet whenever possible. gray = discovered, but not finished black = finished Edges are explored out of the most recently discovered vertex v that still has unexplored edges. When all of v's edges have - u.d : discovery time of node u been explored, the search "backtracks" to explore the edges incident on the vertex from which v was discovered. - u.f : finishing time of node u We will use an algorithm with a stack, S, to manage the set of - u.π : predecessor of u in Depth-First tree nodes. DFS node Depth-First Search Each node has fields for predecessor (π), discovery time (d), finish time (f) and color (c). Each node also has an associated DFS-Init (G, s): Initialize global timer to 0. adjacency list with pointers to neighboring nodes. 1. time = 0 2. for all nodes v Set discovery time and finish 3. v.d = v.f = ∞ 4. v.c = white times of all nodes to infinity 5. v.π = NONE and color them white. 6. S = ∅ 7. DFS (G, s) d π Initialize stack S to ∅. Adjacency NAME List Call DFS (G, s) f c Depth-First Search Using a Stack Depth-First Search (recursive version) DFS (G,s) Initially, time (counter) = 0 1. S.push(s) DFS-Visit (G,u) 2. while S is not empty Complexity is based on 1. u.c = gray 3. u = S.peek() After execution, for every number of edges |E| 2. u.d = time 4. if u.c == WHITE vertex u, u.d < u.f 5. u.c = GRAY 3. time = time + 1 6. u.d = time 4. for each v adjacent to u DFS (G) 7. time = time + 1 5. if v.c == white 8. for all white neighbors v of u 1. for each w ∈ G 6. v.π = u 9 v.π = u 2. if w.c == white 10. S.push(v) 7. DFS-Visit(G,v) Complexity (Adjacency List) 3. DFS-Visit (G,w) 11. else if u.c == GRAY 8. end if 12. S.pop() • check all edges 13. u.c = BLACK adjacent to each node Note: If G = (V, E) is not 9. end for 14. u.f = time from both directions - connected, then DFS will still 10. u.c = black 15. time = time + 1 O(E) time visit the entire graph with the 11. u.f = time 16. else // u is BLACK • total = O(V + E) = 17. S.pop() additional code above. 12. time = time + 1 2 18. end while O(V ) (w.c.) 2 11/3/16 Example DFS Traversal Depth-first Search Forest The first subscript G H G indicates A A E the time at which each H C F node is C discovered F D B I and pushed onto stack; the B J I J second D e7,8 indicates the time at which E b6,9 j 16,17 the node was d3,4 f i 5,10 15,18 finished. Tree edges are solid lines and dashed lines are back c2,11 h14,19 edges. a 1,12 g13,20 Breadth-first Search Forest Facts about DFS and BFS on undirected graph G A DFS BFS H E Data Structure Stack Queue C D No. of vertex 2 orderings 1 ordering I orderings B Edge Types tree and back tree and cross edges edges J Applications connectivity, connectivity, F acyclicity, acyclicity, articulation minimum-edge points paths Tree edges are solid lines and dashed lines are cross Efficiency for θ(|V| + |E|) θ(|V| + |E|) edges. adjacency lists Depth-First Search Depth-First Search Theorem 22.7 (parenthesis theorem) For any two vertices u and v, exactly one of the following three conditions hold: Theorem 22.7 (parenthesis theorem) For any two vertices u and v, 1. Either the intervals [u.d, u.f] and [v.d, v.f] are entirely disjoint, or exactly one of the following three conditions hold: 2. the interval [u.d, u.f] is contained entirely within the interval [v.d, 1. Either the intervals [u.d, u.f] and [v.d, v.f] are entirely disjoint, or v.f], (and u is a descendant of v), or 2. the interval [u.d, u.f] is contained entirely within the interval [v.d, 3. the interval [v.d, v.f] is contained entirely within the interval [u.d, v.f], (and u is a descendant of v), or u.f], (and v is a descendant of u). 3. the interval [v.d, v.f] is contained entirely within the interval [u.d, u.f], (and v is a descendant of u). Proof: Case 1 – u.d < v.d. If v.d < u.f, then v was discovered when u was still gray, implying v is a descendant of u. Since v was discovered Proof: Case 2 – v.d < u.d. Similar argument to case 1 on last slide before u is finished, all of v's outgoing edges will have been explored (condition 2 if u.f < v.f or condition 1 if v.f < u.f). n before u is finished. Therefore, the interval [v.d,v.f ] is contained within the interval [u.d,u.f] (condition 3 holds, v is descendant of u). Corollary 22.8 (nesting of descendant’s intervals): Vertex v is a If u.d < v.d and u.f < v.d, then u was discovered and finished before v descendant of vertex u in the DFS forest for a graph G iff was discovered (condition 1 holds, intervals are disjoint). u.d < v.d < v.f < u.f. 3 11/3/16 Depth-First Search Theorem 22.9 (White-Path theorem): In a depth-first forest of a graph G, vertex v is a descendant of vertex u Theorem 22.9 (White-Path theorem): iff at the time u.d that the search discovers u, vertex v can be reached In a depth-first forest of a graph G, vertex v is a descendant from u along a path consisting entirely of white vertices. of vertex u iff at the time u.d that the search discovers u, Proof: vertex v can be reached from u along a path consisting Backward Direction of iff: (by contradiction) Assume vertex v is entirely of white vertices. reachable from u along a path consisting of white vertices at time u.d, but v does not become a descendant of u in the DFS. Assume, wlog, that every other vertex between u and v becomes a descendant of u. Proof: Let w be the immediate predecessor of v on a path from u. Then by corollary 22.8, w.f ≤ u.f (or w.f=u.f if w=u). Forward direction of iff: Assume v is a descendant of u in a depth-first tree. Let w be any vertex on the path between u Since v is reachable from u via a path of white vertices by assumption, v and v in the depth-first tree, so that w is also a descendant must be discovered after u, but before w is finished. So u.d < v.d < w.f ≤ of u. By Corollary 22.8, u.d < w.d, and so w is white at time u.f. So by the parenthesis theorem (22.7), [v.d, v.f] must be contained within [u.d,u.f] and v must be a descendant of u, a contradiction.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages5 Page
-
File Size-