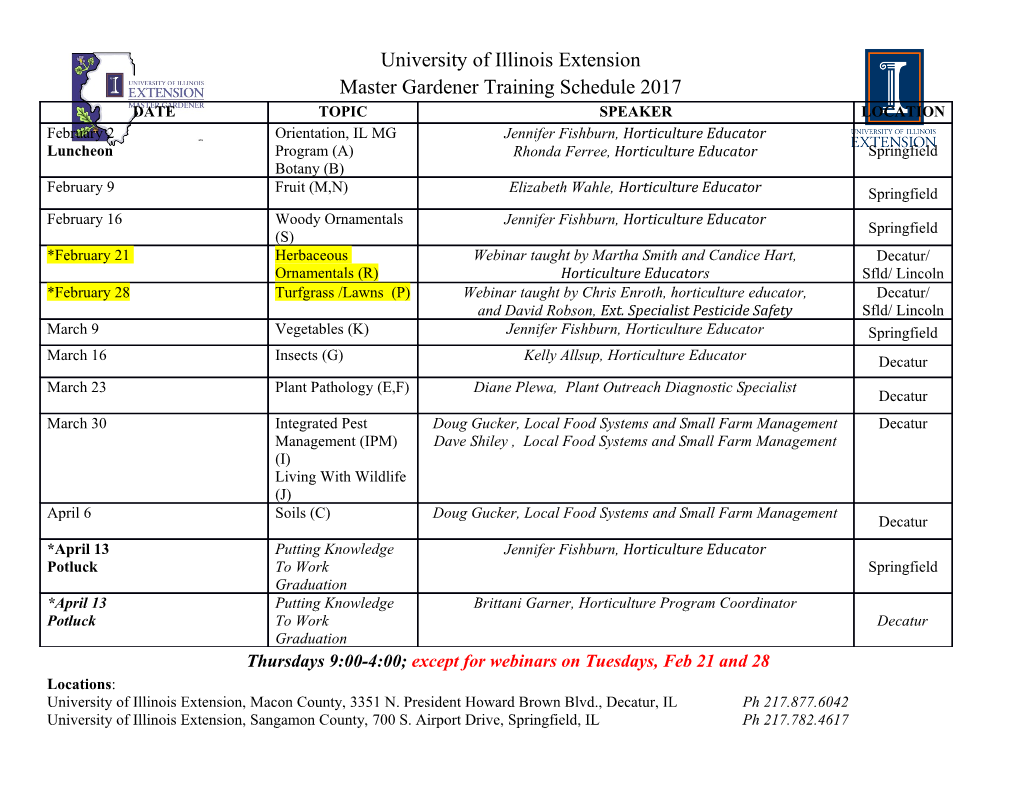
229 SQL • After completing this chapter, you should be able to . write advanced SQL queries including, e.g., multiple tuple variables over different/the same relation, . use aggregation, grouping, UNION, . be comfortable with the various join variants, . evaluate the correctness and equivalence of SQL queries, This includes the sometimes tricky issues of (deciding the presence) duplicate result tuples. judge the portability of certain SQL constructs. 230 SQL Overview 1. SELECT-FROM-WHERE Blocks, Tuple Variables 2. Subqueries, Non-Monotonic Constructs 3. Aggregations I: Aggregation Functions 4. Aggregations II: GROUP BY, HAVING 5. UNION, Conditional Expressions 6. ORDER BY 7. SQL-92 Joins, Outer Join 231 Basic SQL Query Syntax Basic SQL query (extensions follow) SELECT A1, ... ,An FROM R1, ... ,Rm WHERE C • The FROM clause declares which table(s) are accessed in the query. • The WHERE clause specifies a condition for rows (or row combinations) in these tables that are considered in this query (absence of C is equivalent to C TRUE). ≡ • The SELECT clause specifies the attributes of the result tuples (* output all attributes occurring in R ,...,R ). ≡ 1 m 232 Example Database (again) STUDENTS RESULTS SID FIRST LAST EMAIL SID CAT ENO POINTS 101 Ann Smith ... 101 H 1 10 102 Michael Jones (null) 101 H 2 8 103 Richard Turner ... 101 M 1 12 104 Maria Brown ... 102 H 1 9 102 H 2 9 EXERCISES 102 M 1 10 CAT ENO TOPIC MAXPT 103 H 1 5 103 M 1 7 H 1 Rel.Alg. 10 H 2 SQL 10 M 1 SQL 14 233 Tuple Variables (1) • The FROM clause can be understood as declaring variables that range over tuples of a relation: SELECT E.ENO, E.TOPIC FROM EXERCISES E WHERE E.CAT = ’H’ . This may be executed as foreach E EXERCISES do ∈ if E.CAT = ’H’ then print E.ENO, E.TOPIC fi od . Tuple variable E represents a single row of table EXERCISES (the loop assigns each row in succession). 234 Tuple Variables (2) • For each table in the FROM clause, a tuple variable is automatically created: if not given explicitly, the variable will have the name of the relation: SELECT EXERCISES.ENO, EXERCISES.TOPIC FROM EXERCISES WHERE EXERCISES.CAT = ’H’ . In other words, stating FROM EXERCISES only is understood as FROM EXERCISES EXERCISES (the tuple variable EXERCISES ranges over the rows of the table EXERCISES). 235 Tuple Variables (3) • If a tuple variable is explicitly declared, e.g., FROM EXERCISES E then the implicit tuple variable EXERCISES is not declared, i.e., EXERCISES.ENO will yield an error. • A reference to an attribute A of a tuple variable R may be simply written as A (instead of R.A) if R is the only variable which is bound to tuples having an attribute named A. 236 Attribute References (1) • In general, attributes are referenced in the form R.A • If an attribute may be associated to a tuple variable in an unambiguous manner, the variable may be omitted, e.g.: SELECT CAT, ENO, POINTS FROM STUDENTS S, RESULTS R WHERE S.SID = R.SID AND FIRST = ’Ann’ AND LAST = ’Smith’ FIRST, LAST can only refer to S. CAT, ENO, POINTS can only refer to R. SID on its own is ambiguous (may refer to S or R). 237 Attribute References (2) • Consider this query: SELECT ENO, SID, POINTS, MAXPT FROM RESULTS R, EXERCISES E WHERE R.ENO = E.ENO AND R.CAT = ’H’ AND E.CAT = ’H’ . Although forced to be equal by the join condition, SQL requires the user to specify unambiguously which of the ENO attributes (bound to R or E) is meant in the SELECT clause. The unambiguity rule thus is purely syntactic and does not depend on the query semantics. 238 Joins (1) • Consider a query with two tuple variables: SELECT A1, ... ,An FROM STUDENTS S, RESULTS R WHERE C . S will range over 4 tuples in STUDENTS, R will range over 8 tuples in RESULTS. In principle, all 4 8 = 32 combinations × will be considered in condition C: foreach S STUDENTS do foreach∈ R RESULTS do if C then∈ print A1,...,An fi od od 239 Joins (2) • A good DBMS will use a better evaluation algorithm (depending on the condition C). This is the task of the query optimizer. For example, if C contains the join condition S.SID = R.SID, the DBMS might loop over the tuples in RESULTS and find the corresponding STUDENTS tuple by using an index over STUDENT.SID (many DBMS automatically create an index over the key attributes). • In order to understand the semantics of a query, however, the simple nested foreach algorithm suffices. The query optimizer may use any algorithm that produces the exact same output, although possibly in different tuple order. 240 Joins (3) • A join needs to be explicitly specified in the WHERE clause: SELECT R.CAT, R.ENO, R.POINTS FROM STUDENTS S, RESULTS R WHERE S.SID = R.SID -- Join Condition AND S.FIRST = ’Ann’ AND S.LAST = ’Smith’ Output of this query? SELECT S.FIRST, S.LAST FROM STUDENTS S, RESULTS R WHERE R.CAT = ’H’ AND R.ENO = 1 Notes 241 Joins (4) • Guideline: it is almost always an error if there are two tuples variables which are not linked (directly or indirectly) via join conditions. In this query, all three tuple variables are connected: SELECT E.CAT, E.ENO, R.POINTS, E.MAXPT FROM STUDENTS S, RESULTS R, EXERCISES E WHERE S.SID = R.SID AND R.CAT = E.CAT AND R.ENO = E.ENO AND S.FIRST = ’Ann’ AND S.LAST = ’Smith’ 242 Joins (5) • The tuple variable connection works as follows: S R E '&%$ !"# S.SID = R.SID '&%$ !"# R.CAT = E.CAT '&%$ !"# AND R.ENO = E.ENO • The conditions correspond to the foreign key relationships between the tables. • The omission of a join condition will usually lead to numerous duplicates in the query result. Usage of DISTINCT to get rid of duplicate does not fix the error in such a case, of course. 243 Query Formulation (1) Formulate the following query in SQL “Which are the topics of all exercises solved by Ann Smith?” • To formulate this query, . consider that Ann Smith is a student, requiring a tuple variable, S say, over STUDENTS and the identifying condition S.FIRST = ’Ann’ AND S.LAST = ’Smith’. Exercise topics are of interest, so a tuple variable E over EXERCISES is needed, and the following piece of SQL can already be generated: SELECT DISTINCT E.TOPIC Several exercises may have the same topic. 244 Query Formulation (2) • Note: S and E are still unconnected. • The connection graph of the tables in a database schema (edges correspond to foreign key relationships) helps in understanding the connection requirements: STUDENTS RESULTS EXERCISES . We see that the S–E connection is indirect and needs to be established via a tuple variable R over RESULTS: S.SID = R.SID AND R.CAT = E.CAT AND R.ENO = E.ENO 245 Query Formulation (3) • It is not always that trivial. The connection graph may contain cycles, which makes the selection of the “right path” more difficult (and error-prone). • Consider a course registration database that also contains TA (“Hiwi”) assignments: TA eee YYYY eeeeeee YYYYYY STUDENTS e Y COURSES YYYY eee YYYYY eeeeee ENROLLMENTS 246 Unnecessary Joins (1) • Do not join more tables than needed. Query will run slowly if the optimizer overlooks the redundancy. Results for homework 1 SELECT R.SID, R.POINTS FROM RESULTS R, EXERCISES E WHERE R.CAT = E.CAT AND R.ENO = E.ENO AND E.CAT = ’H’ AND E.ENO = 1 Will the following query produce the same results? SELECT SID, POINTS FROM RESULTS R WHERE R.CAT = ’H’ AND R.ENO = 1 247 Unnecessary Joins (2) What will be the result of this query? SELECT R.SID, R.POINTS FROM RESULTS R, EXERCISES E WHERE R.CAT = ’H’ AND R.ENO = 1 Is there any difference between these two queries? SELECT S.FIRST, S.LAST FROM STUDENTS S SELECT DISTINCT S.FIRST, S.LAST FROM STUDENTS S, RESULTS R WHERE S.SID = R.SID Notes 248 Self Joins (1) • In some query scenarios, we might have to consider more than tuple of the same relation in order to generate a result tuple. Is there a student who got 9 points for both, homework 1 and homework 2? SELECT S.FIRST, S.LAST FROM STUDENTS S, RESULTS H1, RESULTS H2 WHERE S.SID = H1.SID AND S.SID = H2.SID AND H1.CAT = ’H’ AND H1.ENO = 1 AND H2.CAT = ’H’ AND H2.ENO = 2 AND H1.POINTS = 9 AND H2.POINTS = 9 249 Self Joins (2) Find students who solved at least two exercises.a aThis may also be solved using aggregations (later). SELECT S.FIRST, S.LAST FROM STUDENTS S, RESULTS E1, RESULTS E2 WHERE S.SID = E1.SID AND S.SID = E2.SID “Unexpected” result What is going wrong here? We need to make explicit that E1 and E2 refer to distinct exercises: . AND (E1.CAT <> E2.CAT OR E1.ENO <> E2.ENO) 250 Duplicate Elimination (1) • A core difference between SQL and relational algebra is that duplicates have to explicitly eliminated in SQL. Which exercises have already been solved by at least one student? CAT ENO H 1 H 2 SELECT CAT, ENO M 1 FROM RESULTS H 1 H 2 M 1 H 1 M 1 251 Duplicate Elimination (2) • If a query might yield unwanted duplicate tuples, the DISTINCT modifier may be applied to the SELECT clause to request explicit duplicate row elimination: CAT ENO SELECT DISTINCT CAT, ENO H 1 FROM RESULTS H 2 M 1 • To emphasize that there will be duplicate rows (and that these are wanted in the result), SQL provides the ALL modifier.4 4SELECT ALL is the default.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages63 Page
-
File Size-