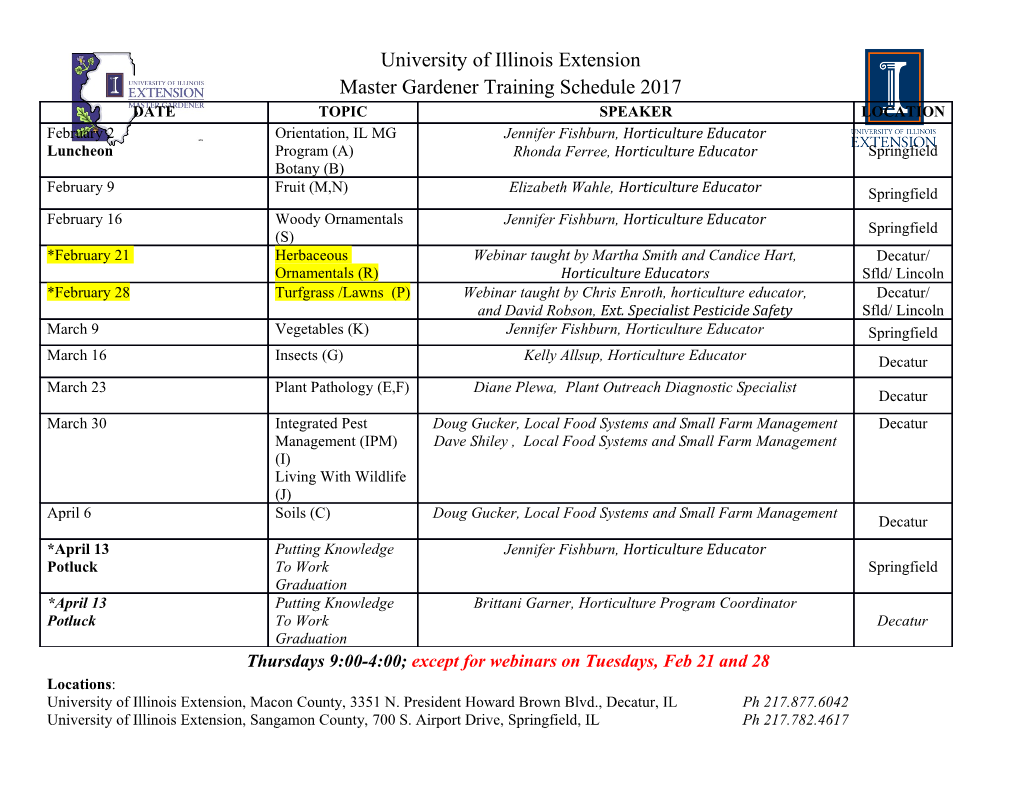
2D Graphics 2D Raster Graphics Integer grid Sequential (left-right, top-down) scan j i Computer Graphics Line drawing A very important operation used frequently, block diagrams, bar charts, engineering drawing, architecture plans, etc. curves as concatenation of small line segments Criteria line should appear straight illuminate nearest grid point Computer Graphics Line drawing Line should terminate correctly Line should have a constant intensity specify both end points instead of end point + slope +length 5 / 4 2 brightness adjustment (antialiasing) 5/4 intensity ~ # of dots/unit length Computer Graphics Line drawing Line should not have “gaps” y=f(x) if |slope|<1 x=f(y) if |slope|>1 y=f(x) Computer Graphics Line drawing Line should be drawn as fast as possible Brute-force method DDA (digital differential analyzer) y mx b 1 fp * 1 fp + for(i xo ;i xn ;i ) yi m i b yi1 mxi1 b m(xi 1) b 1 fp + mxi b m yi m Computer Graphics Bresenham’s Line Algorithm integer operations only 0 slope 1 (0,0) (x2 x1, y2 y1) ti si (x , y ) (0,0) i1 i1 if s t or s t 0 ti else s t or s t 0 si Computer Graphics o 45 possible next intersection possible range current intersection range Computer Graphics Bresenham’s Line Algorithm dy (x 1) dx i1 dy ti si (xi1 1) yi1 (0,0) dx s dy i ti ( yi1(x1, y) ) (xi1 1) i1 i1 dx dy floating point s t 2 (x 1) 2 y 1 i i dx i1 i1 dx(si ti ) 2(xi1dy yi1dx) 2dy dx Integer!! di Computer Graphics Bresenham’s Line Algorithm di 2(xi1dy yi1dx) 2dy dx di1 2(xidy yidx) 2dy dx di1 di 2dy(xi xi1) 2dx( yi yi1) di1 di 2dy 2dx( yi yi1) di1 di 2dy 2dx( yi yi1) Computer Graphics Bresenham’s Line Algorithm di1 di 2dy 2dx( yi yi1) if di 0 choose ti yi yi1 1 di1 di 2(dy dx) if di 0 choose si yi yi1 di1 di 2dy initial condition d1 2dy dx (x0, y0 ) (0,0) •Complexity: 1 left shift + 2 integer additions Computer Graphics Circle Drawing Symmetry reduces drawing to 1/8 (-y,x) (y,x) x xc r cos y yc r sin (-x,y) (x,y) (-x,-y) (x,-y) (-y,-x) (y,-x) Computer Graphics Bresenham’s Circle Algorithm integer operations only (xc , yc ) (0,0) 45o 90o ti si (0,0) (xi1, yi1) 2 2 2 D(si ) (xi1 1) ( yi1 1) r | D(si )|| D(ti )| ti 2 2 2 D(ti ) (xi1 1) yi1 r | D(si )|| D(ti )| si Computer Graphics Bresenham’s Circle Algorithm di | D(si )|| D(ti )| D(si ) D(ti ) 2 2 2 2 di 2r 2(xi1 1) ( yi1 1) yi1 2 2 2 2 di1 2r 2(xi1 2) ( yi 1) yi 2 2 di1 di 4xi1 6 2( yi yi1 ) 2( yi yi1) Computer Graphics Bresenham’s Circle Algorithm d1 3 2r (x0, y0 ) (0,r) if di 0 choose ti yi yi1, di1 di 4xi1 6 if di 0 choose si yi yi1 1, di1 di 4xi 4 yi 6 •Complexity: only integer and shift operations Computer Graphics Other primitives Ellipse symmetry reduces to 1/4 Bresenhem’s ellipse algorithm Curve difficult approximation using short line segments general curve forms (Bezier, B-spline, etc.) Characters rectangular grid patterns Computer Graphics Polygon Filing Arbitrary # of sides Convex or concave Holes Y X Computer Graphics Scan Line Algorithm Edge table sort edges by scanline (using min Y) ymax record x coordinate of ymin ymax yi1 x to be added yi (x, ymin ) Computer Graphics Scan Line Algorithm Set y to smallest y in ET Initialize AET to be Null Repeat until AET and ET are empty move from ET bucket y to AET those edges whose ymin=y sort edges in AET by x (insertion sort) fill in pixel values in between x pairs remove from AET those edges whose ymax = y increment y by 1 update x for all edges in AET x x x Computer Graphics Polygon Patterned Filling Y Y logical and X X ComputerX Graphics Polygon Patterned Filling Pattern can be anchored at a fixed point: transparent object moves on a patterned background a polygon corner: patterned object Computer Graphics 2D Transformation For animation, manipulation, user interaction translation, rotation, scaling x' x Tx y' y Ty x' cos sinx y' sin cos y x' Sx 0 x y' 0 S y y Computer Graphics A Very Common Confusion What is being transformed? Points or coordinate system? For CG, pipeline operations are always applied to features (points, lines, curves, planes) But you can think in either way: Points are physically moved in a fixed coordinate system (e.g., in modeling transform), or A coordinate system is moved, while points stay stationary (e.g., in viewing transform) Both interpretations are useful Computer Graphics 2D Rigid Transformations A rigid transformation maps one coordinate system into another Preserves distances and angles To transform points from one coordinate frame to another, find the rigid transformation that brings the two coordinate frames in alignment Translate so that their origins coincide Rotate so that their axes coincide (x with x, y with y, and z with z) Computer Graphics 2D examples y y’ y’ y x’ x x’ x Rot Trans y’ P y x’ x Trans+Rot Computer Graphics 2D Translation Translate the coordinate system by (Tx,Ty) What is the translated point? y T P P x P Ty x (Tx,Ty) Computer Graphics 2D rotation matrix Rotate θ counterclockwise P RP What is the transformation R? y’ y P cos sin P P x’ sin cos x Computer Graphics 2D Rigid Transformations A rigid transformation moves an object from one location to another location Preserves distances and angles To transform points from one place to another, find the rigid transformation that Translate so that the object moves Rotate so that the object reorients Computer Graphics 2D examples P y P P’ y P’ x x Trans y Rot P x Trans+Rot P’ Computer Graphics 2D Translation Translate the coordinate system by (Tx,Ty) What is the translated point? y (Tx,Ty) T P P x P Ty x (-Tx,-Ty) Computer Graphics 2D rotation matrix Rotate θ counterclockwise P RP What is the transformation R? y P’ cos sin P P P sin cos x - Computer Graphics 2D Transformation For animation, manipulation, user interaction translation, rotation, scaling x' x Tx y' y Ty x' cos sinx y' sin cos y x' Sx 0 x y' 0 S y y Computer Graphics 2D Transformation (cont.) Inconsistent representation for translation Cannot be concatenated Troublesome for Hierarchical transforms Interactive, incremental display P Rn (R3 (R2 (R1P T1) T2 ) T3 ) Tn Computer Graphics Homogeneous Coordinates consistent representation for all three can be concatenated & pre-computed (x, y) (wx, wy, w), w 0 (wx, wy, w) (wx / w, wy / w) Computer Graphics x' 1 0 Tx x y' 0 1 T y y 1 0 0 1 1 x' cos sin 0x y' sin cos 0 y 1 0 0 11 x' Sx 0 0x y' 0 S 0 y y 1 0 0 11 x' x y' (TRS ) y 1 1 Computer Graphics How about other transforms? For example, reflection Mirrored images of each other Computer Graphics Try to represent the new transform as a composite of T, R, S (a,b) cos sin 0 1 0 0 cos sin 0 sin cos 0 0 1 0 sin cos 0 0 0 1 0 0 1 0 0 1 b b tan 1( ) tan 1( ) a a Computer Graphics Clipping Against Upright Rectangular Window Points if xmin x xmax & ymin y ymax then accept otherwise reject Computer Graphics Clipping Against Upright Rectangular Window Lines – trivially accepted if both end points inside – otherwise Points x1 t(x2 x1) x'1 t'(x'2 x'1 ) y1 t( y2 y1) y'1 t'( y'2 y'1 ) 0 t,t' 1 (x1, y1),(x2 , y2 ) : end points of line (x'1 , y'1 ),(x'2 , y'2 ) : end points of window boundary Computer Graphics Cohen-Sutherland Line-Clipping Algorithm xmin xmax 1001 1000 1010 ymax Outcodes bit1 --above: ymax-y 0001 0000 0010 bit2 --below: y-ymin ymin bit3 --right of: xmax-x bit4 --left of: x-xmin 0101 0100 0110 Computer Graphics Trivially-accept: both end points having outcode 0000 Trivially-reject: corresponding bits in two outcodes are set, or outcode1 & outcode2 nonzero 1001 1000 1010 0001 0000 0010 0101 0100 0110 Computer Graphics Neither: need more testing E.g. mid-point algorithm divide a line segment (x1, y1),(x2, y2 ) into two line segments (x1, y1),((x1 x2 ) / 2,( y1 y2 ) / 2) ((x1 x2 ) / 2,( y1 y2 ) / 2),(x2, y2 ) test each line independently recursive division if necessary guarantee to stop in O(logn) steps Computer Graphics Cyrus-Beck (Liang-Basky) Line Clipping Can be more efficient when intersection tests are unavoidable Work in the parameter (t) space to locate true intersections before calculating 2D coordinates Work for all kinds of clipping polygons and in 3D Two basic steps: find intersections (t) classify intersections Computer Graphics Cyrus-Beck (Liang-Basky) Line Clipping Find intersections ax by c 0 N (P(t) PE ) 0 PE N (Po t(P1 Po ) PE ) 0 t N (P P ) N (P P ) 0 P 1 o o E 1 N (P P ) N (P(t) PE ) 0 t o E N (P1 Po ) N (P(t) PE ) 0 Po P (t) N (P(t) PE ) 0 N (a,b) Computer Graphics Intersections outside (0,1) range are not valid But intersection inside (0,1) range might not be valid either Computer Graphics Cyrus-Beck (Liang-Basky) Line Clipping Classify intersections N (P1 Po ) 0 PE (potentially entering) N (P1 Po ) 0 PL (potentially leaving) P1 N P0 Computer Graphics Locate the largest PE point & t>0 Locate the smallest PL point & t<1 PE < PL for a valid line PE PL P1 P0 Computer Graphics Polygon Clipping (Sutherland-Hodgman) Given an ordered sequence of polygon vertices And a convex clipping polygon Output ordered clipped polygon vertices Using divide-and-conquer, one clipping edge at a time Computer Graphics IN OUT IN OUT IN OUT IN OUT Computer Graphics 1 1 6 4 2 5 2 4 3 3 Original Right boundary clipping Computer Graphics 7 7 6 6 1 8 5 5 1 4 4 2 3 2 3 Bottom boundary clipping Left boundary clipping Computer Graphics 7 6 5 8 4 9 3 1 2 Top boundary clipping Computer Graphics Other Primitives Use of extents (extents for a whole string, words, individual characters) Divide and Conquer Computer Graphics.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages53 Page
-
File Size-