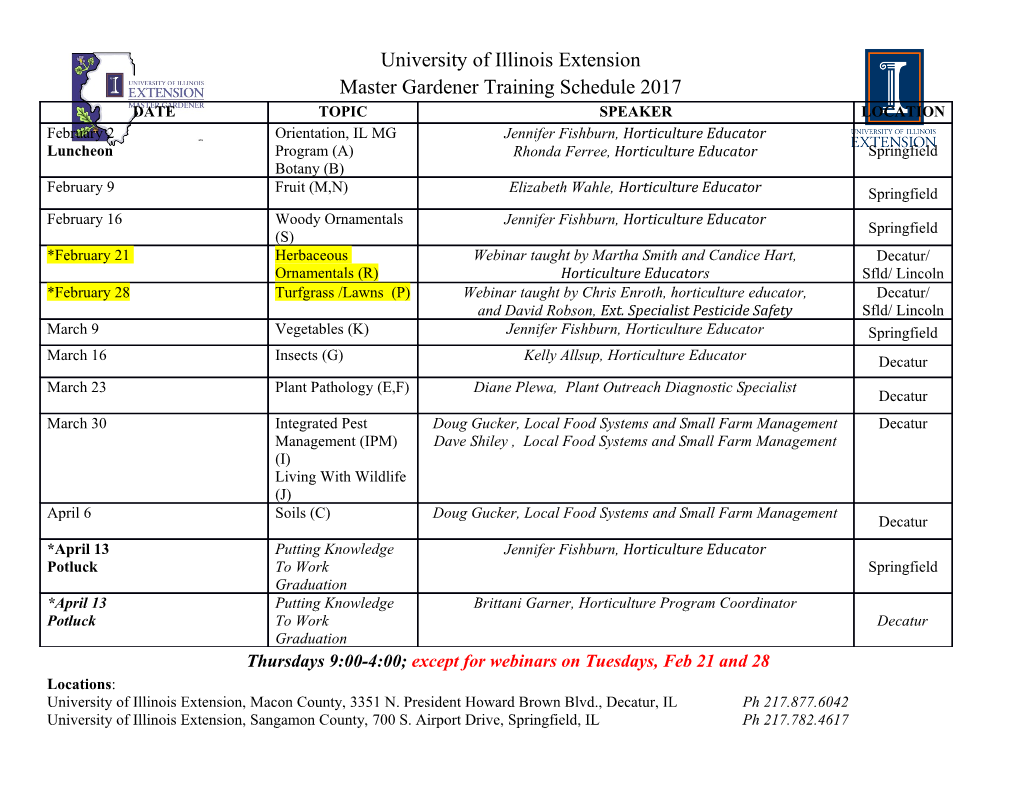
Advanced 3D Computer Graphics Prof. Dr. Renato Pajarola Information & Computer Science University of California Irvine ICS 186b, October 20. 2000 1 Polygonal Meshes Polygonal meshes are (still) the most common representations of 3D surfaces for interactive render- ing. Even complicated smooth surfaces are often approximated as a collection of planar polygons. Also more complicated modeling primitives such as spheres and curved surfaces, or solid modeling constructions used in advanced modeling applications, or rendering systems such as ray-tracing often have to be tessellated for interactive rendering or further processing. Furthermore, today’s graphics hardware is optimized for fast processing of triangle meshes. Handling triangle meshes is sufficient in the sense that planar polygons can easily be triangulated to form a collection of triangles covering exactly the same planar region. If not specified otherwise, we refer to triangle meshes in this section. 1.1 Mesh Representation 1.1.1 Mesh properties To talk about triangle meshes it is important to define the following few terms that are very often used, and that characterize a triangle mesh. Incidence is defined between vertices, edges, and faces. All faces sharing an edge are incident to that edge, all faces sharing a vertex are incident to that ver- tex, all edges sharing a vertex are incident to that vertex, and vice versa. Definition 1.1 The boundary or boundaries of a mesh is defined by the set of edges that have only one incident face. Definition 1.2 A mesh is called manifold if the surrounding of every point on the surface is homeo- morphic, or topologically equivalent to a disk, or half-disk if the point is on the boundary. Definition 1.3 The genus of a closed manifold surface (with no boundaries) is defined as the number of topologically different closed paths (cycles, or loops) on the surface that cannot be contracted along the surface to a singular point. Sometimes this is also referred to as the number of handles of the shape. Definition 1.4 A mesh is called orientable if a consistent orientation of the vertices of the planar faces exists such that the two vertices of each edge are ordered oppositely for the incident faces. A polygonal mesh is also often called matching or conforming if every edge has exactly two incident faces, or one if the edge is on the boundary. A mesh is not matching if there exist so called T-vertices that lie exactly on an edge but are not an endpoint of that edge. Even if T-vertices are manifold with respect to the surface topology, they are regarded as a singularity since they form a boundary of col- linear edges. Non-matching triangulations can also create rendering artifacts when using vertex nor- mals for shading properties. Most of the time a triangulation is assumed to be matching. Mesh Representation Boundary edges Non-manifold vertex Non-manifold edge Non-orientable mesh Shape of genus 1 The Euler formula of planar graphs with V vertices, E edges, F faces, and C connected components is given by VE– +1F = + C . For planar triangulations – one component, orientable, manifold, matching – where each edge is shared by two faces, and each face has three edges 2E = 3F one can get the relation for vertices and faces as V –22F = , thus there are roughly twice as many faces as vertices in a typical triangular mesh (and three times as many edges as vertices). 1.1.2 Indexed face list The indexed face list is a very simple structure to represent a polygonal mesh M consisting of n ver- tices V = {v1, v2, …, vn} and m faces F = {f1, f2, …, fm}. Since each k-sided planar face can uniquely be defined by its k vertices (i.e. ordered counter clockwise), a list of k indices into the list of vertices can be used to represent one face. For triangle meshes an indexed face list consists of an array of ver- tices each having three coordinates, and an array of faces each having three indices into the vertex array. faces vertices xyz f1 v1 vk vi v f f vi vj vk i vk vj vj Since triangle meshes have about m = 2·n faces, each triangle has to index 3 vertices, and n vertices can be indexed by log2n bits, the indexed triangle list requires 6·n·log2n bits to represent the inci- 2 Mesh Representation dence relations, or connectivity of the triangle mesh. Additionally, 3·n·q bits are required to store the 3D vertex coordinates with fixed resolution of q bits, this is also called the geometry information. 1.1.3 Half-edge data structure While representing the mesh structure exactly, the indexed face list does not provide an efficient way of traversing the mesh, or accessing adjacent elements through incidence relations. Given a vertex, it is very time consuming to find all incident faces or edges, or given a triangle it is complicated to retrieve its incident triangle on a particular edge. Mesh traversal and neighbor finding are very important operations on polygonal meshes and efficiently supported by the half-edge data structure. The half-edge data structure represents a polygonal mesh as a list of vertices, and a list of directed half-edges, each k-sided planar face is represented by k consecutive entries in the half-edges array. Each entry h[i] in the half-edge array has to following fields: h[i].s - index of start vertex of the half-edge h[i].r - index of reverse half-edge of adjacent face incident upon this edge h[i].n - index of the next half-edge that follows in counter clockwise ordering h[i].p - index of the previous half-edge h.r h.n h h.s h.p For triangular meshes with all 3-sided faces, the next and previous pointers h.n and h.p can be omit- ted, since organizing the 3 half-edges of each face consecutively in the array allows for simple mod- ulo arithmetic to access next and previous edges (but we will keep the notation): h[i].n = (i + 1) MOD 3 + 3 (i DIV 3) h[i].p = (i + 2) MOD 3 + 3 (i DIV 3) Faces are also easily indexed, edge i belongs to face i DIV 3, and the first half-edge of face j is j · 3. Given such a half-edge data structure, most incidence relations can easily be accessed without searching all list elements. For simplicity we will abbreviate array indexing h[i].s by h.s in the fol- lowing examples. • vertices of and edge are given by h.s and h.n.s (note that using h.r.s will not work for edges on the boundary of the mesh!) • index of adjacent face upon h is given by h.r DIV 3 • rotating counter clockwise around the start vertex of h is performed by h = h.p.r • clockwise rotation about start vertex is performed by h = h.r.n Operations accessing the reverse half-edge index must always be performed very carefully for meshes with boundaries, and special cases must handle boundary conditions. Building a half-edge data structure from an indexed face input list can also be done very efficiently as follows: 3 Mesh Representation 1. Copy or keep the vertex array. 2. Read the indexed faces list and initialize the half-edge triples per face with the correct start- ing vertices. 3. Read the indexed faces list again, and create an auxiliary edge list with 3 entries per face, however, each such entry consists of both endpoints of the edge and an index to the half- edge created in the previous step. The vertex indices of the two endpoints are stored with the smaller vertex index first, and the larger vertex index in the second field. 4. Order the auxiliary edge list lexicographically according to the two vertex indices of each entry. Thus shared edges between two faces will now be adjacent in the auxiliary table, and the reverse half-edge information can be completed for every half-edge. Omitting the reverse information that can be recomputed when loading the data-structure, the half- edge structure needs exactly the same storage for representing a mesh as the indexed face list. If it includes the reverse information, a mesh with n vertices would require 6·n (log2n + log26n) bits on average. Doubly-connected edge list The doubly-connected edge list (DCL) often used in computational geometry and graph methods consists of three set of records: one for vertices, one for faces, and one for half-edges. Each vertex v stores its coordinates, and a pointer to an arbitrary half-edge that has v as its origin. Each face stores one pointer to a outside bounding half-edge (and a list of half-edge pointers to inner boundaries of holes). Each half-edge stores a pointer to its origin vertex, a pointer to its reverse (twin) edge, and a pointer to the face it bounds, as well as two pointers to the next and previous half-edges bounding the same face. The half-edge data structure is a simplified version of the DCL without vertex-to-edge and face-to- edge relations, and omitted face pointer per edge. Face to edge relations are rather captured by con- secutively ordering and grouping the half-edges that bound a particular face. Winged-edge list The winged-edge data structure, another commonly used graph or mesh representation, stores for each edge pointers to its endpoints, the two faces sharing the edge, and the four incident edges that bound the same faces.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages15 Page
-
File Size-