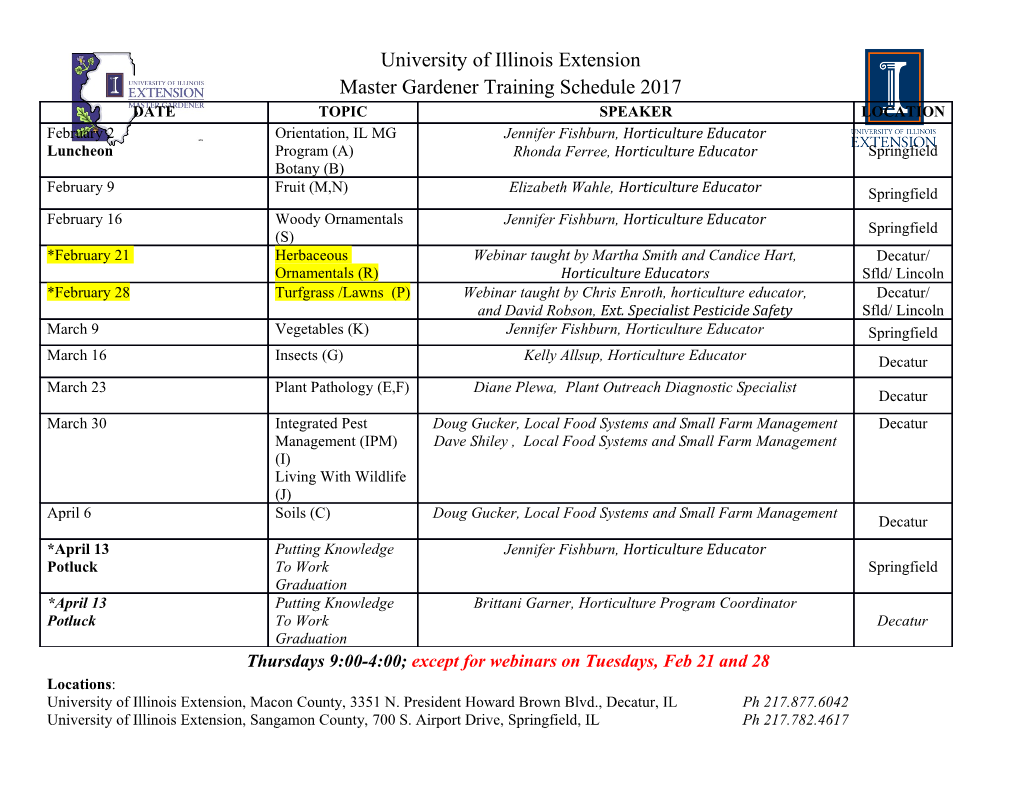
Reactors: A Deterministic Model of Concurrent Computation for Reactive Systems Marten Lohstroh Electrical Engineering and Computer Sciences University of California at Berkeley Technical Report No. UCB/EECS-2020-235 http://www2.eecs.berkeley.edu/Pubs/TechRpts/2020/EECS-2020-235.html December 21, 2020 Copyright © 2020, by the author(s). All rights reserved. Permission to make digital or hard copies of all or part of this work for personal or classroom use is granted without fee provided that copies are not made or distributed for profit or commercial advantage and that copies bear this notice and the full citation on the first page. To copy otherwise, to republish, to post on servers or to redistribute to lists, requires prior specific permission. Reactors: A Deterministic Model of Concurrent Computation for Reactive Systems by Hendrik Marten Frank Lohstroh A dissertation submitted in partial satisfaction of the requirements for the degree of Doctor of Philosophy in Computer Science in the Graduate Division of the University of California, Berkeley Committee in charge: Professor Edward A. Lee, Chair Professor Gul A. Agha Professor Alberto L. Sangiovanni-Vincentelli Professor Sanjit A. Seshia Fall 2020 Reactors: A Deterministic Model of Concurrent Computation for Reactive Systems Copyright 2020 by Hendrik Marten Frank Lohstroh i Abstract Reactors: A Deterministic Model of Concurrent Computation for Reactive Systems by Hendrik Marten Frank Lohstroh Doctor of Philosophy in Computer Science University of California, Berkeley Professor Edward A. Lee, Chair Actors have become widespread in programming languages and programming frameworks focused on parallel and distributed computing. While actors provide a more disciplined model for concurrency than threads, their interactions, if not constrained, admit nondeter- minism. As a consequence, actor programs may exhibit unintended behaviors and are less amenable to rigorous testing. The same problem exists in other dominant concurrency mod- els, such as threads, shared-memory models, publish-subscribe systems, and service-oriented architectures. We propose \reactors," a new model of concurrent computation that combines synchronous- reactive principles with a sophisticated model of time to enable determinism while preserving much of the style and performance of actors. Reactors promote modularity and allow for dis- tributed execution. The relationship that reactors establish between events across timelines allows for: 1. the construction of programs that react predictably to unpredictable external events; 2. the formulation of deadlines that grant control over timing; and 3. the preservation of a deterministic distributed execution semantics under quantifiable assumptions. We bring the deterministic concurrency and time-based semantics of reactors to the world of mainstream programming languages through Lingua Franca (LF), a polyglot coordination language with support (so far) for C, C++, Python, and TypeScript. In LF, program logic is given in one or more of those target languages, enabling developers to use familiar languages and integrate extensive libraries and legacy code. The main contributions of this work consist of a formalization of reactors, the imple- mentation of an efficient runtime system for the execution of reactors, and the design and implementation of LF. i To Rusi and Luka. ii Contents Contents ii List of Algorithms iii List of Figuresv List of Code Listings vii List of Tables ix 1 Introduction1 1.1 Motivation..................................... 1 1.2 Background.................................... 4 1.3 Contributions................................... 11 1.4 Related Work................................... 12 1.5 Outline....................................... 17 2 Reactors 18 2.1 Ports, Hierarchy, and Actions .......................... 19 2.2 State Variables .................................. 20 2.3 Connections.................................... 20 2.4 Example: Drive-by-wire System......................... 21 2.5 Formalization................................... 23 2.6 Dependency Analysis............................... 40 2.7 Execution Algorithm............................... 44 2.8 Implementations ................................. 50 3 Lingua Franca 54 3.1 Overview...................................... 54 3.2 Target Declaration ................................ 59 3.3 Import Statement................................. 60 3.4 Preamble Block.................................. 60 3.5 Reactor Definition ................................ 61 iii 3.6 Reaction Definition................................ 73 3.7 Banks and Multiports .............................. 74 3.8 Semantics ..................................... 78 4 Concurrency and Timing 83 4.1 Physical Actions in Reactive Systems...................... 84 4.2 Runtime Scheduling and Real-Time Constraints................ 88 4.3 Exposing More Parallelism............................ 91 4.4 Further Optimizations .............................. 94 4.5 Subroutines.................................... 97 4.6 Performance Benchmarks............................. 100 5 Federated Execution 106 5.1 Reasoning About Time.............................. 107 5.2 Decentralized Coordination ........................... 110 5.3 Centralized Coordination............................. 114 5.4 Support for Federated Programs in LF ..................... 114 5.5 Conclusion..................................... 119 6 Conclusion 121 6.1 Further Work................................... 121 6.2 Applications.................................... 126 6.3 Final Remarks................................... 127 Bibliography 128 A Summary of the Reactor Model 147 Index 149 iv List of Algorithms 1 Set a value on port p and trigger reactions................... 33 2 Schedule an action a................................ 34 3 Request execution to come to a halt....................... 36 4 Create a reactor instance given a reactor class and a container instance... 37 5 Start the execution of a reactor......................... 37 6 Delete a given reactor............................... 38 7 Connect port p to downstream port p0 ..................... 38 8 Disconnect p from downstream port p0 ..................... 40 9 Return the reaction graph of reactor r ..................... 41 10 Report the dependencies between all ports in reactor r ............ 43 11 Execute top-level reactor r ............................ 45 12 Execute triggered reactions until QR is empty................. 46 13 Detach and remove defunct reactors from reactor r .............. 48 14 Stop the execution of reactor r .......................... 48 15 Process the next event(s) for a top-level reactor r ............... 49 16 Recursively reset the values of all ports and actions of reactor r to absent.. 49 17 Assign levels to all reactions in a top-level reactor r .............. 89 18 Propagate deadlines between reactions in top-level reactor r ......... 91 19 Assign chain identifiers to reactions in a top-level reactor r .......... 95 v List of Figures 2.1 A reactor implementation of the introductory example. Reactor X has a startup reaction that produces an event on output ports dbl and inc. The second reaction of Y, triggered by input port inc, cannot execute 1) before Relay has reacted; and 2) until after the first reaction of Y has executed in case it was triggered by an event on dbl. ..................................... 19 2.2 A reactor that implements a simplified power train control module. 22 2.3 A reactor implementation of a simple \rock, paper, scissors" game. 44 2.4 A causality loop due to reaction priority....................... 44 2.5 A filtered version of diagram in Figure 2.4...................... 45 3.1 A flow chart describing the Lingua Franca compiler toolchain. 56 3.2 Graphical rendering of the \Hello World" program in Figure 3.7.......... 64 3.3 Timers are syntactic sugar for periodically recurring logical actions. 70 3.4 Constructing a strictly contracting function GN that models an LF program. 82 4.1 Diagram generated from the LF code in Listing 4.1. ............... 84 4.2 A deadline defines the maximum delay between the logical time of an event and the physical time of the start of a reaction that it triggers............. 86 4.3 A diagram of an LF program realizing a typical scatter/gather pattern. 90 4.4 A diagram of a pipeline pattern in LF; each stage executes in parallel. 90 4.5 An example reaction graph with assigned levels and IDs.............. 93 4.6 The reactor equivalent of a subroutine. ...................... 97 4.7 An alternative implementation of Figure 4.6 using a caller and callee port. 98 4.8 Reaction graphs explaining the dependencies in subroutine-like interactions. 99 4.9 A reactor implementation of the Savina PingPong benchmark. 101 4.10 PingPong: a comparison between Akka actors and reactors. 102 4.11 A reactor implementation of the Savina Philosophers benchmark. 103 4.12 A reactor implementation of the Savina Trapezoid benchmark. 103 4.13 Trapezoid: reduced execution time with a larger number of worker threads. 104 4.14 Trapezoid: a comparison between Akka actors and reactors. 105 5.1 A federated reactor that controls an aircraft door. Each reactor runs on a different host........................................... 106 vi 5.2 Different observers may see events in a different order. An additional logical timeline allows to establish a global ordering. After a certain safe-to-process (STP) threshold, Door received all relevant messages and can use the logical timeline to determine that
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages169 Page
-
File Size-