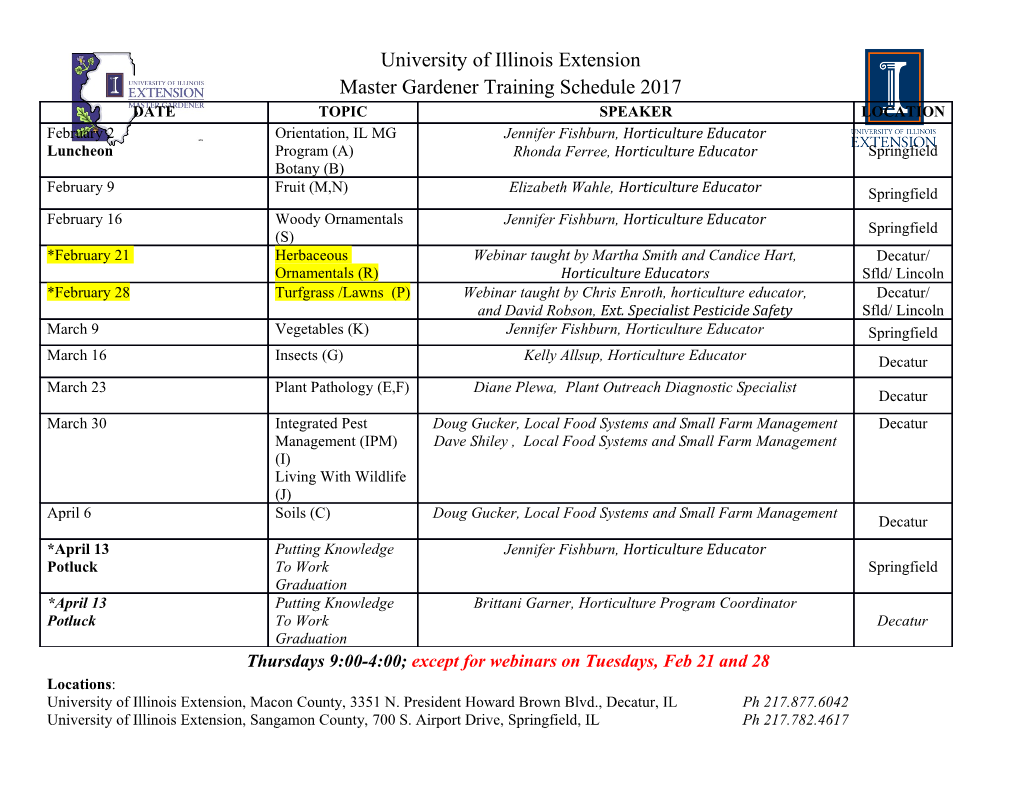
Lab 7: Filters and convolution One of the most remarkable properties of a linear dynamical system is its response to sinusoidal inputs. As we learned in lecture, a sinusoidal input to a linear dynamical system produces a sinusoidal output having the same frequency as the input. However, the output sinusoid can differ in two ways from the input sinusoid: 1) its amplitude, and 2) its phase. Measurements of the phase and amplitude of the output to a sinusoidal input can be used to identify the differential equation which best captures the input-output re- lationships of the dynamical system. The graphical technique for estimating the transfer function from these experimental observations is the Bode plot. Once the transfer function has been determined the response of the dynam- ical system to any realizable input can be predicted. The purpose of this laboratory is to use computer experiments to verify these observations and to introduce the concepts of a filter and convolution. 1 Background The lecture briefly reviews the concept of an impulse function, filtering and convolution. A practical, but important, detail concerns the representation of a sine wave wave in a computer program. We can write a simple program that plots a 10 second interval of a sine wave for a given frequency, f, and a given time step. import numpy as np import matplotlib.pyplot as plt f=0.1 N=11 NN=101 1 start=0.0 stop=100.0 t=np.linspace(start,stop,num=N,endpoint=True) tt=np.linspace(start,stop,num=NN,endpoint=True) plt.plot(t,np.sin(2*np.pi*f*t),'k-o') plt.plot(tt,np.sin(2*np.pi*f*tt),'r-') plt.axis([0,stop,-1.1,1.1]) plt.show() 1 The period, T , is f , so when f = 0:1, we have T = 10. If we choose start and stop to be, respectively, 0 and 100, then this would draw ten cycles of a 0:1Hz provided that N is chosen appropriately (red line in figure produced by this program). The positive integer, N, is the number of equally spaced time samples between, in this case, 0 and 100. It is important to remember that by choosing the endpoint=True option for np.linspace() both the starting and the end point are included, N points altogether1. The time step, dt is stop-start dt = times per period N What is a good choice of the number of time steps, N? If we choose N = 10, then dt = 1 per period and we would obtain 10 identical xalues of x since we are sampled once per period and the rtime series is 10 periods long (compare black and red lines). This is not a good choice of N since we cannot uniquely represent a function using a single value. Next we could try N = 20 which would give us two point per period. In principle we can uniquely represent a sine wave if we sample at twice the frequency (this is the concept of the Nyquist frequency that we will discuss in the next lab). However, x would not look like a nice sine wave. So we must pick N larger: the larger N, the smaller dt, and hence the more frequently the sine wave is sampled. However, it is also true that the larger N the more computer memory it takes to store in this case x and t, and the greater the number of calculations that the computer must make. Thus there is a practical trade{ off between the accuracy by which functions are to be represented and the time it takes the computer to complete its calculations. The bottom line is that as we change f we need to adjust dt. A reasonable choice might be to sample the sine wave 10 − 100 times per period. Ideally we would like to keep the number of sample points per period the same as we change f. 1Note that to get say 10 evenly spaced samples you need to set num= N + 1. 2 Browser use: Have available the document intro_python.pdf on your browser for review, in particular, the sections that deal with how to de- clare a function. As always, information concerning the use of Python and matplotlib functions can be obtained from the Internet using a browser. Housekeeping: Labs 8 and 9 use some of the programs and results that we develop in this lab. Thus it is useful to put today's material in a sub- directory of ~\pyprogs called, for example, convol, namely ~\pyprogs\convol. This will make it easier for you to find these results when we need them. Exercise 1: Bode plots: low{pass filter 1. Write a computer program to integrate dx = k x + sin 2πft ; (1) dt 1 where k1 is a constant. 2. Take k1 = −1. 3. Vary frequency over a large range (say f = 0:01 to f = 100) and measure the amplitude. Plot the amplitude versus frequency. 4. Vary the frequency and measure the shift between the sine wave and the solution of the equation. For example, we can plot the input and output sine wave n the same figure. Plot the phase shift versus the frequency. 5. Now change a to, let's say, k1 = 10 and then k1 = 0:1. What happens? 6. Construct the Bode plot by choosing a suitable range of f. For each choice of f enter the amplitude and phase shift of the steady{state solution and write these values in a *.tsv file. Use this *.tsv file to construct the phase part of the Bode plot. 7. You have just constructed your first low pass filter. Answer the follow- ing: • The frequency on the input sine wave and the output sine wave is the same. True of false? 3 • The output amplitude changes as a function of frequency. True or false? • The output sine wave is phase shifted compared to the input sine wave. True or false? • Why is this type of filter called a low pass filter? 8. What is the transfer function for (1)? 9. Show that the impulse function, I(t; t0), is 0 I(t; t ) = k1 exp(k1t) : (Remember that the impulse function corresponds to the case when the input is a delta function.) Is this the same as the answer we derived in class (Section 7.3)? 10. The transfer function, C(s), for the thermometer is of the form 1 C(s) = : 1 + αs Sketch the Bode plot. What is the corner frequency? 11. Consider the transfer function 10 C(s) = : s(1 + 0:5s)(1 + 0:1s) Sketch the Bode plot for the amplitude showing the contribution of each term. Exercise 2: The convolution integral: The concept of convolution is one of the most important concepts in science: every time one uses a device to make a measurement, one essentially performs a convolution. All physical observations are limited by the resolving power of instruments, and for this reason alone convolution is ubiquitous. This principle does not only apply to laboratory instruments: our senses, e.g. vision, touch, smell, audition, proprioception, are also convoluted by the sensory receptors which translate physical stimuli into neural spikes trains. This convolution is mathematically as the operation Z 1 y(t) = x(t)h(t − u)du ; (2) −∞ 4 where x(t) describes the input to the dynamical system and h(t − u) de- scribes the impulse function. In practice it is very easy to perform a convolu- tion numerically. In Python this is accomplished using the single command np.convolve() (similar commands exist, for example, in MATLAB). In or- der to use the convolution integral to solve (1) we need to know that the impulse function. Convolution: Graphical interpretation Although the mechanics of evaluating the convolution integral seem straight- forward, it is quite difficult to understand in a simple way the process of evalu- ating the convolution integral. The purpose of this exercise is to demonstrate a graphical interpretation of convolution [1]. This graphical method is very useful and, for example, we can use it to understand issues related to zero- padding and to appreciate the difference between convolution and correlation (Lab 14). Figure 1: Graphical method for convolving a delta{function input (vertical dashed line in 'Multiplication panel') with an alpha{type impulse response (solid line). See text for discussion. The graphical convolution method divides the process of convolution into 5 four steps [1]: 1. Folding: Reflect the impulse response, I(t), about the ordinate axis to obtain I(−t). 2. Displacement Shift I(−t) by an amount ∆t to obtain I(−t + ∆t). 3. Multiplication: Multiply I(−t + ∆t) by the input, b(t). 4. Integration: Determine the area under the curve produced by I(−t + ∆t) and b(t). Figure 1 illustrates this procedure in the evaluation of the alpha function response, I(t), of a neuron to a single delta-function input, b(t). The step `Folding' means that we reflect the impulse response about the y-axis. The step `Displacement' means that we slowly shift the reflected impulse in the positive direction along the x-axis. The step `Multiplication' means that we multiple the reflected impulse with the input. It is easiest to understand this step iteratively: for each time step do the multiplication (and then Step 4), then displace the reflected impulse response another time step to the right and repeat the process. In general this corresponds to the shaded region under the two curves for which both functions are non-zero. Finally the step `Integration' calculates the area under the the two curves.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages12 Page
-
File Size-