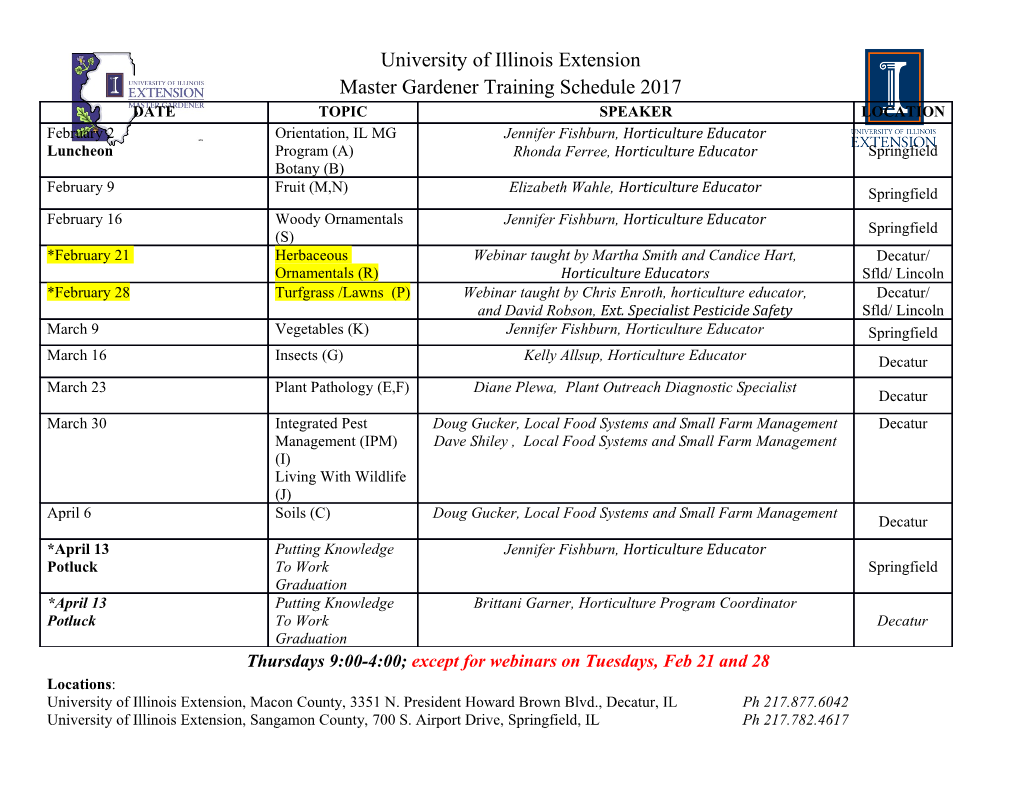
Department of Computer Science Real-time Code Generation in Virtualizing Runtime Environments Dissertation submitted in fulfillment of the requirements for the academic degree doctor of engineering (Dr.-Ing.) to the Department of Computer Science of the Chemnitz University of Technology from: Dipl.-Inf. (Univ.) Martin Däumler born on: 03.01.1985 born in: Plauen Assessors: Prof. Dr.-Ing. habil. Matthias Werner Prof. Dr. rer. nat. habil. Wolfram Hardt Chemnitz, March 3, 2015 Acknowledgements The work on this research topic was a professional and personal development, rather than just writing this thesis. I would like to thank my doctoral adviser Prof. Dr. Matthias Werner for the excellent supervision and the time spent on numerous professional discus- sions. He taught me the art of academia and research. I would like to thank Alexej Schepeljanski for the time spent on discussions, his advices on technical problems and the very good working climate. I also thank Prof. Dr. Wolfram Hardt for the feedback and the cooperation. I am deeply grateful to my family and my girlfriend for the trust, patience and support they gave to me. Abstract Modern general purpose programming languages like Java or C# provide a rich feature set and a higher degree of abstraction than conventional real-time programming languages like C/C++ or Ada. Applications developed with these modern languages are typically deployed via platform independent intermediate code. The intermediate code is typi- cally executed by a virtualizing runtime environment. This allows for a high portability. Prominent examples are the Dalvik Virtual Machine of the Android operating system, the Java Virtual Machine as well as Microsoft .NET’s Common Language Runtime. The virtualizing runtime environment executes the instructions of the intermediate code. This introduces additional challenges to real-time software development. One issue is the transformation of the intermediate code instructions to native code instructions. If this transformation interferes with the execution of the real-time application, this might introduce jitter to its execution times. This can degrade the quality of soft real-time systems like augmented reality applications on mobile devices, but can lead to severe problems in hard real-time applications that have strict timing requirements. This thesis examines the possibility to overcome timing issues with intermediate code execution in virtualizing runtime environments. It addresses real-time suitable generation of native code from intermediate code in particular. In order to preserve the advantages of modern programming languages over conventional ones, the solution has to adhere to the following main requirements: • Intermediate code transformation does not interfere with application execution • Portability is not reduced and code transformation is still transparent to a program- mer • Comparable performance Existing approaches are evaluated. A concept for real-time suitable code generation is developed. The concept bases on a pre-allocation of the native code and the elimination of indirect references, while considering and optimizing startup time of an application. This concept is implemented by the extension of an existing virtualizing runtime envi- ronment, which does not target real-time systems per se. It is evaluated qualitatively and quantitatively. A comparison of the new concept to existing approaches reveals high execution time determinism and good performance and while preserving the portability deployment of applications via intermediate code. Contents 1 Introduction 1 1.1 Motivation . .1 1.2 Problem Statement . .2 1.3 Objectives of this Work . .4 1.4 Structure . .5 2 Fundamentals 7 2.1 Introduction . .7 2.2 Real-Time . .7 2.3 Conventional Applications . .8 2.3.1 Introduction . .8 2.3.2 Compilation . 10 2.3.3 Linking . 13 2.3.4 Loading . 20 2.4 Virtualizing Runtime Environments . 22 2.4.1 Introduction . 22 2.4.2 Architecture and Services of a VM . 24 2.4.3 Workflow . 26 2.4.4 Examples of High-Level Language Virtual Machines . 30 2.5 Summary . 31 3 State of the Art 33 3.1 Introduction . 33 3.2 Real-Time Specifications . 33 3.2.1 Java Platform . 33 3.2.2 CLI Platform . 35 3.3 Hardware-supported Execution . 35 3.3.1 Java Platform . 35 3.3.2 CLI Platform . 36 3.4 Software-supported Execution . 37 3.4.1 Introduction . 37 3.4.2 Java Platform . 38 3.4.3 CLI Platform . 41 3.4.4 Low-Level Virtual Machine . 43 3.5 Summary . 43 4 Allocation of Native Code 45 4.1 Evaluation of Existing Approaches . 45 4.1.1 Hardware-supported Execution . 45 4.1.2 Software-supported Execution . 45 vii Contents 4.2 Concept . 48 4.3 JIT Compiler-based Pre-Compilation . 50 4.3.1 Principle . 50 4.3.2 Implementation . 52 4.4 Testing Environment . 53 4.5 Experimental Results and Evaluation . 61 5 Patching of Native Code 69 5.1 Lazy Compilation and Reference Handling . 69 5.2 Concept . 71 5.3 Implementation . 71 5.4 Experimental Results and Evaluation . 75 6 Optimization of Startup Time 79 6.1 Interim Analysis . 79 6.2 Reduction of Allocated Code . 79 6.2.1 Concept . 79 6.2.2 Implementation . 81 6.2.3 Experimental Results and Evaluation . 82 6.3 Checkpoint and Restore . 86 6.3.1 Concept . 86 6.3.2 Implementation . 86 6.3.3 Experimental Results and Evaluation . 87 7 Evaluation 89 7.1 Internal Experiments . 89 7.1.1 Introduction . 89 7.1.2 Standard Execution Mode . 89 7.1.3 Real-Time Code Generation Mode . 90 7.1.4 Summary . 94 7.2 Comparative Experiments . 95 7.2.1 Introduction . 95 7.2.2 Instance methods benchmark . 96 7.2.3 Interface Methods benchmark . 101 7.2.4 Class Methods benchmark . 104 7.2.5 Type Initializer benchmark . 108 7.2.6 Static Methods benchmark . 112 7.2.7 Static Class Methods benchmark . 115 7.2.8 Summary . 118 8 Summary and Outlook 121 8.1 Discussion . 121 8.2 Conclusion . 122 8.3 Outlook . 124 viii Contents List of Abbreviations 125 Bibliography 136 ix List of Figures 1.1 Normalized execution times of 1000 interface methods that are written in C# and run by the virtualizing runtime environment “Mono” version 2.6.1 in JIT mode . .3 2.1 Process’ point of view on a machine. .8 2.2 Workflow of a native program from source code to a running executable . 10 2.3 Calling a dynamically linked function in ELF executable. 21 2.4 Process Virtual Machine . 23 2.5 Compiler and Loader in conventional and HLL VM environment (derived from Figure 5.1 in [109, Ch. 5]) . 24 2.6 Abstract Architecture of a High-level Language Virtual Machine . 25 2.7 Workflow of a program from source code to execution by a HLL VM . 27 4.1 Pre-compilation based on Mono’s JIT compiler. 50 4.2 Triggering Mono’s JIT compiler via managed code. 53 4.3 Standard Deviation of the simple and complex benchmark variant written in C++, IA-32 . 60 4.4 Frequency Distribution of the observed execution times of the simple and complex benchmark variant written in C++, IA-32 . 60 4.5 Frequency Distribution of the observed execution times of the third and fourth measurement of Mono in JIT mode, IA-32 . 62 4.6 Standard Deviation of execution times of 1000 methods (note the logarith- mic scale), IA-32 . 63 4.7 Startup time of 1000 methods . 67 5.1 Call of a lazily compiled method in Mono . 70 5.2 Interplay of Pre-Compilation and Pre-Patch . 72 5.3 Pre-Patch of a method call through the Virtual Table . 73 5.4 Pre-Patch of a method call through the Interface Method Table . 74 5.5 Frequency Distribution of the observed execution times of the all four mea- surements of MonoRT in Pre-Patch mode, IA-32 . 76 5.6 Standard deviation of execution times of 1000 methods, IA-32 . 76 5.7 Startup time of 1000 methods, IA-32 . 77 6.1 Interplay of AOT compiler based Pre-Compilation and Pre-Patch . 80 6.2 Frequency Distribution of the observed execution times of the all four mea- surements of MonoRT in AOT-based Pre-Patch mode, IA-32 . 83 6.3 Standard deviation of execution times of 1000 methods, IA-32 . 84 6.4 Startup time of 1000 methods, IA-32 . 85 6.5 Startup time of 1000 methods, ARM . 86 xi List of Figures 6.6 Execution times of 1000 methods using the JIT-based pre-compilation mode of MonoRT, ARM . ..
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages152 Page
-
File Size-