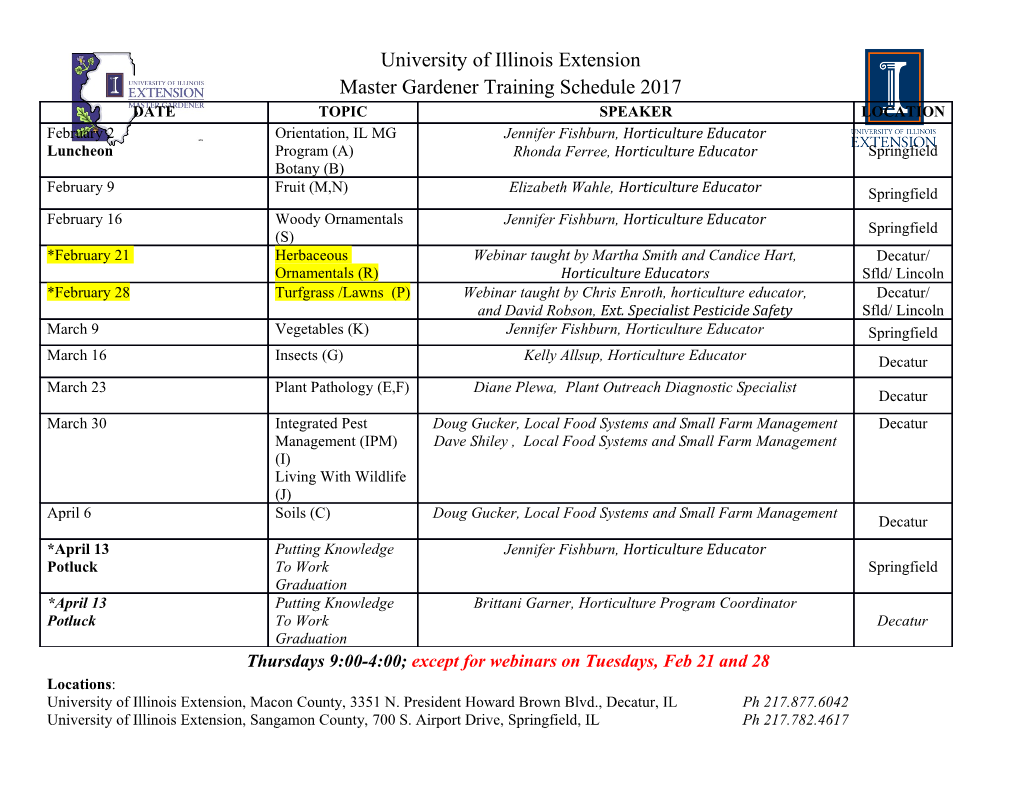
04Chap.fm Page 77 Monday, November 25, 2002 9:15 PM C HAPTER 4 The ADO .NET Class Library ActiveX Data Objects .NET is in some ways a misnomer. It implies that ADO .NET is implemented as an ActiveX object library, the same way as ADO was. In a sense this is true, because like ADO, ADO .NET wraps the OLEDB COM classes. However, unlike ADO, ADO .NET is not itself an ActiveX object library. It is implemented as a collection of .NET classes. ADO .NET also has a high-performance native mode for SQL Server that does not use OLEDB at all! The basis for ADO .NET is XML. Perhaps it should have been called XDO for XML Data Objects. As we dissect the class library, you will see why. ADO .NET CLASS LIBRARY OVERVIEW The central class in ADO .NET is the DataSet. While it is tempting to com- pare the DataSet to the ADO RecordSet object, it is really a different type of object entirely. The ADO .NET DataSet is really a repository for XML data. ADO .NET has more to do with reading and persisting XML data than it does accessing databases. Instead of its primary function being to access data- bases, its primary function is to access, store, and save XML documents from many different sources. The database is just one of those sources. Data is per- sisted as World Wide Web Consortium (W3C)–compliant XML, so any con- sumer complying with the standard, no matter the platform, can use it. The DataSet can be used as a stand-alone object for storing any data you can imagine. It is also designed to be the remoting agent of choice. Since it is designed to read any XML data stream, it uses this method for marshalling data between remote sources and local programs. 77 04Chap.fm Page 78 Monday, November 25, 2002 9:15 PM 78 Chapter 4 • The ADO .NET Class Library The DataSet is composed of a collection of tables, relations, and con- straints. Does anyone remember the in-memory database that was supposed to be released with Windows 2000 and then was pulled from the release at the last minute? Well here it is, reincarnated as the DataSet. That’s right, the class has all the power you need for an in-memory database, including tables, indexes, foreign keys, and DRI. It can draw its data from a database, or you can use it as a stand-alone in-memory data cache that can be persisted to disk or sent over the network. The other main classes are for connecting to and running queries on data- bases. There are two main branches to the class library, the SqlClient classes and the OleDbClient classes. As we said before, SqlClient is optimized for SQL Server 7.0 and later databases. It uses native access and does not require OLEDB. The OleDbClient classes use the OLEDB data providers. They can be used for Oracle, Access, and any other supported database except ODBC. There is a special version of the OLEDB ODBC driver available for down- load from the Microsoft site. More on that later. Connection Class The connection classes provide the means to connect to the database. They also manage transactions, and connection pooling. The various parameters for connecting to a database are set via the ConnectionString property. In previous versions of ADO, most of the ConnectionString values were exposed through properties. For security reasons, ADO .NET only exposes the ConnectionString. We will enumerate the details of the various values of the ConnectionString in the chapter on the Connec- tion object. Command Class The Command class provides methods for storing and executing SQL state- ments and stored procedures. It has a Parameters collection that is used to pass parameters into and out of stored procedures. The collection can also be used to set the parameters for a parameterized SQL statement. The Data- Adapter class contains four Command classes for selecting, updating, insert- ing, and deleting data. The class can also be used by itself for non-databound access to the database. Of all the ADO .NET classes, the Command class is the closest to the ADO Command class in functionality and remains essen- tially unchanged as far as properties and methods go. Of course, it is a .NET Framework class and does not use COM at all. It also has some enhance- ments over the old ADO Command object. 04Chap.fm Page 79 Monday, November 25, 2002 9:15 PM Comparison of ADO .NET and ADO 2.x 79 DataReader Class The DataReader is used to retrieve data. It is used in conjunction with the Command class to execute an SQL Select statement and then access the returned rows. It provides forward-only read-only access to the data. The DataReader is also used by the DataAdapter to pump data into a DataSet when the Fill method of the DataAdapter is called. The DataReader pro- vides high-speed access to data. You can use a DataReader to pull data for web pages that are read-only, reports, graphs or any other application that does not require data binding and only displays data. The DataAdapter Class The DataAdapter is used to connect DataSets to databases. It is a go-between class. It is comprised of four Command classes and one DataReader class. The DataAdapter is most useful when using data-bound controls in Windows Forms, but it can also be used to provide an easy way to manage the connec- tion between your application and the underlying database tables, views, and stored procedures. Another way of looking at the DataAdapter class is as a way of containing SQL statements and stored procedures for easy program- matic access. All of the SQL needed to maintain a database entity can be con- tained in a DataAdapter object. The DataAdapter also uses support classes that will create the SQL statements for updating the database from a pre- defined Select statement, and will fill the parameter collections of the Command objects contained in the class. There is also a wizard that helps create the DataAdapter and another wizard that will create a DataSet from the Command objects contained within the DataAdapter. COMPARISON OF ADO .NET AND ADO 2.X Since ADO .NET is so different from ADO 2.x (or just ADO), a comparison is a good idea. Those of you who are fluent with ADO will find the transition easier if you have some basis for associating ADO practices with ADO .NET’s. For those of you who have never used ADO, it is still worth the time to see how ADO .NET evolved from ADO and why the changes were made. Here we will look at the differences from a programming point of view. Also, just because ADO .NET is the new kid on the block doesn’t mean that there are not still applications that would be better served by ADO. Each has its strengths and weaknesses. 04Chap.fm Page 80 Monday, November 25, 2002 9:15 PM 80 Chapter 4 • The ADO .NET Class Library Two Different Design Goals ADO and ADO .NET are fundamentally different because the designers had two different goals in mind when they designed the software. ADO is a descendant of the Data Access Objects (DAO)/Remote Data Object (RDO) models. One goal of the designers of ADO was to combine the two older data access technologies into one homogeneous object model that could be used to access any supported database. The other goal was to reduce dependency on open database connectivity (ODBC), an older database standard and replace it with OLEDB, a COM-based standard for connecting to databases. Because of this, the ADO object model was aligned with the two older technologies, DAO and RDO. ADO is also an inherently connected technology. This means that an open database connection is maintained for the life of any of the RecordSet objects that hold data. In later versions of ADO (2.1 and later) it was possible to use a discon- nected architecture. This was added to support stateless web applications. Most desktop applications continued to use the connected model. In some cases this is because the applications needed the concurrency support and were highly transactional. In other cases it was because that was what the programmer knew how to do. ADO .NET was designed to support the stateless model by default. It is an inherently disconnected architecture. While ADO .NET continues to sup- port the OLEDB COM-based database access standard, the new goal is to eliminate even that. ADO .NET uses the new SOAP object protocol and uses XML as its storage and communications medium. The SqlClient classes that support SQL Server are the example of the direction Microsoft is headed. The plan is for developers to create native ADO .NET class libraries to support their database product. The SqlClient classes do not use OLEDB. It is one of the long-term goals of Microsoft to eliminate COM. If it seems to you that they took a 180-degree turn, you are not imagining things. That is part of the reason for this book. The ADO Architecture and Practice With all that said, let’s very quickly review the ADO object model. There are three main objects in ADO. • The Connection object manages database connections and transactions. • The Command object encapsulates SQL statements and manages access to stored procedures. It contains a Parameters collection to manage pass- ing data into and out of stored procedures. 04Chap.fm Page 81 Monday, November 25, 2002 9:15 PM Comparison of ADO .NET and ADO 2.x 81 • The RecordSet object holds the results of SQL queries and implements methods for navigating through the rows and for adding, deleting, and updating rows.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages19 Page
-
File Size-