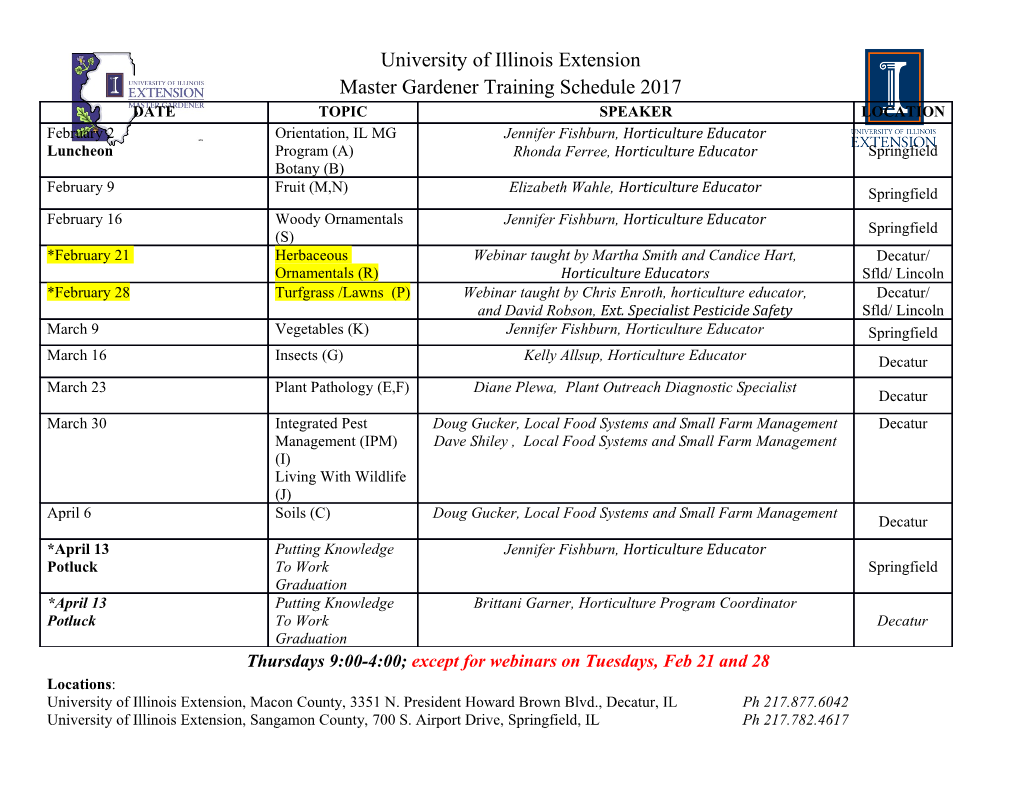
PHP A server-side, cross-platform, HTML-embedded Lecture 28 scripting language used to create dynamic Web pages (like Perl open source). We can write PHP code directly into HTML and don’t PHP need to worry about CGI (like Java Server Pages). April 6, 2005 Looks like Perl and knowledge of one can help you understand the other. Web server needs to be configured to parse files with certain extensions (e.g. php or phtml) appropriately. PHP Serve-side Scripting Server-side scripting SSI: Server-side includes: Most CGI tools: very old scripting language write page headers, define layout, etc. mostly provides #include few really dynamic parts, most static PHP: have to write many static print statements complete language Let’s design pages by writing static HTML documents free with pieces of code inside: integrates nicely into Apache, IIS, other servers code is executed at request time pages can take parameters ASP: Active Server Pages Scripting languages come with libraries providing Microsoft version of PHP functions specially designed for Web programming: language is essentially Basic e.g.: URL/parameters manipulations, database gateways, ... JSP: Java Server Pages Java version PHP PHP Most popular third-party module for Apache: <html> code and extensive documentation available from <head><title>PHP Test</title></head> http://www.php.net/ <body><? print(“Hello World!”); ?></body> </html> Pieces of code are enclosed into <?...?> tags Example: <html> <html> <head><title>PHP Test</title></head> <head><title>PHP Test</title></head> <body>Hello World!</body> <body><? print(“Hello World!”); ?></body> </html> </html> 1 Parameter Decoding Parameter Decoding Each PHP script can be invoked as a CGI. Each PHP script can be invoked as a CGI. Parameters are decoded automatically and appear to the script as Parameters are decoded automatically and appear to the script as normal variables. normal variables. Example: Example: http://www.cse.nd.edu/~cpoellab/myphp.php?x=2&y=hello http://www.cse.nd.edu/~cpoellab/myphp.php?x=2&y=hello <html> <html> <head><title>PHP Test</title></head> <head><title>PHP Test</title></head> <body> <body> <? <? print(“x= \n”); print(“x=$x\n”); print(“y= \n”); print(“y=$y\n”); ?> ?> </body> </body> </html> </html> More Preset Variables Variables PHP creates many other variables automatically: All names begin with $ e.g. $variable $HTTP_USER_AGENT, $REMOTE_ADDR, etc. list: <? phpinfo(); ?> Alphabetic character or underscore (_) must Example: follow $ <? if(strstr($HTTP_USER_AGENT, “MSIE”)) { ?> Remaining chars are alphanumeric or <center><b>You are using Internet Explorer</b></center> underscore <? } else { ?> <center><b>You are not using Internet Names are case-sensitive $A is not $a Explorer</b></center> <? } ?> Types determined by first assignment Did you notice that you can split a PHP program into pieces separated by HTML? Data Types String Handling Integer Characters within strings can be obtained via Whole numbers –2,147,483,648 to 2,147,483,647 subscripting Floating Point Subscripts start at 0 Decimal values in the range 1.7E-308 to 1.7E308 String $hello = “Hello World” Sequence of characters e.g. “Hello World” $hello[1] = ? 2 Outputting a String Length of a String The function echo() outputs a string to the The function strlen() returns a string’s length standard output e.g. echo(“Hello World”); $hello=“Hello World” $astring = “Hello World”; The value of strlen($hello) is 11 echo($astring); Getting a Number from a Expressions String Includes normal arithmetic operations Use the string in a calculation $i=$i+1 or $i++ PHP converts as much as it can to a number $i=$i-1 or $i-- Also / (divide) and * (multiply) % is the modulo operation $var=“1234” May use brackets to specify precedence $num=$var[2] + 5 Statements separated by semi-colons Expressions evaluate to true or false $num contains the number...? PHP Control Structures PHP Arrays Like all languages, PHP has control structures: Very useful feature: array variable type (set <? of key-value associations). while ($x!=0) { ... $role = array { } “Poellabauer” => “teacher” if ($x==0) { “You” => “student” ... } }; elseif ($x==1) { $role[“You”] = “student”; ... $me = $role[“Poellabauer”]; } ?> 3 PHP Arrays PHP Arrays Special case: array indexed by integers: What about array of arrays? $students = array ( $names = array(“Poellabauer”, “You”, “Your neighbor”); “You” => array ( “name” => “yourname”, “student nb” => 1234), $myname = $names[0]; “Another” => array ( “name” => “hisname”, “student nb” => 5678), “Foobar” => 42 $names[3] = “Another student”; ); $you = $students[“You”]; $yourname = $students[“You”][“name”]; print(“<pre>”); print_r($students); // This will print a human-readable version of $students print(“</pre>”); print_r print_r <pre> Array ( <? [a] => apple $a = array ('a' => 'apple', 'b' => 'banana', 'c' [b] => banana => array ('x', 'y', 'z')); [c] => Array ( print_r ($a); [0] => x ?> [1] => y [2] => z </pre> ) ) PHP Functions PHP Functions You can define functions: Predefined functions: tons! function addition($x, $y) { Classified into categories: return $x+$y; Apache, arrays, Aspell, BC, Bzip2, Calendar, CCVS, COM, Classes/Objects, ClibPDF, Crack, } CURL, Cybercash, CyberMUT, Cyradm, ctype, $foo = addition(31,11); dba, Date/Time, dBase, DBM, dbx, DB++, DIO, Directories, DOM XML, .NET, Errors and Logging, FrontBase, filePro, Filesystem, FDF, FriBiDi, FTP, Functions, gettext, GMP, HTTP, Hyperwave, ... check http://www.php.net/manual/en/ 4 PHP Functions Getting Data From Client Look at following code: Data is often supplied to server side <? programs via HTML forms function lm($x) { printf(“<font size=-1>[Last modified: %s]</font> “, date(“D, d M Indicated by the <form> tag Y H:i:s”, filemtime(“$x”))); } Specifies HTTP method and field names ?> Field names become variables in PHP scripts <html> Field name myfield becomes $myfield <body> Download your new <a href=“foo.ps.gz”>assignment</a> <? lm(“foo.ps.gz”); ?> </body> </html> A Simple Form Simple Form Output <html> <head> <title>Multiply Form</title> </head> <body><h1>Enter multiplier</h1> <form action="multiply.php" method="POST"> <input type="text" name="multiplier"> </form></body> </html> PHP Script Output <html> <head><title>Times Table</title></head> <body> <?for($i=1;$i<=12;$i++){ echo($i); ?> * <?echo($multiplier);?> = <?echo($multiplier*$i);?> <br> <?}?> </body> </html> 5 Getting it on the Server Manipulating HTTP Headers Treat PHP scripts like ordinary HTML pages By default, PHP scripts are assumed to generate HTML: Except save in files called .php Content-Type field of response defaults to text/html Suitably equipped Web Server does the rest You can force it otherwise: <? $len = filesize(“foo.pdf”); header(“Content-Type: application/pdf”); header(“Content-Length: $len”); readfile(“foo.pdf”); ?> You can only manipulate HTTP headers BEFORE displaying anything. This won’t work: <? print(“foo”); header(“Content-Type: text/plain”); ?> Access Control Access Control You may want to control access to certain pages: You can also use HTTP basic authentication: administration pages, secrets, ... first HTTP request: return HTTP code 401 “please Check IP address: authenticate yourself” if ($REMOTE_ADDR!=“130.207.7.245”) { client types username and password header(“HTTP/1.0 403 forbidden access”); another HTTP request containing both: authorized to enter print(“You are not authorized to access my diary”); PHP has standard variables for both: exit; $PHP_AUTH_USER and $PHP_AUTH_PW } will only work if you know exactly who can send requests Documents are not encrypted: from that address even username/password are sent in clear text what if you want to access page from Cybercafe? anyone who intercepts the request can gain access Access Control Access Control <? //Check if the request carries an authentication if (!isset($PHP_AUTH_USER)) { //No authentication header(‘HTTP/1.0 401 Unauthorized’); header(‘WWW-Authenticate: Basic realm=“PHP Secured”’); print(“Please authenticate yourself”); exit; } elseif (($PHP_AUTH_USER==“Poellabauer”)&&($PHP_AUTH_PW==42)) { //Correct authentication print(“Hello, $PHP_AUTH_USER. You can enter.”); } else { //Wrong authentication header(‘HTTP/1.0 403 Forbidden’); print(“Go away, you are not authorized here!”); exit; } ?> 6 Session Tracking Session Tracking By default, a web server is stateless. Send pieces of data (“cookies”) to client: client attaches same cookie to each further request However, often you want to establish a if server sends a different cookie to each new client, then it can session: track which request has been made by which client Cookie: keep memory of past requests of user name (“userid”) example: e-commerce: value (“174848848”) expiration date (“21 march 2005) browse to view products path (“/myshop/”) add items to shopping basket server domain (“.cse.nd.edu”) checkout security level (“0”) until cookie expires, name and values will be sent together with every request addressed at a page whose server belongs to “.cse.nd.edu” and whose path starts with “/myshop/” Session Tracking Session Tracking Cookies are transmitted in HTTP response header. You can store whatever you need to <? remember about each user with cookies. if (!isset($userid)) { srand((double)microtime()*1000000); PHP has a feature to automate sessions for $newid = rand(); $expire = time() + 3600; // in 1 hour you: setcookie(“userid”,$newid,$expire,”/myshop/”,”.cse.nd.edu”,0);
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages9 Page
-
File Size-