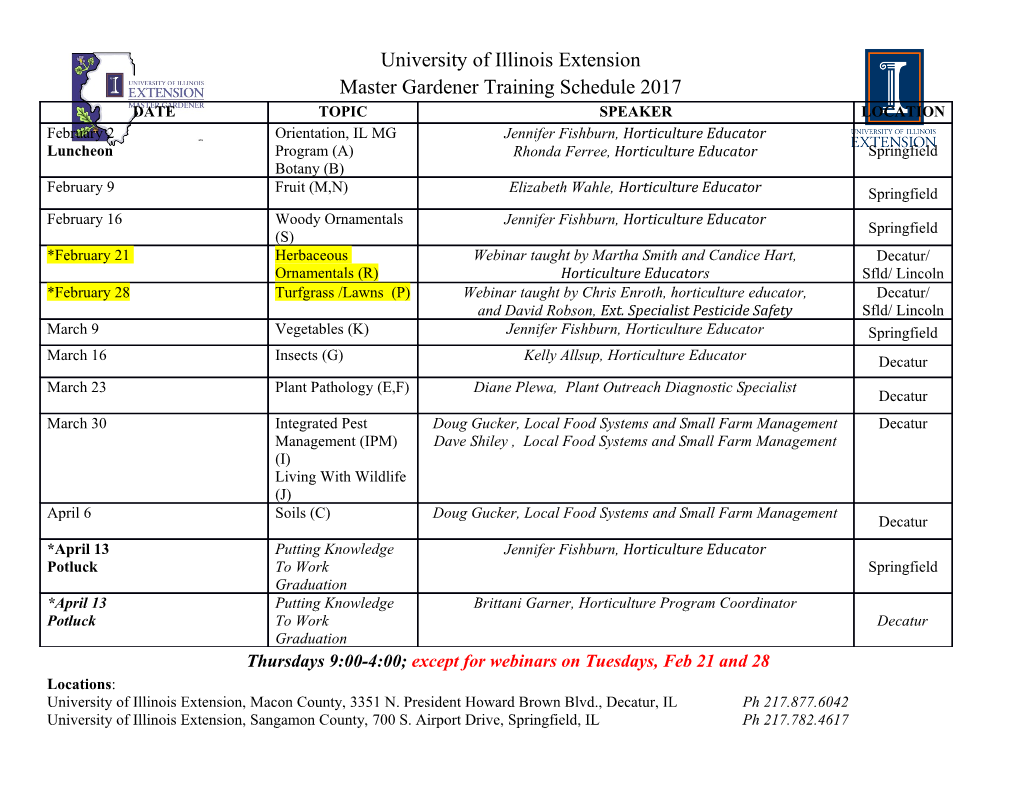
A PROCEDURE MECHANISM FOR BACKTRACK PROGRAMMING* David R. HANSON + Department o£ Computer Science, The University of Arizona Tucson, Arizona 85721 One of the difficulties in using nondeterministic algorithms for the solution of combinatorial problems is that most programming languages do not include features capable of easily repre- senting backtracking processes. This paper describes a procedure mechanism that uses co- routines as a means for the description and realization of nondeterministic algorithms. A solution to the eight queens problem is given to illustrate the application of the procedure mechanism to backtracking problems. I. INTRODUCTION Although backtrack programming has been known for To facilitate comparison with previous work, the several years [1-4], the method has yet to become eight queens problem [13-15] is used as the exam- a common programming technique for the realization ple o£ backtracking throughout this paper. This of nondeterministic algorithms. Floyd [1] alluded is a nontrivial problem whose solution is ideally to the reason for this situation: most program- suited to the backtracking strategy, and has ming languages do not include features that facil- frequently been used as an example that can be itate backtrack programming. He suggested that solved by nondeterministic programming. programming languages ought to possess mechanisms capable of representing nondeterministic algo- 2. THE SL5 PROGRAMMING LANGUAGE rithms. SL5 is an expression-oriented language that is Since the appearance of Floyd's paper, consider- structurally similar to BLISS or Algol 68. SL5 able research has been undertaken to add facili- is a "typeless" language in the same sense that ties of this kind to new or existing languages. SNOBOL4 is -- a variable can have a value of any This work has covered a large part of the spec- datatype at any time during program execution. trum of programming languages, from general de- scriptions with a slant toward Algol-like lan- 2.1 Control Structures and Signaling guages [5,6], to languages for artificial intel- ligence research [7], and even to Fortran [8]. An expression returns a signal, "success" or In all the work cited, features that were added "failure", as well as a value. The combination or proposed for backtracking were cast in a o£ a value and a signal is called the result of framework of recursive functions with additional the expression. SL5 possesses most o£ the "mod- built-in mechanisms or primitives with which to ern" control structures, each of which is an implement backtracking. That is, the basic pro- expression and returns a result. Control cedure mechanism of the proposed languages or structures are driven by signals rather than by language extensions was the traditional recursive boolean values. For an example, in the expression function. i_~f e 1 then e 2 else e 3 This paper presents a general procedure mechanism that includes coroutines as a means for the de- e I is evaluated first. If the resulting signal scription and realization of nondeterministic is success, e 2 is evaluated. Otherwise, e 5 is algorithms. The SL5 programming language [9-12] evaluated. The result of the if-then-else ex- in which this procedure mechanism is implemented pression is the result (value and signal) o£ e 2 is the vehicle used to describe this method and or e 3, whichever is evaluated. its application to backtrack programming. Other typical control structures are: *This work was supported by the National Science while e I do e 2 Foundation under Grant DCR75-01307. until e I do e 2 repeat e +Author's present address: Department of Computer for v from e I to e 2 do e 5 Science, Yale University, New Haven, Connecticut I 06520. 401 The while and for expressions behave in the con- whichreturn V as the value of the procedure and ventlo~-6n~manner. The until expression repeated- signal either success or failure as indicated. ly evaluates e 2 until e I succeeds. The repeat If the procedure is activated by a resume, the expression evaluates e repeatedly until a failure result given in succeed or fail is transmitted signal is returned. Expressions may be grouped and becomes the result of teh-6"~esume expression. together as a single expression using The execution of succeed or fail causes the sus- begin ... end or { ... }. pension of that environment. If the environment is again resumed, execution proceeds from where 2.2 Procedures it left off. The argument v may be omitted, in which case the null string is assumed. In SL5, procedures and their environments (activation records) are separate source-language A label generator illustrates a simple example of data objects. A procedure is "created" by an coroutine usage: expression such as genlab :={procedure (n) gcd := procedure (x, y) repeat while x ~= y do succeed "U' l] lp~Cn, 3, "0"); if x > y then x := x-y else y := y-x; n := n+1 succeed x } end; end; which assigns to gcd a procedure that computes An environment for genlab generates the next the greatest common divisor of its arguments. label of the form Lnnn each time it is resumed. The sequence begins at the integer given by the The invocation of a procedure in the standard argument. (lpad is a built-in procedure that recursive fashion is accomplished using the usual pads n on the left with zeros to form a functional notation f(el,e 2 ..... en) , which S-character string, and [] denotes string concat- invokes the procedure that is the current value enation.) For example, an expression such as of the variable f. gen := create genZab with 10 Procedure activation may be decomposed into sev- eral distinct source-language operations that assigns to gen an environment for genlab that permit SL5 procedures to be used as coroutines. generates a sequence of labels beginning at L010. These operations are the creation of an environ- To obtain the next label, the execution of the ment for the execution of the given procedure, environment is resumed; the bindin~ of the actual arguments to that envi- ronment, and the resumption of the execution of x := resume gen the procedure. Notice that the sequence may be restarted by The create expression takes a single argument of retransmitting the argument, e.g., datatype procedure, creates an environment for its execution, and returns this environment as gen := gen with 10 its value. For example, the expression 2.3 Declarations e := create f SL5 has declarations for identifiers that are assigns to e an environment for the execution of used to determine only the interpretation and f. scope of identifiers that appear in a procedure, not their type. The declaration The with. expression is used to bind the actual arguments to an environment. The expression private x e with (el,e 2, .... e n) declares x to be a private identifier whose value is available only to the procedure in which it is binds the actual arguments, e I through en, to the declared; it cannot be examined or modified by environment e. any other procedure. Private identifiers are used, for example, when a coroutine must "remem- The execution of a procedure is accomplished by ber" information from one resumption to the next. "resuming" it via the resume expression. The Other declarations and the scope of identifiers expression are described in refs. 9 and 12. resume e 3. BACKTRACKINGAND THE EIGHT QUEENS PROBLEM suspends execution of the current procedure and There are many problems for which an analytic activates the procedure for which e is an envi- solution is not known, but for which a solution ronment. can be constructed by trial and error. A classic example is the eight queens problem, sometimes A procedure usually "returns" a result to its referred to as the n-by-n nonattacking queens "resumer". This is accomplished by the expressions problem. The object is to place eight queens on a chess board so that no queen can capture any of succeed v the others. One such solution is shown in fig. I. 402 There are 92 solutions to this problem, although For example, it is easy to place the first five only 12 are unique. queens to form the partial solution (1,3,5,2,4). But the sixth queen cannot be placed. It is necessary to backtrack to the partial solution (1,3,5,2) and try again. This partial solution 8 can be extended to (1,3,5,2,8) but no further. It is necessary to backtrack all the way to the 7 partial solution (1,3,5), which can then be ex- 6 tended to (1,3,5,7,2,4,6). This backtracking process continues until the solution 5 (1,5,8,6,3,7,2,4) is found, which is shown in tOW fig. 1. 4 A more formal description of the backtracking 5 strategy is given in ref. 2. A particularly lucid explanation can be found in ref. 16, which 2 describes a method for estimating the efficiency of backtracking programs. 5.2 Realization of the Nondeterministic I 2 5 4 5 6 7 8 Algorithm column The usual method for programming the solution to the eight queens problem is to use a procedure Fig. 1 - A Solution to the Eight Queens Problem that generates all solutions with the first queen on rows 1 to 8 by calling itself recursively to generate all solutions for the second queen in A brute force approach to this problem is to test rows 1 to 8, etc. The foliowing procedure, simi- all the possible configurations of the queens to lar to the Pascal solution given in ref. 15, find the 92 "safe" ones.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages5 Page
-
File Size-