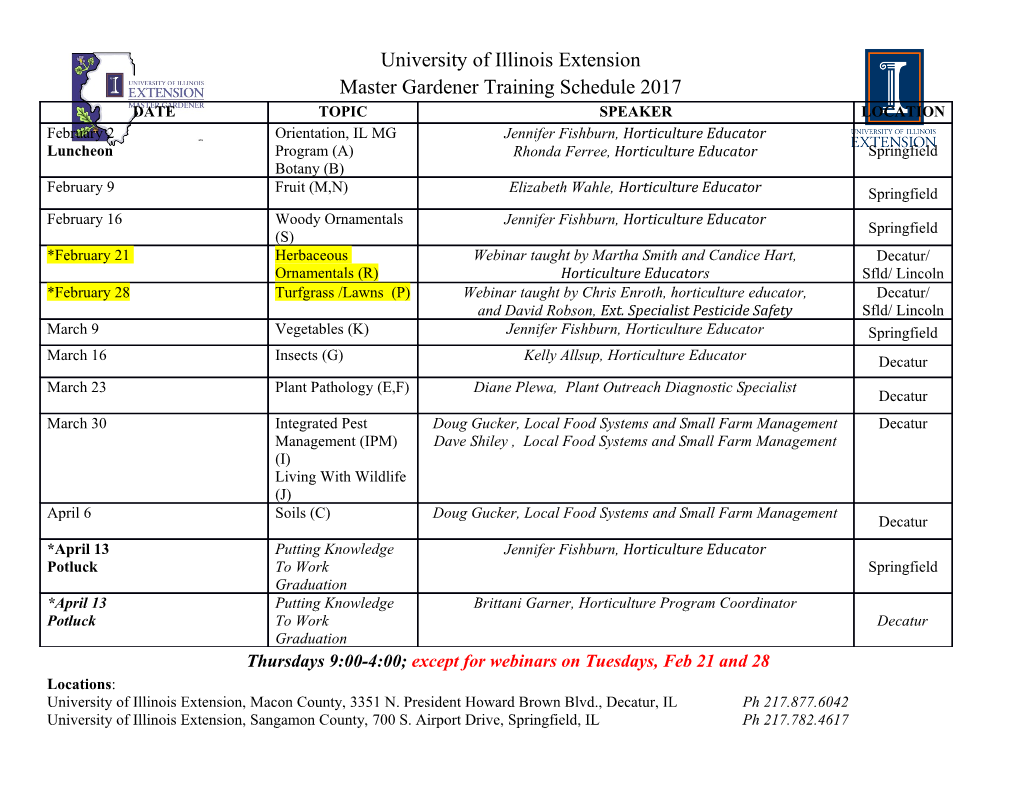
ICCAS2003 October 22-25, Gyeongju TEMF Hotel, Gyeongju, Korea Development of a Frame Buffer Driver for Embedded Linux Graphic System Ga-Gue Kim, Woo-Chul Kang, Young-Jun Jung, and Hyung-Seok Lee Embedded S/W Technology Center, ETRI, Daejeon, Korea (Tel : +82-42-860-1123; E-mail: {ggkim, wchkang, jjing, [email protected]) Abstract : A frame buffer device is an abstraction for the graphic hardware. It allows application software to access the graphic hardware through a well-defined interface, so that the software doesn’t need to know anything about the low-level inter face stuff. We develop a frame buffer driver for VIA’s CLE266 graphic system based on ‘Qplus’, an embedded linux operating system developed by ETRI. Then, it will be seen that our frame buffer system is applied to embedded solutions such as movie player an d X server successfully. Keywords: frame buffer, embedded linux, Qplus, abstract console 1. INTRODUCTION framebuffer devices abstract them. Also, we will see that fbdev actually handles most of the mode setting issues for you Many machines (M68K, SPARC, PowerMac) use graphical to make life much easier. In the older API, the console code console because either the hardware does not support (VGA) was heavily linked to the framebuffer devices. The newer API text mode, or because the firmware programs the hardware has now moved nearly all console handling code into fbcon into a graphical mode. Thus the linux kernel needs to be aware itself. Now, fbcon is a true wrapper around the video card’s of this and support graphical consoles on these architechtures. abilities. This allows for massive code reduction and easier Graphical consoles will also gain more importance on the driver development. A good example of a framebuffer driver Intel platform, as VGA compatibility will be phased out by is the virtual framebuffer (vfb). The vfb driver is not a true many graphics chipset manufacturers in the near future. An framebuffer driver. All it does is map a chunk of memory to early example of this the Cyrix MediaGX], which provides userspace. It's used for demonstration purposes and testing. VGA compatibility through its BIOS only. 2.1 Data Structures The frame buffer device provides an abstraction for the graphics hardware. It represents the frame buffer of some The framebuffer drivers depend heavily on four data video hardware and allows application software to access the structures. These structures are declared in fb.h. They are graphics hardware through a well-defined interface, so the fb_var_screeninfo , fb_fix_screeninfo , fb_monospecs , and software does not need to know about the low level (hardware fb_info . The first three can be made available to and from register) stuff (except for hardware acceleration)[1]. userland. First let me describe what each means and how they Since years there has been minor support for graphical are used. consoles in the linux kernel, but it differed a lot among the fb_var_screeninfo is used to describe the features of a video different platforms. Starting with kernel 2.1.107, the frame card you normally can set. With fb_var_screeninfo , you can buffer device abstraction become completely integrated in the define such things as depth and the resolution you want. standard kernel sources and will become the standard way to The next structure is fb_fix_screeninfo . This defines the access graphics hardware. But it is definitely not new: it properties of a card that are created when you set a mode and originated from linux/M68K at the end of 1994 and has can't be changed otherwise. A good example is the start of the proven to fulfill its task during the previous years. framebuffer memory. This can depend on what mode is set. The frame buffer device abstraction has the following Now while using that mode, you don't want to have the advantages: memory position change on you. In this case, the video hardware tells you the memory location and you have no say (1) It provides a unified method to access graphics about it. hardware across different platforms. The third structure is fb_monospecs . In the old API, the (2) Drivers can be shared among different architectures, importance of fb_monospecs was very little. This allowed for which reduces code duplication. There were already three forbidden things such as setting a mode of 800x600 on a fix different drivers for the ATI Mach64 before. In the ideal case, frequency monitor. With the new API, fb_monospecs prevents a frame buffer device driver contains a chipset driver core, such things, and if used correctly, can prevent a monitor from with machine and bus dependent probe being cooked. code(Zorro/PCI/ISA/Open Firmware/…). The final data structure is fb_info . This defines the current (3) It provides simple multi-head: currently up to 8 frame state of the video card. fb_info is only visible from the kernel. buffer devices (displays) are supported. Unfortunately the Inside of fb_info , there exist a fb_ops which is a collection of input part of the console subsystem is not ready for multi-head needed functions to make fbdev and fbcon work. yet. (4) On boot up, you get one or more penguin logos (with or 2.2 Driver Layout without beer) The more CPUs you have, the more penguins Here I describe a clean way to code your drivers. A good you will see. example of the basic layout is vfb.c. In the example driver, we first present our data structures in the beginning of the file. Note that there is no fb_monospecs since this is handled by 2. FRAME BUFFER INTERNAL API code in fbmon.c. This can be done since monitors are Now that we understand the basic ideas behind video card independent in behavior from video cards. First, we define our technology and mode setting, we can now look at how the three basic data structures. For all the data structures I defined them static and declare the default values. The reason I do this is because it's less memory intensive than to allocate a piece of #ifdef CONFIG_FB_YOUR_CARD memory and filling in the default values. Note that drivers that extern void xxxfb_of_init(struct device_node *dp); support multihead (multiple video cards) of the same card, #endif /* CONFIG_FB_YOUR_CARD */ then the fb_info should be dynamically allocated for each card Then in the function offb_init_driver, you add something present. For fb_var_screeninfo and fb_fix_screeninfo , they still similar to the following: are declared static since all the cards can be set to the same #ifdef CONFIG_FB_YOUR_CARD mode. if (!strncmp(dp->name,"Open Firmware number of your card ", size_of_name)) { xxxfb_of_init(dp); 2.3 Initialization and boot time parameter handling There are two functions that handle the video card at boot return 1; time: } #endif /* CONFIG_FB_YOUR_CARD */ int xxfb_init(void); int xxfb_setup(char*); If Open Firmware doesn't detect your card, Open Firmware sets up a generic video mode for you. Now in your driver you In the example driver as with most drivers, these functions really need two initialization functions. are placed at the end of the driver. Both are very card specific. The next major part of the driver is declaring the functions In order to link your driver directly into the kernel, both of of fb_ops that are declared in fb_info for the driver. these functions must add the above definition with extern in The first two functions, xxfb_open and xxfb_release , can be front to fbmem.c. Add these functions to the following in called from both fbcon and fbdev. In fact, that's the use of the fbmem.c: user flag. If user equals zero then fbcon wants to access this device, else it's an explicit open of the framebuffer device. static struct { This way, you can handle the framebuffer device for the const char *name; console in a special way for a particular video card. For most drivers, this function just does a MOD_INC_USE_COUNT or int (*init)(void); MOD_DEC_USE_COUNT . int (*setup)(char*); These are the functions that are at the heart of mode setting. } fb_drivers[] __initdata = { There do exist a few cards that don't support mode changing. #ifdef CONFIG_FB_YOURCARD For these we have this function return an -EINVAL to let the { "driver_name", xxxfb_init, xxxfb_setup }, user know he/she can't set the mode. Actually, set_var does #endif more than just set modes. It can check them as well. In fb_var_screeninfo , there exists a flag called activate. This flag Setup is used to pass card specific options from the boot can take on the following values: FB_ACTIVATE_NOW , prompt of your favorite boot loader. A good example is: FB_ACTIVATE_NXTOPEN , and FB_ACTIVATE_TEST . FB_ACTIVATE_TEST tells us if the hardware can handle boot: video=matrox: vesa: 443 what the user requested. FB_ACTIVATE_NXTOPEN sets the values wanted on the next explicit open of fbdev. The final The basic setup function is: one FB_ACTIVATE_NOW checks the mode to see if it can be done and then sets the mode. You MUST check the mode int __init xxxfb_setup(char *options) before all things. Note that this function is very card specific, { but I will attempt to give you the most general layout. The char *this_opt; basic layout then for xxxfb_set_var is: if (!options || !*options) static int vfb_set_var(struct fb_var_screeninfo *var, struct return 0; fb_info *info) for (this_opt = strtok(options, ","); this_opt; { this_opt = strtok(NULL, ",")) int line_length; if (!strcmp(this_opt, "my_option")) { /* Basic setup test. Here we look at what the user passed /* Do your stuff. Usually set some static flags that the in that he/she wants.For example to test the fb_var_screeninfo driver later uses */ field vmode like its done in vfb.c.Here we see if the user has } else if (!strncmp(this_opt, "Other_option:", 5)) FB_VMODE_YWARP.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages5 Page
-
File Size-