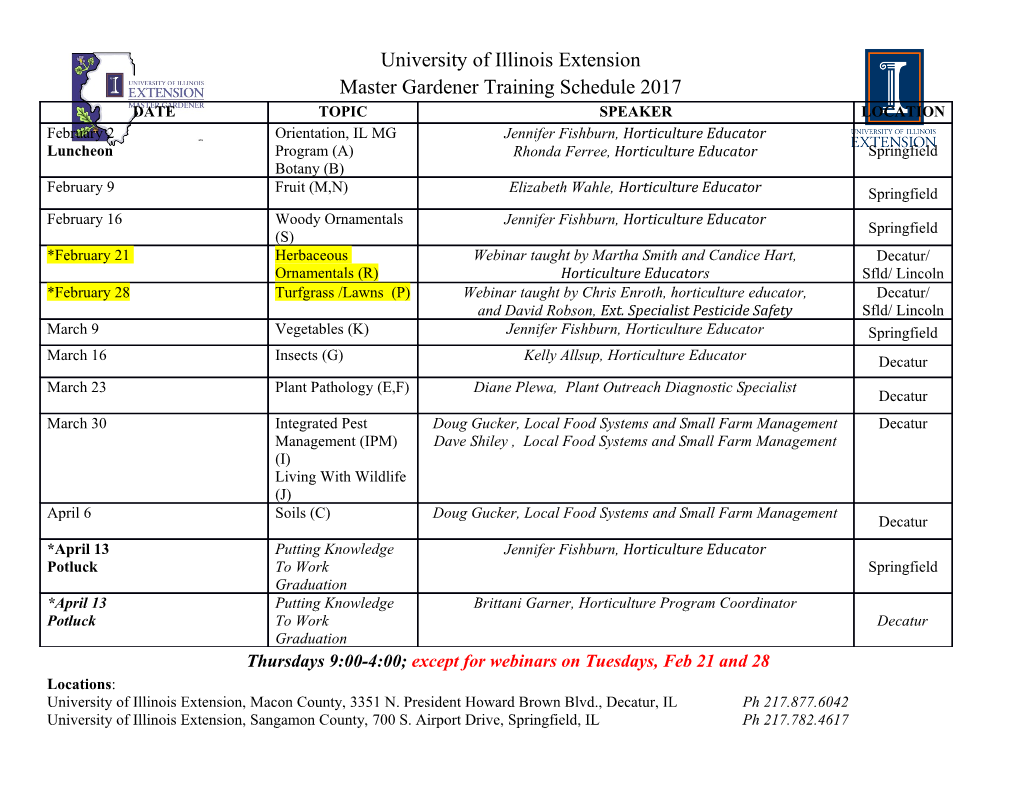
A Bit of Prolog Course Wrap-Up CS F331 Programming Languages CSCE A331 Programming Language Concepts Lecture Slides Monday, April 27, 2020 Glenn G. Chappell Department of Computer Science University of Alaska Fairbanks [email protected] © 2017–2020 Glenn G. Chappell Review 2020-04-27 CS F331 / CSCE A331 Spring 2020 2 Review Introduction to Scheme Scheme is a Lisp-family PL with a minimalist design philosophy. Scheme code consists of parenthesized lists, which may contain atoms or other lists. List items are separated by space; blanks and newlines between list items are treated the same. (define (hello-world) (begin (display "Hello, world!") (newline) ) ) When a list is evaluated, the first item should be a procedure (think “function”); the remaining items are its arguments. 2020-04-27 CS F331 / CSCE A331 Spring 2020 3 Review Scheme: Macros [1/5] We have noted that Scheme (and other Lisp-family PLs) offer excellent support for reflection: the ability of a program to examine and modify its own code. The most common way that reflection is done in Scheme is through the use of a macro: a procedure-like entity that takes its arguments unevaluated. Because the arguments of a macro are not evaluated before the macro sees them, the macro has access not only to their values, but also to their structure (AST). It can do anything it wants with that structure, transforming the code in arbitrary ways. After the macro has transformed the code, the resulting code is evaluated. (A macro may return the transformed code unevaluated, by quoting it.) 2020-04-27 CS F331 / CSCE A331 Spring 2020 4 Review Scheme: Macros [2/5] One kind of Scheme macro is the pattern-based macro. We give a pattern that code can match. Any matching code is transformed in a manner that we specify. The resulting code is evaluated. (Again, to avoid this last step, we can quote the transformed code). Define a single-pattern macro using define-syntax-rule. (define-syntax-rule (PATTERN) TEMPLATE) Here is our own version of quote: myquote. (define-syntax-rule (myquote x) 'x ) 2020-04-27 CS F331 / CSCE A331 Spring 2020 5 Review Scheme: Macros [3/5] define-syntax-rule accepts the dot syntax, just like define. Here is a quoting macro that goes inside the list it quotes. qlist is like list, except that it does not evaluate its arguments. (define-syntax-rule (qlist . args) 'args ) Try it. > (qlist (+ 1 3) (* 3 8)) ; Arguments NOT evaluated ((+ 1 3) (* 3 8)) > (list (+ 1 3) (* 3 8)) ; Arguments evaluated (4 24) 2020-04-27 CS F331 / CSCE A331 Spring 2020 6 Review Scheme: Macros [4/5] A more general way to define macros uses define-syntax. This allows for multiple patterns. The first matching pattern is used. (define-syntax IDENTIFIER (syntax-rules () [(PATTERN1) TEMPLATE1] … [(PATTERNn) TEMPLATEn] ) ) 2020-04-27 CS F331 / CSCE A331 Spring 2020 7 Review Scheme: Macros [5/5] (define-syntax IDENTIFIER (syntax-rules (KEYWORD1 … KEYWORDm) [(PATTERN1) TEMPLATE1] … [(PATTERNn) TEMPLATEn] ) ) The mysterious list after syntax-rules is the list of keywords. A keyword in a pattern matches only that exact word. For example, the “else” facility in the cond construction might be implemented as a keyword. 2020-04-27 CS F331 / CSCE A331 Spring 2020 8 A Bit of Prolog 2020-04-27 CS F331 / CSCE A331 Spring 2020 9 A Bit of Prolog Logic Programming Languages [1/3] In the late 1960s, Stanford researcher Cordell Green proposed representing a computer program in terms of logical statements. The result was a programming paradigm that became known as logic programming. In logic programming, a computer program can be thought of as a knowledge base. It typically contains two kinds of knowledge. § Facts. Statements that are known to be true. § Rules. Ways to find other true statements from those known. Execution is then driven by a query: essentially a question. The computer attempts to answer the question using facts and rules. 2020-04-27 CS F331 / CSCE A331 Spring 2020 10 A Bit of Prolog Logic Programming Languages [2/3] In a typical logic programming language: § A program consists of facts and rules. § Execution begins with a query, which establishes a goal. During execution, various subgoals may be established. § Execution is primarily interactive, based on a source file. § Execution is about finding what can be proven—not what is true. § So negation says something fails to be provable, not that it is false. § Unification* is the primary proof tool. § Typing is dynamic and implicit. *See the next slide. 2020-04-27 CS F331 / CSCE A331 Spring 2020 11 A Bit of Prolog Logic Programming Languages [3/3] Execution in logic PLs is typically based on unification. To unify two constructions means to make them the same by setting values of variables as necessary. Can we unify X and 42? Yes. Set X to 42. Unification, in full generality, includes assignment and expression evaluation as special cases. But unification can also do things that assignment/evaluation cannot do. Can we unify [A, 6] and [4, B]? Yes. Set A to 4, B to 6. Can we unify [A, 6] and [B, 7]? No. 2020-04-27 CS F331 / CSCE A331 Spring 2020 12 A Bit of Prolog History In the early 1970s, a group at the U. of Aix-Marseille (France) began an effort to produce a logic programming language. The group was led by Alain Colmeraur, and Cordell Green’s ideas were their starting point. The PL was called Prolog, short for “PROgrammation en LOGique” (French for “programming in logic”). The first version of Prolog was released in 1972. Prolog was, and continues to be, the most important logic PL. Prolog execution is driven by a query, which establishes a goal. The primary execution strategy involves unification. Prolog attempts to unify using backtracking search. 2020-04-27 CS F331 / CSCE A331 Spring 2020 13 A Bit of Prolog Code Prolog programs consist of facts and rules. Here is a Prolog fact. is_child(glenn, gilford). If something can be unified with a fact, then it is considered to be proven. Here are two Prolog rules. is_parent(A, B) :- is_child(B, A). is_grandparent(A, B): is_parent(A, X), is_parent(X, B). In order to be unified with the head of a rule (part before “:-”), the various parts in the tail (the rest) must be proven. 2020-04-27 CS F331 / CSCE A331 Spring 2020 14 Course Wrap-Up 2020-04-27 CS F331 / CSCE A331 Spring 2020 15 Course Wrap-Up From the First Day of Class: Course Overview — Description In this class, we study programming languages with a view toward the following. § How programming languages are specified, and how these specifications are used. § What different kinds of programming languages are like. § How certain features differ between various programming languages. 2020-04-27 CS F331 / CSCE A331 Spring 2020 16 Course Wrap-Up From the First Day of Class: Course Overview — Goals After taking this class, you should: § Understand the concepts of syntax and semantics, and how syntax can be specified. § Understand, and have experience implementing, basic lexical analysis, parsing, and interpretation. § Understand the various kinds of programming languages and the primary ways in which they differ. § Understand standard programming language features and the forms these take in different programming languages. § Be familiar with the impact (local, global, etc.) that choice of programming language has on programmers and users. § Have a basic programming proficiency in multiple significantly different programming languages. 2020-04-27 CS F331 / CSCE A331 Spring 2020 17 Course Wrap-Up From the First Day of Class: Course Overview — Topics The following topics will be covered: § Formal Languages & Grammars. § PL Feature. Execution I: compilers, interpreters. § PL #1. Lua. Red: Track 1 — Syntax & semantics § Lexing & Parsing. of programming languages § PL Feature. Type Systems. Blue: Track 2 — Programming- § PL #2. Haskell. language features & categories, and specific programming languages § PL Feature. Values & variables. § PL #3. Forth. § Semantics & Interpretation. We are § PL Feature. Reflection. here. § PL #4. Scheme. § PL Feature. Execution II: execution models, flow of control. § PL #5. Prolog. We lost a week of classes, so we did not have time to cover these. 2020-04-27 CS F331 / CSCE A331 Spring 2020 18 Course Wrap-Up Specification [1/2] We covered specifying the syntax of a programming language. Our primary tool was the (phrase structure) grammar, specified in practice using EBNF or something similar. statement → ‘print’ ‘(’ print_arg { ‘,’ print_arg } ‘)’ We also covered informal methods and regular expressions. We took a close look at two classes of formal languages—and the associated grammars that generate them—regular languages and context-free languages. We briefly mentioned formal methods for specifying semantics, but did not actually use any of these. 2020-04-27 CS F331 / CSCE A331 Spring 2020 19 Course Wrap-Up Specification [2/2] Our 2 main classes of languages are in the Chomsky Hierarchy. Language Category Generator Recognizer Number Name Type 3 Regular Grammar in which each production Deterministic Finite has one of the following forms. Automaton • A → ε Think: Program that uses a small, fixed amount of memory. • A → b • A → bC Another kind of generator: regular expressions. Type 2 Context- Grammar in which the left-hand side Nondeterministic Push-Down Free of each production consists of a Automaton single nonterminal. Think: Finite Automaton + Stack • A → [anything] (roughly). Type 1 Context- Don’t worry about it. Don’t worry about it. Sensitive Type 0 Computably Grammar (no restrictions). Turing Machine Enumerable Think: Computer Program 2020-04-27 CS F331 / CSCE A331 Spring 2020 20 Course Wrap-Up Lexing, Parsing, Interpretation We looked at how to write a lexical analyzer (lexer) and a syntax analyzer (parser).
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages24 Page
-
File Size-