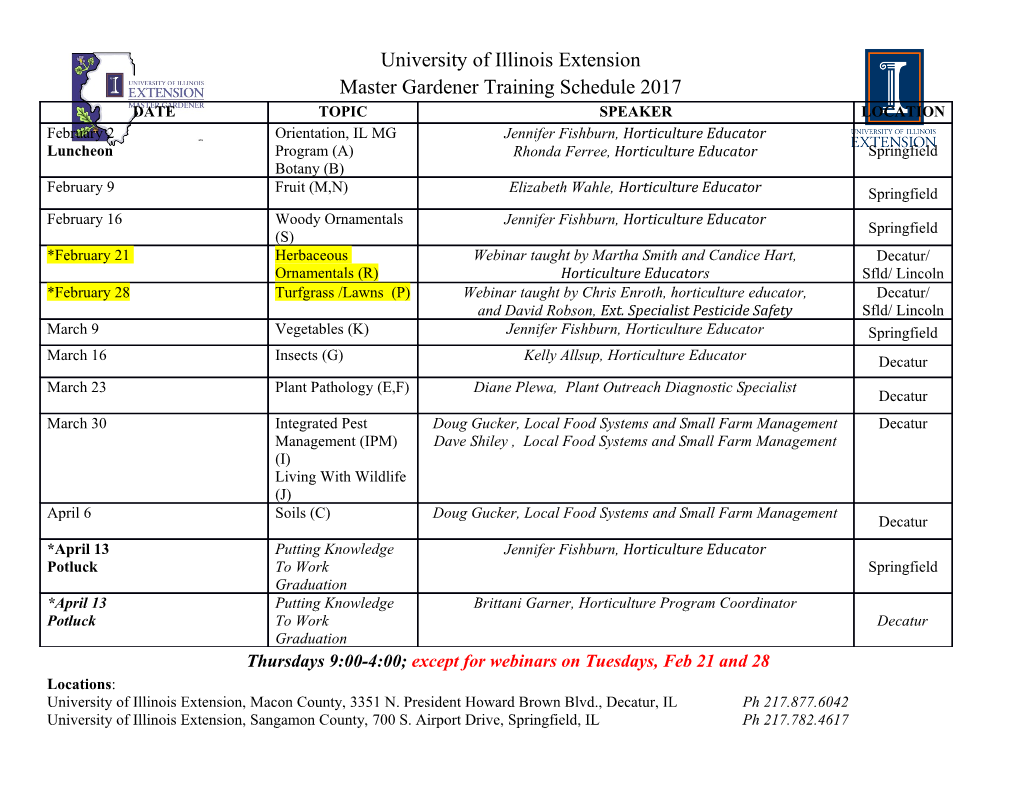
Table of Contents Introduction .................................................... -3- Computer Languages .............................................. -4- Problem Solving Strategies ......................................... -7- The Liberty Basic Environment ...................................... -11- Liberty Basic Commands ........................................... -13- Variables and Constants ............................................ -19- Mathematical Operators ............................................ -23- Error Checking and Debugging ...................................... -27- Manipulating Strings .............................................. -31- Mathematical Functions ........................................... -32- Decision Statements .............................................. -37- Iteration ....................................................... -44- Structured Programming ........................................... -47- Graphic User Interface (GUI) ....................................... -49- Graphics ....................................................... -64- Preface This guide was produced to introduce students to the Liberty Basic programming language. The topics of graphical user interface and graphics introduce concepts related to “Windows” object oriented programming. These topics were included so students might experience the transition from code-based programming to the object oriented environment of Visual Basic that is used in the CMP 621 course. The Teacher's Guide and Lab Manual for Liberty Basic were created by Lianne Garland during the summer of 2006. The Department appreciates the time and work that has gone into the creation of these manuals and would like to thank Ms. Garland for her efforts. We are interested in the ongoing development of these materials. Any comments, suggestions or further exercises would be welcome. Efforts will be made to share these content suggestions and support materials through an online workspace. Please contact the Senior High Technology Specialist at (902) 368-5725 with your suggestions or ideas. Liberty Basic Beginning Computer Programming Introduction Computer technology is all around us: ATM machines, fuel injection in cars, digital cameras, and telephone communications as well as our personal computers. It is important to know that none of this technology would be possible without computer programming. Programming provides instructions that tell the machine how to operate. Whether it is playing video games or typing a document, the computer requires a set of instructions in order to accomplish the task that you would like it to perform. Hardware and Software Recall that all of the physical components of a computer make up the hardware and may include your printer, monitor, and other peripheral device. It is easiest to think of of the word “hardware” as describing those parts of a computer that can be touched. Programs represent software. Recall that there are two main types of software: application software and system software. Application software refer to a set of programs that make a real life task easier to do, like word processors. System software helps the computer deal with the basic operations, like opening, closing, saving and printing files, as well as communicating with other peripheral devices. System software includes the operating system. A computer program tells the computer how to accept some type of data (input), manipulate that data (process and/or store), and spit it back out again (output). They can be thousands, even hundred thousands of single line commands. Each command doing a small task that the computer obeys. Input and output for various programs Type of program Input What the program does Output Word Processor Characters typed from Formats the text, corrects Displays and prints keyboard spelling neatly organized text Game Keystrokes or joystick Calculates how fast and moves a cartoon Figure movements how far to move a cartoon on screen figure on screen Web browser HTML codes stored On Converts the HTML codes Displays web pages on other computers into text and graphics the computer screen If you want your computer to do something specific, you can purchase a software package that will do this for you, or you could write your very own program which tells the computer what to do, step by step. 3 Liberty Basic Beginning Computer Programming Computer Languages Languages are used in everyday life for communication. In order to communicate effectively, you need to have an understanding of the language in order to convey to others your needs and wants. Computer languages are similar to spoken languages in that you must use them very precisely so that you are not misunderstood. Computers don’t understand English (or French, etc), so a set of step by step instructions must be written using a language that the computer can understand, called a programming language. Each language has its own grammar and spelling or syntax which must be followed for the computer to understand that language. Consider these examples from spoken languages: English: Hello, how are you? French: Bonjour! Ça va bien? German: Guten Tag. Wie geht’s? Japanese: Konnichi wa. O genki desu ka? All these examples mean the same thing: Each sentence has a greeting followed by a question asking how you are. But each example is a completely different group of words. Unless you know these languages, you may not know that each means the same thing. Computer languages are similar in that there are basic tasks which any computer language must do for a programmer. The programmer just has to learn to “speak” the language. One advantage of a computer language over a spoken language is that is does not take that long to become fluent in a computer language! Many programmers learn several languages during their careers. Now read these examples from some computer languages: QBasic if ( x > 5) then: print “greater” Pascal if x > 5 then writeln (‘greater’) C++ if ( x > 5) cout << “greater” Java if (x > 5) System.out.println (“greater.”) All these statements accomplish the same task: If the contents in the variable called “x” is greater than the number “5,” then we will print the word “greater” on the computer screen. 4 Liberty Basic Beginning Computer Programming Programming Languages Pascal, FORTRAN, C++, BASIC, ...each language serves a specific purpose. There are two levels of language among programming languages: high level languages and low level languages. Low Level Programming Languages Low level programming languages are those that are closest to what the computer understands. In reality, computers talk in a language that has 2 words: 0 and 1. It's called a binary system. Other than ten, the most common number-system base is two. In fact, the base- two (or binary) system, which consists of nothing but 0's and 1's, is the foundation of every computer's CPU. Assembly language was developed by programmers so programming would be easier. It uses short, easy to remember phrases such as JMP, MOV, and ADD, which represent specific machine language instructions. Computers can’t read assembly language, so it must be translated into machine language using assemblers. High Level Programming Languages High level languages, such as FORTRAN, COBOL, BASIC and Pascal, were developed because writing machine language or assembly language programs was difficult and confusing. Programming languages were made to look more like ordinary human language in hopes that it would be easier to write programs and make modifications. 5 Liberty Basic Beginning Computer Programming Translating the code Translators break down high level and low level language code into machine language understood by the particular processor in the C.P.U. There are two kinds of translators: interpreters and compilers. When translating any language, human or computer, into another language, one of two methods is generally used. 1. The complete file is translated at one time and then read later. This means that the original needs only to be translated once and then the translated version can be used as often as necessary. This is known as compiling and a compiler is used. 2. The file is translated one line at a time, and is used as it is translated. This means that the original is translated every time that it is used. This is known as interpreting, and an interpreter is used. Rapid Application Development (RAD) programming languages Most programming languages were designed back in the days when a computer screen displayed nothing but text. No graphics, mouse pointers, buttons or windows. When computers developed Graphical User Interfaces (GUI) with windows, scroll bars, and toolbars, people began demanding programs that included all these GUI features as well. To help programmers create programs with GUI features, many companies developed special dialects of existing languages, dubbed rapid application development (RAD) languages. RAD languages enable programmers to design the way they want their program to look and then write source code to make that user interface actually do something useful, such as display information in a window. Three popular RAD languages are Visual Basic (derived from BASIC), Delphi (based on Pascal), and C++ Builder (based on C++). Liberty Basic can use this feature as well. 6 Liberty Basic Beginning Computer Programming Programming is Problem Solving A program tells the computer how to solve a specific problem. A program can be as short as a single instruction or it can contain thousands of instructions.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages74 Page
-
File Size-