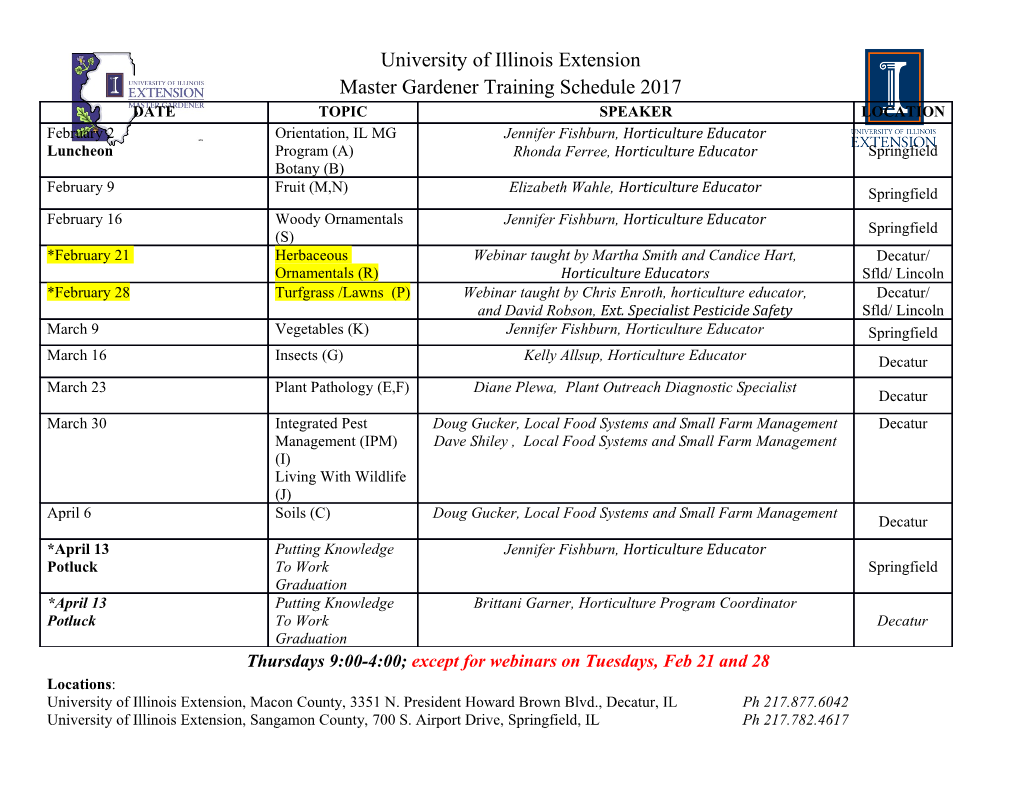
Networks • Mechanism by which two processes exchange information and coordinate activities Computer Computer Inter-process Communication process Computer CS 217 process NetworkNetwork Computer Computer 1 2 Inter-process Communication Pipes •Sockets • Provides an interprocess communication channel o Processes can be on any machine Processes can be created independently o output input o Used for clients/servers, distributed systems, etc. Process A Process B •Pipes o Processes must be on same machine o One process spawns the other • A filter is a process that reads from stdin and writes to stdout o Used mostly for filters stdin Filter stdout 3 4 Pipes (cont) Creating a Pipe • Many Unix tools are written as filters output o grep, sort, sed, cat, wc, awk ... Process A input Process B • Shells support pipes ls –l | more • Pipe is a communication channel abstraction who | grep mary | wc o Process A can write to one end using “write” system call ls *.[ch] | sort o Process B can read from the other end using “read” system call cat < foo | grep bar | sort > save • System call int pipe( int fd[2] ); return 0 upon success –1 upon failure fd[0] is open for reading fd[1] is open for writing • Two coordinated processes created by fork can pass data to each other using a pipe. 5 6 Pipe Example Dup int pid, p[2]; • Duplicate a file descriptor (system call) ... int dup( int fd ); if (pipe(p) == -1) duplicates as the lowest unallocated descriptor exit(1); fd pid = fork(); • Commonly used to redirect stdin/stdout if (pid == 0) { close(p[1]); • Example: redirect stdin to “foo” ... read using p[0] as fd until EOF ... int fd; } fd = open(“foo”, O_RDONLY, 0); else { close(0); close(p[0]); dup(fd); ... write using p[1] as fd ... close(fd); close(p[1]); /* sends EOF to reader */ wait(&status); } 7 8 Dup (cont) Pipes and Standard I/O • For convenience… int pid, p[2]; if (pipe(p) == -1) dup2( int fd1, int fd2 ); exit(1); use fd2(new) to duplicate fd1 (old) pid = fork(); closes fd2 if it was in use if (pid == 0) { • Example: redirect stdin to “foo” close(p[1]); dup2(p[0],0); fd = open(“foo”, O_RDONLY, 0); close(p[0]); dup2(fd,0); ... read from stdin ... close(fd); } else { close(p[0]); dup2(p[1],1); close(p[1]); ... write to stdout ... wait(&status); } 9 10 Pipes and Exec() K&P’s Example int pid, p[2]; #include <signal.h> close(tty); #include <stdio.h> if (pipe(p) == -1) istat = signal(SIGINT, SIG_IGN); exit(1); system( char *s) { pid = fork(); int status, pid, w, tty; qstat = if (pid == 0) { signal(SIGQUIT, SIG_IGN); fflush(stdout); close(p[1]); tty = open(“/dev/tty”, while ( dup2(p[0],0); O_RDWR); (w = wait(&status)) != pid close(p[0]); if (tty == -1) { && (w != -1) execl(...); fprintf(stderr, “...” ); ; return -1; } if (w == -1) status = -1; } else { signal(SIGINT, istat); close(p[0]); if ((pid = fork()) == 0 ) { signal(SIGQUIT, qstat); dup2(p[1],1); close(0); dup(tty); close(1); dup(tty); close(p[1]); return status; close(2); dup(tty); } ... write to stdout ... execlp(“sh”, “sh”, “-c”, s, wait(&status); NULL); } exit(127); } 11 12 Unix shell (sh, csh, bash, ...) Client-Server Model • Loop o Read command line from stdin o Expand wildcards o Interpret redirections < > | o pipe (as necessary), fork, dup, exec, wait Server Network (file server, • Start from code on previous slides, edit it until it’s a Unix Network mail server, shell! Client web server) Web browser Passive participant Emailer Waiting to be contacted Applications Active participant Initiate contacts 13 14 Message Passing Network Subsystem • Mechanism to pass data between two processes Sender sends a message from its memory User Application o Level Receiver receives the message and places it into its memory program o Socket API • Message passing is like using a telephone TCP or UDP Reliable data stream o Caller or unreliable data grams o Receiver Kernel IP Routes through the internet Level Device Driver Transmit or receive on LAN HW NIC Network interface card 15 16 Names and Addresses Socket •Host name • Socket abstraction o like a post office name; e.g., www.cs.princeton.edu o An end-point of network connection Treat like a file descriptor • Host address o o like a zip code; e.g., 128.112.92.191 • Conceptually like a telephone Connect to the end of a phone plug • Port number o o You can speak to it and listen to it o like a mailbox; e.g., 0-64k NetworkNetwork 17 18 Steps for Client and Server Creating A Socket (Install A Phone) Client Server • Creating a socket • Create a socket with the socket() • Create a socket with the socket() #include <sys/types.h> system call system call #include <sys/socket.h> • Connect the socket to the • Bind the socket to an address int socket(int domain, int type, int protocol) address of the server using the using the bind() system call. For – Domain: PF_INET (Internet), PF_UNIX (local) connect() system call a server socket on the Internet, an address consists of a port – Type: SOCK_STREAM, SOCK_DGRAM, SOCK_RAW • Send and receive data, using number on the host machine. – Protocol: 0 usually for IP (see /etc/protocols for details) write() and read() system calls or send() and recv() system calls • Listen for connections with the • Like installing a phone listen() system call o Need to what services you want • Accept a connection with the – Local or long distance accept() system call. This call – Voice or data typically blocks until a client – Which company do you want to use connects with the server. • Send and receive data 19 20 Connecting To A Socket Binding A Socket • Active open a socket (like dialing a phone number) • Need to give the created socket an address to listen to int connect(int socket, (like getting a phone number) struct sockaddr *addr, int addr_len) int bind(int socket, struct sockaddr *addr, int addr_len) – Passive open on a server 21 22 Specifying Queued Connections Accepting A Socket • Queue connection requests (like “call waiting”) • Wait for a call to a socket (picking up a phone when it rings) int listen(int socket, int backlog) int accept(int socket, – Set up the maximum number of requests that will be queued struct sockaddr *addr, before being denied (usually the max is 5) int addr_len) – Return a socket which is connected to the caller – Typically blocks until the client connects to the socket 23 24 Sending and Receiving Data Close A Socket • Sending a message • Done with a socket (like hanging up the phone) int send(int socket, char *buf, int blen, int flags) • Receiving a message close(int socket) int recv(int socket, char *buf, int blen, int flags) • Treat it just like a file descriptor 25 26 Summary •Pipes o Process communication on the same machine o Connecting processes with stdin and stdout • Messages o Process communication across machines o Socket is a common communication channels o They are built on top of basic communication mechanisms 27.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages7 Page
-
File Size-