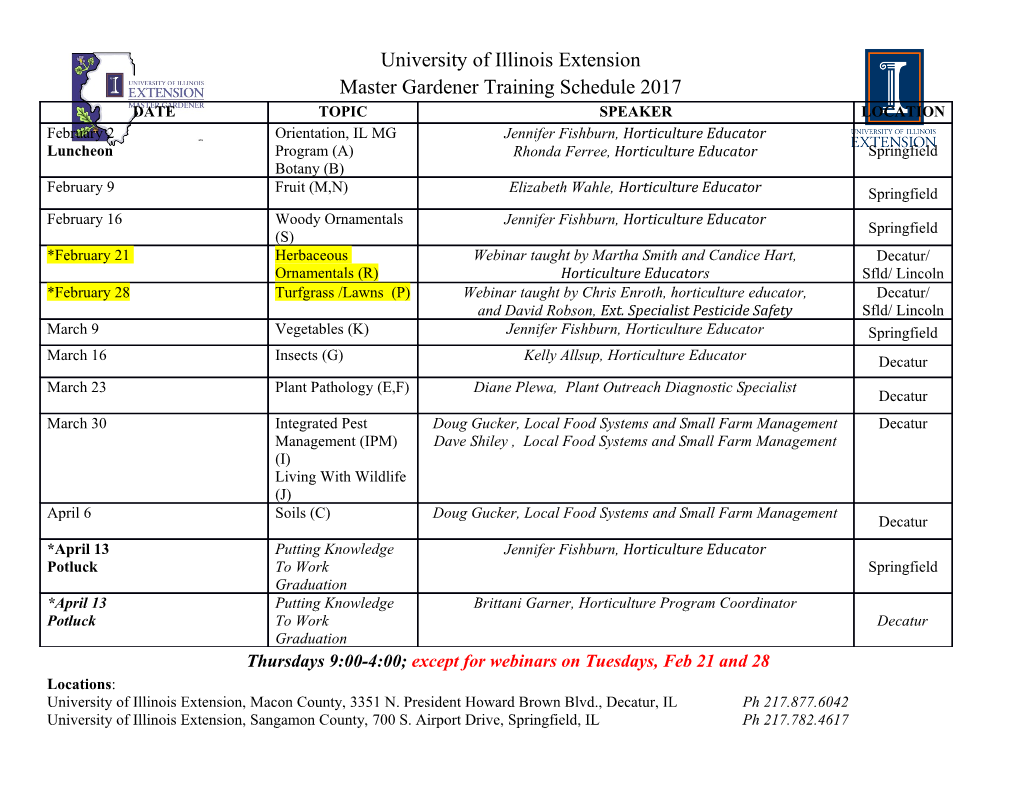
The C++ Programming Inheritance Preview Language Inheritance Example: Ada-style Vectors Dynamic Binding Preview Overloading New-Style Comments A Tour Through C++ Typ e-Safe Linkage Inline Functions Dynamic Memory Management Outline Const Typ e Quali er Stream I/O C++ Overview Bo olean Typ e C++ Design Goals References Major C++ Enhancements Typ e Cast Syntax Other Enhancements Default Parameters Language Features Not Part of C++ Declaration Statements Function Prototyp es Abbreviated Typ e Names C++ Classes User-De ned Conversions Class Vector Example Static Initializ ati on C++ Objects Miscellaneous Di erences C++ Object-Oriented Features 1 C++ Overview C++ Design Goals C++ was designed at AT&T Bell Labs by As with C, run-time eciency is imp ortant Bjarne Stroustrup in the early 80's { e.g., unlike Ada, complicated run-time libraries { The original cfront translated C++ into C for have not traditionally b een required for C++ portability However, this was dicult to debug and p o- Note, that there is no language-sp eci c sup- tentially inecient port for concurrency, p ersistence, or distri- bution in C++ { Many native host machine compilers now exist e.g.,Borland, DEC, GNU, HP, IBM, Microsoft, Compatibility with C libraries and UNIX Sun, Symantec, etc. to ols is emphasized, e.g., { Object co de reuse C++ is a mostly upwardly compatible ex- tension of C that provides: The storage layout of structures is compat- ible with C 1. Stronger typ echecking Supp ort for X-windows, standard ANSI C li- 2. Supp ort for data abstraction brary, UNIX system calls via extern blo ck 3. Supp ort for object-oriented programming { C++ works with the make recompilation utility { C++ supp orts the Object-Oriented paradigm but do es not require it 2 3 Major C++ Enhancements C++ Design Goals cont'd 1. C++ supp orts object-oriented program- ming features \As close to C as p ossible, but no closer" e.g., single and multiple inheritance, abstract { i.e., C++ is not a prop er sup erset of C, so that base classes, and virtual functions backwards compatibility is not entirely main- tained Typically not a problem in practice:: : 2. C++ facilitates data abstraction and en- capsulation that hides representations b e- hind abstract interfaces Note, certain C++ design goals con ict e.g., the class mechanism and parameterized with mo dern techniques for: typ es 1. Compiler optimization 3. C++ provides enhanced error handling ca- { e.g., p ointers to arbitrary memory lo cations pabilities complicate register allo cation and garbage collection e.g., exception handling 2. Software engineering 4. C++ provides a means for identifying an { e.g., separate compilation complicates inlin- object's typ e at runtime ing due to diculty of interpro cedural anal- ysis e.g., Run-Time Typ e Identi cation RTTI 4 5 Other Enhancements Other Enhancements cont'd C++ enforces typ e checking via function prototyp es References provide \call-by-reference" pa- rameter passing Allows several di erent commenting styles Declare constants with the const typ e qual- Provides typ e-safe linkage i er Provides inline function expansion New mutable typ e quali er Built-in dynamic memory management via New bool b o olean typ e new and delete op erators New typ e-secure extensible I/O interface Default values for function parameters called streams and iostreams Op erator and function overloading 6 7 Other Enhancements cont'd A new set of \function call"-style cast no- Language Features Not Part of tations C++ Variable declarations may o ccur anywhere 1. Concurrency statements may app ear within a blo ck See \Concurrent C" by Nehrain Gehani The name of a struct, class, enum, or union is a typ e name 2. Persistence See Exo dus system and E programming lan- Allows user-de ned conversion op erators guage Static data initial i zer s maybearbitrary ex- 3. Garbage Collection pressions See pap ers in USENIX C++ 1994 C++ provides a namespace control mech- anism for restricting the scop e of classes, functions, and global objects 9 8 Function Prototyp es cont'd Function Prototyp es Prop er prototyp e use detects erroneous parameter passing at compile-time, e.g., C++ supp orts stronger typ e checking via function prototyp es STDC jj de ned cplusplus if de ned { Unlike ANSI-C, C++ requires prototyp es for extern int freop en const char *nm, b oth function declarations and de nitions const char *tp, FILE *s; extern char *gets char *; { Function prototyp es eliminate a class of com- extern int p errorconst char *; mon C errors else /* Original C-style syntax. */ extern int freop en , p error ; e.g., mismatched or misnumb ered parameter extern char *gets ; values and return typ es endif /* de ned STDC */ /* :::*/ int main void f Prototyp es are used for external declara- char buf[80]; tions in header les, e.g., if freop en "./fo o", "r", stdin == 0 extern char *strdup const char *s; p error "freop en", exit 1; extern int strcmp const char *s, const char *t; FILE *fop en const char * lename, const char *typ e; while gets buf != 0 extern void print error msg and die const char *msg; /* :::*/; g 11 10 Overloading Function Prototyp es cont'd Two or more functions or op erators may The preceeding program fails mysteriously b e given the same name provided the typ e if the actual calls are: signature for each function is unique: 1. Unique argument typ es: /* Extra argument, also out-of-order! */ freop en stdin, "new le", 10, 'C'; double square double; Complex &square Complex &; /* Omitted arguments. */ 2. Unique numb er of arguments: freop en "new le", "r"; void move int; void move int, int; A \Classic C" compiler would generally not detect erroneous parameter passing at A function's return typ e is not considered compile time though lint would when distinguishing b etween overloaded in- stances { Note, C++ lint utilities are not widely avail- able, but running GNU g++ -Wall provides { e.g., the following declarations are ambiguous similartyp echecking facilities to the C++ compiler: extern double op erator / Complex &, Complex &; extern Complex op erator / Complex &, Complex &; Function prototyp es are used in b oth def- initions and declarations Note, overloading is really just \syntactic { Note, the function prototyp es must b e consis- tent!!! sugar!" 12 13 C++ Classes C++ Classes cont'd The class is the basic protection and data abstraction unit in C++ For eciency and C compatibility reasons, C++ has two typ e systems { i.e., rather than \p er-object" protection 1. One for built-in typ es, e.g., int, oat, char, double, etc. The class mechanism facilitates the cre- ation of user-de ned Abstract Data Typ es 2. One for user-de ned typ es, e.g., classes, structs, ADTs unions, enums etc. { A class declarator de nes a typ e comprised of data memb ers,aswell as metho d op erators Note that constructors, overloading, in- Data memb ers may b e b oth built-in and user- heritance, and dynamic binding only apply de ned to user-de ned typ es { This minimizes surprises, but is rather cumb er- { Classes are \co okie cutters" used to de ne ob- some to do cument and explain:: : jects a.k.a. instances 15 14 C++ Classes cont'd C++ Classes cont'd General characteristics of C++ classes: A class is a \typ e constructor" { Any numb er of class objects may b e de ned { e.g., in contrast to an Ada package oraMod- ula 2 mo dule i.e., objects, which have identity, state, and b ehavior Note, these are not typ es, they are \encap- sulation units" { Class objects may b e dynamically allo cated and deallo cated { Until recently, C++ did not have a higher-level mo dularization mechanism:: : { Passing class objects, p ointers to class objects, and references to class objects as parameters This was a problem for large systems, due to functions are legal to lack of library management facilities and visibility controls { Vectors of class objects may b e de ned { Recent versions of the ANSI C++ draft stan- dard include mechanisms that addresses names- pace control and visibility/scoping, e.g., A class serves a similar purp ose to a C struct Name spaces { However, it is extended to allow user-de ned Nested classes b ehavior, as well 17 16 Class Vector Example There are several signi cant limitati ons with Class Vector Example cont'd built-in C and C++ arrays, e.g., 1. The size must be a compile-time constant, We can write a C++ class to overcome e.g., some of these limitati ons, i.e., void fo o int i { 1 compile-time constant size f int a[100],b[100];//OK int c[i]; // Error! { 4 lack of range checking g 2. Array size cannot vary at run-time Later on we'll use inheritance to nish the 3. Legal array b ounds run from 0 to size 1 job, i.e., { 2 resizing 4. No range checking p erformed at run-time, e.g., f { 3 non-zero lower b ounds int a[10],i; for i = 0; i <= 10; i++ { 5 array assignment a[i]= 0; g 5. Cannot p erform full array assignments, e.g., a = b; // Error! 19 18 Class Vector Example cont'd Class Vector Example cont'd /* File Vector.h this ADT is incomplete wrt initial i zati on and assignment:::! */ /* File Vector.C */ if !de ned VECTOR H // Wrapp er section de ne VECTOR H include "Vector.h" t i, T item f bool Vector::set size typ edef int T; if this->in range i f class Vector f [i]= item; this->buf public: return true; t len = 100 f Vector size g this->size = len; else = new T[len]; this->buf return false; g g ~Vectorvoid f delete [] this->buf ; g t size void const f return this->size ; g size bool set size t i, T item; t i, T &item const f bool Vector::get size bool
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages23 Page
-
File Size-