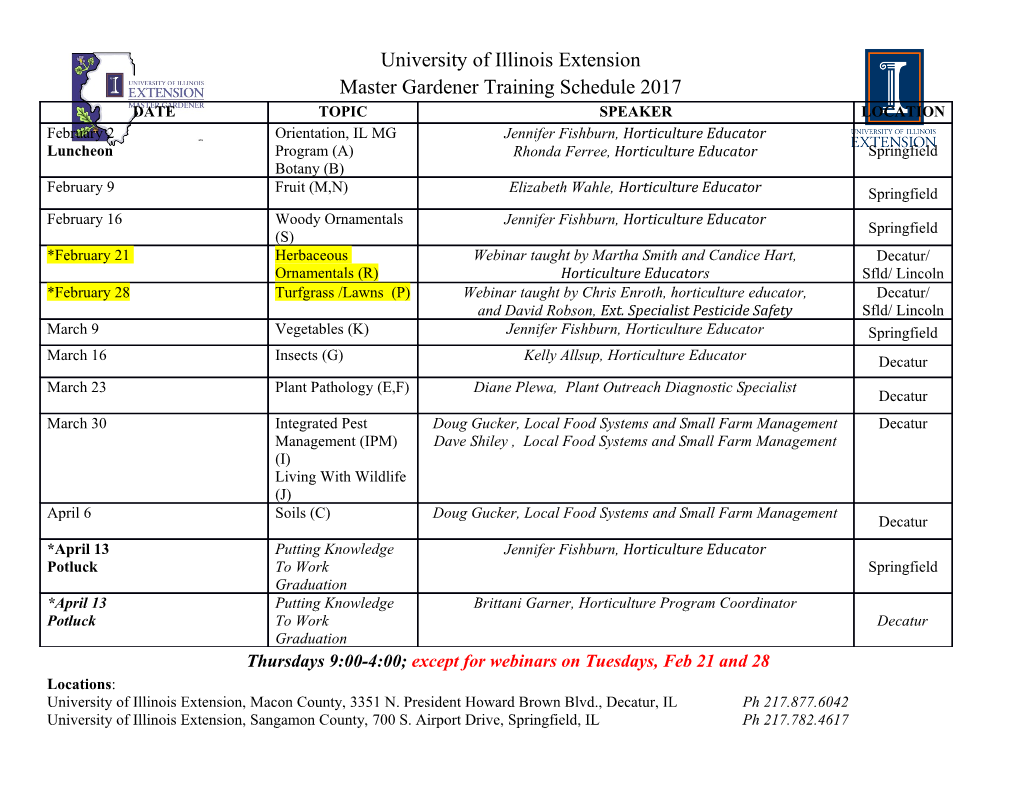
The Racket Guide Version 8.2.0.8 Matthew Flatt, Robert Bruce Findler, and PLT September 25, 2021 This guide is intended for programmers who are new to Racket or new to some part of Racket. It assumes programming experience, so if you are new to programming, consider instead reading How to Design Programs. If you want an especially quick introduction to Racket, start with Quick: An Introduction to Racket with Pictures. Chapter 2 provides a brief introduction to Racket. From Chapter 3 on, this guide dives into details—covering much of the Racket toolbox, but leaving precise details to The Racket Reference and other reference manuals. The source of this manual is available on GitHub. 1 Contents 1 Welcome to Racket 16 1.1 Interacting with Racket . 17 1.2 Definitions and Interactions . 17 1.3 Creating Executables . 18 1.4 A Note to Readers with Lisp/Scheme Experience . 19 2 Racket Essentials 20 2.1 Simple Values . 20 2.2 Simple Definitions and Expressions . 21 2.2.1 Definitions . 21 2.2.2 An Aside on Indenting Code . 22 2.2.3 Identifiers . 23 2.2.4 Function Calls (Procedure Applications) . 23 2.2.5 Conditionals with if, and, or, and cond .............. 25 2.2.6 Function Calls, Again . 28 2.2.7 Anonymous Functions with lambda ................. 28 2.2.8 Local Binding with define, let, and let* ............. 30 2.3 Lists, Iteration, and Recursion . 32 2.3.1 Predefined List Loops . 33 2.3.2 List Iteration from Scratch . 34 2.3.3 Tail Recursion . 35 2.3.4 Recursion versus Iteration . 37 2.4 Pairs, Lists, and Racket Syntax . 38 2.4.1 Quoting Pairs and Symbols with quote ............... 39 2 2.4.2 Abbreviating quote with ' ...................... 41 2.4.3 Lists and Racket Syntax . 42 3 Built-In Datatypes 44 3.1 Booleans . 44 3.2 Numbers . 44 3.3 Characters . 47 3.4 Strings (Unicode) . 49 3.5 Bytes and Byte Strings . 50 3.6 Symbols . 52 3.7 Keywords . 54 3.8 Pairs and Lists . 55 3.9 Vectors . 58 3.10 Hash Tables . 59 3.11 Boxes . 61 3.12 Void and Undefined . 61 4 Expressions and Definitions 63 4.1 Notation . 63 4.2 Identifiers and Binding . 64 4.3 Function Calls (Procedure Applications) . 65 4.3.1 Evaluation Order and Arity . 66 4.3.2 Keyword Arguments . 66 4.3.3 The apply Function . 67 4.4 Functions (Procedures): lambda ....................... 68 4.4.1 Declaring a Rest Argument . 69 3 4.4.2 Declaring Optional Arguments . 70 4.4.3 Declaring Keyword Arguments . 71 4.4.4 Arity-Sensitive Functions: case-lambda .............. 73 4.5 Definitions: define .............................. 74 4.5.1 Function Shorthand . 74 4.5.2 Curried Function Shorthand . 75 4.5.3 Multiple Values and define-values ................ 76 4.5.4 Internal Definitions . 78 4.6 Local Binding . 79 4.6.1 Parallel Binding: let ......................... 79 4.6.2 Sequential Binding: let* ...................... 80 4.6.3 Recursive Binding: letrec ..................... 81 4.6.4 Named let .............................. 82 4.6.5 Multiple Values: let-values, let*-values, letrec-values .. 83 4.7 Conditionals . 84 4.7.1 Simple Branching: if ........................ 84 4.7.2 Combining Tests: and and or .................... 85 4.7.3 Chaining Tests: cond ......................... 85 4.8 Sequencing . 87 4.8.1 Effects Before: begin ........................ 87 4.8.2 Effects After: begin0 ........................ 88 4.8.3 Effects If...: when and unless .................... 89 4.9 Assignment: set! .............................. 90 4.9.1 Guidelines for Using Assignment . 91 4.9.2 Multiple Values: set!-values ................... 93 4 4.10 Quoting: quote and ’ ............................ 94 4.11 Quasiquoting: quasiquote and ‘ ...................... 96 4.12 Simple Dispatch: case ............................ 98 4.13 Dynamic Binding: parameterize ...................... 99 5 Programmer-Defined Datatypes 103 5.1 Simple Structure Types: struct ....................... 103 5.2 Copying and Update . 104 5.3 Structure Subtypes . 104 5.4 Opaque versus Transparent Structure Types . 105 5.5 Structure Comparisons . 106 5.6 Structure Type Generativity . 107 5.7 Prefab Structure Types . 108 5.8 More Structure Type Options . 110 6 Modules 115 6.1 Module Basics . 115 6.1.1 Organizing Modules . 116 6.1.2 Library Collections . 117 6.1.3 Packages and Collections . 118 6.1.4 Adding Collections . 119 6.2 Module Syntax . 120 6.2.1 The module Form . 120 6.2.2 The #lang Shorthand . 121 6.2.3 Submodules . 122 6.2.4 Main and Test Submodules . 124 5 6.3 Module Paths . 126 6.4 Imports: require ............................... 130 6.5 Exports: provide ............................... 133 6.6 Assignment and Redefinition . 134 6.7 Modules and Macros . 136 6.8 Protected Exports . 137 7 Contracts 139 7.1 Contracts and Boundaries . 139 7.1.1 Contract Violations . 139 7.1.2 Experimenting with Contracts and Modules . 140 7.1.3 Experimenting with Nested Contract Boundaries . 141 7.2 Simple Contracts on Functions . 141 7.2.1 Styles of -> .............................. 142 7.2.2 Using define/contract and -> .................. 143 7.2.3 any and any/c ............................ 143 7.2.4 Rolling Your Own Contracts . 144 7.2.5 Contracts on Higher-order Functions . 147 7.2.6 Contract Messages with “???” . 147 7.2.7 Dissecting a contract error message . 149 7.3 Contracts on Functions in General . 150 7.3.1 Optional Arguments . 150 7.3.2 Rest Arguments . 151 7.3.3 Keyword Arguments . 151 7.3.4 Optional Keyword Arguments . 152 7.3.5 Contracts for case-lambda ..................... 153 6 7.3.6 Argument and Result Dependencies . 154 7.3.7 Checking State Changes . 156 7.3.8 Multiple Result Values . 157 7.3.9 Fixed but Statically Unknown Arities . 159 7.4 Contracts: A Thorough Example . 160 7.5 Contracts on Structures . 166 7.5.1 Guarantees for a Specific Value . 166 7.5.2 Guarantees for All Values . 166 7.5.3 Checking Properties of Data Structures . 168 7.6 Abstract Contracts using #:exists and #:D ................. 170 7.7 Additional Examples . 171 7.7.1 A Customer-Manager Component . 172 7.7.2 A Parameteric (Simple) Stack . 174 7.7.3 A Dictionary . 177 7.7.4 A Queue . 179 7.8 Building New Contracts . 181 7.8.1 Contract Struct Properties . 186 7.8.2 With all the Bells and Whistles . 188 7.9 Gotchas . 193 7.9.1 Contracts and eq? .......................... 193 7.9.2 Contract boundaries and define/contract ............ 193 7.9.3 Exists Contracts and Predicates . 195 7.9.4 Defining Recursive Contracts . 195 7.9.5 Mixing set! and contract-out .................. 196 8 Input and Output 197 7 8.1 Varieties of Ports . 197 8.2 Default Ports . 199 8.3 Reading and Writing Racket Data . 200 8.4 Datatypes and Serialization . ..
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages401 Page
-
File Size-