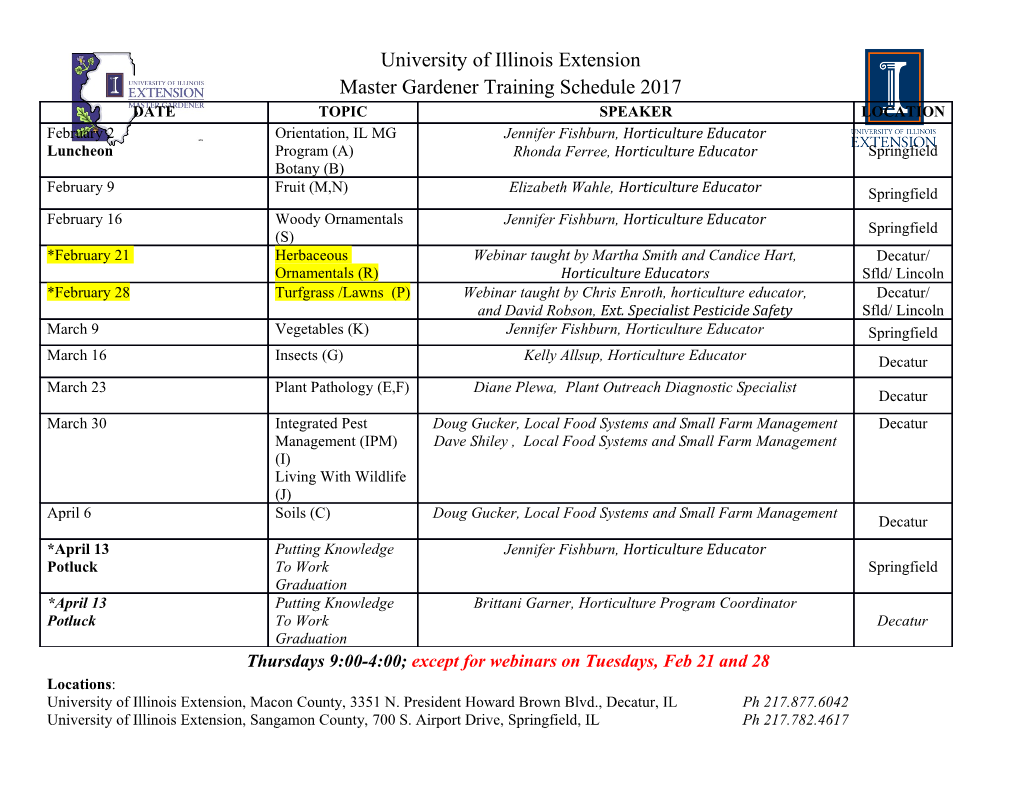
Computational Geometry Lecture Notes1 HS 2012 Bernd Gärtner <[email protected]> Michael Hoffmann <[email protected]> Tuesday 15th January, 2013 1Some parts are based on material provided by Gabriel Nivasch and Emo Welzl. We also thank Tobias Christ, Anna Gundert, and May Szedlák for pointing out errors in preceding versions. Contents 1 Fundamentals 7 1.1 ModelsofComputation ............................ 7 1.2 BasicGeometricObjects. 8 2 Polygons 11 2.1 ClassesofPolygons............................... 11 2.2 PolygonTriangulation . 15 2.3 TheArtGalleryProblem ........................... 19 3 Convex Hull 23 3.1 Convexity.................................... 24 3.2 PlanarConvexHull .............................. 27 3.3 Trivialalgorithms ............................... 29 3.4 Jarvis’Wrap .................................. 29 3.5 GrahamScan(SuccessiveLocalRepair) . .... 31 3.6 LowerBound .................................. 33 3.7 Chan’sAlgorithm................................ 33 4 Line Sweep 37 4.1 IntervalIntersections . ... 38 4.2 SegmentIntersections . 38 4.3 Improvements.................................. 42 4.4 Algebraic degree of geometric primitives . ....... 42 4.5 Red-BlueIntersections . .. 45 5 Plane Graphs and the DCEL 51 5.1 TheEulerFormula............................... 52 5.2 TheDoubly-ConnectedEdgeList. .. 53 5.2.1 ManipulatingaDCEL . 54 5.2.2 GraphswithUnboundedEdges . 57 5.2.3 Remarks................................. 58 3 Contents CG 2012 6 Delaunay Triangulations 61 6.1 TheEmptyCircleProperty . 64 6.2 TheLawsonFlipalgorithm . 66 6.3 Termination of the Lawson Flip Algorithm: The Lifting Map ....... 67 6.4 CorrectnessoftheLawsonFlip Algorithm . ..... 68 6.5 TheDelaunayGraph.............................. 70 6.6 Every Delaunay Triangulation Maximizes the Smallest Angle ....... 72 6.7 ConstrainedTriangulations . ... 75 7 Delaunay Triangulation: Incremental Construction 79 7.1 Incrementalconstruction. ... 79 7.2 TheHistoryGraph............................... 82 7.3 Thestructuralchange ............................. 83 8 The Configuration Space Framework 85 8.1 The Delaunay triangulation — an abstract view. ...... 85 8.2 ConfigurationSpaces. .. .. .. .. .. .. ... .. .. .. .. .. .. .. 86 8.3 Expectedstructuralchange . .. 87 8.4 Bounding location costs by conflict counting. ....... 89 8.5 Expectednumberofconflicts . 90 9 Trapezoidal Maps 95 9.1 TheTrapezoidalMap ............................. 95 9.2 Applicationsoftrapezoidalmaps . .... 96 9.3 Incremental Construction of the Trapezoidal Map . ........ 96 9.4 Usingtrapezoidalmapsfor pointlocation . ...... 98 9.5 Analysis of the incremental construction . ....... 99 9.5.1 DefiningTheRightConfigurations . 99 9.5.2 UpdateCost .............................. 102 9.5.3 TheHistoryGraph. 103 9.5.4 Cost of the Find step..........................104 9.5.5 ApplyingtheGeneralBounds. 104 9.6 Analysisofthepointlocation . 106 9.7 Thetrapezoidalmapofasimplepolygon . 108 10 Voronoi Diagrams 115 10.1PostOfficeProblem .............................. 115 10.2VoronoiDiagram ................................ 116 10.3Duality ..................................... 119 10.4LiftingMap................................... 120 10.5 PointlocationinaVoronoiDiagram . 121 10.5.1 Kirkpatrick’sHierarchy . 122 4 CG 2012 Contents 11 Linear Programming 129 11.1 LinearSeparabilityofPointSets . .. .. 129 11.2 LinearProgramming . .. .. .. .. .. .. ... .. .. .. .. .. .. .. 130 11.3 Minimum-areaEnclosingAnnulus . 133 11.4 SolvingaLinearProgram . 135 12 A randomized Algorithm for Linear Programming 137 12.1Helly’sTheorem ................................ 138 12.2 Convexity,oncemore. 139 12.3TheAlgorithm ................................. 140 12.4 RuntimeAnalysis................................ 141 12.4.1 ViolationTests .. .. .. .. .. .. ... .. .. .. .. .. .. .. 142 12.4.2 BasisComputations . 143 12.4.3 TheOverallBound. 143 13 Line Arrangements 145 13.1Arrangements.................................. 146 13.2Construction .................................. 147 13.3ZoneTheorem ................................. 147 13.4 ThePowerofDuality ............................. 149 13.5 SortingallAngularSequences. 150 13.6 SegmentEndpointVisibilityGraphs . .. .. 151 13.7 HamSandwichTheorem. 153 13.83-Sum ......................................155 13.93-Sumhardness................................. 156 14 Davenport-Schinzel Sequences 161 14.1 Davenport-SchinzelSequences . .. .. 162 15 Epsilon Nets 167 15.1Motivation ................................... 167 15.2 Range spaces and ε-nets............................. 168 15.2.1 Examples ................................ 168 15.2.2 Nopointinalargerange. 169 15.2.3 Smallestenclosingballs. 170 15.3 Either almost all is needed or a constant suffices. ........ 170 15.4 What makes the difference: VC-dimension . .. .. 171 15.4.1 The size of projections for finite VC-dimension. ....... 172 15.5 VC-dimension of Geometric RangeSpaces . .. .. 174 15.5.1 Halfspaces. ............................... 174 15.5.2 Balls. .................................. 175 15.6 Small ε-Nets,anEasyWarm-upVersion . 176 15.6.1 Smallestenclosingballs,again . 177 5 Contents CG 2012 15.7 Even Smaller ε-Nets ..............................178 6 Chapter 1 Fundamentals 1.1 Models of Computation Real RAM Model. A memory cell stores a real number. Any single arithmetic operation or comparison can be computed in constant time. In addition, sometimes also roots, logarithms, other analytic functions, indirect addressing (integral), or floor and ceiling are used. This is a quite powerful (and somewhat unrealistic) model of computation, as a single real number in principle can encode an arbitrary amount of information. Therefore we have to ensure that we do not abuse the power of this model. Algebraic Computation Trees (Ben-Or [1]). A computation is regarded as a binary tree. ¯ The leaves contain the (possible) results of the compu- tation. a − b ¯ Every node v with one child has an operation of the form +, −, , /, √,... associated to it. The operands of ≤ 0 this operation∗ are constant input values, or among the ancestors of v in the tree. a − c b − c ¯ Every node v with two children has associated to it a branching of the form > 0, > 0, or = 0. The branch ≤ 0 ≤ 0 is with respect to the result of v’s parent node. If the expression yields true, the computation continues with a c b c the left child of v; otherwise, it continues with the right child of v. If every branch is based on a linear function in the input values, we face a linear computation tree. Analogously one can define, say, quadratic computation trees. The term decision tree is used if all of the results are either true or false. 7 Chapter 1. Fundamentals CG 2012 The complexity of a computation or decision tree is the maximum number of vertices along any root-to-leaf path. It is well known that Ω(n log n) comparisons are required to sort n numbers. But also for some problems that appear easier than sorting at first glance, the same lower bound holds. Consider, for instance, the following problem. Element Uniqueness Input: {x1,..., x } R, n N. n ⊂ ∈ Output: Is x = x , for some i, j {1,..., n} with i = j? i j ∈ 6 Ben-Or [1] has shown that any algebraic decision tree to solve Element Uniqueness for n elements has complexity Ω(n log n). 1.2 Basic Geometric Objects We will mostly be concerned with the d-dimensional Euclidean space Rd, for small d N; typically, d = 2 or d = 3. The basic objects of interest in Rd are the following. ∈ Points. A point p, typically described by its d Cartesian r = (7, 1) p = (−4, 0) coordinates p =(x1,..., xd). q = (2, −2) Directions. A vector v Sd−1 (the (d − 1)-dimensional ∈ unit sphere), typically described by its d Cartesian coor- d 2 dinates v =(x1,..., xd), with ||v|| = i=1 xi = 1. qP Lines. A line is a one-dimensional affine subspace. It can p be described by two distinct points p and q as the set of all points r that satisfy r = p + λ(q − p), for some λ R. q ∈ While any pair of distinct points defines a unique line, a line in R2 contains infinitely many points and so it may happen that a collection of three or more points lie on a line. Such a collection of points is termed collinear 1. Rays. If we remove a single point from a line and take the closure of one of the connected components, then we obtain a ray. It can be described by two distinct points p and q as the set of all points r that satisfy r = p+λ(q−p), q for some λ > 0. The orientation of a ray is the direction p (q − p)/ q − p . k k 1Not colinear, which refers to a notion in the theory of coalgebras. 8 CG 2012 1.2. Basic Geometric Objects Line segment. A line segment is the intersection of two collinear rays of opposite orientation. It can be described by two points p and q as the set of all points r that satisfy q r = p + λ(q − p), for some λ [0,1]. We will denote ∈ the line segment through p and q by pq. Depending p on the context we may allow or disallow degenerate line segments consisting of a single point only (p = q in the above equation). Hyperplanes. A hyperplane H is a (d−1)-dimensional affine subspace. It can be described algebraically by d + 1 coefficients λ1,..., λd+1 R, where (λ1,..., λd+1) = 1, as the ∈ k d k set of all points (x1,..., xd) that satisfy the linear equation H : i=1 λixi = λd+1. If the above equation is converted into an inequality, we obtain the algebraic descrip- tion of a halfspace (in R2: halfplane). P Spheres and balls. A sphere is the set of all points that are equidistant to a fixed point. It can be described by a point c (center) and a number ρ R (radius) as the set of all
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages182 Page
-
File Size-