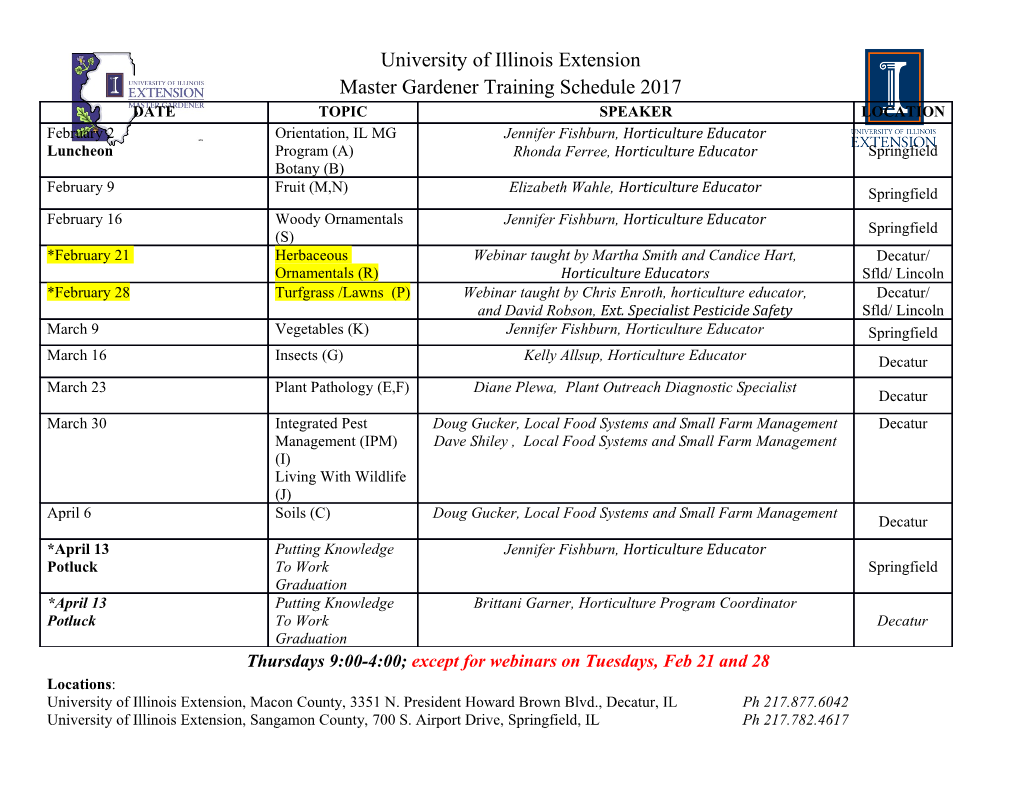
Plan • Introduce lambda calculus – Syntax Lambda Calculus – Substitution – Operational Semantics (… with contexts!) – Evaluation strategies – Equality • Relationship to programming languages (next time) • Study of types and type systems (later) #1 #2 Lambda Background Lambda Celebrity Representative • Developed in 1930’s by Alonzo Church • Subsequently studied by many people (still studied • Milton Friedman? today!) • Morgan Freeman? • Considered the “testbed” for procedural and functional languages • C. S. Friedman? – Simple – Powerful – Easy to extend with features of interest – Plays similar role for PL research as Turing machines do for computability and complexity – Somewhat like a crowbar … “Whatever the next 700 languages turn out to be, they will surely be variants of lambda calculus. ” (Landin ’66) #3 #4 Gordon Freeman Lambda Syntax • Best-selling PC FPS to date • The λ-calculus has three kinds of expressions (terms) e ::= x Variables | λx.e Functions (abstraction) | e1 e2 Application • λx.e is a one-argument function with body e • e1 e2 is a function application • Application associates to the left x y z means (x y) z • Abstraction extends to the right as far as possible λx.x λy.x y z means λx.(x (λy. ((x y) z))) #5 #6 1 Why Should I Care? • A language with 3 expressions? Woof! Examples of Lambda Expressions • Li and Zdancewick. Downgrading policies and relaxed noninterference . POPL ’05 • The identity function: – Just one example of a recent PL/security paper … I = def λx. x • A function that given an argument y discards it and yields the identity function: λy. ( λx. x) • A function that given a function f invokes it on the identity “There goes function our grant λf. f ( λx. x) money.” #7 #8 Scope of Variables Free and Bound Variables • As in all languages with variables it is • A variable is said to be free in E if it has important to discuss the notion of scope occurrences that are not bound in E – The scope of an identifier is the portion of a • We can define the free variables of an program where the identifier is accessible expression E recursively as follows: Free( x) = { x } • An abstraction λx. E binds variable x in E Free( E1 E2) = Free( E1) ∪ Free( E2) – x is the newly introduced variable Free( λx. E ) = Free( E) - { x } –E is the scope of x (unless x is shadowed …) • Example: Free( λx. x ( λy. x y z) ) = { z } – We say x is bound in λx. E • Free variables are (implicitly or explicitly) – Just like formal function arguments are bound in declared outside the term the function body #9 #10 Free Your Mind! Renaming Bound Variables • Just like in any language with statically nested • λ-terms that can be obtained from one another scoping we have to worry about variable shadowing by renaming of the bound variables are – An occurrence of a variable might refer to different considered identical. things in different contexts • This is called α-equivalence . • Renaming bound vars is called α-renaming . • e.g., IMP with locals: let x = E in x + (let x = E’ in x) + x • Example: λx. x is identical to λy. y and to λz. z • Intuition: – By changing the name of a formal argument and of all its occurrences in the function body, the behavior of • In λ-calculus: λx. x ( λx. x) x the function does not change – In λ-calculus such functions are considered identical #11 #12 2 Make It Easy On Yourself Substitution • Convention: we will always try to rename bound variables so that they are all unique • The substitution of E’ for x in E (written [E’/x]E ) – e.g., write λx. x ( λy.y) x instead of λx. x ( λx.x) x – Step 1. Rename bound variables in E and E’ so they are unique • This makes it easy to see the scope of – Step 2. Perform the textual substitution of E’ for x in E bindings and also prevents confusion! • Called capture-avoiding substitution . • Example: [y ( λx. x) / x] λy. ( λx. x) y x – After renaming: [y ( λv. v)/x] λz. ( λu. u) z x – After substitution: λz. ( λu. u) z (y ( λv. v)) • If we are not careful with scopes we might get: λy. ( λx. x) y (y ( λx. x)) #13 #14 The deBruijn Notation Combinators • An alternative syntax that avoids naming of bound • A λ-term without free variables is closed or a variables (and the subsequent confusions) combinator • The deBruijn index of a variable occurrence is the • Some interesting combinators: number of lambdas that separate the occurrence from its binding lambda in the abstract syntax tree I = λx. x • The deBruijn notation replaces names of K = λx. λy.x occurrences with their deBuijn index S = λf. λg. λx.f x (g x) • Examples: D = λx. x x – λx.x λ.0 Identical terms Y = λf.( λx. f (x x)) ( λx. f (x x)) – λx. λx.x λ.λ.0 have identical • Theorem: Any closed term is equivalent to one – λx. λy.y λ.λ.0 representations ! written with just S, K, I –(λ x. x x) ( λ z. z z) (λ.0 0) ( λ.0 0) – Example: D = β S I I – λx. ( λx. λy.x) x λ.( λ.λ.1) 0 – (we’ll discuss this form of equivalence in a bit) #15 #16 Informal Semantics Operational Semantics • We consider only closed terms • Many operational semantics for the λ-calculus • All are based on the equation • The evaluation of (λx. e) e’ =β [e’/x]e (λx. e) e’ usually read from left to right 1. Binds x to e’ • This is called the β-rule and the evaluation step a β-reduction 2. Evaluates e with the new binding • The subterm (λx. e) e’ is a β-redex 3. Yields the result of this evaluation • We write e →β e’ to say that e β-reduces to e’ in • Like a function call, or like “let x = e’ in e ” one step * • We write e →β e’ to say that e β-reduces to e’ in 0 • Example: or more steps (λf. f (f e)) g evaluates to g (g e) – Should remind you of small-step opsem term-rewriting #17 #18 3 Examples of Evaluation Evaluation and the Static Scope • The identity function: • The definition of substitution guarantees that (λx. x) E → [E / x] x = E evaluation respects static scoping: • Another example with the identity: (λ x. ( λy. y x)) ( y (λx. x)) →β λz. z ( y (λv. v)) (λf. f ( λx. x)) ( λx. x) → [λx. x / f] f ( λx. x)) = [( λx. x) / f] f ( λy. y)) = (λx. x) ( λy. y) → (y remains free, i.e., defined externally) [λy. y /x] x = λy. y • If we forget to rename the bound y: ∗ • A non-terminating evaluation: (λ x. ( λy. y x)) ( y (λx. x)) → β λy. y (y ( λv. v)) (λx. xx)( λy. yy) → [λy. yy / x]xx = (λy. yy)( λy. yy) → … • Try T T , where T = λx.x x x (y was free before but is bound now) #19 #20 Another View of Reduction Normal Forms • The application APP • A term without redexes is in normal form λx. • A reduction sequence stops at a normal form e e’ * x x x • If e is in normal form and e → β e’ then e is • becomes: identical to e’ e Terms can “grow” •K = λx. λy. x is in normal form substantially through e’ e’ e’ β-reduction ! • K I is not in normal form #21 #22 Nondeterministic Evaluation Lambda Calculus Contexts • We define a small-step reduction relation • Define contexts with one hole (λx. e) e’ → [e’/x]e H ::= • | λx. H | H e | e H • Write H[e] to denote the filling of the hole in e → e ’ e → e ’ 1 1 2 2 H with the expression e → e1 e2 → e1’ e2 e1 e2 e1 e2’ e → e’ • Example: λx. e → λx. e’ H = λx. x • H[ λy.y] = λx. x ( λy. y) • Filling the hole allows variable capture! • This is a non-deterministic semantics H = λx. x • H[x] = λx.x x • Note that we evaluate under λ (where?) #23 #24 4 Contextual Opsem The Order of Evaluation e → e’ • In a λ-term there could be more than one (λx. e) e’ → [e’/x]e H[e] → H[e’] instance of (λx. E) E’, as in: • Contexts allow concise formulations of congruence (λy. (λx. x) y ) E rules (application of local reduction rules on subterms) – could reduce the inner or the outer λ • Reduction occurs at a β-redex that can be – which one should we pick? anywhere inside the expression (λy. ( λx. x) y) E • The latter rule is called a congruence or structural inner outer rule (λy. [y/x] x) E = ( λy. y) E [E/y] ( λx. x) y =( λx. x) E • The above rules do not specify which redex must be reduced first E #25 #26 The Diamond Property The Diamond Property • A relation R has the diamond property if whenever • Languages defined by non-deterministic sets e R e 1 and e R e 2 then there exists e’ such that e1 R of rules are common e’ and e2 R e’ e – Logic programming languages R R – Expert systems – Constraint satisfaction systems e1 e2 • and thus most pointer analyses … R R e’ – Dataflow systems – Makefiles • →β does not have the diamond property • → * has the diamond property • It is useful to know whether such systems β have the diamond property • Also called the confluence property #27 #28 (Beta) Equality The Church-Rosser Theorem → * • Let = be the reflexive, transitive and • If e1 =β e2 then there exists e’ such that e1 β e’ β and e → * e’ 2 β • symmetric closure of →β • * =β is (→β ∪ ←β) • e1 e2 • That is, e1 =β e2 if e1 converts to e2 via a sequence of forward and backward →β •• • • e’ e • e2 • Proof (informal): apply the diamond property as 1 many times as necessary #29 #30 5 Corollaries Evaluation Strategies • If e1 =β e2 and e1 and e2 are normal forms • Church-Rosser theorem says that independent of the reduction strategy we will find 1 normal form then e1 is identical to e2 * * • But some reduction strategies might find 0 – From C-R we have ∃e’.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages6 Page
-
File Size-