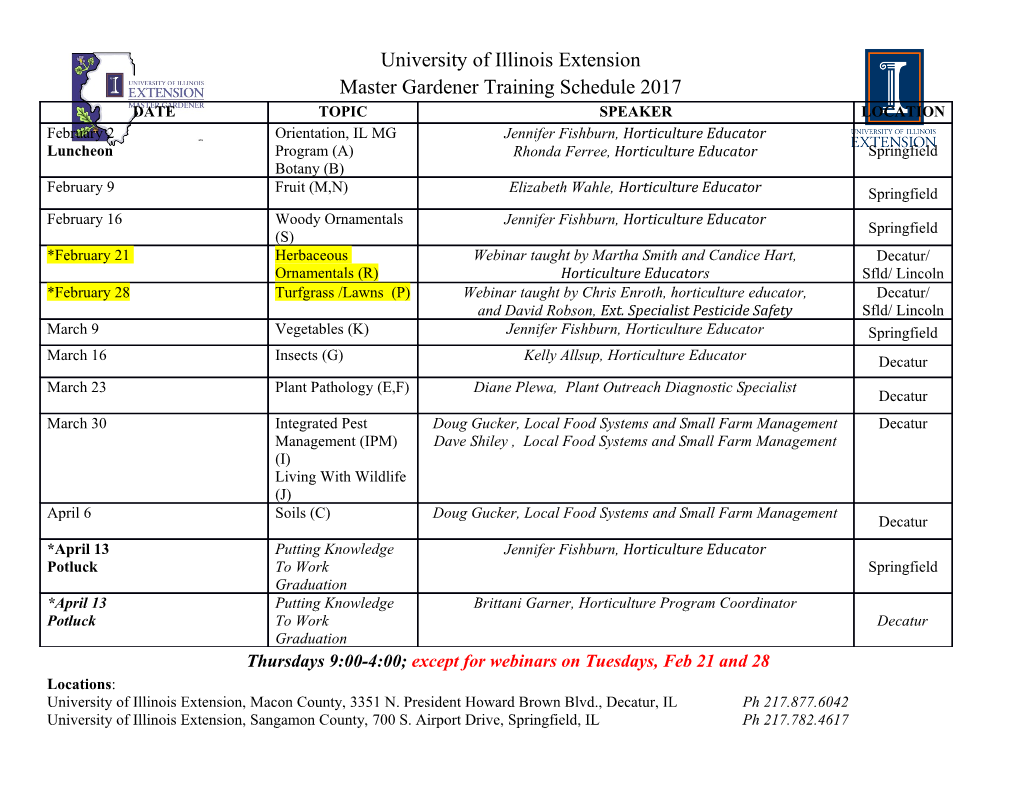
The Java Modeling Language Software Fiável Mestrado em Engenharia Informática Mestrado em Informática Faculdade de Ciências da Universidade de Lisboa 2015/2016 Vasco T. Vasconcelos, Antónia Lopes Software Fiável The Java Modeling Language Contracts I The key ingredient of Design by contract, software design methodology promoted by Bertrand Meyer and the Eiffel programming language in 1986. I Inspired by assertions of Hoare logic, contracts place special obligations on both clients and suppliers of a procedure or method. I What does a method require from it’s caller? I What does the method ensure? Software Fiável The Java Modeling Language DBC DBC key idea is to associate a specification with every software element. These specifications (or contracts) govern the interaction of the element with the rest of the world. I Forces the programmer to write exactly what the method does and needs I No need to program defensively (defensive checks can be exensive and increases the complexity of the code of the method and worsen its maintainability) I Assigns blame: specifies who is to blame for not keeping the contract I Provides a standard way to document classes: client programmers are provided with a proper description of the interface properties of a class that is stripped of all implementation information but retains the essential usage information: the contract. Software Fiável The Java Modeling Language Language support for Contracts Language support for contracts exists in different forms: I Eiffel is an object-oriented language that includes contracts I JML adds contracts to the Java language. JML specifications are conventional Java boolean expressions, with a few extensions I Spec# adds contracts to C# I Code Contracts is an API (part of .NET) to author contracts In this course we focus on JML. Software Fiável The Java Modeling Language JML Contracts by example /∗@ @ r e q u i r e s n >= 0 ; @ ensures \ r e s u l t ∗ \ r e s u l t <= n ; @ ensures n < (\ r e s u l t + 1) ∗ (\ r e s u l t + 1 ) ; @∗/ i n t i n t S q r t ( i n t n ) requires says what must be true to call intSqrt (); specifies the client’s obligation ensures says what must be true when intSqrt () terminates; specifies the implementer’s obligations Software Fiável The Java Modeling Language Overview Flavor Overview Flavor ManyJML: Many Tools, tools, One one language Language How Tools Complement Each Other Different strengths: Runtime checking — real errors. Static checking — better coverage. Warnings JML Annotated Java Verification — guarantees. ESC/Java2 public class ArrayOps { Usual ordering: jmldoc private /*@ spec_public @*/ Object[] a; //@ public invariant 0 < a.length; 1 Runtime checker (jmlc and jmlunit). Web pages /*@ requires 0 < arr.length; @ ensures this.a == arr; Daikon 2 @*/ Extended Static Checking (ESC/Java2). jmlunit public void init(Object[] arr) { this.a = arr; Data trace file 3 } Verification tool (e.g., KeY, JACK, Jive). Unit tests } jmlc Bogor JACK, Jive, Krakatoa, KeY, LOOP Class file Model checking Correctness proof XVP [Picture by G. Leavens] Software Fiável The Java Modeling Language Gary T. Leavens (ISU UCF) JML Tutorial CAV 2007 18 / 225 Gary T. Leavens (ISU UCF) JML Tutorial CAV 2007 19 / 225 → → Overview Interest Overview Interest Interest in JML Advantages of Working with JML Many tools. Reuse language design. State of the art language. Ease communication with researchers. Large and open research community: Share customers. 23 groups, worldwide. Over 135 papers. Join us! See jmlspecs.org Gary T. Leavens (ISU UCF) JML Tutorial CAV 2007 20 / 225 Gary T. Leavens (ISU UCF) JML Tutorial CAV 2007 21 / 225 → → Runtime Verification I The most obvious way to use JML annotations is to test them at runtime and report any detected violations I jmlrac, a runtime assertion checker, was the first tool for JML, developed together with jmlc, the compiler of Java code annotated with JML I jmlc compiles Java programs annotated with JML specifications into Java bytecode. Compiled bytecode includes instructions that check JML specifications I jmlrac a wrapper for java that knows about the necessary runtime libraries for runtime assertion checking I The fact that JML was developed having runtime assertion checking in mind lead to some design decisions in the language I Slightly different variants of JML were considered by other tools Software Fiável The Java Modeling Language Runtime Verification I When jmlrac is used to run a Java program (compiled with jmlc) I it runs slower and uses more memory I it halts when any JML contract is violated I False negatives are possible only if JML assertions that specify invariants are also used. I But the runtime assertion checker can fail to report assertions that might be false (it is acceptable for the runtime assertion checker to not execute some parts of assertions, especially in postconditions) Software Fiável The Java Modeling Language Runtime Verification I Typically, the runtime assertion checker will not be used in production. I There are several benefits with respect to conventional testing: I more properties are tested, at more points in time and providing better feedback I some test code is generated automatically I less time is needed to think about what to test I assertions are useful for other purposes namely program documentation I unlike documentation written in a natural language, assertions can be mechanically checked to agree with the program text (a common problem with informal documentation) I Runtime verification is limited by the execution paths exercised by the test suite being used and, hence, does not establishes the correctness for all possible execution paths! Software Fiável The Java Modeling Language JML assertions I JML assertions are boolean valued Java expressions inserted in special comments: /∗@ assertion goes here; ∗/ or //@ assertion goes here; I Assertions cannot have side-effects. This both allows assertion checks to be used safely during debugging and supports mathematical reasoning about assertions. I Do not forget the semicolon at the end of the assertion! Software Fiável The Java Modeling Language Multiple clauses I Clauses r e q u i r e s P; r e q u i r e s Q; are equivalent to r e q u i r e s P && Q; I Similarly for ensures and invariant I Runtime checker gives better feedback with multiple clauses Software Fiável The Java Modeling Language Preconditions I The requires clause states the conditions under which the method is defined I It is needed if the method is partial, ie., if the behavior is not defined on some inputs I If the method is total, ie., if the behavior is defined on all (type correct) inputs, then the precondition is true I Clauses of the form requires true can be omitted Software Fiável The Java Modeling Language Postconditions I The ensures clause describes the behavior of the method for all inputs not ruled out by the requires clause I It must define what outputs are produced and also what modifications are made to the inputs I The ensures clause is written under the assumption that the requires clause is satisfied, and says nothing about the method’s behavior when the requires clause is not satisfied I Clauses of the form ensures true can be omitted Software Fiável The Java Modeling Language Extensions to the language of Java expressions Expression Meaning \result method’s return value \old(E) pre-state value of expression E (\ forall T x; P; Q) ^fQ j x 2 T ^ Pg (\exists T x; P; Q) _fQ j x 2 T ^ Pg (\min T x; P; E) minfE j x 2 T ^ Pg (\sum T x; P; E) PfE j x 2 T ^ Pg (\num_of T x; P; Q) Pf1 j x 2 T ^ P ^ Qg P <==> Q P == Q, lower priority than == P ==> Q !P || Q Software Fiável The Java Modeling Language Using forall I Use the second element of the forall to specify the range of the array index. The array is sorted: (\ f o r a l l i n t i; 1< i && i < a.length; a [ i −1] < a [ i ] ) ; I Use the second element of the forall to specify a predicate over the elements of a collection. All elements in a collection c of Strings start with letter A. (\ f o r a l l String s; c.contains(s); s.startsWith("A")); Software Fiável The Java Modeling Language Rights and obligations in contracts /∗@ @ r e q u i r e s n >= 0 ; @ ensures \ r e s u l t ∗ \ r e s u l t <= n ; @ ensures n < (\ r e s u l t + 1) ∗ (\ r e s u l t + 1 ) ; @∗/ i n t i n t S q r t ( i n t n ) Obligation Right Client Provide non-negative Get a square root ap- n proximation of the re- sult Implementer Compute and return a Assume that n is non- square root negative Software Fiável The Java Modeling Language Blame assignment in contracts Assertion violation Blame Precondition The client, the caller (intSqrt () has not even started to run) Postcondition The supplier, the code of intSqrt () (and the methods that it calls) Software Fiável The Java Modeling Language Design by Contract and Hoare logic I The triple below says “if x ≥ 0 and S terminates, then result 2 ≤ x < (result + 1)2 holds” fx ≥ 0g S fresult2 ≤ x < (result + 1)2g I The contract below says “It is an error to call the method intSqrt with x < 0” //@ r e q u i r e s x >= 0 ; i n t i n t S q r t ( i n t x ) Software Fiável The Java Modeling Language Design by Contract and Hoare logic I The triple below says “if x ≥ 0 and S terminates, then result 2 ≤ n < (result + 1)2 holds” fx ≥ 0g S fresult2 ≤ n < (result + 1)2g I The contract below says “If the method intSqrt was called with x ≥ 0 then it is an error to return an integer that does not satisfy result 2 ≤ x < (result + 1)2 ” //@ r e q u i r e s x >= 0 ; //@ ensures \ r e s u l t ∗ \ r e s u l t <= x ; //@ ensures
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages69 Page
-
File Size-