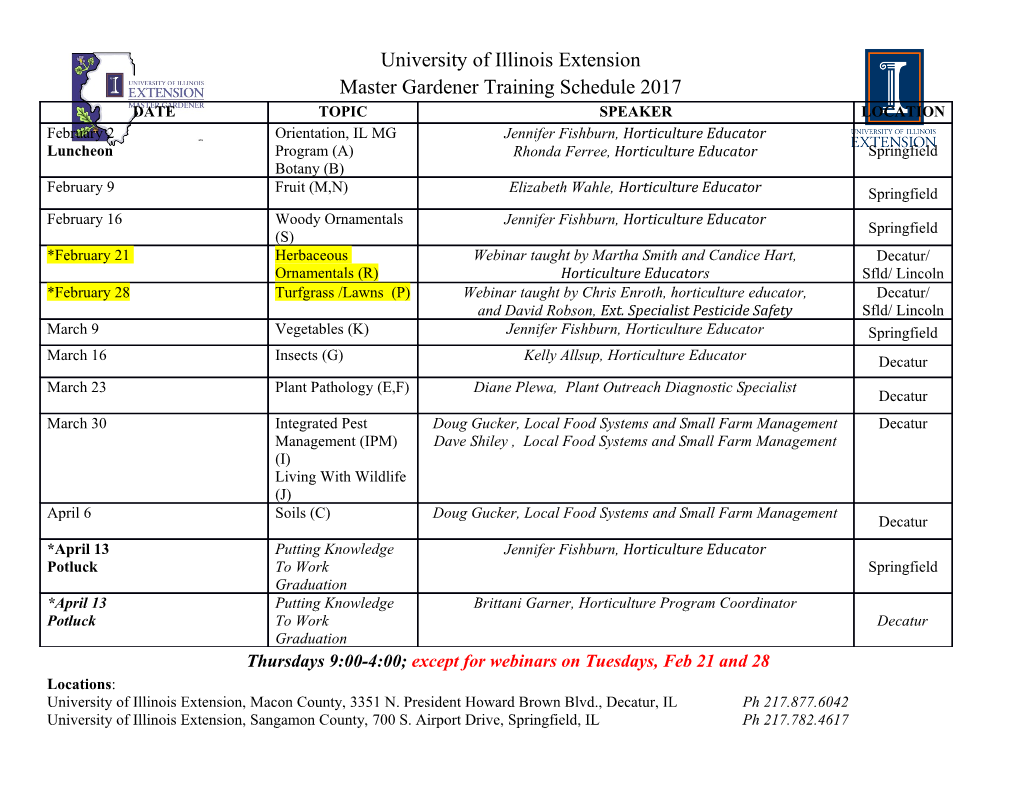
MEAP Edition Manning Early Access Program Haskell in Depth Version 5 Copyright 2019 Manning Publications For more information on this and other Manning titles go to www.manning.com ©Manning Publications Co. We welcome reader comments about anything in the manuscript - other than typos and other simple mistakes. These will be cleaned up during production of the book by copyeditors and proofreaders. https://forums.manning.com/forums/haskell-in-depth welcome Thank you for purchasing the MEAP for Haskell in Depth. I’m excited to see the book reach this stage and look forward to its continued development and eventual release. This is a book for those of you who already know and like Haskell and want to start using Haskell in production. I tried not repeat things that you are aware of or can find in other books that have been published already. Nevertheless, there is always a tradeoff when deciding whether to include material in a book. As this is a work in progress, things may change significantly. We’re releasing the first three chapters with a small technical introduction on how to work with the code examples to start. I truly believe that it is impossible to learn programming at any level by reading. I recommend that you work with the examples on your own, extending or completely rewriting them. I will occasionally suggest possible directions in which to take your work. Chapter 1 is an attempt to give a short overview of the current state of Haskell and programming in Haskell, what its ecosystem consists of. As this is a book in Manning’s in Depth series, there is no need to introduce you to the basic concepts of Haskell programming, but you may not be aware of some of the available options, so it’s worth reviewing the programming style that is supported in Haskell. In Chapter 2, I chose to talk about the concept of writing functional programs with types and type classes, the idea being to get us on the same page when talking about Haskell. Readers with different backgrounds may find new things in this chapter, although most of its material should be well known. Chapter 3 is an extended example. We’ll discuss an implementation of a practical program for analyzing and presenting analysis results for financial information. We’ll extensively use various Haskell packages here that you can use in your own projects, too. Chapters 2 and 3 constitute part 1 of this book, Core Haskell. Looking ahead, part 2 will cover practical needs when developing software projects. We’ll talk about structuring programs via various tools such as modules, packages, monads and monad transformers, error-handling, logging, testing, profiling, benchmarking, etc. Part 3 will cover advanced Haskell features, mainly its type system in its current state and generic metaprogramming. In part 4, we’ll discuss the most prominent Haskell libraries and the concepts they introduce into everyday Haskell programming. We’ll conclude in part 5 with a description of several large Haskell projects, including the Glasgow Haskell Compiler, which is the largest Haskell project in existence (at least in the open source world). ©Manning Publications Co. We welcome reader comments about anything in the manuscript - other than typos and other simple mistakes. These will be cleaned up during production of the book by copyeditors and proofreaders. https://forums.manning.com/forums/haskell-in-depth We expect to have updates to the book every three or four weeks, whether that is a new chapter or an update to an existing one. As you’re reading, I hope you’ll take advantage of the book’s Manning forum. I’ll be reading your comments and responding, and your feedback is very much appreciated in the development process. —VitalyBragilevsky ©Manning Publications Co. We welcome reader comments about anything in the manuscript - other than typos and other simple mistakes. These will be cleaned up during production of the book by copyeditors and proofreaders. https://forums.manning.com/forums/haskell-in-depth brief contents 0 How to work with the source code examples 1 Haskell nowadays PART 1: CORE HASKELL 2 Functional programming with types and type classes 3 Processing stock quote data: An example PART 2: DEVELOPING PROJECTS IN HASKELL 4 Structuring programs with modules and packages 5 Monads as practical functionality providers 6 Structuring programs with monads and monad transformers 7 Exceptions handling and logging 8 Testing and profilingSummary PART 3: ADVANCED HASKELL 9 Programming polymorphically 10 Type-level programming for type safety 11 Writing Haskell in Haskell PART 4: HASKELL TOOLKIT 12 Concurrent programming 13 Effective input-output 14 Parsing, serializing, and storing data 15 Featureful datatypes in data processing 16 Implementing web services PART 5: HASKELL AT LARGE 17 Case-study: large applications in Haskell 18 Haskell ecosystem ©Manning Publications Co. We welcome reader comments about anything in the manuscript - other than typos and other simple mistakes. These will be cleaned up during production of the book by copyeditors and proofreaders. https://forums.manning.com/forums/haskell-in-depth 1 Haskell in Depth: How to work with the source code0 examples All the source code in this book is written in Haskell so you will definitely need to get a Haskell compiler to work with it. We rely exclusively on the GHC, the Glasgow Haskell Compiler, although there are other compilers as well. Apart from your system distribution you can get GHC by either: • Stack (haskellstack.org) • A Minimal GHC installation (www.haskell.org/downloads) • The Haskell Platform (www.haskell.org/platform/) In what follows we’ll provide you with the short instructions on how to work with the source code examples that accompany this book. Depending on your own preferences you may choose which packaging and building approach to take, we support both stack and cabal tools which are customary for Haskell projects. 0.1 Getting the sources All the source code examples in this book are organized into a Haskell package which is uploaded to Hackage (hackage.haskell.org/package/hid-examples). Consequently, the easiest way to get all the examples is to download this package from Hackage using cabal (updating may be required in order to get the most current version): $ cabal update $ cabal get hid-examples or with stack: $ stack unpack hid-examples This will create a hid-examples-0.1.0.0 subdirectory in the current directory and you ©Manning Publications Co. We welcome reader comments about anything in the manuscript - other than typos and other simple mistakes. These will be cleaned up during production of the book by copyeditors and proofreaders. https://forums.manning.com/forums/haskell-in-depth 2 can start exploring the package content on your own. Alternatively, you may clone GitHub repository (github.com/bravit/hid-examples/): $ git clone https://github.com/bravit/hid-examples.git 0.2 Using Stack 0.2.1 Building $ stack build Note that building may take some time. 0.2.2 Running $ stack exec <executable> [ -- <arguments>] For example, to run the stockquotes example from Chapter 3 you need to specify the CSV file location and (optionally) several switches: $ stack exec stockquotes -- data/quotes.csv -p -v 0.2.3 Exploring in GHCi $ stack ghci <module file> For example: $ stack ghci stockquotes/Statistics.hs 0.3 Using Cabal sandbox 0.3.1 Building $ cabal sandbox init $ cabal install --only-dependencies $ cabal configure $ cabal build 0.3.2 Running $ cabal run <executable> [ -- <arguments>] For example: $ cabal run stockquotes -- data/quotes.csv -p -v 0.3.3 Exploring in GHCi $ cabal repl <executable> For example: ©Manning Publications Co. We welcome reader comments about anything in the manuscript - other than typos and other simple mistakes. These will be cleaned up during production of the book by copyeditors and proofreaders. https://forums.manning.com/forums/haskell-in-depth 3 $ cabal repl stockquotes To work with the particular module, you have to load it in GHCi with :load. 0.4 Using Cabal new-* 0.4.1 Building $ cabal new-build Cabal new-* features are expected to be heavily reworked and updated in the summer of 2018. When this job is done this approach may become the most convenient. ©Manning Publications Co. We welcome reader comments about anything in the manuscript - other than typos and other simple mistakes. These will be cleaned up during production of the book by copyeditors and proofreaders. https://forums.manning.com/forums/haskell-in-depth 4 Haskell nowadays1 This chapter covers: • How functional programming with types and support for laziness makes it easier to write programs • Which tools are available for Haskell development • Problems Haskell is good for The history of Haskell started more than 30 years ago, in 1987 (see haskell.cs.yale.edu/wp-content/uploads/2011/02/history.pdf for many exciting details). Nowadays it is a mature programming language, full of features, with a stable implementation, a helpful and friendly community, and a big enough ecosystem. Paraphrasing the Haskell 2010 Language Report (www.haskell.org/onlinereport/haskell2010/), which is an effective standard description of the Haskell Language, we can give it the following definition: DEFINITION Haskell is a general-purpose, purely functional programming language featuring higher-order functions, non-strict semantics, static polymorphic typing, user-defined algebraic datatypes, pattern matching, a module system, a monadic I/O system, and a rich set of primitive datatypes (including lists, arrays, arbitrary- and fixed-precision integers, and floating-point numbers). This definition is feature-centric, but gives a little information about how to use all this stuff professionally. Haskell is by far not the most popular programming language in the world, however, and there are two unfortunate myths that contribute to its limited adoption: ©Manning Publications Co.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages16 Page
-
File Size-