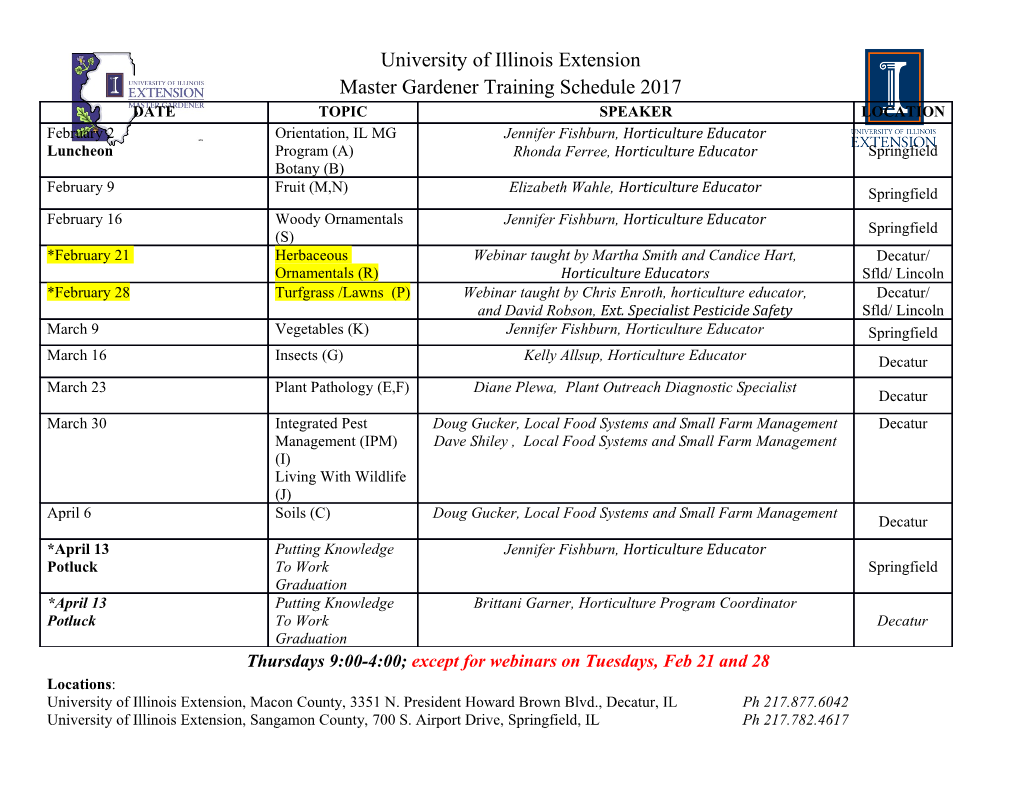
Ajax ● Asynchronous JavaScript and XML ● Combination of JavaScript, CSS, XHTML, XML, and the XMLHttpRequest object to dynamically modify a portion of a web page using data from the server ● Goal is to make web applications look and “feel” like desktop applications Slide Set 5 1 Ajax Examples ● Google Suggest http://www.google.com/webhp?complete=1&hl=en Compare this to www.google.com where you'll see a page refresh when google returns with search results ● PHP free chat http://www.phpfreechat.net/demo.en.php ● Plastic shore chat http://www.plasticshore.com/projects/chat Slide Set 5 2 Ajax Examples ● Online spreadsheet http://numsum.com/spreadsheet/new ● Auto completion and live search http://www.papermountain.org/demos/live ● Viewing amazon products http://lmap.co.nr/Amazon1.htm Slide Set 5 3 A first Ajax example ● Simple example to read a text file off of the server and display the contents in a div http://www.cs.appstate.edu/~can/classes/5530/set5/ajaxgetmsg1.html Slide Set 5 4 var XMLHttpRequestObject = false; if (window.XMLHttpRequest) //Firefox way to create object XMLHttpRequestObject = new XMLHttpRequest(); else if (window.ActiveXObject) //IE way to create object XMLHttpRequestObject = new ActiveXObject("Microsoft.XMLHTTP"); else alert("Ajax not supported"); function getData(dataSource, divID) { var obj = document.getElementById(divID); obj = obj.getElementsByTagName("p")[0]; if (XMLHttpRequestObject) { XMLHttpRequestObject.open("GET", dataSource); XMLHttpRequestObject.onreadystatechange = function() { if (XMLHttpRequestObject.readyState == 4 && XMLHttpRequestObject.status == 200) obj.innerHTML = XMLHttpRequestObject.responseText; } XMLHttpRequestObject.send(null); } else obj.innerHTML = "Ajax not supported"; } Slide Set 5 5 <body> <form action=""> <p> <input type="button" value="Fetch the message" onclick = "getData('msg.txt', 'targetDiv')" /> </p> </form> <div id="targetDiv"> <p>Fetched message will appear here.</p> </div> </body> When button is clicked, call getData passing it the name of the message file and the id of the div to hold the message Slide Set 5 6 XMLHttpRequest object ● Allows requests for XML (and other types of data) to made and occur in the background ● Different properties and methods are available depending upon the browser Slide Set 5 7 XMLHttpRequest properties for IE ● onreadystatechange – contains name of the event handler that should be called when the value of the readyState property changes ● readyState – contains the state of the request. read-only ● responseBody – contains a response body, which is one way HTTP responses can be returned. read-only ● responseStream – contains a response stream, a binary stream to the server. read-only ● responseText – contains the response body as a string. read-only ● responseXML – contains the response body as XML. read-only ● status – contains the HTTP status code returned by a request. read- only ● statusText – contains the HTTP response status text. read-only Slide Set 5 8 XMLHttpRequest methods for IE ● abort – aborts the HTTP request ● getAllResponseHeaders – returns all the HTTP headers ● getResponseHeader - returns the value of an HTTP header ● open – opens a request to the server ● send – sends an HTTP request to the server ● setRequestHeader – sets the name and value of an HTTP header Slide Set 5 9 XMLHttpRequest properties for Firefox ● channel – contains the channel used to perform the request. read- only ● readyState – contains the state of the request. read-only ● responseText – contains the response body as a string. read-only ● responseXML – contains the response body as XML. read-only ● status – contains the HTTP status code returned by a request. read- only ● statusText – contains the HTTP response status text. read-only ● onreadystatechange – contains the name of the event handler that should be called when the value of the readyState property changes. Slide Set 5 10 XMLHttpRequest methods for Firefox ● abort – aborts the HTTP request ● getAllResponseHeaders – returns all the HTTP headers ● getResponseHeader – returns the value of an HTTP header ● openRequest – native (non-script) method to open a request ● overrideMimeType – overrides the MIME type the server returns ● open – opens a request to the server ● send – sends an HTTP request to the server Slide Set 5 11 Back to the example ● First, create the XMLHttpRequest object ● Firefox, Mozilla, Netscape (7.0+) , Safari (1.2+) method: XMLHttpRequestObject = new XMLHttpRequest(); ● IE (5.0 and above) method: XMLHttpRequestObject = new ActiveXObject("Microsoft.XMLHTTP"); ● Both of these need to be preceded by a check for whether the method is available ● Neither will work on older browsers Slide Set 5 12 Back to the example ● Next, open a connection to a given URL using a specified method ● Syntax: open(method, url [,async, username, password]). method – GET or POST async – true (asynchronous; the default), false username/password if needed by the server ● The example: XMLHttpRequestObject.open("GET", dataSource); Slide Set 5 13 Back to the example ● Register the event handler to be executed when the value of the readyState property changes ● The example uses a function a literal, but can be done with declarative function instead XMLHttpRequestObject.onreadystatechange = function() { if (XMLHttpRequestObject.readyState == 4 && XMLHttpRequestObject.status == 200) obj.innerHTML = XMLHttpRequestObject.responseText; } Slide Set 5 14 Back to the example ● Next, send the data ● If the HTTP action is a POST then the data is sent via the XMLHttpRequest send method ● Since this example is doing a GET the parameter to the send is null: XMLHttpRequestObject.send(null); Slide Set 5 15 Back to the example ● Check the readyState property for information about the download XMLHttpRequestObject.onreadystatechange = function() { if (XMLHttpRequestObject.readyState == 4 && XMLHttpRequestObject.status == 200) obj.innerHTML = XMLHttpRequestObject.responseText; } ● Possible property values: 0 – uninitialized, 1 – loading, 2 – loaded, 3 – interactive, 4 – complete ● During successful action, the readyState property starts at 0 and changes to 4 Slide Set 5 16 Back to the example ● Also, check the status property; same status code is retrieved by HTTP requests XMLHttpRequestObject.onreadystatechange = function() { if (XMLHttpRequestObject.readyState == 4 && XMLHttpRequestObject.status == 200) obj.innerHTML = XMLHttpRequestObject.responseText; } ● possible property values 200 – OK, 201 – Created, 204 – No Content, 205 – Reset Content, 206- Partial Content, 400 – Bad Request, 401 – Unauthorized, 403 – Forbidden, 404 – Not Found, ... (lots more) Slide Set 5 17 Back to the example ● Finally, retrieve the data ● If the data is in XML form, retrieve from the responseXML property XMLHttpRequestObject.responseXML ● If the data is text (like in this example), retrieve from the responseText property obj.innerHTML = XMLHttpRequestObject.responseText Slide Set 5 18 More ways to create XMLHttpRequest objects ● There are actually various versions the object available in IE – create the normal version with Microsoft.XMLHTTP ActiveX object – more recent versions available: Msxml2.XMLHTTP, Msxml2.XMLHTTP.3.0, Msxml2.XMLHTTP.4.0, Msxml2.XMLHTTP.5.0 ● A common approach to creating the object is to use nested try and catch statements Slide Set 5 19 window.onload = getXMLHttpRequest; var XMLHttpRequestObject = false; function getXMLHttpRequest() { if (window.XMLHttpRequest) //Firefox way to create object XMLHttpRequestObject = new XMLHttpRequest(); else { try { XMLHttpRequestObject = new ActiveXObject("Msxml2.XMLHTTP"); } catch (e) { try { XMLHttpRequestObject = new ActiveXObject("Microsoft.XMLHTTP"); } catch (e) { alert("Ajax not supported"); } } } http://www.cs.appstate.edu/~can/classes/5530/set5/ajaxgetmsg2.html } Slide Set 5 20 Running server side code ● Need a server side language that can process the Ajax requests – a number of languages are available (Perl, Ruby, Java, PHP, C#, Visual Basic, ...) ● Here's a PHP program that returns text to client <?php echo 'Welcome to Ajax!' ?> Slide Set 5 21 Running server side code ● Invoking the php program <body> <form action=""> <p> <input type="button" value="Fetch the message" onclick = "getData('ajaxphp1.php', 'targetDiv')" /> </p> </form> <div id="targetDiv"> <p>Fetched message will appear here.</p> </div> </body> http://www.cs.appstate.edu/~can/classes/5530/set5/ajaxphp1.html 22 Passing Data to PHP Script using GET ● GET uses URL encoding so data must be appended to the URL that is sent to the server ● Suppose we want to send values: a = 10 b = 32 c = “hello there” ● These would be sent like: http://www.servername.com/path/script.php?a=10&b=32&c=hello+there Slide Set 5 23 Simple Example ● XHTML <form action=""> <p> <input type="button" value="Fetch message 1" onclick = "getData('ajaxphp2.php?data=1', 'targetDiv')" /> <input type="button" value="Fetch message 2" onclick = "getData('ajaxphp2.php?data=2', 'targetDiv')" /> </p> </form> <div id="targetDiv"> <p>Fetched message will appear here.</p> </div> http://www.cs.appstate.edu/~can/classes/5530/set5/ajaxphp2.html Slide Set 5 24 Simple Example ● PHP code <?php extract($_GET); if ($data == "1") echo 'Welcome to Ajax!'; if ($data == "2") echo 'Ajax is fun!'; ?> Slide Set 5 25 Passing data to PHP script using POST ● Using a post request, the data is encoded in the body of the message ● Modifying the simple example to use a post: – change the PHP code so that it accesses POST array instead of GET array – change the XMLHttpRequest
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages101 Page
-
File Size-