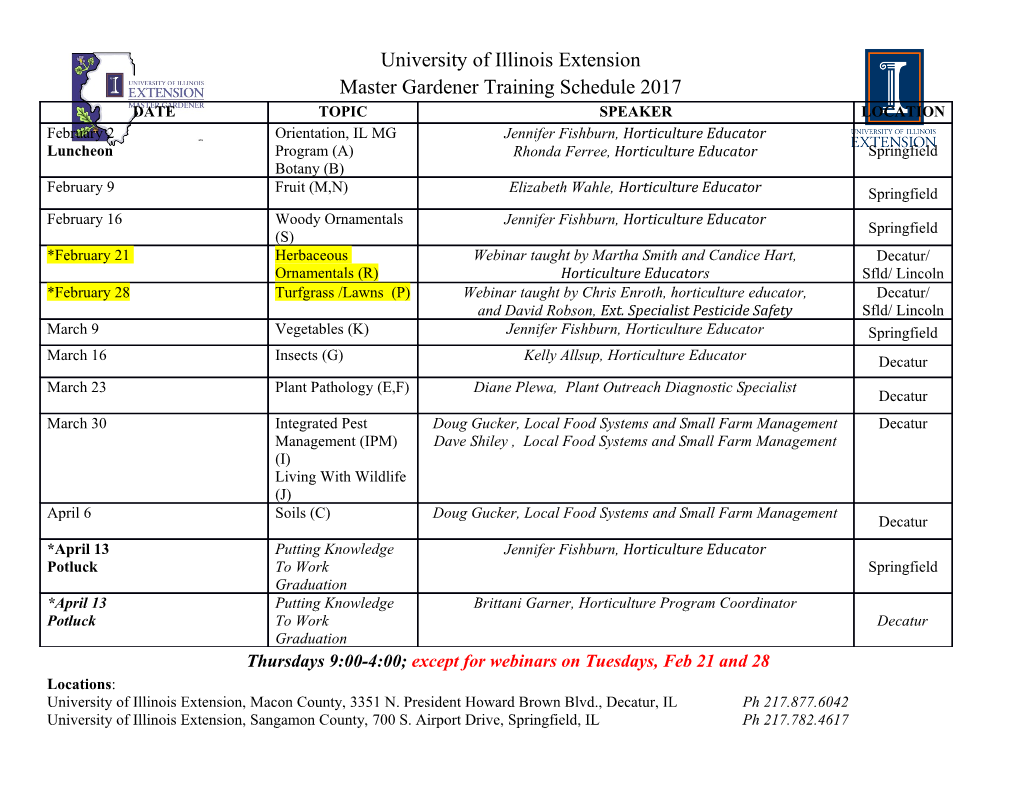
CS 242 Thanks! Review Teaching Assistants • Mike Cammarano • TJ Giuli • Hendra Tjahayadi John Mitchell Graders • Andrew Adams Tait Larson Final Exam • Kenny Lau Aman Naimat • Vishal Patel Justin Pettit Wednesday Dec 8 8:30 – 11:30 AM • and more … Gates B01, B03 Course Goals There are many programming languages Understand how programming languages work Early languages Appreciate trade-offs in language design • Fortran, Cobol, APL, ... Be familiar with basic concepts so you can Algol family understand discussions about • Algol 60, Algol 68, Pascal, …, PL/1, … Clu, Ada, Modula, • Language features you haven’t used Cedar/Mesa, ... • Analysis and environment tools Functional languages • Implementation costs and program efficiency • Lisp, FP, SASL, ML, Miranda, Haskell, Scheme, Setl, ... • Language support for program development Object-oriented languages • Smalltalk, Self, Cecil, … • Modula-3, Eiffel, Sather, … • C++, Objective C, …. Java General Themes in this Course Concurrent languages Language provides an abstract view of machine • Actors, Occam, ... • We don’t see registers, length of instruction, etc. • Pai-Lisp, … The right language can make a problem easy; Proprietary and special purpose languages wrong language can make a problem hard • Could have said a lot more about this • TCL, Applescript, Telescript, ... Language design is full of difficult trade-offs • Postscript, Latex, RTF, … • Expressiveness vs efficiency, ... • Domain-specific language • Important to decide what the language is for Specification languages • Every feature requires implementation data structures • CORBA IDL, ... and algorithms • Z, VDM, LOTOS, VHDL, … 1 Good languages designed with specific A good language design presents abstract goals (often an intended application) machine, an idealized view of computer • C: systems programming • Lisp: cons cells, read-eval-print loop • Lisp: symbolic computation, automated reasoning • FP: ?? • FP: functional programming, algebraic laws • ML: functions are basic control structure, memory model • ML: theorem proving includes closures and reference cells • Clu, ML modules: modular programming • C: the underlying machine + abstractions • Simula: simulation • Simula: activation records and stack; object references • Smalltalk: Dynabook, • Smalltalk: objects and methods • C++: add objects to C • C++: ?? • Java: set-top box, internet programming • Java: Java virtual machine Design Issues Language design involves many trade-offs In general, high-level languages/features are: • space vs. time • slower than lower-level languages • efficiency vs. safety – C slower than assembly • efficiency vs. flexibility – C++ slower than C – Java slower than C++ • efficiency vs. portability • provide for programs that would be • static detection of type errors vs. flexibility difficult/impossible otherwise • simplicity vs. "expressiveness" etc – Microsoft Word in assembly language? These must be resolved in a manner that is – Extensible virtual environment without objects? • consistent with the language design goals – Concurrency control without semaphores or monitors? • preserves the integrity of abstract machine Many program properties are undecidable (can't determine statically ) Languages are still evolving • Halting problem • Object systems • nil pointer detection • Adoption of garbage collection • alias detection • Concurrency primitives; abstract view of concurrent • perfect garbage detection systems • etc. • Domain-specific languages Static type systems • Network programming • detect (some) program errors statically • Aspect-oriented programming and many other “fads” – Every good idea is a fad until is sticks • can support more efficient implementations • are less flexible than either no type system or a dynamic one 2 Summary of the course Lisp Summary Lisp, 1960 Modularity and objects Successful language Fundamentals • encapsulation • Symbolic computation, experimental programming • lambda calculus • dynamic lookup Specific language ideas • denotational semantics • subtyping • Expression-oriented: functions and recursion • functional prog • inheritance • Lists as basic data structures ML and type systems Simula and Smalltalk • Programs as data, with universal function eval Block structure and C++ • Stack implementation of recursion via "public pushdown list" activation records Java • Idea of garbage collection. Exceptions and Concurrency continuations Fundamentals Algol Family and ML Grammars, parsing Evolution of Algol family Lambda calculus • Recursive functions and parameter passing Denotational semantics • Evolution of types and data structuring Functional vs. Imperative Programming ML: Combination of Lisp and Algol-like features • Is implicit parallelism a good idea? • Expression-oriented • Is implicit anything a good idea? • Higher-order functions • Garbage collection • Abstract data types • Module system • Exceptions Types and Type Checking Type inference algorithm Types are important in modern languages Example • Program organization and documentation - fun f(x) = 2+x; Graph for λx. ((plus 2) x) • Prevent program errors > val it = fn : int → int • Provide important information to compiler t→int = int→int How does this work? λ Type inference int (t = int) • Determine best type for an expression, based on Assign types to leaves @ known information about symbols in the expression Propagate to internal @ int→int x : t Polymorphism nodes and generate : int • Single algorithm (function) can have many types constraints + 2 int → int → int Overloading real → real→real • Symbol with multiple meanings, resolved at compile Solve by substitution time 3 Block structure and storage mgmt Summary of scope issues Block-structured languages and stack storage Block-structured lang uses stack of activ records In-line Blocks • Activation records contain parameters, local vars, … • activation records • Also pointers to enclosing scope • storage for local, global variables Several different parameter passing mechanisms First-order functions Tail calls may be optimized • parameter passing Function parameters/results require closures • tail recursion and iteration • Closure environment pointer used on function call Higher-order functions • Stack deallocation may fail if function returned from call • deviations from stack discipline • Closures not needed if functions not in nested blocks • language expressiveness => implementation complexity Control Modularity and Data Abstraction Structured Programming Step-wise refinement and modularity • Go to considered harmful • History of software design Exceptions Language support for information hiding • “structured” jumps that may return a value • Abstract data types • dynamic scoping of exception handler • Datatype induction Continuations • Packages and modules • Function representing the rest of the program Generic abstractions • Generalized form of tail recursion • Datatypes and modules with type parameters • Design of STL Concepts in OO programming Simula 67 Four main language ideas First object-oriented language • Encapsulation Designed for simulation • Dynamic lookup • Later recognized as general-purpose prog language • Subtyping Extension of Algol 60 • Inheritance Standardized as Simula (no “67”) in 1977 Why OOP ? Inspiration to many later designers • Extensible abstractions; separate interface from impl • Smalltalk Compare oo to conventional (non-oo) lang • C++ • Can represent encapsulation and dynamic lookup • ... • Need inheritance and subtyping as basic constructs 4 Objects in Simula Smalltalk Class Major language that popularized objects • A procedure that returns a pointer to its activation record Developed at Xerox PARC 1970’s (Smalltalk-80) Object Object metaphor extended and refined • Activation record produced by call to a class • Used some ideas from Simula, but very different lang Object access • Everything is an object, even a class • Access any local variable or procedures using dot • All operations are “messages to objects” notation: object. • Very flexible and powerful language Memory management – Similar to “everything is a list” in Lisp, but more so • Objects are garbage collected Method dictionary and lookup procedure • Simula Begin pg 48-49: user destructors undesirable • Run-time search; no static type system Independent subtyping and inheritance C++ Examples Design Principles: Goals, Constraints If circle <: shape, then Object-oriented features • Some good decisions, some problem areas circle → shape Classes, Inheritance and Implementation • Base class and Derived class (inheritance) circle → circle shape → shape • Run-time structures: offset known at compile time Subtyping shape → circle • Subtyping principles • Abstract base classes • Specializing types of public members C++ compilers recognize limited forms of function subtyping Multiple Inheritance Subtyping with functions Java function subtyping class Point { class ColorPoint: public Point { Signature Conformance public: Inherited, but repeated public: • Subclass method signatures must conform to those of int getX(); here for clarity int getX(); superclass virtual Point *move(int); int getColor(); protected: ... ColorPoint * move(int); Argument types, Return type, Exceptions: void darken(int); private: ... How much conformance is really needed? protected: ... }; private: ... Java rule }; • Arguments and returns must have identical types, may remove exceptions In principle: can have ColorPoint <: Point In practice: some compilers allow, others have not This is covariant case; contravariance is another story 5 A
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages7 Page
-
File Size-