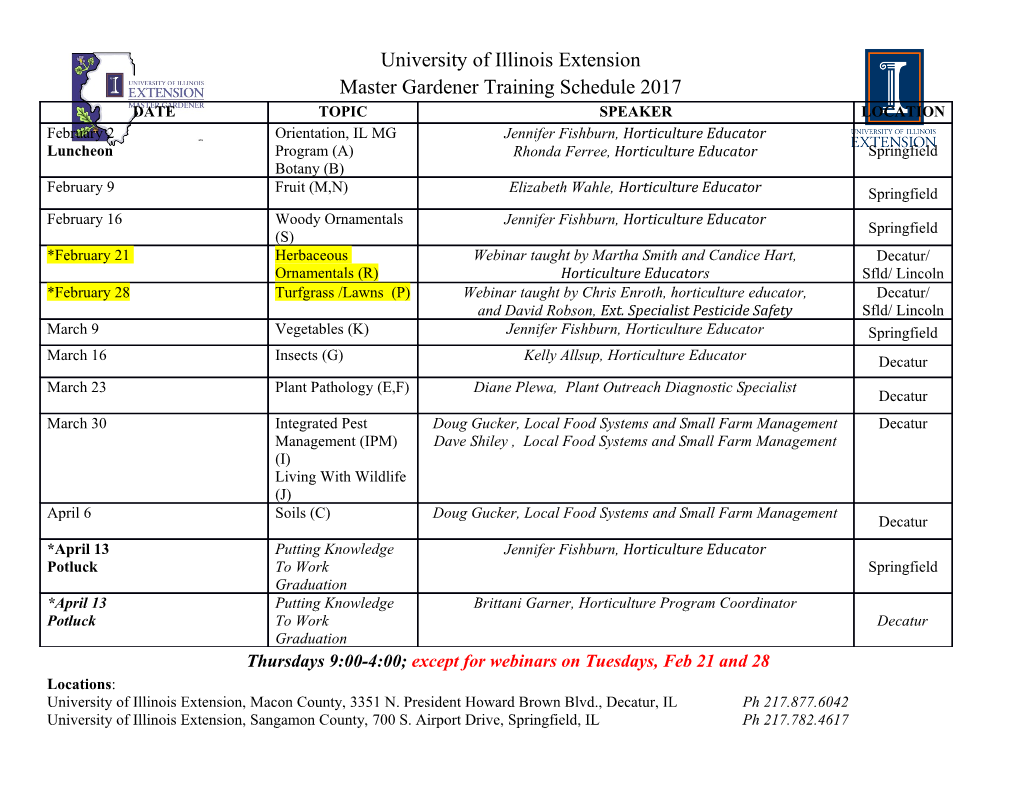
Lisp Introduction Dr. Neil T. Dantam CSCI-534, Colorado School of Mines Spring 2020 Dantam (Mines CSCI-534) Lisp Spring 2020 1 / 80 What is Lisp? Definition (Lisp) A family of programming languages based on s-expressions. Data Structure “Math” NIL ( defun factorial NIL 1 if n = 0 n! = n ∗ (n − 1)! if n 6= 0 n 1 NIL if 0 NIL Code = n ( defun f a c t ( n ) NIL ( i f (= n 0) n * NIL 1 (∗ n ( f a c t (− n 1 ) ) ) ) ) factorial 1 NIL - n Dantam (Mines CSCI-534) Lisp Spring 2020 2 / 80 Homoiconic Code is Data Code Data Structure NIL ( defun f a c t ( n ) defun factorial NIL ( i f (= n 0) n 1 (∗ n ( f a c t (− n 1 ) ) ) ) ) 1 NIL if 0 NIL = n NIL n * NIL factorial 1 NIL - n “data processing” () “code processing” Dantam (Mines CSCI-534) Lisp Spring 2020 3 / 80 Introduction Why study lisp? Outcomes I Understand Lisp abstractions I Functional Programming: I Symbols I Planning algorithms often are I S-expressions functional/recursive I First-class functions Lisp has good support for functional I (closures) programming. Implement Lisp programs in I Symbolic Computing: I functional style I Planning algorithms must often process symbolic expressions I (Review differential calculus I Lisp has good support for symbolic processing and numerical methods) I Understanding the abstractions in Lisp will make you a better programmer. Dantam (Mines CSCI-534) Lisp Spring 2020 4 / 80 The Lisp Learning Process Yee-haw! start “Lisp is weird!” “I dislike Learn to think differences!” give symbolically? not yet up yes end “Lisp is awesome!” end Lisp might feel like it’s “from outer space” (at first). The symbolic view of programming is different, often useful. Dantam (Mines CSCI-534) Lisp Spring 2020 5 / 80 Lots of Irritating Silly Parenthesis? “LISP has jokingly been described as ‘the most intelligent way to misuse a com- puter’. I think that description a great compliment because it transmits the full flavour of liberation: it has assisted a number of our most gifted fellow humans in thinking previously impossible thoughts.” [emphasis added] https://xkcd.com/297/ Dantam (Mines CSCI-534) Lisp Spring 2020 6 / 80 Common Lisp by Example Outline Common Lisp by Example Basics Recursion First-class functions Higher-order Functions Implementation Details Programming Environment Dantam (Mines CSCI-534) Lisp Spring 2020 7 / 80 Common Lisp by Example Basics Booleans and Equality “Math” Lisp Notes False nil equivalent to the empty list () True t or any non-nil value :a (not a) a = b (= a b) numerical comparison a = b (eq a b) same object a = b (eql a b) same object, same number and type, or same character a = b (equal a b) eql objects, or lists/arrays with equal elements a = b (equalp a b) = numbers, or same character (case-insensitive), or recursively-equalp cons cells, arrays, structures, hash tables a 6= b (not (= a b)) similarly for other equality functions Dantam (Mines CSCI-534) Lisp Spring 2020 8 / 80 Common Lisp by Example Basics Example: Lisp Equality Operators I (= 1 1) t I (= "a" "a") error I (eq 1 1) t I (eq "a" "a") nil integer float I (eql "a" "a") nil z}|{ z}|{ (= 1 1:0 ) t I I (equal "a" "a") t integer float z}|{ z}|{ I (equal "a" "A") nil I (eq 1 1:0 ) nil I (equalp "a" "A") t integer float z}|{ z}|{ I (not t) nil I (eql 1 1:0 ) nil (not nil) t integer float I z}|{ z}|{ I (not "a") nil I (equal 1 1:0 ) nil integer float z}|{ z}|{ I (equalp 1 1:0 ) t Dantam (Mines CSCI-534) Lisp Spring 2020 9 / 80 nil nil nil t t nil nil t nil t t Common Lisp by Example Basics Exercise: Lisp Equality Operators (eq (list "a""b") (list "a""b")) I (not 0) I (equal (list "a""b") (list "a""b")) I (not 1) I I (eq t (not nil)) I (eq t 1) I (eq (list "a""b") (list "a""B")) (eq nil (not 1)) I I (equal (list "a""b") (list "a""B")) I (eq nil (not "a")) I (equalp (list "a""b") (list "a""B")) Dantam (Mines CSCI-534) Lisp Spring 2020 10 / 80 Common Lisp by Example Basics Inequality “Math” Lisp a < b (< a b) a ≤ b (<= a b) a > b (> a b) a ≥ b (>= a b) Dantam (Mines CSCI-534) Lisp Spring 2020 11 / 80 Common Lisp by Example Basics Function Definition DEFUN Defines a new function in the global environment. (defun FUNCTION-NAME ARGUMENTS BODY...) Pseudocode Common Lisp function name function arguments Procedure increment(n) z }| { z}|{ 1 return n + 1; (defun increment (n) (+ n 1)) | {z } result Dantam (Mines CSCI-534) Lisp Spring 2020 12 / 80 ( defun sinc (theta) (/( s i n t h e t a ) t h e t a ) ) Common Lisp by Example Basics Exercise: Function Definition Sine Cardinal sin θ sinc θ = θ Pseudocode Common Lisp Procedure sinc(θ) 1 return sin(θ)/θ; What’s wrong (mathematically) with this definition? Dantam (Mines CSCI-534) Lisp Spring 2020 13 / 80 Common Lisp by Example Basics Limit of sinc θ d sin θ l’Hôpital’s rule dθ sin θ cos θ lim lim lim 1 θ!0 θ θ!0 d θ!0 1 dθ θ 1 0:5 0 −15 −10 −5 0 5 10 15 Dantam (Mines CSCI-534) Lisp Spring 2020 14 / 80 Common Lisp by Example Basics Conditional IF IF Conditional execution based on a single test: (if TEST THEN-EXPRESSION [ELSE-EXPRESSION]) Pseudocode Common Lisp Procedure even?(n) (defun even? (n) test 1 if 0 = mod(n; 2) then z }| { (if (= 0 (mod n 2)) 2 return true; then clause 3 else z}|{t 4 return false; NIL )) |{z} else clause Dantam (Mines CSCI-534) Lisp Spring 2020 15 / 80 ( defun sinc (theta) (if (= 0 theta) 1 (/( s i n t h e t a ) t h e t a ) ) ) Common Lisp by Example Basics Exercise: Conditionals IF ( 1 if θ = 0 sinc(θ) = sin θ θ if θ 6= 0 Pseudocode Common Lisp Procedure sinc(θ) 1 if 0 = θ then 2 return 1; 3 else 4 return sin(θ)/θ; Dantam (Mines CSCI-534) Lisp Spring 2020 16 / 80 Common Lisp by Example Basics Taylor Series Represent function f (x) as infinite sum of derivatives around point a: f 0(a) f 00(a) f 000(a) f (x) = f (a) + (x − a) + (x − a) + (x − a) + ::: 1! 2! 3! 1 ! X f (n)(a) = (x − a)n n! n=0 Polynomial approximation of functions Dantam (Mines CSCI-534) Lisp Spring 2020 17 / 80 Common Lisp by Example Basics Sinc Taylor Series sin θ θ2 θ4 θ6 θ8 θ10 = 1 − + − + − + ::: θ 6 120 5040 362880 39916800 2 n = 1 n = 0 n = 2 1 n = 4 n = 6 n = 8 0 n = 10 −1 −15 −10 −5 0 5 10 15 Dantam (Mines CSCI-534) Lisp Spring 2020 18 / 80 Common Lisp by Example Basics Conditional COND COND Conditional execution of the first clause with a true test: (cond CLAUSES...) where each clause is of the form: (TEST EXPRESSIONS...) Pseudocode Common Lisp Procedure sign(n) (defun sign (n) test result 1 if n > 0 then return 1 ; z }| { z}|{ (cond ((> n 0) 1 ) 2 else if n < 0 then return −1 ; test result 3 else return 0 ; z }| { z}|{ ((< n 0) −1 ) test result z}|{ (z}|{t 0 ))) Dantam (Mines CSCI-534) Lisp Spring 2020 19 / 80 ( defun sinc (theta) ( cond ((= 0 theta) 1) ((< (∗ theta theta) .00001) (+ 1 (/( expt t h e t a 2) −6) (/( expt theta 4) 120))) ( t (/ ( s i n t h e t a ) t h e t a ) ) ) ) Common Lisp by Example Basics Exercise: Conditionals COND sin θ θ2 θ4 = 1 − + + ::: θ 6 120 Pseudocode Common Lisp Procedure sinc(θ) 1 if 0 = θ then 2 return 1; 3 else if θ2 < :00001 then 4 return 1 − θ2=6 + θ4=120; 5 else 6 return sin(θ)/θ; Dantam (Mines CSCI-534) Lisp Spring 2020 20 / 80 Common Lisp by Example Recursion Recursion Example: Factorial ( 1 if n = 0 n! = Recursion: A function (or other object) defined n ∗ (n − 1)! if n 6= 0 in terms of itself Base Case: Terminating condition Procedure factorial(n) Recursive Case: Reduction towards the base case 1 if 0 = n then // base case 2 return 1; 3 else // recursive case 4 return n ∗ factorial(n − 1); Dantam (Mines CSCI-534) Lisp Spring 2020 21 / 80 Common Lisp by Example Recursion Example: Factorial ( 1 if n = 0 n! = n ∗ (n − 1)! if n 6= 0 Pseudocode Common Lisp Procedure factorial(n) ( defun factorial (n) 1 if 0 = n then // base case ( i f (= n 0) 2 return 1; 1 3 else // recursive case (∗ n (factorial (− n 1 ) ) ) ) ) 4 return n ∗ factorial(n − 1); Dantam (Mines CSCI-534) Lisp Spring 2020 22 / 80 Common Lisp by Example Recursion Factorial Execution Trace factorial(3) Procedure factorial(n) 3*factorial(2) 1 if 0 = n then // base case 2 return 1; 3*(2*factorial(1)) 3 else // recursive case 4 return n ∗ factorial(n − 1); 3*(2*(1*factorial(0))) 3*(2*(1*1)) Dantam (Mines CSCI-534) Lisp Spring 2020 23 / 80 Common Lisp by Example Recursion Factorial Call Stack fact(0) fact(1) fact(1) fact(2) fact(2) fact(2) fact(3) fact(3) fact(3) fact(3) fact(3) 3*fact(2) 3*(2*fact(1)) 3*(2*(1*fact(0))) fact(0) 1 fact(1) fact(1) 1 fact(2) fact(2) fact(2) 2 fact(3) fact(3) fact(3) fact(3) 6 3*(2*(1*1)) 3*(2*1) 3*2 6 Dantam (Mines CSCI-534) Lisp Spring 2020 24 / 80 Common Lisp by Example Recursion Exercise: Recursion, Fibonacci Sequence (1; 1; 2; 3; 5; 8; 13; 21; 34; 55;:::) 8 1 if n = 0 <> fib(n) = 1 if n = 1 :>fib(n − 1) + fib(n − 2) if n ≥ 2 Dantam (Mines CSCI-534) Lisp Spring 2020 25 / 80 Procedure fib(x) 1 if 0 = n then return 1 ; ( defun f i b ( n ) 2 else if 1 = n then return 1 ; ( cond ((= n 0) 1) 3 else ((= n 1) 1) 4 return fib(n − 1) + fib(n − 2); ( t (+ ( f i b (− n 1) ) ( f i b (− n 2 ) ) ) ) ) ) Common Lisp by Example Recursion Exercise: Recursion, Fibonacci Sequence continued Pseudocode Common Lisp Dantam (Mines CSCI-534) Lisp Spring 2020 26 / 80 Function accumulate(S) 1 if S then // Recursive Case 2 return car(S) + accumulate (cdr (S)); 3 else // Base Case 4 return 0; Common Lisp by Example Recursion Exercise: Recursion, Accumulate Iterative Recursive Function accumu- late(S) 1 a 0; 2 i 0; 3 while i < jSj do 4 a a + Si ; 5 return a; Dantam (Mines CSCI-534) Lisp Spring 2020 27 / 80 Recursive Implementation of Accumulate Function accumulate(S) 1 if S then // Recursive Case ( defun accumulate ( l i s t ) 2 return ( i f l i s t car(S) + accumulate
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages80 Page
-
File Size-