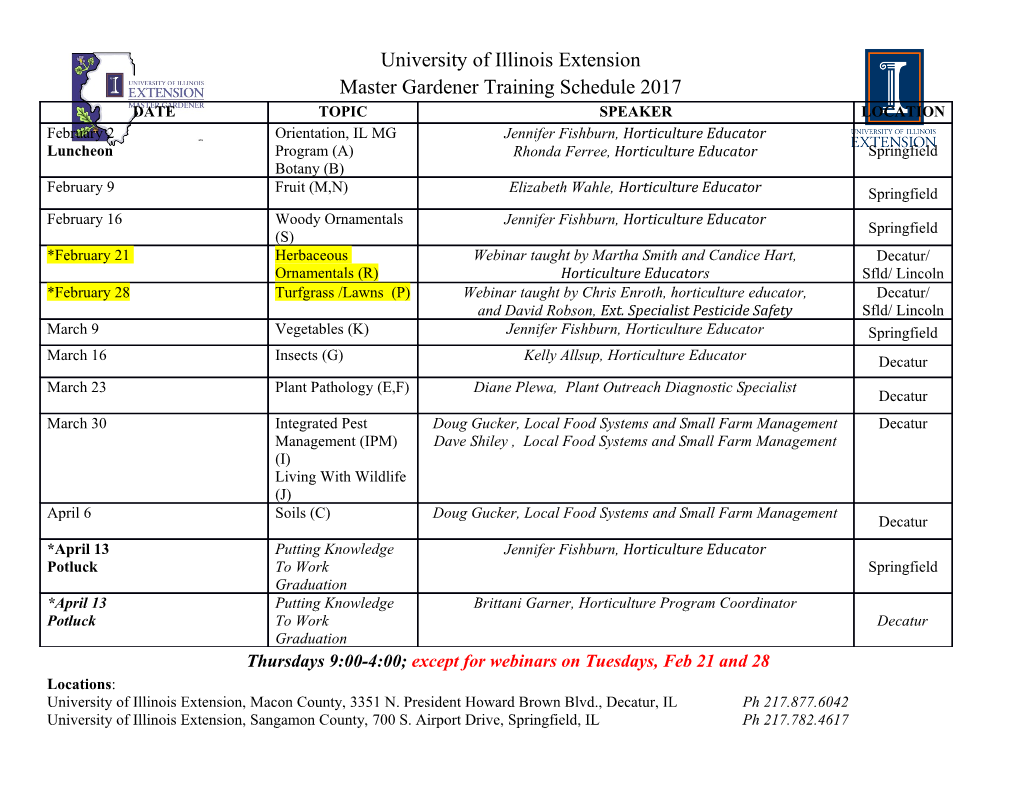
Programming Tools Lecture Plan for Group Projects ● Unit testing ● Version control ● Building Richard Smith ● Debugging [email protected] – Logging ● Documenting – Code – Reports 1 2 Biased General advice ● Use a Unix based operating system – Solaris in the labs – Mac OS X if you are buying a laptop – Install Linux if you have a PC at home Unit testing ● Learn how to do everything using the command line, then use an IDE! – Xemacs – difficult to learn, but integrates well with command line and has modes for everything – Eclipse – easier to learn, specialised for Java ● Use Java or C++ or 'script' (Python, Ruby, Perl) as appropriate 3 4 Background: Extreme Unit Testing Programming ● Pair programming ● Write tests before code. ● Small releases ● Code not done until tests all run. ● Metaphor ● Re-run tests after any major changes ● Simple design ● Can be confident that nothing has ● Testing broken. ● Refactoring ● Hence not afraid to refactor. www.extremeprogramming.org 5 6 Tools for Testing Installing CPPUnit ● Some functional tests can be manual. ● We use GNU compiler on Unix-like OS (Linux, FreeBSD) ● Possible to automate most tests. ● wget http://aleron.dl.sourceforge.net/sourceforge/cppunit/cppuni ● Tools exist to make this easy and t-1.10.2.tar.gz provide GUI. ● tar xvfz cppunit-1.10.2.tar.gz ● cd cppunit-1.10.2 ● Java – JUnit – www.junit.org ● ./configure; make ● C++ – CPPunit – cppunit.sf.net ● su -c 'make install' ● su -c 'ldconfig' ● Installation of GUI library is a bit more complicated :-( 7 8 Or you can use mine! C++ Example ● bash ● Class diagram ● export LD_LIBRARY_PATH=/cs/research/nets/home/marine/ucacrts /g2/local/lib ● export PATH=/cs/research/nets/home/marine/ucacrts/g2/local/b in:$PATH 9 10 C++ Example C++ Example Cat.h Animal. #ifndef CAT_H h#ifndef ANIMAL_H #define CAT_H ● Source files (arrows show includes) #define ANIMAL_H #include "Animal.h" class Animal{ ● Additional includes class Cat: public Animal{ public: are not necessary, public: virtual int getNoOfLegs(); but are allowed, virtual int getNoOfLegs(); }; because of }; #endif #IFNDEF guards #endif Animal.cpp Cat.cpp #include "Animal.h" #include "Cat.h" int Animal::getNoOfLegs(){ int Cat::getNoOfLegs(){ return 0; return 5; } } 11 12 C++ Example C++ Example TestCat.h #ifndef TESTCAT_H TestCat.cpp #define TESTCAT_H #include "TestCat.h" #include <cppunit/extensions/HelperMacros.h> #include "Cat.h" CPPUNIT_TEST_SUITE_REGISTRATION( TestCat ); class TestCat: public CPPUNIT_NS::TestFixture{ void TestCat::testLegs(){ CPPUNIT_TEST_SUITE(TestCat); Cat cat; CPPUNIT_TEST(testLegs); CPPUNIT_ASSERT_EQUAL(4, cat.getNoOfLegs()); CPPUNIT_TEST_SUITE_END(); } public: void setUp(); void TestCat::setUp(){ protected: } void testLegs(); }; #endif 13 14 C++ Example CPPUnit Output Options runtest.cp #pinclude <cppunit/TextTestRunner.h> ● TestRunner with CompilerOutputter – #include "TestCat.h" prints results suitable for use with IDE int main( int argc, char* argv[] ) { ● with XMLOutputter – results as XML CppUnit::TextTestRunner runner; runner.addTest(TestCat::suite()); document for processing by another bool result = runner.run("TestCat::testLegs", true, true, true); return result ? 0 : 1; application } ● TextTestRunner – human readable $> g++ -O2 -o runtest Animal.cpp Cat.cpp TestCat.cpp output runtest.cpp -ldl -lcppunit ● QtTestRunner – output in GUI ● (MfcTestRunner, WxTestRunner) 15 16 TextTestRunner Output Success $> ./runtest .F $> ./runtest . !!!FAILURES!!! Test Results: OK (1 tests) Run: 1 Failures: 1 Errors: 0 <RETURN> to continue 1) test: TestCat::testLegs (F) line: 7 TestCat.cpp equality assertion failed - Expected: 4 - Actual : 5 17 18 Installing CPPUnit QT QT GUI Example ● Install tmake from runtestgui.cpp #include <qapplication.h> ftp.trolltech.com/freebies/tmake #define QTTESTRUNNER_API __declspec(dllimport) #include <cppunit/ui/qt/TestRunner.h> ● After installing cppunit: #include "TestCat.h" ● cd src/qttestrunner int main( int argc, char* argv[] ) { ● TMAKEPATH=/usr/lib/tmake/linux-g++ tmake QApplication app( argc, argv ); qttestrunner.pro -o makefile CppUnit::QtTestRunner runner; runner.addTest( TestCat::suite() ); ● make runner.run(true); ● su -c 'cp -d ../../lib/* /usr/local/lib' return 0; } ● su -c 'ldconfig' 19 20 QT GUI QT GUI Results $> g++ -O2 -o runtestgui Animal.cpp Cat.cpp TestCat.cpp runtestgui.cpp -L/usr/qt/3/lib -I/ usr/qt/3/include -lqt-mt -ldl -lcppunit -lqttestrunner 21 22 Java Example Java Example Animal.java ● Source files (no #includes!) class Animal{ public int getNoOfLegs(){ return 0; } } Cat.java class Cat extends Animal{ public int getNoOfLegs(){ return 5; } } 23 24 Java Example JUnit Output Options TestCat.java ● We don't need to write a tester import junit.framework.*; program – provided program will load public class TestCat extends TestCase{ our class at run-time. ● junit.textui.TestRunner – text output public void testLegs(){ Cat cat = new Cat(); ● junit.awtui.TestRunner – GUI output Assert.assertEquals(4, cat.getNoOfLegs()); } ● junit.swingui.TestRunner – Nicer GUI output protected void setUp(){} } ● Further options, such as XML output, provided by Ant. 25 26 JUnit Example JUnit Results $> java junit.textui.TestRunner TestCat ● javac TestCat.java .F Time: 0.006 ● Invoking JUnit is much easier than There was 1 failure: 1) testLegs(TestCat)junit.framework.AssertionFailedError: CPPUnit. expected:<4> but was:<5> at TestCat.testLegs(TestCat.java:7) ● TestRunner programs are provided at sun.reflect.NativeMethodAccessorImpl.invoke0 (Native Method) which load your bytecode via reflection, at sun.reflect.NativeMethodAccessorImpl.invoke no need to compile your own runners. (NativeMethodAccessorImpl.java:39) at sun.reflect.DelegatingMethodAccessorImpl.invoke ● java junit.textui.TestRunner TestCat (DelegatingMethodAccessorImpl.java:25) ● java junit.awtui.TestRunner TestCat FAILURES!!! Tests run: 1, Failures: 1, Errors: 0 ● java junit.swingui.TestRunner TestCat 27 28 JUnit GUI JUnit GUI Results 29 30 Questions? Building 31 32 Building Make ● Large projects consist of many source ● GNU Make is the most popular tool to files which must be compiled in the solve this problem. correct order. ● You write 'Makefile' which describes all ● This can take hours. If only a few files the dependencies between the source have been edited, we don't need to files. recompile the whole lot. ● You run Make which then runs compiler ● But how do we know which ones to for you. recompile? ● Only files that have changed (and their dependants) are recompiled. 33 34 Make: additional benefits Makefile rules ● Makefile stores info (e.g. flags) making it ● <target>: <dependencies> easy for others to compile your program. <action> ● If you want to change all flags, e.g. to add ● Note the second line must be indented debug info, only one change needed. with a tab (not spaces) ● Make can invoke any command, not just ● compiler. target – name of object file (usually) ● ● Common uses: installation, removing object dependencies – list of other targets files, generating docs, packaging releases ● action – command to update target 35 36 C++ Dependency Tree Make Behaviour ● A target is up-to-date if – a file of the name exists – none of the files listed as dependencies were modified since it was last updated – all its dependencies are up-to-date ● If it is not up-to-date, first its dependencies are updated (recursively), then it is updated, by executing action. ● Make begins by bringing up-to-date the target named on the command line. If none is supplied, Make uses the first target in the Makefile. 37 38 Makefile Implicit rules ● Make is (slightly) intelligent – it can infer obvious runtest: Animal.o Cat.o TestCat.o runtest.o actions. g++ runtest.o TestCat.o Cat.o Animal.o -o runtest -ldl -lcppunit runtestgui: Animal.o Cat.o TestCat.o runtestgui.o ● blah.o: blah.c g++ runtestgui.cpp TestCat.o Cat.o Animal.o -o runtestgui -ldl \ -lcppunit -L/usr/qt/3/lib -lqt-mt -lqttestrunner implies blah.o: blah.c Animal.o: Animal.cpp Animal.h gcc -c -o blah.o blah.c g++ Animal.cpp -c -o Animal.o Cat.o: Cat.cpp Cat.h Animal.h ● If you do not specify any rule for a dependency, g++ Cat.cpp -c -o Cat.o Make will search its list of implicit rules and TestCat.o: TestCat.cpp TestCat.h Cat.h g++ TestCat.cpp -c -o TestCat.o attempt to find one that will produce the needed runtest.o: TestCat.h runtest.cpp file. g++ runtest.cpp -c -o runtest.o runtestgui.o: TestCat.h ● Thus you can use Make with no Makefile at all! g++ runtestgui.cpp -c -o runtestgui.o -I/usr/qt/3/include $> make test 39 gcc -o test test.c 40 Environment Variables Pseudo-targets ● Inherited from shell environment ● Pseudo-targets are not files, and hence can never be up-to-date. Specifying a pseudo-target ● Define: MY_VAR = some text on the command line will always force make to ● update the listed dependencies and execute the Use: $(MY_VAR) action. ● Implicit rules make use of many ● Common pseudo-targets variables, e.g. – default (must be first target) to specify which – CFLAGS – options for C compiler components are built when no arguments are given – LDFLAGS – options for linker – clean to delete all object files 41 42 Improved Makefile OBJECTS = Animal.o Cat.o TestCat.o Other uses for Make LDFLAGS = -ldl -lcppunit -L/usr/qt/3/lib -lqt-mt -lqttestrunner CPPFLAGS = -I/usr/qt/3/include ● Packaging distribution default: runtest runtestgui ● Running program runtest: $(OBJECTS) runtest.o runtestgui: $(OBJECTS) runtestgui.o ● Running tests automatically Animal.o: Animal.cpp Animal.h ● Cat.o: Cat.cpp Cat.h Animal.h Version control operations TestCat.o: TestCat.cpp TestCat.h Cat.h ● runtest.o: TestCat.h runtest.cpp Running Make again in sub-directories runtestgui.o: TestCat.h runtest.cpp ● Saving you from re-typing long clean: -rm -f $(OBJECTS) runtest.o runtest runtestgui.o runtestgui commands .PHONY: clean 43 44 Java Dependency Tree Makefile generic rules ● Make knows how to compile many languages by default, but it is easy to add new ones. ● %.class : %.java javac $< ● %.pdf : %.ps ps2pdf $< $@ 45 46 Java Makefile Make & Java %.class : %.java javac $< -d /my/classes -sourcepath /my/sources ● Make doesn't support Java by default CLASSPATH=/usr/share/junit/lib/junit.jar:.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages21 Page
-
File Size-