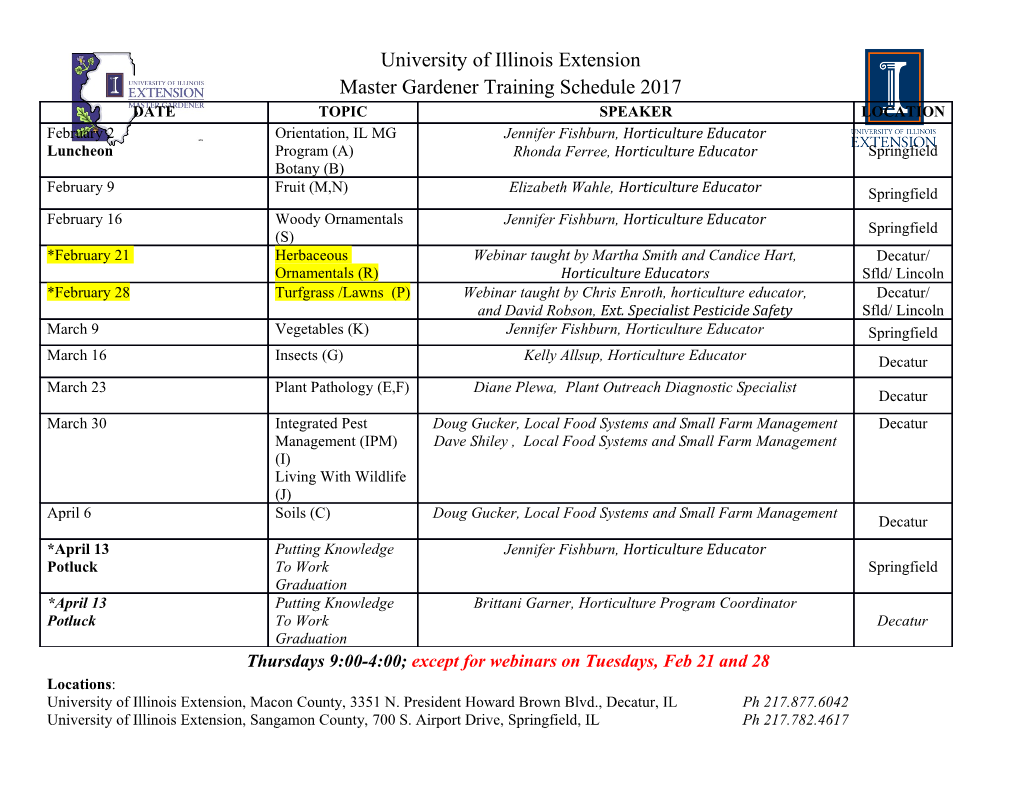
Team Reference Document ACM-ICPC World Finals, 2018 April 15{20, Beijing Contestants: Coach: Filip Bialas doc. Mgr. Zdeněk Dvoøák, Ph.D. Richard Hladík Václav Volhejn Charles University 1 Prologue 1 Treap 6 Link-cut tree 15 trinerdi/base.hpp 1 Counting the number of spanning trees 16 trinerdi/sc.sh 1 Numerical 6 Geometry 16 trinerdi/check.sh 1 Polynomial 6 Binary search 6 Geometric primitives 16 Mathematics (text) 1 Golden section search 6 Point 16 Equations 1 Polynomial roots 6 Line distance 16 Recurrences 1 Determinant 7 Segment distance 16 Trigonometry 2 Linear programming 7 Segment intersection 16 Geometry 2 Linear equations 7 Segment intersection (boolean version) 17 Triangles 2 Linear equations++ 7 Line intersection 17 Quadrilaterals 2 Linear equations in Z 7 2 Point-line orientation 17 Spherical coordinates 2 Matrix inversion 8 Point on segment 17 Derivatives/Integrals 2 FFT 8 Linear transformation 17 Sums 2 Angle 17 Series 2 Number theory 8 Probability theory 2 Fast exponentiation 8 Circles 18 Discrete distributions 2 Primality test 8 Circle intersection 18 Binomial distribution 2 Sieve of Eratosthenes 8 Circle tangents 18 First success distribution 2 Extended Euclid's Algorithm 8 Circumcircle 18 Poisson distribution 2 Modular arithmetic 8 Minimum enclosing circle 18 Continuous distributions 2 Modular inverse (precomputation) 9 Uniform distribution 2 Modular multiplication for ll 9 Polygons 18 Exponential distribution 2 Modular square roots 9 Inside general polygon 18 Normal distribution 3 Discrete logarithm 9 Polygon area 18 Markov chains 3 NTT 9 Polygon's center of mass 18 Number-theoretical 3 Factorization 9 Polygon cut 18 Pythagorean Triples 3 Phi function 10 Convex hull 19 Primes 3 Chinese remainder theorem 10 Polygon diameter 19 Estimates 3 Inside polygon (pseudo-convex) 19 Combinatorics 10 Intersect line with convex polygon (queries) 19 Combinatorial (text) 3 Permutation serialization 10 Misc. Point Set Problems 20 Permutations 3 Derangements 10 Closest pair of points 20 Cycles 3 Binomial coefficient 10 KD tree 20 Involutions 3 Binomial modulo prime 10 Delaunay triangulation 20 Stirling numbers of the first kind 3 Rolling binomial 10 3D point 20 Eulerian numbers 3 Multinomial 10 Polyhedron volume 21 Burnside's lemma 3 3D hull 21 Partitions and subsets 3 Graphs and trees 11 Spherical distance 21 Partition function 3 Bellman{Ford 11 Floyd{Warshall 11 Stirling numbers of the second kind 3 Strings 21 Topological sorting 11 Bell numbers 3 KMP 21 Euler walk 11 Triangles 3 Longest palindrome 21 Goldberg's (push-relabel) algorithm 11 General purpose numbers 3 Lexigographically smallest rotation 21 Min-cost max-flow 11 Catalan numbers 3 Suffix array 21 Edmonds{Karp 12 Super Catalan numbers 4 Suffix tree 22 Min-cut 12 Motzkin numbers 4 String hashing 22 Narayana numbers 4 Globalp min-cut 12 O Aho-Corasick 22 Schr¨odernumbers 4 ( VE) maximum matching (Hopkroft{Karp) 12 O(EV ) maximum matching (DFS) 13 Various 23 Data structures 4 Min-cost matching 13 Bit hacks 23 Order statistics tree 4 General matching 13 Closest lower element in a set 23 Lazy segment tree 4 Minimum vertex cover 13 Coordinate compression 23 Persistent segment tree 4 Strongly connected components 14 Interval container 23 Union-find data structure 4 Biconnected components 14 Interval cover 23 Matrix 5 2-SAT 14 Split function into constant intervals 23 Line container 5 Tree jumps 14 Divide and conquer DP 23 Fast line container 5 LCA 14 Knuth DP optimization 23 Fenwick Tree 5 Tree compression 15 Ternary search 23 2D Fenwick tree 5 Centroid decomposition 15 Longest common subsequence 24 RMQ 6 HLD + LCA 15 Longest increasing subsequence 24 Prologue 67 done Many algorithms in this notebook were taken from the KACTL notebook (with mi- 7d } nor modifications). Its original version is maintained at https://github.com/kth- competitive-programming/kactl. trinerdi/check.sh The sources of this notebook are maintained at https://github.com/trinerdi/icpc- 8a IFS= notebook. Authorship information for each source file and much more can be found bf while read -r a; do there. 83 # Doesn't really work in general (multiline comments are broken, ...), but works well enough f5 printf "%s %s\n" "$(echo "$a" | sed -re 's/\s+|\/\/.*"//g' | md5sum | trinerdi/base.hpp head -c2)" "$a" 67 done 84 #include <bits/stdc++.h> 07 using namespace std; e7 typedef long long ll; Mathematics (text) 5e typedef long double ld; 3e #define rep(i, a, n) for (int i = (a); i < (n); i++) Equations b4 #define per(i, a, n) for (int i = (n) - 1; i >= (a); i--) p de #define FOR(i, n) rep(i, 0, (n)) −b b2 − 4ac ax2 + bx + c = 0 ) x = 2a The extremum is given by x = −b=2a. trinerdi/sc.sh − ec e(){ # run on [problem].in* ed bf ax + by = e x = − f7 t=$(basename `pwd`) ) ad bc 36 if [ "$1" = -d ]; then fl=-g; v=valgrind af − ec cx + dy = f y = 76 else fl=-O2\ -fsanitize=address; v=; fi ad − bc 16 g++ -std=c++11 -lm -Wall -Wno-sign-compare -Wshadow $fl $t.cpp -o $t In general, given an equation Ax = b, the solution to a variable xi is given by c2 for i in $t.in*; do echo $i:; $v ./$t < $i; done 0 7d } det Ai xi = ab create(){ det A 73 for i in {a..z}; do # Change z as needed 0 where Ai is A with the i'th column replaced by b. 15 mkdir "$i" && cd "$i" || continue 16 cp -n ../template.cpp "$i".cpp; touch "$i".in1; cd .. Charles University 2 Recurrences Sums k k−1 If an = c1an−1 + ::: + ckan−k, and r1; : : : ; rk are distinct roots of x + c1x + ::: + ck, cb+1 − ca there are d1; : : : ; dk s.t. ca + ca+1 + ::: + cb = ; c =6 1 n n − an = d1r1 + ::: + dkrk : c 1 Non-distinct roots r become polynomial factors, e.g. a = (d n + d )rn. n(n + 1) n 1 2 1 + 2 + 3 + ::: + n = 2 n(2n + 1)(n + 1) Trigonometry 12 + 22 + 32 + ::: + n2 = 6 n2(n + 1)2 sin(v + w) = sin v cos w + cos v sin w 13 + 23 + 33 + ::: + n3 = 4 cos(v + w) = cos v cos w − sin v sin w 2 − 4 4 4 4 n(n + 1)(2n + 1)(3n + 3n 1) tan v + tan w 1 + 2 + 3 + ::: + n = tan(v + w) = 30 1 − tan v tan w v + w v − w sin v + sin w = 2 sin cos 2 2 Series v + w v − w cos v + cos w = 2 cos cos 2 2 x2 x3 ex = 1 + x + + + :::; (−∞ < x < 1) (V + W ) tan(v − w)=2 = (V − W ) tan(v + w)=2 2! 3! x2 x3 x4 where V; W are lengths of sides opposite angles v; w. ln(1 + x) = x − + − + :::; (−1 < x ≤ 1) 2 3 4 a cos x + b sin x = r cos(x − ϕ) p x x2 2x3 5x4 a sin x + b cos x = r sin(x + ϕ) 1 + x = 1 + − + − + :::; (−1 ≤ x ≤ 1) p 2 8 32 128 2 2 where r = a + b , ϕ = atan2(b; a). x3 x5 x7 sin x = x − + − + :::; (−∞ < x < 1) 3! 5! 7! Geometry x2 x4 x6 cos x = 1 − + − + :::; (−∞ < x < 1) 2! 4! 6! Triangles Side lengths: a; b; c a+b+c Semiperimeter:p p = 2 Probability theory Area: A = p(p − a)(p − b)(p − c) Let X be a discrete random variable with probability pX (x)P of assuming the value x. abc It will then have an expected value (mean) µ = E(X) = xp (x) and variance Circumradius: R = 4A P x X 2 2 2 2 A σ = V (X) = E(X ) − (E(X)) = (x − E(X)) pX (x) where σ is the standard Inradius: r = p x Length of median (divides triangle into two equal-area triangles): deviation. If X is instead continuous it will have a probability density function fX (x) p and the sums above will instead be integrals with pX (x) replaced by fX (x). 1 2 2 2 Expectation is linear: ma = 2b + 2c − a 2 E(aX + bY ) = aE(X) + bE(Y ) s [ ] ( )2 − a For independent X and Y , Length of bisector (divides angles in two): sa = bc 1 b+c 2 2 sin α sin β sin γ 1 V (aX + bY ) = a V (X) + b V (Y ): Law of sines: a = b = c = 2R Law of cosines: a2 = b2 + c2 − 2bc cos α Law of tangents: a + b tan α+β Discrete distributions = 2 a − b α−β tan 2 Binomial distribution The number of successes in n independent yes/no experiments, each of which yields Quadrilaterals success with probability p is Bin(n; p); n = 1; 2;:::; 0 ≤ p ≤ 1. With side lengths a; b; c; d, diagonals e; f, diagonals angle θ, area A and magic flux ( ) 2 2 − 2 − 2 n − F = b + d a c : p(k) = pk(1 − p)n k p k 4A = 2ef · sin θ = F tan θ = 4e2f 2 − F 2 µ = np; σ2 = np(1 − p) ◦ pFor cyclic quadrilaterals the sum of opposite angles is 180 , ef = ac + bd, and A = (p − a)(p − b)(p − c)(p − d). Bin(n; p) is approximately Po(np) for small p. Spherical coordinates First success distribution z The number of trials needed to get the first success in independent yes/no experiments, each of which yields success with probability p, is Fs(p); 0 ≤ p ≤ 1. r y p(k) = p(1 − p)k−1; k = 1; 2;::: 1 1 − p µ = ; σ2 = x p p2 p x = r sin θ cos ϕ r = x2 + y2 + z2 ( p ) Poisson distribution y = r sin θ sin ϕ θ = acos z= x2 + y2 + z2 The number of events occurring in a fixed period of time t if these events occur with a known average rate κ and independently of the time since the last event is Po(λ); λ = tκ.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages25 Page
-
File Size-