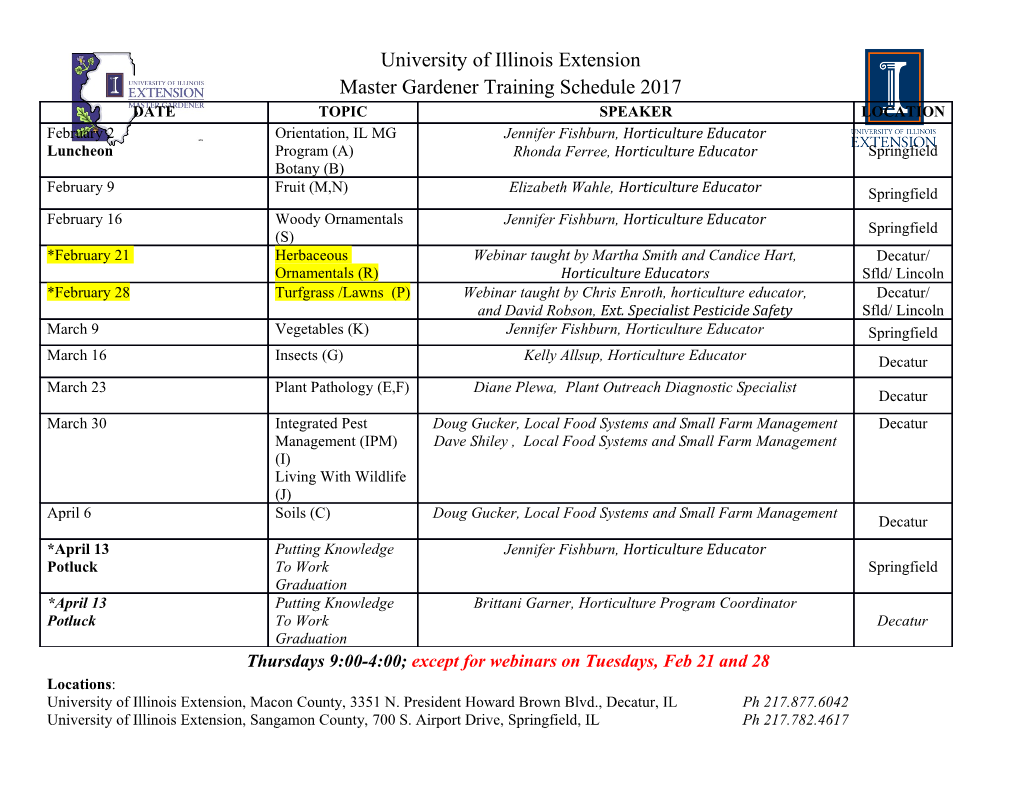
Vulkan Tutorial Alexander Overvoorde April 2020 Contents Introduction 7 About .................................... 7 E-book .................................... 8 Tutorial structure .............................. 8 Overview 10 Origin of Vulkan .............................. 10 What it takes to draw a triangle ..................... 11 Step 1 - Instance and physical device selection ........... 11 Step 2 - Logical device and queue families ............. 11 Step 3 - Window surface and swap chain .............. 11 Step 4 - Image views and framebuffers ............... 12 Step 5 - Render passes ........................ 12 Step 6 - Graphics pipeline ...................... 13 Step 7 - Command pools and command buffers .......... 13 Step 8 - Main loop .......................... 13 Summary ............................... 14 API concepts ................................ 15 Coding conventions .......................... 15 Validation layers ........................... 15 Development environment 17 Windows .................................. 17 Vulkan SDK .............................. 17 GLFW ................................. 19 GLM .................................. 19 Setting up Visual Studio ....................... 20 Linux .................................... 27 Vulkan SDK .............................. 27 GLFW ................................. 29 GLM .................................. 29 Setting up a makefile project .................... 29 MacOS ................................... 33 Vulkan SDK .............................. 33 1 GLFW ................................. 34 GLM .................................. 34 Setting up Xcode ........................... 35 Base code 40 General structure .............................. 40 Resource management ........................... 41 Integrating GLFW ............................. 42 Instance 45 Creating an instance ............................ 45 Checking for extension support ...................... 47 Cleaning up ................................. 48 Validation layers 49 What are validation layers? ........................ 49 Using validation layers ........................... 50 Message callback .............................. 52 Debugging instance creation and destruction .............. 57 Testing ................................... 59 Configuration ................................ 59 Physical devices and queue families 61 Selecting a physical device ......................... 61 Base device suitability checks ....................... 62 Queue families ............................... 64 Logical device and queues 69 Introduction ................................. 69 Specifying the queues to be created .................... 69 Specifying used device features ...................... 70 Creating the logical device ......................... 70 Retrieving queue handles ......................... 72 Window surface 73 Window surface creation .......................... 73 Querying for presentation support .................... 75 Creating the presentation queue ..................... 76 Swap chain 78 Checking for swap chain support ..................... 78 Enabling device extensions ........................ 80 Querying details of swap chain support .................. 80 Choosing the right settings for the swap chain .............. 82 Surface format ............................ 82 Presentation mode .......................... 83 Swap extent .............................. 84 2 Creating the swap chain .......................... 85 Retrieving the swap chain images ..................... 89 Image views 91 Introduction 94 Shader modules 98 Vertex shader ................................ 99 Fragment shader .............................. 101 Per-vertex colors .............................. 101 Compiling the shaders ........................... 103 Loading a shader .............................. 104 Creating shader modules .......................... 106 Shader stage creation ........................... 107 Fixed functions 109 Vertex input ................................ 109 Input assembly ............................... 110 Viewports and scissors ........................... 110 Rasterizer .................................. 112 Multisampling ............................... 113 Depth and stencil testing ......................... 113 Color blending ............................... 114 Dynamic state ............................... 116 Pipeline layout ............................... 116 Conclusion ................................. 117 Render passes 118 Setup .................................... 118 Attachment description .......................... 118 Subpasses and attachment references ................... 120 Render pass ................................. 121 Conclusion 123 Framebuffers 126 Command buffers 129 Command pools .............................. 129 Command buffer allocation ........................ 131 Starting command buffer recording .................... 132 Starting a render pass ........................... 133 Basic drawing commands ......................... 134 Finishing up ................................. 134 Rendering and presentation 136 3 Setup .................................... 136 Synchronization ............................... 136 Semaphores ................................. 137 Acquiring an image from the swap chain ................. 138 Submitting the command buffer ...................... 139 Subpass dependencies ........................... 140 Presentation ................................. 141 Frames in flight ............................... 143 Conclusion ................................. 150 Swap chain recreation 151 Introduction ................................. 151 Recreating the swap chain ......................... 151 Suboptimal or out-of-date swap chain .................. 154 Handling resizes explicitly ......................... 155 Handling minimization ........................... 156 Vertex input description 158 Introduction ................................. 158 Vertex shader ................................ 158 Vertex data ................................. 159 Binding descriptions ............................ 159 Attribute descriptions ........................... 160 Pipeline vertex input ............................ 162 Vertex buffer creation 163 Introduction ................................. 163 Buffer creation ............................... 163 Memory requirements ........................... 165 Memory allocation ............................. 167 Filling the vertex buffer .......................... 168 Binding the vertex buffer ......................... 169 Staging buffer 172 Introduction ................................. 172 Transfer queue ............................... 172 Abstracting buffer creation ........................ 173 Using a staging buffer ........................... 174 Conclusion ................................. 177 Index buffer 178 Introduction ................................. 178 Index buffer creation ............................ 179 Using an index buffer ........................... 181 Descriptor layout and buffer 183 Introduction ................................. 183 4 Vertex shader ................................ 184 Descriptor set layout ............................ 185 Uniform buffer ............................... 187 Updating uniform data ........................... 189 Descriptor pool and sets 192 Introduction ................................. 192 Descriptor pool ............................... 192 Descriptor set ................................ 194 Using descriptor sets ............................ 196 Alignment requirements .......................... 198 Multiple descriptor sets .......................... 200 Images 201 Introduction ................................. 201 Image library ................................ 202 Loading an image .............................. 203 Staging buffer ................................ 205 Texture Image ............................... 205 Layout transitions ............................. 210 Copying buffer to image .......................... 213 Preparing the texture image ........................ 214 Transition barrier masks .......................... 215 Cleanup ................................... 217 Image view and sampler 218 Texture image view ............................. 218 Samplers .................................. 220 Anisotropy device feature ......................... 225 Combined image sampler 226 Introduction ................................. 226 Updating the descriptors .......................... 226 Texture coordinates ............................ 228 Shaders ................................... 230 Depth buffering 235 Introduction ................................. 235 3D geometry ................................ 235 Depth image and view ........................... 238 Explicitly transitioning the depth image .............. 242 Render pass ................................. 243 Framebuffer ................................. 244 Clear values ................................. 245 Depth and stencil state .......................... 245 Handling window resize .......................... 247 5 Loading models 249 Introduction ................................. 249 Library ................................... 249 Sample mesh ................................ 250 Loading vertices and indices ........................ 251 Vertex deduplication ............................ 255 Generating Mipmaps 258 Introduction ................................. 258 Image creation ............................... 259 Generating Mipmaps ...........................
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages285 Page
-
File Size-