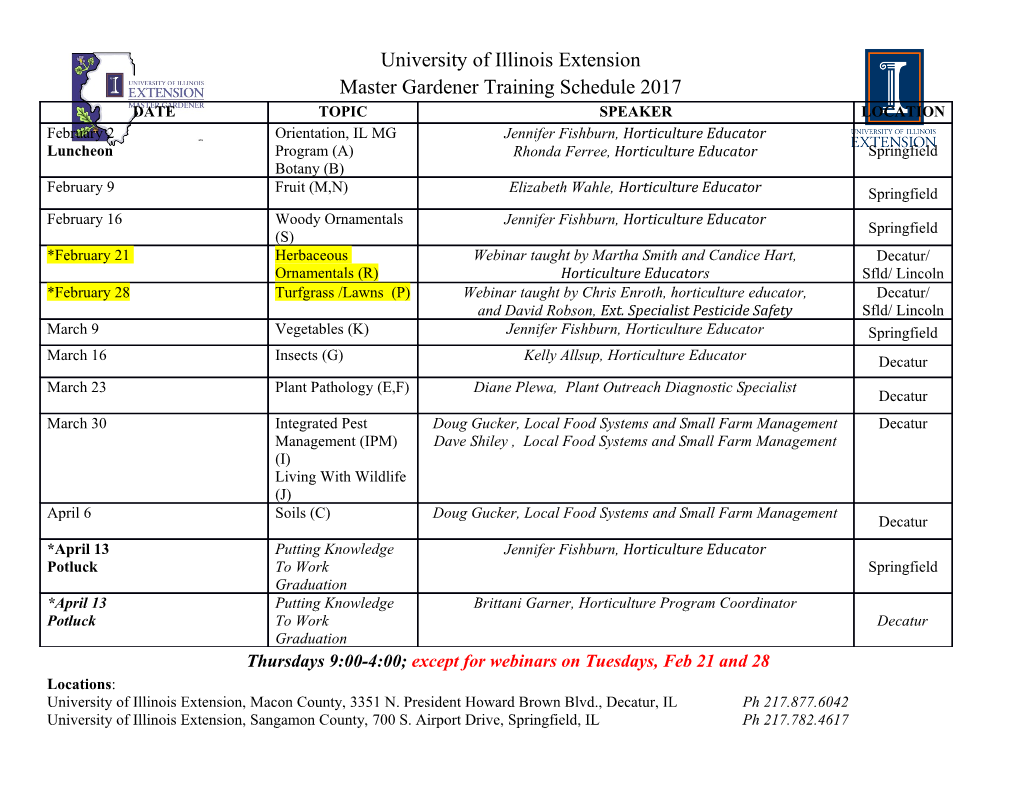
Using Python for Interactive Data Analysis Perry Greenfield Robert Jedrzejewski Space Telescope Science Institute June 13, 2005 1 Copyright (c) 2005, Association of Universities for Research in Astronomy, Inc (AURA). All rights reserved. 2 Contents Purpose 7 Prerequisites 7 Practicalities 7 1 Reading and manipulating image data 8 1.1 Example session to read and display an image from a FITS file . 8 1.2 Starting the Python interpreter . 8 1.3 Loading modules . 8 1.4 Reading data from FITS files . 9 1.5 Displaying images . 9 1.6 Array expressions . 9 1.7 FITS headers . 10 1.8 Writing data to FITS files . 10 1.9 Some Python basics . 11 1.9.1 Memory vs. data files . 11 1.9.2 Python variables . 11 1.9.3 How does object oriented programming affect you? . 11 1.9.4 Errors and dealing with them . 12 1.10 Array basics . 13 1.10.1 Creating arrays . 13 1.10.2 Array numeric types . 14 1.10.3 Printing arrays . 14 1.10.4 Indexing 1-D arrays . 15 1.10.5 Indexing multidimensional arrays . 16 1.10.6 Compatibility of dimensions . 16 1.10.7 ufuncs . 17 1.10.8 Array functions . 18 1.10.9 Array methods . 20 1.10.10 Array attributes: . 21 1.11 Example . 21 1.12 Exercises . 21 2 Reading and plotting spectral data 23 2.1 Example session to read spectrum and plot it . 23 2.2 An aside on how Python finds modules . 23 2.3 Reading FITS table data . 24 2.4 Quick introduction to plotting . 25 2.4.1 Simple x-y plots . 25 2.4.2 Labeling plot axes . 26 2.4.3 Overplotting . 27 2.4.4 Legends and annotation . 28 2.4.5 Saving and printing plots . 28 2.5 A little background on Python sequences . 29 2.5.1 Strings . 29 2.5.2 Lists . 31 2.5.3 Tuples . 31 2.5.4 Standard operations on sequences . 32 2.5.5 Dictionaries . 32 2.5.6 A section about nothing . 34 2.6 More on plotting . 34 2.6.1 matplotlib terminology, configuration and modes of usage . 34 3 2.6.2 matplot functions . 35 2.7 Plotting mini-Cookbook . 36 2.7.1 customizing standard plots . 36 2.7.2 implot example . 38 2.7.3 imshow example . 39 2.7.4 figimage . 40 2.7.5 histogram example . 40 2.7.6 contour example . 41 2.7.7 subplot example . 42 2.7.8 readcursor example . 43 2.8 Exercises . 44 3 More advanced topics in PyFITS, numarray and IPython 45 3.1 IPython . 45 3.1.1 Obtaining information about Python objects and functions . 45 3.1.2 Access to the OS shell . 46 3.1.3 Magic commands . 47 3.1.4 Syntax shortcuts . 48 3.1.5 IPython history features . 49 3.2 Python Introspection . 49 3.3 Saving your data . 50 3.4 Python loops and conditionals . 50 3.4.1 The for statement . 50 3.4.2 Blocks of code, and indentation . 51 3.4.3 Python if statements . 51 3.4.4 What’s True and what’s False . 52 3.4.5 The in crowd . 52 3.5 Advanced PyFITS Topics . 52 3.5.1 Header manipulations . 52 3.5.2 PyFITS Object Oriented Interface . 53 3.5.3 Controlling memory usage . 53 3.5.4 Support for common FITS conventions . 54 3.5.5 Noncompliant FITS data . 54 3.6 A quick tour of standard numarray packages . 55 3.6.1 random_array . 55 3.6.2 fft . 56 3.6.3 convolve . 57 3.6.4 linear_algebra . 57 3.6.5 MA (Masked Arrays) . 57 3.6.6 nd_image (Multi-dimensional array processing) . 58 3.6.7 ieespecial . 58 3.7 Intermediate numarray topics . ..
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages136 Page
-
File Size-