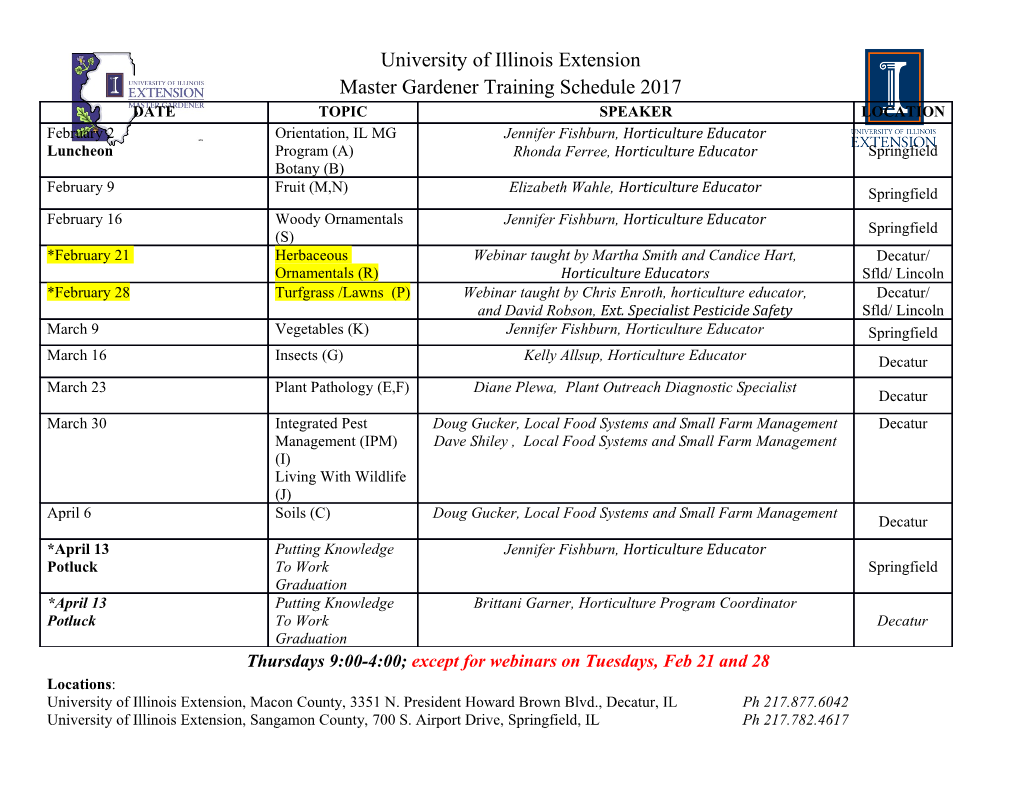
www.YoYoBrain.com - Accelerators for Memory and Learning Questions for Objective-C Category: Default - (44 questions) Objective-C: to create an object you send a alloc messageNSMutableArray ___ to a class *arrayInstance = [NSMutableArray alloc]; Objective-C: the first message you always init send to a newly allocated message is ____ Objective-C: normal way to combine alloc NSMutableArray *arrayInstance = and init when creating object [[NSMutableArray alloc] init] Objective-C: to destroy an object, send it the release message ____ Objective-C: what to do after [arrayInstance arrayInstance = nil; release] Objective-C: type of array that lets you add / NSMutableArray remove objects Objective-C: how to add an object to addObject NSMutableArray Objective-C: how to put an object in a [items insertObject:@"xx" atIndex] particular spot in NSMutableArray Objective-C: grab an object at particular objectAtIndex:count index in NSMutableArray Objective-C: how to send string output to log NSLog(@"my message"); Objective-C: the C language retains all its the @ prefix keywords and any keywords from Objective-C are distinguishable by ____ Objective-C: to declare a class, you use @interfacename of the new class keyword ___ followed by _____ Objective-C: how to declare getter / setter @property int fido; accessors to a variable (ex: int fido) Objective-C: how to control whether lock attribute atomic / nonatomic@property must be obtained to getter or setter variable (nonatomic) int something; with @property Objective-C: file type for implementation of .m extension for implementation file header declarations Objective-C: how to start and finish @implementation MyClass@end implementation of @interface in .m file Objective-C: how to declare a method is an - in first line of code- (NSString *) instance method (not class) description{} Objective-C: at top of implementation file import the header (.h) file of the class you always _____ Objective-C: how to invoke method in super [super method]; class Objective-C: how to reserve / return memory char *buffer = malloc(100);free(buffer); with C syntax Objective-C: what is malloc / free replaced malloc => allocfree => dealloc with in Objective-C Objective-C: you can mark an object for autorelease future release by sending it the message ______ Objective-C: how to increase a variable's retain message[d retain]; reference count Objective-C: how to decrease a variables [d release] reference count explicitly Objective-C: when using @property how to retain property@property (retain) Dog *pet; have compiler generate proper retain / release for memory management Objective-C: how to relinquish control of [myVar autorelease] object and allow it to be garbage collected when everyone else finished Objective-C: how to declare which protocol listing the names of the protocols in a a class conforms to comma delimited list in angle brackets after the name of the superclass@interface MyController: NSObject <UITextDelegate, SomeOtherDelegate> Objective-C: terminology for interfaces protocols Objective-C: how to declare methods in @optional -method1; -method2; protocol that are not required Objective-C: define a retain cycle when a child retains a reference to a parent, prevent parent from responding to release call Objective-C: define - blocks language level feature added to C, Objective-C and C++, which allow creation of distinct segments of code that can be passed around to methods or functions as if they were values. Similar to lambdas or closures in other languages Objective-C: syntax to define a block literal use the caret ^ symbol^{ NSLog(@"This is a block"); } Objective-C: syntax to declare a variable to void (^simpleBlock)(void); keep track of a block Objective-C: how to declare a block that ^double (double firstValue, double takes 2 doubles and returns a double secondValue) { return firstValue*secondvalue;} Objective-C: syntax to allow the changing of use _ block storage type modifier_block int a captured variable from within the block anInteger=42; Objective-C: meaning of syntax in expected (void (^)(void)) specifies a block that doesn't parameter-(void) take any segments or return any values beginTaskWithCallbackBloc(void (^)(void)) ... { } Objective-C: 2 main task-scheduling operation queuesGrand Central Dispatch mechanisms are _____ and ____ Objective-C: For operation queues ____ NSOperationNSOperationQueue object to create an instance to encapsulate a unit of work, and then add that operation to ____ for execution Objective-C: Use ___ to create an operation NSBlockOperation using a block Objective-C: If you need to schedule an dispatch queuesGrand Central Dispatch arbitrary block of code for execution, you can (GCD) work directly with ____ controlled by ______ Objective-C: how to get a reference to dispatch_get_global_queue existing queue using GCD Objective-C: to dispatch a block to a GCD dispatch_asynch()dispatch_synch() queue Objective-C: class for creating Queue NSInvocationOperation operation that you use as-is to create an operation based on an object and selector from your application Objective-C: define - selector name used to select a method to execute for an object, or the unique identifier that replaces the name when the source code is compiled Category: Extensions to C - (16 questions) XCode - shortcut to pull up the XCode Cmd - Shift - R console window for standard i/o Objective-C: file extension for source code .m Objective-C: how to include header files #import <Foundation/Foundation.h> Objective-C: Logs an error message to the NSLog( NSString ); Apple System Log facility Objective-C: how to designate a string literal @"my string" Objective-C: type for a sequence of NSString characters in Cocoa Objective-C: type for boolean value BOOL Objective-C: syntax for sending a message [ object, action] to an object Objective-C: how to define an interface @interface name : superClass{ type data1; type data2;} Objective-C: syntax for method declarations - (returnType) methodName: (type) var1, ... ; for interfaces Objective-C: syntax for implementation of @implementation className@end class Objective-C: syntax to create a class [className new] Objective-C: method that gets called when init new object is created Objective-C: syntax for getter and setter for prop1- (Type *) prop1;- (void) setProp1: methods (Type *) newProp1; Objective-C: syntax for getter / setters for - void setTire: (Tire *) tire atIndex: (int) property that is array - like Tire *tires[4] index;- (Tire *) tireAtIndex: (int) index; Objective-C: syntax for not importing a @class className; class but saying we are referring to it via a pointer Category: Xcode - (14 questions) Objective-C: Xcode shortcut to open a Command - Option - Shift - D import source file Objective-C: Xcode shortcut to add a book Command D mark Objective-C: Xcode shortcut to show the Esc completion menu Objective-C: Xcode shortcut to cycle forward Control . (period)Shift Control . (period) / backward through the code completions Objective-C: Xcode how to search Option - Double Click documentation for keyword Objective-C: Xcode shortcut to run the Command Y program with debugger Objective-C: what is NSRange struct used to represent a range of things, characters in a string or items in an arrayunsigned int location;unsigned in length; Objective-C: how to create a NSRange NSRange range = {17, 4};NSRange range = NSMakeRange (17, 4); Objective-C: syntax to created a formatted [NSString stringWithFormat: @"Your height string with variables is %d feed, %d inches", 5, 11] Objective-C: syntax to create a class + (id) myFunction: .....starts with plus sign method Objective-C: how to get length of string [myString length] Objective-C: how to compare strings for - (BOOL) isEqualToString: (NSString *) equality aString; Objective-C: how to compare a string - (NSComparisonResult) compare: (NSString character by character to another *) string; Objective-C: 3 string comparison methods - (BOOL) hasPrefix: (NSString *) aString;- to search for substrings (BOOL) hasSuffix: (NSString *) aString;- (NSRange) rangeOfString: (NSString *) aString; Category: Foundation Kit - (12 questions) Objective-C: class for mutable strings NSMutableString Objective-C: syntax to create a mutable + (id) stringWithCapacity: (unsigned) string with initial capacity of 50 capacity; Objective-C: class for ordered list of objects NSArray Objective-C: how to create an NSArray with [NSArray arrayWithObjects: @"one", @"two", initial objects @"three", nil]; Objective-C: how to fetch an object at an - (id) objectAtIndex: (unsigned int) index; index from NSArray Objective-C: array class that can have NSMutableArray mutable number of objects Objective-C: class used for iteration over a NSEnumerator collection Objective-C: how to get a NSEnumerator - (NSEnumerator *) objectEnumerator; from NSArray object Objective-C: syntax for fast enumeration for (NSString *string in array) {} Objective-C: object with key / value map NSDictionary Objective-C: class used to put structures NSValue into NSArrays Objective-C: class to represent nil values NSNull Category: Memory Management - (16 questions) Objective-C: basic pattern for accessor - (void) setXxx: (Xxx *) newXxx{ [newXxx memory management when using objects retain]; [Xxx release]; Xxx = newXxx;} Objective-C: method call to put a memory - (id) autorelease; release into the NSAutoreleasePool Objective-C: OS X 10.4 and higher - (init) drain NSAutoreleasePool method for issuing a pool release without destroying pool Objective-C: older method for removing all [pool release]; memory
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages7 Page
-
File Size-