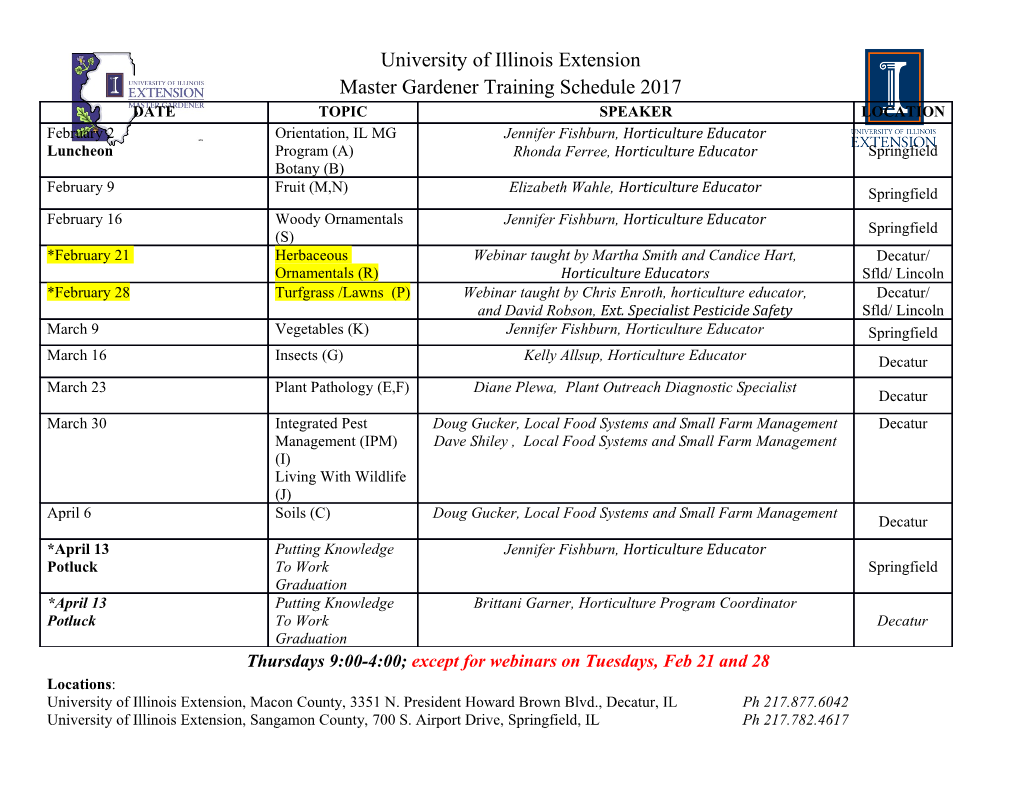
Design and implementation of virtualized sparse voxel octree ray casting Master Thesis Computer Science Lund University, Faculty of Engineering by Markus Arvidsson December 2010 ABSTRACT Sparse voxel octree ray casting is an alternative rendering technique to rasteri- zation for static geometry. The hierarchical structure of the octree enables some nice properties for the ray casting method: the traversal of the octree data has a logarithmic time complexity and level of detail is handled automatically by terminating the traversal at a lower depth level. Furthermore, the octree data structure is well suited for dynamic streaming and virtualization since both the geometry and the material data can be stored in one data structure. It is possi- ble that in the future highly detailed scenes with complex geometry and unique texturing will perform better with sparse voxel octree ray casting than with ras- terization of indexed triangle meshes. On the negative side, the memory re- quirements are generally higher and the image quality is currently not as good as rasterization with triangle meshes. In this thesis, we design and implement a method for virtualized sparse voxel octree ray casting. We compare our method to other published methods and discuss the differences as well as present some ideas for future work. A novel octree traversal algorithm is also described (but not performance evaluated) for a SIMD type of instruction set. ACKNOWLEDGEMENTS I would like to thank my supervisor, Tomas Akenine-Moller,¨ whose guidance helped me from the initial to the final version of this thesis. I would also like to thank my family and friends for giving me support. iii TABLE OF CONTENTS Acknowledgements . iii Table of Contents . iv 1 Background 1 1.1 Real-Time Rendering . .1 1.1.1 Rasterization . .1 1.1.2 Ray Tracing . .4 1.1.3 Ray Casting and Hybrid Rendering . .5 2 The Octree Data Structure 6 2.1 CPU Traversal . .7 2.2 Multi-Core Parallel Traversal . 12 2.3 GPU Traversal . 15 2.3.1 GPU Traversal: Implementation of a Benchmark Algorithm 15 3 Sparse Voxel Octrees as Static Geometry 16 3.1 Sparse Voxel Octree Geometry Format . 16 3.1.1 Comparison with a Triangle Mesh . 18 3.1.2 Voxelization of a Triangle Mesh . 19 3.2 Virtualization of the Static Geometry . 22 3.2.1 Virtualization System Overview . 22 3.2.2 Virtualized Sparse Voxel Octree . 24 3.2.3 Run-Time Loading . 27 4 Sparse Voxel Octree Rendering 32 4.1 Integration With a Traditional Pipeline . 32 4.2 Sparse Voxel Octree Ray Casting . 32 5 Implementation and Results 34 5.1 Voxelization and Virtualization . 34 5.2 Run-Time Environment . 35 5.3 Results and Discussion . 38 6 Future Work 41 6.1 Image Quality Improvements . 41 6.1.1 Voxelization Filtering . 41 6.1.2 Spherical Voxel Traversal . 41 6.1.3 Screen Space Post-Processing . 42 6.2 Performance Improvements . 42 6.2.1 Faster Dynamic Streaming . 43 6.2.2 Data Compression . 44 7 Conclusion 46 iv A Appendix 47 A.1 Pseudocode . 47 Bibliography 52 v 1 BACKGROUND 1.1 Real-Time Rendering Image rendering is the process of synthesizing images from geometric mod- els. The result of the rendering process for one image is commonly called a frame. Realistic rendering techniques attempt to approximately solve the ren- dering equation [1]. In real-time rendering, the duration to render a frame needs to be short (usually around 15-30 ms) to get the impression that the rendering is instantaneous or in real-time. An introduction and reference to the subject of real-time rendering is given in [2]. The above description of real-time rendering is somewhat vague; in practice, real-time rendering is often used in applications that has some kind of user input that influence the rendering. Video games is currently the largest application field for real-time rendering but its use in some other areas are growing [3]. In this section, we will discuss the different fundamental rendering techniques currently used in real-time rendering as well as some techniques that could po- tentially become useful when the hardware has developed further. We also dis- cuss the advantages and disadvantages of each method. 1.1.1 Rasterization Rasterization is by far the most frequenty used technique for real-time render- ing. The rasterizaton pipeline transforms geometric primitives by a projection transformation from camera space to screen space. The primitives are stored in a vector graphics format and are after the projection converted to a raster image format, hence the name rasterization. Indexed triangle meshes are in almost all implementations used as the vector graphics format for the primitives. Rasterization renderers can be categorized into two main rendering types: for- ward rendering and deferred rendering. They differ mainly in how they calcu- late lights and shadows in the scene. Another term for the light and shadow calculations is shading. A forward renderer generally calculates the shading for the objects one object at a time. Multiple rendering passes might be needed if many lights are simultaneously affecting the object. A deferred renderer works 1 differently: instead of calculating the shading for the object it stores the normal and other lightning and shadow properties to a render target buffer. This is done only for the pixels that pass the Z-buffer test. Those properties are then used in the shading stage when the final color for each pixel is calculated. In the shading state, all lights in the scene are iterated over and we calculate and add for each light the contribution to the final pixel color. Each light’s contribution is calculated by taking the stored properties into account. There are advantages and disadvantages with each type of renderer; the strengths of deferred shading is generally maximized in scenes with many lights influencing a large number of objects. On the other hand, an outdoor scene with the sun as the only light source will most likely perform better with forward shading. Modern graphics processors run the rasterization pipeline [4] in parallel on mul- tiple execution units. Each triangle is processed independently from the other triangles and as a consequence each primitive is processed independently from the other primitives. What this means in practice is that rasterization is very cache friendly; the reason being that the vertices for an indexed triangle mesh are stored linearly and each mesh can be processed sequentially without the need of any data from another mesh. However, because of the same indepen- dence between the primitives, the rasterization algorithms can not use any data that is stored within another triangle or within another primitive; if such data is needed it has to be calculated before the rendering of the frame is started and then made available by storing the data in separate buffers. Another issue with the current rasterization pipeline implementations is that they are using normal maps to enhance geometric detail. While this technique improves the image quality significantly it still needs directional lights and the right view angle to give the desired effect. At some point in the future this il- lusional illumination effect will be replaced with real geometry. Displacement maps [5] in combination with micropolygon tessellation [6] are currently the dominant technique to increase real geometric detail. However, indexed trian- gle meshes are not a good choice if the triangle size is smaller than a pixel since the rasterization interpolation does not work well in that case. Furthermore, indexed triangle meshes are not the most memory efficient format for small tri- angles since no matter the size of the triangle three vector coordinates and three integer coordinates have to be stored. Another issue is that displacement maps are not well suited for future ray tracing [7] techniques since the displacement maps have to be evaluated in order to produce correct results. These drawbacks 2 and restrictions have spawned some research into alternative geometry formats. We will later discuss the octree geometry format which partly addresses these problems. Despite these restrictions, the performance and image quality that the raster- ization hardware and algorithms have been able to produce have improved significantly every year for the last twenty years. While this development is expected to continue for the foreseeable future, there may at some point be a need for real-time rendering algorithms and visual effects that will work better if implemented with a technique that does not have the independent triangles restriction. For instance, some reflection and refraction effects are hard to cal- culate without access to other primitives. Ray tracing - a technique that we will discuss in the next section - has access to the whole scene and is often utilized in off-line rendering for similar optical effects. 3 1.1.2 Ray Tracing Ray tracing [7] is a rendering technique that generates the resulting image by simulating light paths. The light path can start from the camera or from the light source (see Figure 1.1). L i g h t s o u r c e V i e w R a y S h a d o w R a y Figure 1.1: Ray tracing. Simulating a light path requires in most cases access to multiple surfaces since some of the light energy will reflect and sometimes also refract when the light reaches a surface. Note that this is different from rasterization where each sur- face can be rendered independently. Because of the surface reflections, ray trac- ing can achieve a higher image quality than rasterization for scenes with indirect illumination. Furthermore, ray tracing can render optical effects such as reflec- tion, refraction and caustics.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages58 Page
-
File Size-