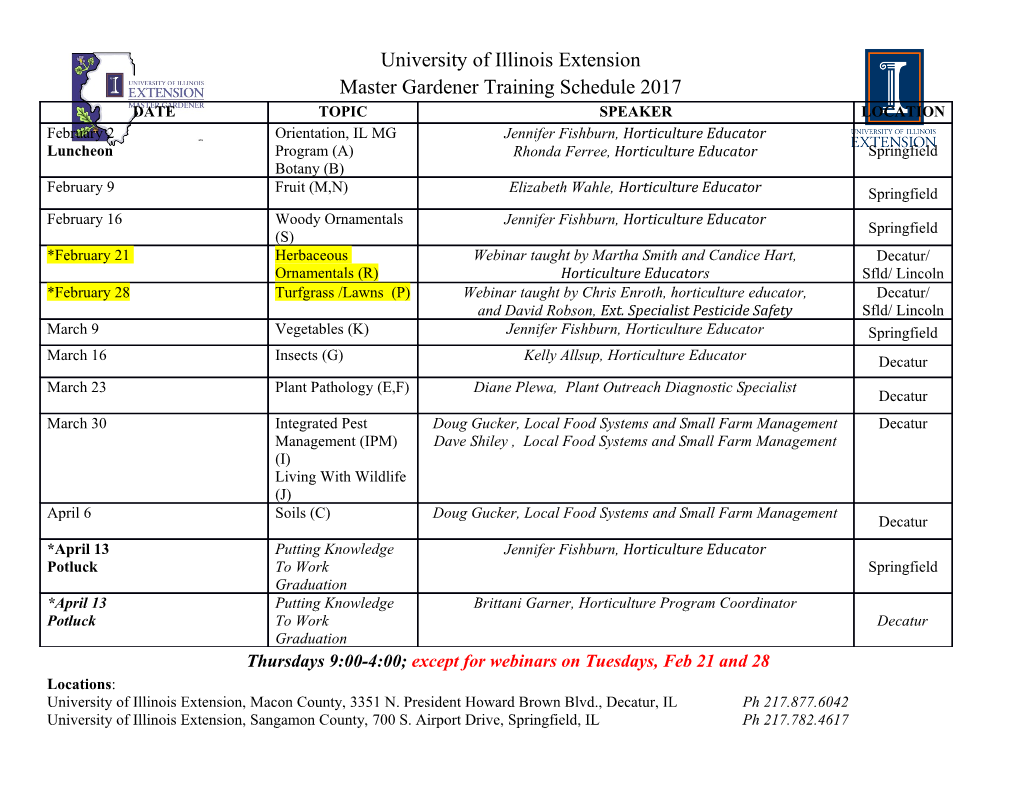
Galil Motion Control Command Reference Galil Motion Control 270 Technology Way Rocklin, California 95765 Phone:(916) 626-0101 Fax:(916) 626-0102 Email: [email protected] Web: www.galilmc.com Hardware Command Reference Edition 5/24/2011 8:37:49 AM (svn 395) ● Including commands that apply to: ❍ All ❍ DMC40x0 ❍ SINEAMP ● Including command details that apply to: ❍ All ❍ DMC40x0 Overview This command reference is a supplement to the Galil User Manual. Resources on www.galilmc.com Printable version Product Manuals Application Notes Newest Firmware Sample DMC code Learning Center Support and Downloads What is DMC code? DMC (Digital Motion Controller) code is the programming language used for all Galil hardware. It is a high-level, interpreted language which is simple to learn and use, yet is surprisingly powerful. Actively developed and refined since 1983, DMC code provides functionality that is particularly well suited to motion control and PLC applications. DMC code can be used manually from a terminal, programmatically from an external device or customer application, and can be fully embedded into a Galil controller's memory to leverage powerful "embedded-only" features and for stand-alone applications. DMC code of course provides symbolic variables, arrays, and math support. The elegance of DMC coding is particularly evident when writing code for embedded applications. When running on the controller, the DMC language supports if-then-else conditionals, code branching, subroutines, a call stack (with parameter passing and local variable scope on some models), multi-threading, and automatic subroutines (i.e. event-driven programming). DMC code runs on the Galil Real Time Operating System (RTOS) which is specifically designed for Galil hardware and for motion control. The learning curve on DMC code is quite fast, usually less than one hour to basic motion, so called, "spinning motors". It is the fastest to learn, the easiest, the simplest, and one of the most flexible and powerful languages in the industry. Don't forget, Galil's Applications Support Team is available to assist you; from the most basic question to the most complicated needs. Top Down: How is a Galil system normally structured? However you want, there are three general approaches to Galil programming. file:///C|/Documents%20and%20Settings/Andy/Desktop/Temp/4Kcom/index.html (1 of 358)5/24/2011 8:38:16 AM Galil Motion Control Command Reference Embedded/Galil-centric Programming In this approach, a host computer is only used during development to program the controller. The program is then downloaded and burned to non- volatile flash using the #AUTO automatic subroutine to indicate where code execution should start on boot-up. The Galil controller will now run "standalone," not requiring any intervention from the host. Note that for serial and Ethernet controllers, the standalone controller can still actively work with other controllers in a network, without host intervention. PCI and other PC bus-based controllers support this approach, although still require the PCI bus for power. GalilTools (GT) is provided as a programming environment for developing embedded applications. Host-centric Programming If a GUI or other frontend is desired to be run on a host, all development can be conducted on the host PC, with the architecture, operating system, and programming language of choice. In this approach, the controller receives every command from the host PC, nothing is running embedded. Many Galil firmware features are available to facilitate host-centric programming including mode-of-motion buffers, data logging buffers, asynchronous data record updates from the controller, PCI and UDP interrupt events, and more. GalilTools (GT) is bundled with a programming library (API) for programming applications from a host. Many popular operating systems and languages are supported. Hybrid Programming Perhaps the most versatile approach to Galil system design, the Hybrid approach allows for both embedded code and host-side code to work in tandem. Typically an application is developed for embedded use in DMC code. The code incorporates all of the detail of an application but relies on the host to provide it data. Through variables, arrays, and other commands, the host is able to define the bounds of the embedded algorithms. The host plays a supervisory role, interrogating status, receiving asynchronous updates from the controller, starting and stopping threads, and so on. The controller takes care of the motion and I/O responsibilities based on its embedded program, and the controller's real time operating system (RTOS) ensures that the application won't suffer from indeterminacy which is common on general purpose PC operating systems (e.g. Microsoft Windows). Because the controller takes care of the details, the host is able to use its resources on other tasks, such as complicated number crunching or user interface. It is noteworthy that Galil Standalone controllers (e.g. DMC-40x0, DMC-41x3, DMC-21x3, RIO-47xxx) can leverage the Ethernet to provide powerful modularity. Using any of the above three system approaches, multiple controllers can work in concert to achieve an application's requirements. Networked controllers also provide easy scalability. Need some more digital or analog I/O? Add an RIO. Need another axis of control? Add another DMC to the network. Both the Galil firmware and the Galil software libraries provide features which allow easy use of multiple controllers on an Ethernet network. RS232/422/485 networks are also possible. Bottom Up: Anatomy of DMC code Classification DMC language can be broken up into the following general classifications Classification Description Examples Example Comments Create a TCP connection on Ethernet handle The command receives its arguments Explicit Notation Only IHC=192,168,1,101<1070>2 C to a device at IP address 192.168.1.101 on only by assignment with the "=" operator. port 1070 The command receives its arguments Implicit Notation Only IA 192,168,1,102 Set the local IP address to 192.168.1.102 only by an implicit argument order. The command receives its arguments either by an explicit assignment using the KPA=64;KPB=32;KPH=128 Assign the proportional constant (KP) of the Explicit & Implicit "=" symbol, or an implicit argument KP 64,32,,,,,,128 PID filter to three different axes. order. The command receives its arguments as Stop (ST) axes A, D and F. Leave other axes Accepts Axis Mask ST ADF a string of valid axis names. running. file:///C|/Documents%20and%20Settings/Andy/Desktop/Temp/4Kcom/index.html (2 of 358)5/24/2011 8:38:16 AM Galil Motion Control Command Reference Burn (BN) controller parameters to flash Two Letter Only The command accepts no arguments BN memory Operators take two arguments and +,-,*,/ Operators Operator or Comparator produce a result. Comparators take two =,<,>,<=,>=,<> Comparators values and return a Boolean (1 or 0). Trig functions Sine and ArcSine Starting with the @ character, these @SIN,@ASIN I/O functions Analog in and Digital in @ Function functions take one argument and perform @AN,@IN Numerical functions Round, Fractional Part, a function, returning its result @RND,@FRAC,@COM Bitwise compliment Not valid from the terminal, or from PC- IF,ELSE,ENDIF IF Conditionals Embedded Only side code, these commands are used in JS,JP Jump commands embedded DMC code only EN, RE End program, Return from Error Operands hold values, and are not valid _TPA Current position of axis A encoder on their own. They can be used as Operand _LFC Forward limit state on C axis arguments to commands, operators or _TC Current Error code comparators Trippoints hold up a thread's execution WT 1000 Wait 1000 ms (block) until a certain condition occurs. Trippoint AMA Wait until axis A completes profiled motion These are a special case of Embedded AI1 Wait for input one to go high Only type commands. There are three types of comments: REM, ', Comment Comments are used to document code. 'This is a comment and NO DMC code is case sensitive. All Galil commands are uppercase. User variables and arrays can be upper-case or lower case. Galil recommends that array and variable names contain at least one lower-case character to help distinguish them from commands. Explicit Notation These commands specify data using an axis designator followed by an equals sign. The * symbol can be used in place of the axis designator. The * defines data for all axes to be the same. For example: Syntax Description PRB=1000 Sets B axis data at 1000 PR*=1000 Sets all axes to 1000 Implicit Notation These commands require numerical arguments to be specified following the instruction. Values may be specified for any axis separately or any combination of axes. The comma delimiter indicates argument location. For commands that affect axes, the order of arguments is axis A first, followed by a comma, axis B next, followed by a comma, and so on. Omitting an argument will result in two consecutive commas and doesn't change that axis' current value. Examples of valid syntax are listed below. Valid Syntax Description AC n Specify argument for A axis only AC n,n Specify argument for A and B only AC n,,n Specify argument for A and C only AC n,n,n,n Specify arguments for A,B,C,D axes AC ,n,,,n Specify arguments for B and E axis only AC ,,,n,n Specify arguments for E and F Where n is replaced by actual values. Accepts Axis Mask These commands require the user to identify the specific axes to be affected. These commands are followed by uppercase X,Y,Z and W or A,B,C,D,E, file:///C|/Documents%20and%20Settings/Andy/Desktop/Temp/4Kcom/index.html (3 of 358)5/24/2011 8:38:16 AM Galil Motion Control Command Reference F,G and H.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages358 Page
-
File Size-