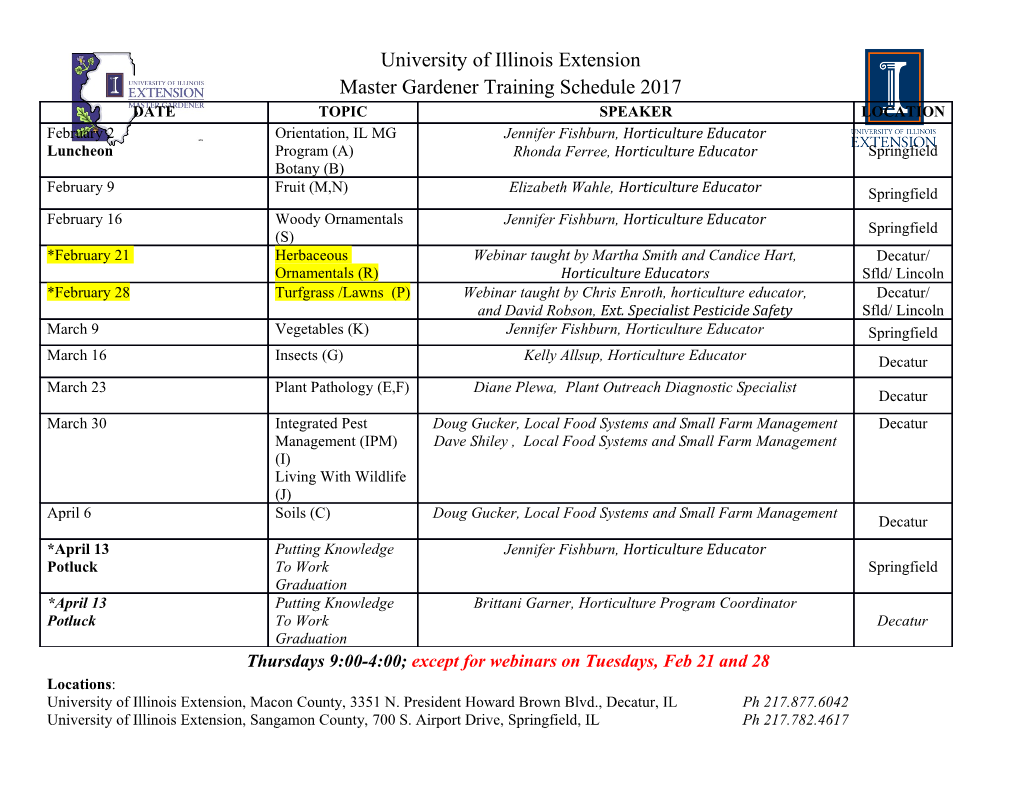
Outline Java Modeling Language (JML) and ESC/Java • What is the Java Modeling Language? • JML tutorial; • JML tools; – ESC/JAVA; • References; • JML / ESC/Java Demos; Marco Zennaro CS 294-3 Fall 2004 10/27/2004 JML and ESC/Java 2 2 Outline Formal specification languages • What is the Java Modeling Language? • C.S. have invented many formal languages to • JML tutorial; model software and specify properties about these models with techniques to verify these properties; • JML tools; – ESC/JAVA; • Formal languages guarantee: • References; – Precision (no ambiguity); • JML / ESC/Java Demos; – Certainty (modulo modeling errors); – Automation (automatic verification tools); Erik Poll. Introduction to JML, a notation to formally specifying Java programs. 2004 10/27/2004 JML and ESC/Java 2 3 10/27/2004 JML and ESC/Java 2 4 Java Modeling Language Java Modeling Language • A formal behavioral interface • Design by contract for Java: specification language for Java: – More expressive than Eiffel; – to specify behaviour of java classes; – Easier to use for the programmer than – to record design/implementation decisions; Larch: • By adding assertions to Java source • Uses Java boolean expression (extended with code: some operators); – Preconditions, postconditions, invariants, etc… Gary T. Leavens, Yoonsik Cheon. Design by Contract with JML, 2004 Gary T. Leavens, Yoonsik Cheon. Design by Contract with JML, 2004 Erik Pool. Introduction to JML […], 2004 Erik Pool. Introduction to JML, 2004 10/27/2004 JML and ESC/Java 2 5 10/27/2004 JML and ESC/Java 2 6 1 JML history Design by Contract (DBC) • Created at Iowa University (Leavens, A way of recording: Cheon); – Details of method responsibilities – Avoiding constantly checking arguments • Soon became an international effort: – Assigning blame across interfaces – University of Nijmegen (Poll, Van den Berg); The caller must ensure precondition holds while – HP (f.k.a. Compaq) SRC group (Leino, the called must ensure postconditions on exit; Nelson); The caller may assume postcondition while the – Kodak (Cok); called may assume preconditions; Gary T. Leavens, Yoonsik Cheon. Design by Contract with JML, 2004 10/27/2004 JML and ESC/Java 2 7 10/27/2004 JML and ESC/Java 2 8 Pre, Postconditions and Contracts as Documentation Invariants Definition • For each method say: – A method’s precondition says what must be true to call it. – What it requires (if anything), and – A method’s normal postcondition says what is true – What it ensures. when it returns normally (i.e., without throwing an exception). • Contracts are: – A method’s exceptional postcondition says what is – More abstract than code, true when a method throws an exception. – An invariant is a property that is always true of an – Often machine checkable, so can help with object’s state (when control is not inside the object’s debugging, and methods). – Machine checkable contracts can always be up-to-date. 10/27/2004 JML and ESC/Java 2 9 10/27/2004 JML and ESC/Java 2 10 Abstraction by contracts Modularity • A contract can be satisfied in many ways: • Typical OO code is modular; – a method can have many implementation … satisfying the contract; source.close(); dest.close(); – Different performances (time, space, etc); getFile().setLastModified(loc.modTime().getTime()); • A contract abstracts from the … implementation details; • We should be able to take advantage of • Hence we can change implementations the code modularity even in specifying / later. prove code properties. 10/27/2004 JML and ESC/Java 2 11 10/27/2004 JML and ESC/Java 2 12 2 Rules for Reasoning Contracts and Intent • Caller code • Code makes a poor contract, because can’t – Must work for every implementation that satisfies the separate: contract, and – What is intended (contract) – Can thus only use the contract (not the code!), i.e., • Must establish precondition, and – What is an implementation decision • Gets to assume the postcondition E.g., if deposit_into_account() rounds to the cent, can that be changed in the next release? • By contrast, contracts: • Called code – Must satisfy contract, i.e., – Allow vendors to specify intent, • Gets to assume precondition – Allow vendors freedom to change details, and • Must establish postcondition – Tell clients what they can count on. – But can do anything permitted by it. 10/27/2004 JML and ESC/Java 2 13 10/27/2004 JML and ESC/Java 2 14 Outline Introduction to JML • What is the Java Modeling Language? • JML specifications are contained in annotations, • JML tutorial; which are comments like: • JML tools; //@ … – ESC/JAVA; or • References; /*@ … @ … • JML / ESC/Java Demos; @*/ At-signs (@) on the beginning of lines are ignored within annotations. 10/27/2004 JML and ESC/Java 2 15 10/27/2004 JML and ESC/Java 2 16 Informal Description Formal Specifications • An informal description looks like: • Formal assertions are written as Java (* some text describing a property *) expressions, but: – Cannot have side effects – It is treated as a boolean value by JML, and • No use of =, ++, --, etc., and – Allows • Can only call pure methods. • Escape from formality, and • Organize English as contracts. – Can use some extensions to Java: Syntax Meaning public class Account { /*@ requires (* x is positive *); \result result of method call @ ensures \result >= 0 && a ==> b a implies b @ (* \result is the updated balance after the deposit*) a <== b b implies a a <==> b a iff b @*/ a <=!=> b !(a <==> b) public static double deposit_into_account(double x) { … } \old(E) value of E in pre-state } 10/27/2004 JML and ESC/Java 2 17 10/27/2004 JML and ESC/Java 2 18 3 Example (pre and post cond.) Example (ex. postcond.) /*@ requires x >= 0.0; /*@ requires x >= 0.0; ensures JMLDouble.approximatelyEqualTo(\result, ensures JMLDouble.approximatelyEqualTo(balance, \old(balance) + x, eps); \old(balance) + x, eps); @*/ exsures (Exception e) public static double deposit_into_account(double x) { … } \old(x) > DEPOSIT_LIMIT && balance == \old(balance) && e.getReason() == AMOUNT_TOO_BIG; Obligations Rights @*/ public static double deposit_into_account(double x) throws… caller Passes non-negative Gets updated balance number Trade-off between preconditions and exceptional postconditions; called Update balance Assumes argument Adding x to it is non-negative 10/27/2004 JML and ESC/Java 2 19 10/27/2004 JML and ESC/Java 2 20 Example (invariant) Quantifiers // File: Account.java public class Account { private /*@ spec_public non_null @*/ String accountNumber; • JML supports several forms of quantifiers private /*@ spec_public @*/ double balance; – Universal and existential (\forall and \exists) //@ public invariant !accountNumber.equals(“”) && balance >= 0; – General quantifiers (\sum, \product, \min, \max) //@ ensures \result == balance; public double getBalance(); – Numeric quantifier (\num_of) /*@ ensures balance >= 0 && balance == \old(balance + deposit); exsures (Exception e) x > DEPOSIT_LIMIT && balance == \old(balance) (\forall Student s; juniors.contains(s); s.getAdvisor() != null) && e.getReason() == AMOUNT_TOO_BIG; @*/ public void deposit_into_account(int kgs); (\forall Student s; juniors.contains(s) ==> s.getAdvisor() != null) /*@ requires !n.equals(“”); ensures n.equals(accountNumber) && balance == 0; @*/ public Account(/*@ non_null @*/ String n); } 10/27/2004 JML and ESC/Java 2 21 10/27/2004 JML and ESC/Java 2 22 Model Variables Example class Counter { model n: int; Are specification only variables – Like domain-level constructs – Given value only by represents clauses: – Information hiding; method Increment() – Data abstraction; modifies only n; ensures old(n) + 1 = n; name abstract (model) method Decrement() modifies only n; represented by ensures old(n) = n + 1; fullName concrete (real) } 10/27/2004 JML and ESC/Java 2 23 10/27/2004 JML and ESC/Java 2 24 4 Example Outline class Counter { model n: int; private a: int; • What is the Java Modeling Language? private b: int; representation n is a – b; • Introduction to JML syntax and semantic; method Increment() modifies only n; • JML tools; ensures old(n) + 1 = n; – ESC/JAVA; { a := a + 1 } method Decrement() • References; modifies only n; ensures old(n) = n + 1; • JML / ESC/Java Demo; { b := b + 1 } } 10/27/2004 JML and ESC/Java 2 25 10/27/2004 JML and ESC/Java 2 26 Tools for JML ESC vision • JML compiler (jmlc/jmlrac): Increased programmer productivity and – perform JML checks at runtime; program reliability through increased – low-cost; rigor: – Record design decisions; • Extended static checker (ESC/Java2): – Utilize automatic checking; – prove JML assertions at compile time; – Detect errors and improve maintainability; – higher cost; – possible for small program or subsystems; • Etc… R. Leino, Hoare-style program verification, May 2004 10/27/2004 JML and ESC/Java 2 27 10/27/2004 JML and ESC/Java 2 28 ESC vision ESC vision • Improve the current software engineering Take a program annotated with process developing practical tools; assertions. Consider a tool capable of: • It is NOT program verification, it is like a type • Automatically check if the assertions are checker: always true; – its warnings are intended to be interpreted by the author of the program; • Statically without any user input; – It does not find all the errors, but reduce the • Reason about non-trivial properties (not process cost finding some of them early; – “We are interested in failed proof only”; just type-correctness); D. Detlefs, R. Leino, G.Nelson, J. Saxe. Extended Static Checking,1998. J. Kiniri, ESC/Java 2, extended static checking
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages7 Page
-
File Size-