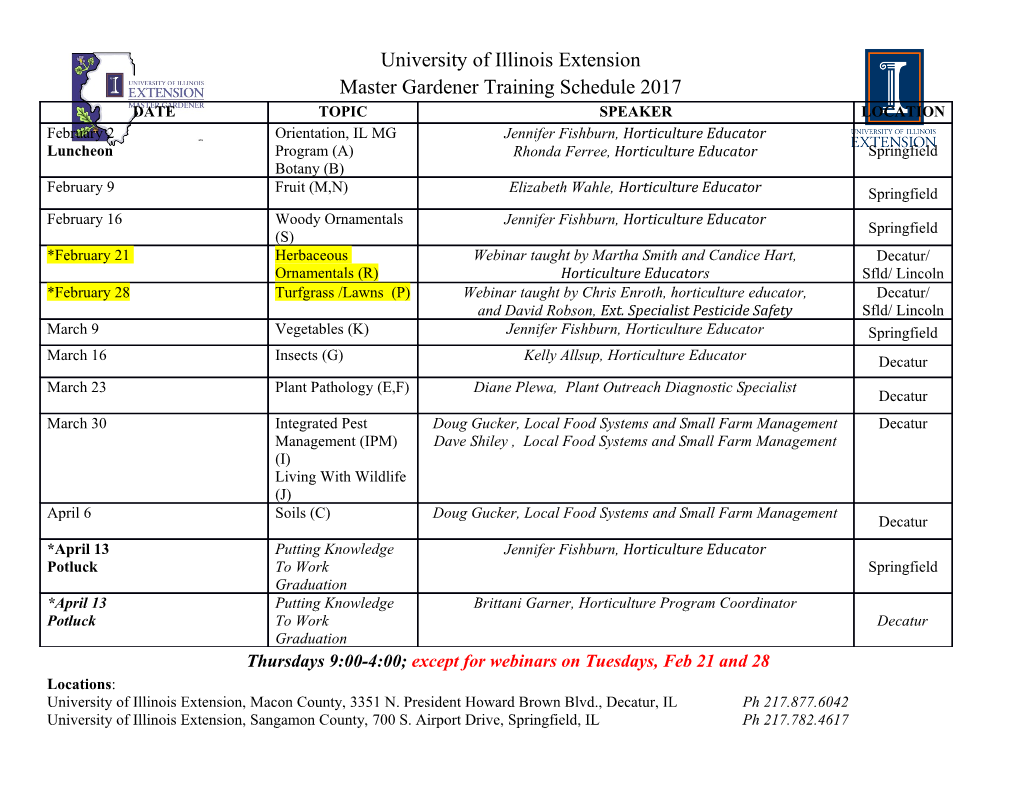
JavaScript Syntax Comments Comments are sections of code that you do not want to run. A single line comment is preceded by //, while multiline comments are surrounded by /* ... */: varx= 100 // This isa comment; it will be ignored when the code is run 2 vary= 50// This isa comment on the same line as some code 4 /* This isa longer 6 comment, that spans multiple lines*/ 8 Line Endings Lines of JavaScript code are separated by a return character (so each line is actually on its own line) or by a semicolon: varx= 100 vary= 50 2 vara= 20; varb= 40 Either is fine, though it's good to be in the habit of ending lines with the ; symbol. This makes it easier to \minify" your code, and put long scripts on a single line. Case Sensitivity JavaScript is a case-sensitive language, which means that the strings 'hello', 'Hello', and 'HELLO' would not be equal. JavaScript keywords, like function and for, must be written in lowercase. Quotation Marks Strings in JavaScript can be surrounded by either single or double quotation marks. var newString="I 'ma string with double quotation marks" var aNewString= 'This isa string with single quotation marks ' 2 var anotherNewString= 'AndI 'ma string with too many quotation marks ' The reason you'd want to use a mix of both can be seen in the color highlighting of the above example; the apostrophe in the word "I'm" was interpreted as the end of the string. Instead, we'll surround the string in double quotes: var anotherNewString="AndI 'ma string with too many quotation marks" Variables A variable in JavaScript can hold one of several data types, including numbers, strings, arrays, booleans, functions, objects, etc. JavaScript is a dynamic language, so we don't need to specify the type of our variable, and we can change it at any time. We assign a value to a variable using the '=' operator. varx=5 You can retrieve the type of a variable using the typeof operator: var sampleString= 'Hello world ' varx= false 2 typeof sampleString// returns 'string ' typeofx// returns 'boolean ' 4 When naming variables, begin with a lowercase letter. You can use any combination of letters and numbers, and the symbol. Do not include a space in the variable name. Data Visualization with JavaScript · Spring 2016 · JavaScript Reference JavaScript Control Flow If and If-Else Statements If statements are used to execute a block of code, given particular conditions are true. varx= 100 vary= 50 2 if(x>0 ) { alert( 'x is positive ') 4 } if(y<2){ 6 alert( 'y is small ') } 8 // there will be an alert 'x is positive ', but the second alert will not be displayed, sincey<2 is false If-else statements are used when you want one block of code to run when a statement is true, and another to run if the statement is false: varx=4 if(x<0){ 2 alert( 'x is negative ') } 4 else{ alert( 'x is not negative ') 6 } // the alert will display 'x is not negative ', sincex<0 is false 8 For even more options, use else if to test for addition conditions: varx=4 if(x<0){ 2 alert( 'x is negative ') } 4 else if(x==0){ alert( 'x is zero ') 6 } else{ 8 alert( 'x is positive ') } 10 // the alert will display 'x is positive ', since x<0 and x==0 are both false For Loops To have a block of code run a particular number of times, use a for loop. A typical for loop takes three arguments: the beginning value, the condition that must be true for the loop to continue, and the increment. for(vari= 0;i< 5;i++) { // definea variablei to be 0, and increasei by1 with each loop 2 // the loop will run untili is5(but not including i=5) console.log(i) 4 } // the console will show 0,1,2,3,4 6 for(vari= 4;i< 20; i=i+2) { 8 // definea variablei to be 4, and increasei by2 with each loop // the loop will run untili is 20(but not including i=5) 10 if(i%2==0){// check ifi is divisible by2 console.log(i)// only printi ifi%2==0 12 } } 14 // the console will show 4,6,8,10,12,14,16,18 Data Visualization with JavaScript · Spring 2016 · JavaScript Reference For loops can also be used to loop over array values or object properties using for..in: var sampleArray= [1,3,6, 'hi '] for(vari in sampleArray){ 2 console.log(i) } 4 // the console will show 1,3,6,hi var sampleObject={ 'first ': 1, 'second ':3, 'third ':'hi ' } 6 for(vari in sampleObject){ //i ranges over all property names 8 // sampleObject[i] produces the value of the propertyi var string="The value of property"+i+" is"+sampleObject[i] 10 console.log(string) } 12 // the console will show: // The value of property first is1 14 // The value of property second is3 // The value of property third is hi 16 While Loops While loops are used to execute a code block while a given condition is true. The condition is tested before the code is executed. varx=2 while(x<1){ 2 console.log(x) x++ 4 } // Nothing is printed. The condition is false to begin with, so the code will not be 6 executed. vary=2 8 while(y<10){ console.log(y) 10 y++// change the value ofy, or else the loop will never end } 12 // console will show 2,3,4,5,6,7,8,9 Do While Loops Do-while loops are used to execute a code block while a given condition is true. The condition is tested after the code is executed, in contrast to while loops. varx=2 do{ 2 console.log(x) x++ 4 } while(x<1) 6 // The console will show 2, andx is increased to 3. Then the condition is tested and found to be false, so the loop ends. 8 vary=2 do{ 10 console.log(y) y++// change the value ofy, or else the loop will never end 12 } while(y< 10) 14 // console will show 2,3,4,5,6,7,8,9,10 Data Visualization with JavaScript · Spring 2016 · JavaScript Reference Break The break command is used to stop a loop. For instance, the following code creates an array. Then we create a for loop that tests if the given value of x is in the array. If it is, we'll set a boolean value equal to true: var testArray= [1,10,2,8,3,15,11,7] varx=2 2 var xHasBeenFound= false for(vari in testArray){ 4 if(i==x){ xHasBeenFound= true 6 } } 8 // xHasBeenFound is now true Since x is actually found at index 2, there was no need to continue the search. Adding a break command will stop the loop when it's no longer necessary to run. var testArray= [1,10,2,8,3,15,11,7] varx=2 2 var xHasBeenFound= false for(vari in testArray){ 4 if(i==x){ xHasBeenFound= true 6 break } 8 } // xHasBeenFound is now true, and the loop stopped after only3 runs instead of8 10 Switch Switch statements can be more convenient that a series of if-then-else statements. Blocks of code are executed depending on the value of an expression. var breed= prompt("What kind of dog do you have?") //a pop-up will display on screen asking the question 2 // the value of breed will bea string equal to whatever the user types as their answer 4 switch( breed){ case"English Bulldog":// if the user typed English Bulldog: 6 alert("Bulldogs are courageous but gentle and were bred to bait bulls.") break;// end the switch statement, since we found the case 8 case"Clumber Spaniel": alert("Clumber spaniels are calm but affectionate and originated in France.") 10 break; case"Labrador Retriever": 12 alert("Labs are athletic and are excellent swimmers.") break; 14 case"Papillon": alert("Papillons are small but intelligent dogs with long hair and big ears.") 16 break; default:// the user entered an expression that wasn 't on our list 18 alert("Sorry,I don 't know about your dog!") } 20 Data Visualization with JavaScript · Spring 2016 · JavaScript Reference JavaScript Strings Strings in JavaScript can be any combination of letters, numbers, and symbols, surrounded by single or double quotes. var sampleString="I ama string" All strings have a length property that returns the number of characters (including whitespace) in the string: var sampleString="I ama string" sampleString.length// returns 13 2 Concatenation Two strings can be concatenated (combined into a single string) using the + symbol: var sampleString="I ama string" var longerString= sampleString+" and nowI am longer" 2 // longerString has value"I ama string and nowI am longer" You can concatenate two strings or a string and a variable or number. Anything surrounded by quotes will be interpreted literally. varx=4 vary=2 2 var firstTry="The sum ofx andy isx+y" // firstTry value is"The sum ofx andy isx+y" 4 var secondTry="The sum of"+x+" and"+y+" isx+y" // secondTry value is"The sum of4 and2 isx+y" 6 var thirdTry="The sum of"+x+" and"+y+" is"+(x+y) // secondTry value is"The sum of4 and2 is 6" 8 Useful string methods Each character in a string is given an index, starting at 0. To find the character at a particular index, use the charAt method: var sampleString="I ama string" sampleString.charAt(0)// returns"I" 2 sampleString.charAt(7)// returns"s" To find the index of a particular character or string, use the indexOf method. This will only return the first index where the character or string appears.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages12 Page
-
File Size-