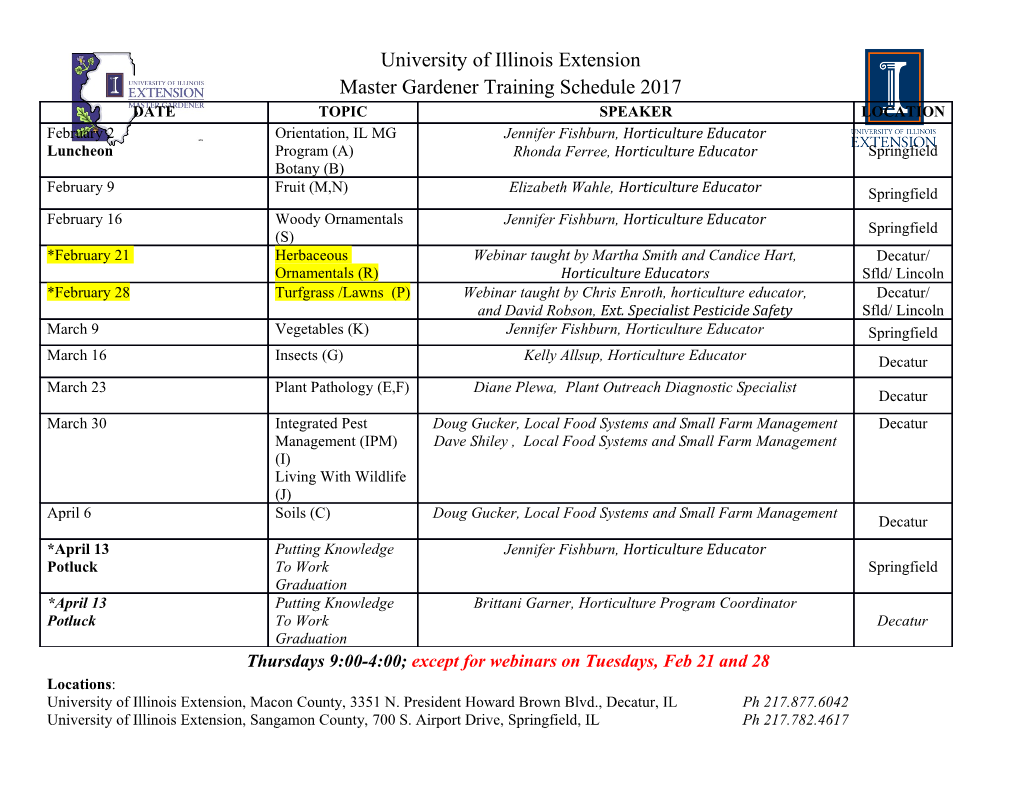
Programming Languages Introduction to Programming in Java: An Interdisciplinary Approach · Robert Sedgewick and Kevin Wayne · Copyright © 2002–2010 · 09/15/10 01:29:19 PM The Tower of Babel Several ways to solve a transportation problem A story about the origins of multiple languages. • [After the flood] “the whole earth was of one language and one speech.” • They built a city and tower at Babel, believing that with a single language, people will be able to do anything they imagine. • Yahweh disagrees and “confounds the language of all the earth” • Why? Proliferation of cultural differences (and multiple languages) is one basis of civilization. 3 4 Several ways to solve a programming problem Java You can write a Java program. ThreeSum.java public class ThreeSum { public static void main(String[] args) { int N = Integer.parseInt(args[0]); int[] a = new int[N]; for (int i = 0; i < N; i++) a[i] = StdIn.readInt(); for (int i = 0; i < N; i++) for (int j = i+1; j < N; j++) for (int k = j+1; k < N; k++) if (a[i] + a[j] + a[k] == 0) StdOut.println(a[i] + " " + a[j] + " " + a[k]); } } % more 8ints.txt 30 -30 -20 -10 40 0 10 5 Ex. Read N int values from standard input; % javac ThreeSum.java % java ThreeSum 8 < 8ints.txt print triples that sum to 0. 30 -30 0 30 -20 -10 [See lecture 8] -30 -10 40 -10 0 10 5 6 C A big difference between C and Java (there are many!) You can also write a C program. ThreeSum.c No data abstraction #include <stdio.h> • no objects in C #include <stdlib.h> C program is sequence of static methods main(int argc, char *argv[]) • { int N = atoi(argv[1]); int *a = malloc(N*sizeof(int)); int i, j, k; for (i = 0; i < N; i++) C++ (Stroustrup 1989) scanf("%d", &a[i]); for (i = 0; i < N; i++) • “C with classes” for (j = i+1; j < N; j++) adds data abstraction to C for (k = j+1; k < N; k++) • if (a[i] + a[j] + a[k] == 0) printf("%4d %4d %4d\n", a[i], a[j], a[k]); } % more 8ints.txt 30 -30 -20 -10 40 0 10 5 Noticable differences: % cc ThreeSum.c library conventions % ./a.out 8 < 8ints.txt array creation idiom 30 -30 0 30 -20 -10 standard input idiom -30 -10 40 -10 0 10 pointer manipulation (stay tuned) 7 8 C++ C++ You can also write a C++ program. BST.cxx template <class Item, class Key> Ex 1. Use C++ like C Ex 2. Use C++ like Java class ST ThreeSum.cxx { private: #include <iostream.h> struct node #include <stdlib.h> { Item item; node *l, *r; int N; main(int argc, char *argv[]) Challenges: node(Item x) { { item = x; l = 0; r = 0; N = 1; } int N = atoi(argv[1]); libraries/idioms }; int *a = new int[N]; pointer manipulation typedef node *link; int i, j, k; link head; for (i = 0; i < N; i++) templates (generics) Item nullItem; cin >> a[i]; for (i = 0; i < N; i++) Item searchR(link h, Key v) for (j = i+1; j < N; j++) { if (h == 0) return nullItem; for (k = j+1; k < N; k++) Key t = h->item.key(); if (a[i] + a[j] + a[k] == 0) if (v == t) return h->item; cout << a[i] << " " << a[j] << " " << a[k] << endl; if (v < t) return searchR(h->l, v); } else return searchR(h->r, v); % cpp ThreeSum.cxx % ./a.out 8 < 8ints.txt } 30 -30 0 ... Noticable differences: 30 -20 -10 } library conventions -30 -10 40 -10 0 10 standard I/O idioms 9 10 A big difference between C/C++ and Java (there are many!) Python Programs directly manipulate pointers You can write a Python program! C/C++: You are responsible for memory allocation Ex 1. Use python as a calculator • system provides memory allocation library • programs explicitly “allocate” and “free” memory for objects % python • C/C++ programmers must learn to avoid “memory leaks” Python 2.7.1 (r271:86832, Jun 16 2011, 16:59:05) Type "help" for more information. >>> 2+2 Java: Automatic “garbage collection”. 4 >>> (1 + sqrt(5))/2 Traceback (most recent call last): C code that reuses an array name File "<stdin>", line 1, in <module> Java code that reuses an array name NameError: name 'sqrt' is not defined double arr[] = >>> import math calloc(5,sizeof(double)); double[] arr = new double[5]; >>> (1 + math.sqrt(5))/2 ... ... 1.618033988749895 free(arr); arr = new double[10]; arr = calloc(10, sizeof(double)); Fundamental challenge: Code that manipulates pointers is inherently “unsafe”. 11 12 Python Compile vs. Interpret You can write a Python program! Def. A compiler translates your entire program to (virtual) machine code. Def. An interpreter simulates a (virtual) machine running your code Ex 2. Use Python like you use Java on a line-by-line basis. Noticable differences: threesum.py no braces (indents instead) import sys def main(): no type declarations threesum.c a.out N = int(sys.argv[1]) array creation idiom a = [0]*N I/O idioms C C compiler machine for i in range(N): source code code a[i] = int(sys.stdin.readline()) for (iterable) idiom for i in range(N): for j in range(i+1, N): range(8) is [0, 1, 2, 3, 4, 5, 6, 7] threesum.py for k in range(j+1, N): if (a[i] + a[j] + a[k]) == 0: Python print repr(a[i]).rjust(4), Python interpreter Another example: TOY (Lecture 11) source code print repr(a[j]).rjust(4), % python threesum.py 8 < 8ints.txt print repr(a[k]).rjust(4) 30 -30 0 main() 30 -20 -10 -30 -10 40 ThreeSum.java ThreeSum.class -10 0 10 “javac” “java” Java Java compiler JVM interpreter source code code 13 14 A big difference between Python and C/C++/Java (there are many!) Matlab No compile-time type checking Python programmers must remember You can write a Matlab program! their variable names and types • no need to declare types of variables • system checks for type errors at RUN time Ex 1. Use Matlab like you use Java. ... Implications: for i = 0:N-1 for j = i+1:N-1 • Easier to write small programs. for k = j+1:N-1 More difficult to debug large programs. if (a(i) + a(j) + a(k)) == 0: • sprintf("%4d %4d %4d\n", a(i), a(j), a(k)); end end Typical (nightmare) scenario: end end • Scientist/programmer misspells variable name ... referring to output file in large program. • Program runs for hours or days. Ex 2 (more typical). Use Matlab for matrix processing. Using Python for large problems • Crashes without writing results. is like playing with fire. A = [1 3 5; 2 4 7] B = [-5 8; 3 9; 4 0] -5 8 1 3 5 24 35 Reasonable approaches C = A*B 3 9 2 4 7 * = 30 52 C = 4 0 • Throw out your calculator; use Python. 24 35 • Prototype in Python, then convert to Java for “production” use. 30 52 15 16 Big differences between Matlab and C/C++/Java/Python (there are many!) Why Java? [revisited from second lecture] 1. Matlab is not free. Java features. 2. Most Matlab programmers use only ONE data type (matrix). • Widely used. • Widely available. • Embraces full set of modern abstractions. • Variety of automatic checks for mistakes in programs. i = 0 Ex. Matlab code “ ” “redefine the value of the complex number i to be a 1-by-1 matrix whose entry is 0” Facts of life. No language is perfect. Notes: • “There are only two kinds of programming Matlab is written in Java. • We need to choose some language. • languages: those people always [gripe] • The Java compiler and interpreters are written in C. about and those nobody uses.” [Modern C compilers are written in C.] Our approach. – Bjarne Stroustrup • Matrix libraries (written in C) accessible from C/C++/Java/Python. • Minimal subset of Java. • Develop general programming skills Reasonable approach: that are applicable to many languages • Use Matlab as a “matrix calculator” (if you own it). Convert to or use Java or Python if you want to do anything else. • It’s not about the language! 17 18 Why do we use Java in COS 126? Why learn another programming language? Good reasons to learn a programming language: full set of modern automatic widely widely offers something new (see next slide) modern libraries checks • used available abstractions and systems for bugs • need to interface with legacy code or with coworkers • better than Java for the application at hand ✓ ✓ ✗ ✗ ✓ • intellectual challenge (opportunity to learn something about computation) ✓ ✓ ✓ maybe ✓ ✓ ✓ ✓ ✓ ✓ ✓ $ maybe* ✓ ✗ ✓ ✓ maybe ✓ ✗ * OOP recently added but not embraced by most users 19 20 Something new: a few examples Programming styles 1960s: Assembly language Procedural • symbolic names • step-by-step instruction execution model • relocatable code • Ex: C 1970s: C Scripted • “high-level” language (statements, conditionals, loops) • step-by-step command execution model, usually interpreted • machine-independent code • Ex: Python, Javascript basic libraries • Special purpose optimized around certain data types 1990s: C++/Java • • Ex: Postscript, Matlab • data abstraction (object-oriented programming) • extensive libraries Object-oriented (next) • focus on objects that do things 2000s: Javascript/PHP/Ruby/Flash • Ex: Java, C++ • scripting Functional (stay tuned) • libraries for web development • focus on defining functions • Ex: Scheme, Haskell, Ocaml 21 22 Object Oriented Programming Procedural programming. [verb-oriented] Object-Oriented Programming • Tell the computer to do this. • Tell the computer to do that. A different philosophy. Software is a simulation of the real world. • We know (approximately) how the real world works.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages14 Page
-
File Size-