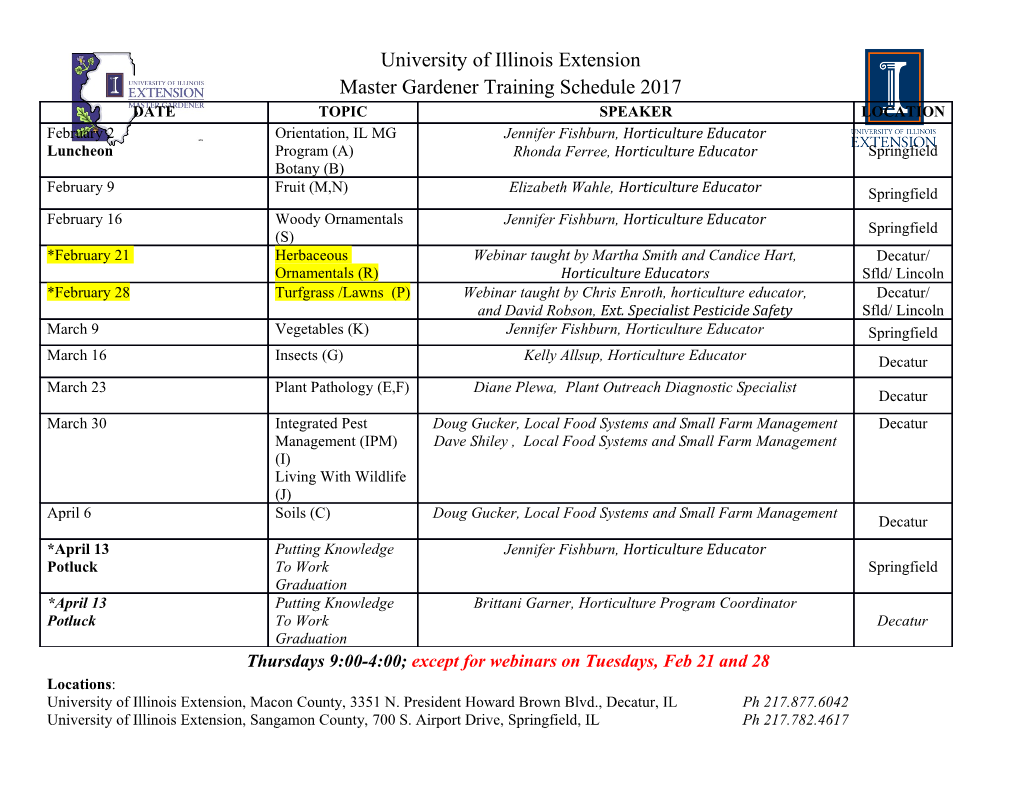
An Introduction to Programming in Simula Rob Pooley This document, including all parts below hyperlinked directly to it, is copyright Rob Pooley ([email protected]). You are free to use it for your own non-commercial purposes, but may not copy it or reproduce all or part of it without including this paragraph. If you wish to use it for gain in any manner, you should contact Rob Pooley for terms appropriate to that use. Teachers in publicly funded schools, universities and colleges are free to use it in their normal teaching. Anyone, including vendors of commercial products, may include links to it in any documentation they distribute, so long as the link is to this page, not any sub-part. This is an .pdf version of the book originally published by Blackwell Scientific Publications. The copyright of that book also belongs to Rob Pooley. REMARK: This document is reassembled from the HTML version found on the web: https://web.archive.org/web/20040919031218/http://www.macs.hw.ac.uk/~rjp/bookhtml/ Oslo 20. March 2018 Øystein Myhre Andersen Table of Contents Chapter 1 - Begin at the beginning Basics Chapter 2 - And end at the end Syntax and semantics of basic elements Chapter 3 - Type cast actors Basic arithmetic and other simple types Chapter 4 - If only Conditional statements Chapter 5 - Would you mind repeating that? Texts and while loops Chapter 6 - Correct Procedures Building blocks Chapter 7 - File FOR future reference Simple input and output using InFile, OutFile and PrintFile Chapter 8 - Item by Item Item oriented reading and writing and for loops Chapter 9 - Classes as Records Chapter 10 - Make me a list Lists 1 - Arrays and simple linked lists Reference comparison Chapter 11 - Like parent like child Sub-classes and complex Boolean expressions Chapter 12 - A Language with Character Character handling, switches and jumps Chapter 13 - Let Us See what We Can See Inspection and Remote Accessing Chapter 14 - Side by Side Coroutines Chapter 15 - File For Immediate Use Direct and Byte Files Chapter 16 - With All My Worldly Goods... Prefixed Blocks And Separate Compilation Chapter 17 - Add To The List More Complex List Structures - SimSet Chapter 18 - Virtually Anything Is Possible A More Formal Definition Of Scope; Virtual, Hidden and Protected Chapter 19 - Class Simulation SIMULA and simulation Chapter 20 - Tying Up Loose Ends The Complete Environment and Where to Go From Here Appendix A - Arithmetic expressions Appendix B - Arithmetic types Appendix C - Alternative number bases Appendix D - Conditional expressions Chapter 1 begin at the beginning...... What is a computer program? A computer program (program is always spelt in the American way) is a series of instructions which contains all the information necessary for a computer to perform some task. It is similar to a knitting pattern, a recipe or a musical score. Like all of these it uses a special shorthand, known in this case as a programming language. This book describes how to write and understand programs written in the language SIMULA. The definition used is the 1985 SIMULA Standard, which extends and clarifies the language previously known as SIMULA 67. The 67 stood for 1967, the year in which this earlier version was first defined. These should not be confused with the older language known as SIMULA 1, which is an ancestor of SIMULA 67 and thus of today's SIMULA. A few old references to SIMULA (notably a 1966 article in CACM) will mean SIMULA 1. More often SIMULA 67 is intended. Many implementations of SIMULA will not yet have all the features given in the 1984 standard, although they will probably have more than those defined in 1967. Wherever sensible this book tries to warn its readers of features which may not be universally available. In addition, it is usually a good idea to check the documentation for the particular SIMULA system to be used, in case it has any peculiarities of its own. SIMULA is highly standardised, but differences may still be found. You may already be familiar with other programming languages such as Fortran, Basic or Pascal. Even so you will find this introduction essential, since SIMULA views the world rather differently in some ways. In particular SIMULA expects you to organise or structure your program carefully. It also allows you to describe actions as if they were happening simultaneously or in parallel. If the next paragraph seems confusing, read it now and see how much you can understand, then come back after each chapter until it makes complete sense. Do the same for any other parts of this book which are not clear at first reading. Remember that in learning a new computer language you are trying to take in many concepts which only make sense together, so that often several re- readings of what will later seem obvious points are necessary. A SIMULA program is made up of sequences of instructions known as blocks, which act independently to varying degrees, but which are combined to produce the desired overall effect. The simplest programs contain only one block, known as the program block. All other blocks follow the same basic rules, so let us have a look at the single block program, example 1.1. Example 1.1: A simple example begin integer Int1; comment The first SIMULA program written for this book; Int1:=3; OutInt(Int1,4); OutImage end This is not the simplest possible program. We could have written begin end and had a complete program block. This tells us the first rule about all blocks. A block always begins with the word begin and ends with the word end. beginand end are called "keywords", since they are reserved for special purposes and may not be used for anything else. A note on letters and "cases". Although the programs shown in this book use bothcapital letters, which are known to computer programmers as "upper case" characters, and small, "lower case", letters. You are free to use whichever case you prefer. A SIMULA program is interpreted as if all its letters, which are not enclosed in double quotes, were first converted to upper case. You can even write BegIN or eNd. The right ingredients So we have to put begin and end. What about the text in between? This is made up of instructions to the SIMULA system, which are of two types; DECLARATIONS and STATEMENTS. There are also pieces of the text which are ignored by the SIMULA system and which simply help any human who reads the program to follow what is happening. These are called comments. Declarations In example 1.1, the first instruction after the word begin is a declaration: integer Int1; Declarations in a block show how much of the computer's memory is going to be needed by that block. They also show into how many sections it is to be divided , how big each is to be and to what sort of use each will be put. Each of these sections is given a name, by which the program will refer to it. The program block in example 1.1 has only one declaration. It starts with the "type" keyword integer, followed by a name or "identifier", Int1. The type (integer in this example) says what the section of memory will be used for. In example 1.1 it will be used to hold a whole number, which is called an integer in SIMULA. Once a section of memory has been given its type in this way, it may not be used to hold something of a different type. Since this location will hold an integer its required size will also be known to the SIMULA system. All items of the same type require the same amount of space in memory to hold them. The identifier Int1 is now the name of the space reserved in this way. We may sometimes refer to the value held in this space as Int1 also. This is a useful shorthand, especially when we are not aware what the current contents are. If we want to use more locations, we can declare them in the same way, being careful to give the correct type to each. Thus we might write begin integer Int1; real Real1; etc. Which gives us a location of type real and called Real1, which we can use in this block. The possible types and their uses are explained more fully in chapter 3. If we want to have more than one location which can hold a whole number, then we can declare a second integer, with a different identifier. We might do this by writing begin integer Int1; integer Count; etc. or, alternatively, by writing begin integer Int1, Count; etc. which many people find neater. This style is called a declaration list, with a series of identifiers of locations with the same type separated by commas. Comments The next line is a comment. It begins with the keyword comment, is followed by some text and ends in a semi-colon. comment The first SIMULA program written for this book; It has no effect on what the program does, but it contains some information on what the program is or does. In example 1.1 it tells us which version of the program we are looking at. This is often very useful. As well as forming lines on their own, comments can also be used in certain places within instructions or combined on the same line as them. This will be shown in the next chapter. When you are writing programs in independent sections, as we will be, you may not look back at a "working" component for some time.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages228 Page
-
File Size-