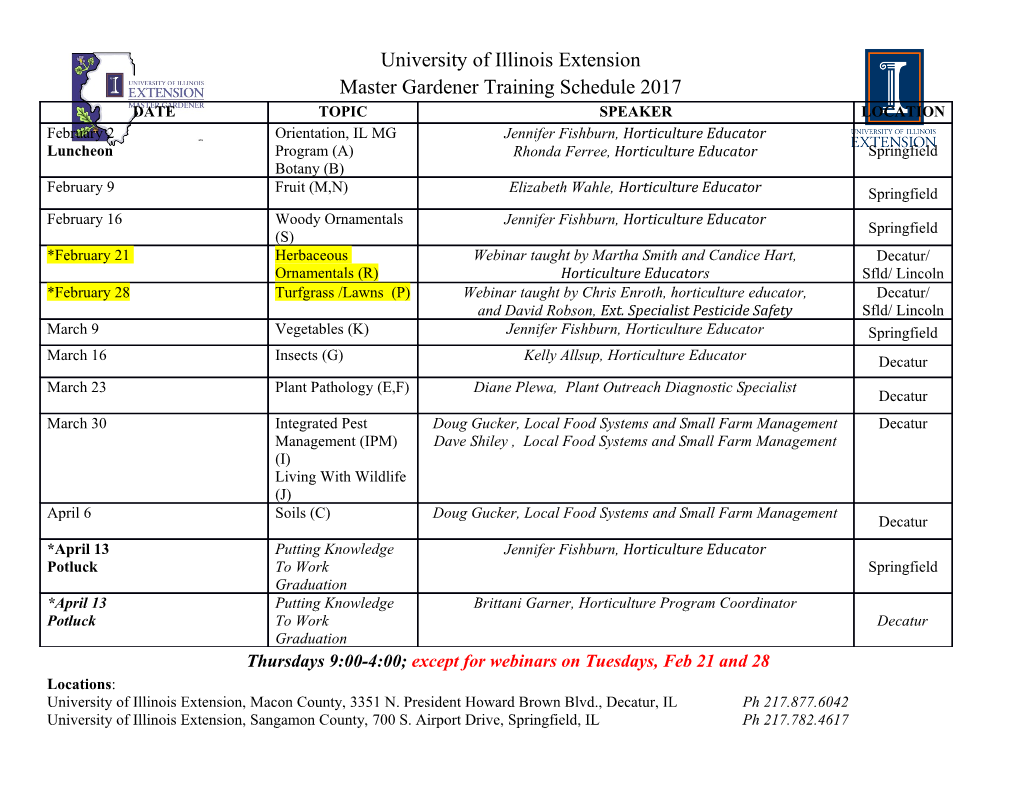
A Gentler Introduction to Mindstorm NXT Programming Zhizhang Shen ∗ Dept. of Computer Science and Technology Plymouth State University Contents 1 An introduction 2 1.1 Thenatureofarobot............................... 2 1.1.1 Whatisarobot? ............................. 2 1.1.2 Robotparadigms ............................. 3 1.1.3 Behaviorcontrol ............................. 6 1.1.4 Evaluationcriteria ... .. .. .. .. ... .. .. .. .. ... .. 9 1.2 LegoMindstormNXTrobot . 9 2 Software configuration 11 2.1 JavaJDK ..................................... 11 2.2 Thelejosdialect................................. 12 2.3 TheEclipeIDE.................................. 12 2.4 USBconnection.................................. 12 2.5 TalktotheBrick ................................. 12 2.6 Wirelessconnection.. .. ... .. .. .. .. ... .. .. .. .. ... 12 2.7 Totalrequirements ............................... 13 2.8 Softwareinstallation . 13 3 Get it started 14 3.1 Lejos........................................ 14 3.2 Gettingstarted .................................. 14 3.3 Createanewproject ............................... 16 3.4 Createanewclass ................................ 18 3.5 Compile,linkanddownload . 19 ∗Address correspondence to Dr. Zhizhang Shen, Dept. of Computer Science and Technology, Plymouth State University, Plymouth, NH 03264, USA. E mail address: [email protected]. 1 3.6 Thefinalkick ................................... 22 4 Robot assembly 22 5 Basic programming 23 5.1 TheLCDscreen.................................. 23 5.2 Delay........................................ 23 5.3 Thebuttons.................................... 24 5.4 Themotors .................................... 25 5.4.1 Basicoperations ............................. 25 5.4.2 The Navigator API ........................... 26 5.5 Sensors....................................... 29 5.5.1 Touchsensor ............................... 29 5.5.2 Lightsensor................................ 30 5.5.3 Soundsensor ............................... 30 5.5.4 Ultrasonicsensor ............................. 30 5.5.5 Rotationsensors ............................. 31 5.5.6 Othersensors ............................... 31 5.5.7 Soundplayer ............................... 33 5.6 Currentprogramming. .. ... .. .. .. .. ... .. .. .. .. ... .. 34 1 An introduction We talk a bit general about what a robot is, and the major paradigms to make it work. We then discuss briefly about the robot kit that we are going to use in this unit, the Lego Mindstorm NXT[1]. 1.1 The nature of a robot 1.1.1 What is a robot? An intelligent robot is a mechanical creature that can function autonomously Being “intelligent” means that the robot does not do things in a mindless, repetitive, way. Being “mechanical” means that we use mechanical building blocks, rather than using biological parts to put together a robot. It also means that a robot is not merely a computer, since as a “creature”, it can move around, change the environment, etc.. Being able to “function autonomously, means that it can operate, self-contained, under all reasonable conditions without the involvement of a human operator. It also means that it can adapt to changes in the environment (e.g., lights go off), and itself (e.g., a wheel falls off) and continue to reach its goal. 2 There are quite a few robot competition, ranging from football games[7] to the DARPA Grand Challenge [2]. Last one, held in October, 2005, a competition for robotic vehicles to complete an under-200 mile, off-road course in the Mojave Desert. The Stanford Racing Team completed the 212.4 km (132-mile) course in just under 7 hours to win a USD 2M prize. The Defense Advanced Research Projects Agency (DARPA) has held its third Grand Challenge competition on November 3, 2007, in Victorville, CA, with a theme of driving in an urban area. Carnegie Mellon’s Tartan Racing Team scored the first place prize of$2 million. Stanford University’s Stanford Racing team came in second for $1 million, and Virginia Tech’s Victor Tango team won the third place prize of $500,000. 1.1.2 Robot paradigms At the very bottom, there are three robotic primitives: SENSE, PLAN, and ACT. A sense function collects information through sensor(s), which a plan function uses to generate a plan. This plan will be carried out by some act functions. More specifically, 1. A function takes in information from its sensors, and produces an output useful for other functions falls into the category of SENSE. 2. A function takes in information either from sensors or from its own knowledge base, and produces one or more tasks for the robot to perform is a PLAN function. 3. Finally, a function produces output commands to motor actuators is an ACT function. A paradigm is a way to look at things. Technically speaking, it is a set of assumptions and/or techniques that characterize an approach to a class of problems. For example, to find a place to live, there are two approaches, one is to rent a house, the other is to buy one. Each of those two approaches comes with a whole set of assumptions (how much money do you have at present? what about the current market? how long do you stay there?...) and techniques (how to pay for it? how to take care of the property? how to remove the snow during the winter?...) There are essential three ways to organize intelligence in robots: hierarchical, reactive, and an hybrid one, which combines the first two. They can be described in the following two ways, either through describing the rela- tionship between the three commonly accepted primitives; or through describing the way in which sensory data is processed and distributed in the robotic system. • The hierarchical paradigm is the oldest one, widely used between 1967 and 1990. Under this paradigm, as shown in Figure 1, a robot operates in a top-down style, based on a world modeling and planning process. This paradigm comes with the assumption that a robot checks out the world, then plans the next action, finally act out the plan. For example, “I see a door, but there is a chair in between, let me come up with a plan to move around the chairs.” 3 Figure 1: Hierarchical paradigm Thus, all the sensing data is gathered into one global model, and fed into a single representation the planner can use, and then routed to actions. Figure 2 shows another way to look at this paradigm, as a transitive flow of events. This Figure 2: Hierarchical paradigm approach has a strong appeal, because there exist planning strategies that, within a finite amount of time, can compute a sequence of motions that guarantees to accomplish the goal or prove that the given task is impossible, as we discussed in the planning part. Moreover, such a sequence can be optimized before it takes place. Essentially, it looks for a shortest path among a bunch of them. This is significant to a mobile robot, since a carefully figured out plan will guarantee that it will not get into a trap of following a long path to a dead-end. Instead, it will be led along a direct, perhaps, even the shortest, path. On the other hand, this approach does have its problems, mainly inefficiency, as we also discussed for the planning task. Such a strategy sometimes leads to unacceptable time and space complexities because of its complicated environment. It might not be a huge concern for a manipulative-type robot, but it can be huge for a mobile robot, since the latter has to carry everything on its back, just like a turtle. Another issue is that it would have to assume the closed world assumption, i.e., the only information you can use are those you know. For example, when the robot reads 4 the sentence that “A girl touches the boy with a bouquet of flowers”, and has to get the flower. It might not be able to do it because it does not have enough information. Something which is not here is that it is usually the boy who has the flower.... Yet another ssue is that sensory information can be directly fed into the action part, without going through a planning stage. For example, the cognitive psychologists suspect that human beings don’t plan ahead, we simply follow our gut feelings. This leads to the next paradigm. • The reactive paradigm, also referred to as the behavior control approach, is intended to overcome some of the shortcomings of the hierarchical paradigm, and had led to many exciting advances during the 1988-1992 period. The reactive paradigm, as shown in Figure 3 simply deletes the planning stage. It thus assumes that the input to an ACT function will always be the direct output of a SENSE function. Sort of gut feeling. Figure 3: Reactive paradigm More specifically, a robot has multiple instances of SENSE-ACT couplings, which are current processes, called behaviors. Each of them takes local sensing data and computes the best action to take, independent of the other processes. Sometimes, it can combine behaviors. For example, One behavior can direct the robot to “move forward 5 feet” (ACT) to reach a goal (as picked up by its SENSE); while the other can direct it to “turn 90◦” since it sensor fears a collision. In this case, the robot will actually turn 45◦ to avoid the collision, although none of the two behaviors directs it to do so. It quickly became obvious that it is too extreme to completely delete the planing stage. For example, if one ACT in a collection leads to “move forward 5 feet”, while the other says “move back 3 feet”, the robot should combine them to move forward 2 feet; rather than move back and forth. 5 • The hybrid model emerged in the 1990’s and continues to be the current area of robotic research. Under this paradigm, a robot firstly plans how to best decompose a task into subtasks, and then find out the best behaviors to get each of them done. Thus, it can be characterized as PLAN, SENSE-ACT. The sensing data is sent to each behavior that needs them, and is also available to the planner for the construction of a task-oriented global model. It is really a mixture of both paradigms. Figure 4 shows a pictorial presentation of this paradigm. Figure 4: Hybrid paradigm 1.1.3 Behavior control As mentioned earlier, behaviors are simply layers of control systems that all run in parallel, implemented as Java threads, whenever appropriate sensors fire.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages35 Page
-
File Size-